Frontend Interview Prep: JavaScript & TypeScript Basics
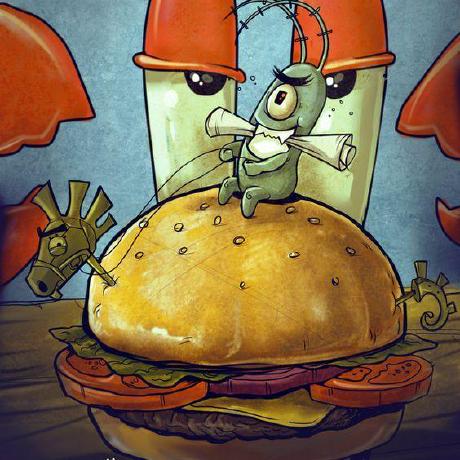
This series looks into what you can expect on an interview for a frontend role. Starting with JavaScript and TypeScript fundamentals.
Topics in the series:
JavaScript & TypeScript Basics [You are here]
React and Next.js
Caching strategies
Testing
Web security
WebSockets & Real-time Communication
Observability
Frontend system design
Trade offs
JavaScript
Fundamental Concepts
To execute JavaScript we need a runtime with an engine, APIs, task queues and an event loop.
The engine is responsible for:
parsing, interpreting and executing synchronous code.
managing memory by keeping a call stack and heap, and running the garbage collector periodically on the heap to clean up memory.
How the engine works: The engine executes synchronous code line by line using a LIFO call stack. When a function is called variables in the lexical scope are pushed onto the stack and when execution finishes, the items are popped out in a Last In-First Out structure.
💡I wrote about memory management in Javascript here 🙂.
The runtime provides APIs and services needed to execute the code such as
DOM
(the window object),Fetch API
,LocalStorage
,setTimeout
,fs
(file system),http
,process
,Buffer
, Array and Object functions such asforEach
, etc.The runtime manages two task queues: the Macrotask queue and the Microtask queue, that are used by the event loop to manage asynchronous code. The Microtask queue has a higher priority than the Macrotask queue.
The event loop acts as a bridge between the call stack and the queues, it moves tasks from the queues to the call stack for execution.
Code execution
JavaScript runs code on a single thread. The engine executes synchronous code sequentially using a Last-In, First-Out (LIFO) call stack. When we run into a function that needs to be executed asynchronousy, e.g. a timeout or a network call, we register it with the runtime.
Some tasks like a timeout will start a timer, and some like a fetch will make the network request and wait for a response.
Once the task is ready (e.g. a network response returns or a timer runs out) the task is added to one of the 2 queues depending on predetermined priority (e.g. a promise would go in the Microtask queue while a timeout would go on the Macrotask queue). The event loop continuously checks the call stack to see if it is empty.
If the call stack is empty, the event loop checks the microtask queue and move tasks from the microtask queue into the call stack to be executed by the JS engine one at a time. Once the microtask queue is empty, the event loop moves one task from the macrotask queue to the call stack, then it checks for new microtasks. This cycle repeats indefinitely.
Sample Q & As
How does JavaScript handle asynchronous code?
JS handles async code using the event loop, the event loop continuously checks the call stack and moves asynchronous tasks from the macrotask queue or microtask queue when the stack is clear. This ensures smooth execution without blocking the main thread.
Asynchronous code is handled using promises. The
.then()
method executes after the promise resolves successfully,.catch()
handles errors, and.finally()
executes regardless of success or failure.What are promises?
An object that represents the eventual completion/failure of an async operation. It has 3 states, pending, fulfilled or rejected.
What is the difference between
async/await
andpromise.then().catch().finally()
?Async/await is syntactic sugar over promises, making asynchronous code more readable and avoiding deeply nested
.then()
chains.How do you handle errors in async/await?
Use try … catch
Use
Promise.all()
(one failure rejects everything)
What is the difference between == and ===?
==
checks for equality of the value,===
checks for equality of the value and typee.g.
"1" == 1 // true "1" === 1 // false
What is the difference between
var
,let
, andconst
?The difference is in scoping, mutability and hoisting of a variable.
var
: is scoped to an entire function and can be redeclared and reassigned.let
: is scoped to only the block{}
it is declared in, it can be reassigned but not redeclared in the same scope; it also doesn’t need to be assigned a value at declaration.const
: is scoped to only the block{}
it is declared in, it cannot be reassigned or redeclared, must be assigned a value at declaration (must be initialized at declaration)
Note, mention: we avoid var
due to scoping issues. We use const by default and let when we need to reassign a variable later.
What is lexical scoping?
Also known as static scoping, a function's scope is determined by where it is declared in the code, not where it is called. Inner functions have access to parent scope but not vice versa.
What is hoisting? How does hoisting work in JavaScript?
Hoisting is JavaScript’s behavior of moving variable and function declarations to the top of their containing scope during compilation. This allows you to use variables and functions before they are declared in the code.
Functions are hoisted with their entire definition and can be called before they appear in code.
var
is hoisted but initialized with undefinedlet
andconst
are hoisted but not initialized, so access to such a variable before declaration will result in a reference error
What is a closure? How do they work?
A closure is a function that has access to variables outside its block scope. e.g.
function someFun() { const name = "John Doe" // this function is a closure because it has access to a variable "name" defined // outside its own block scope function someClosure() { const hello = "Hello, " + name console.log(hello) } }
Note, mention: closures can cause memory leaks if not handled properly. e.g they can keep references to large objects that are no longer needed, preventing garbage collection.
What is a memory leak?
A memory leak occurs when memory that is no longer needed is not released, preventing the garbage collector from reclaiming it. Common causes include global variables, unclosed event listeners, and unreferenced DOM elements.
What are the different ways to clone an object in JavaScript?
Shallow copy: copies first level properties, doesn’t handle nested objects. Nested objects are still referenced, meaning changes affect the original.
- e.g. using the spread operator (…) or Object.assign
Deep copy: fully clones nested objects & arrays
- e.g. using lodash deepClone, JSON methods parse & stringify
How does JavaScript handle primitive vs. reference types?
Primitive types are immutable and stored directly in memory (stored by value) e.g. string, number, boolean etc
Reference types are stored on the heap and hold a hold a reference to the memory address instead of the actual value (stored by reference) e.g. arrays, objects, functions
What happens when you compare objects in JavaScript?
Objects are compared by reference, not by value. Even if two objects have the same properties and values, they are considered different unless they reference the same memory location.
Note, mention: To compare objects by value, use
JSON.stringify()
or Lodash_.isEqual()
for deep comparison.What is
this
keyword?The
this
keyword refers to the execution context, meaning what object is currently executing the function.Note, mention: Arrow functions do not have their own
this
. Instead, they inheritthis
from the surrounding scope at the time they are defined. In a class,this
refers to an instance of the class.How does
fetch()
differ fromXMLHttpRequest
?Both
fetch()
andXMLHttpRequest
(XHR
) are used to make HTTP requests in JavaScript, butfetch()
is newer, more modern, and promise-based, whileXHR
is older and callback-based.How does JavaScript handle memory management and garbage collection?
Using a LIFO call stack to store primitive types and a heap to store reference types that is regularly cleaned up automatically by the garbage collector
What is prototypal inheritance in JavaScript?
A prototype is a built-in mechanism that enables inheritance and shared properties between objects. Every function and object in JavaScript has a hidden property called
prototype
, which allows objects to inherit methods and properties from other objects.
TypeScript
Fundamental concepts
Typescript is a superset of JavaScript built by Microsoft. It builds on JavaScript by adding static type definition.
TypeScript types
Primitive types - these are basic types
boolean
,number
,string
,void
,undefined
,null
Object types - represents any non-primitive type e.g.
object
,array
,function
,interface
,class
,enum
,tuple
Top types - can hold any value.
any
: the most flexible type but comes with a cost — it disables type checking. Used when we don’t want a particular value to cause typechecking errors.unknown
: Likeany
, it can hold any value, but you cannot perform operations on it until you explicitly check its type. It forces you to do type checks before operating on the value, making it a more type-safe alternative toany
.
Bottom types - they represent the absence of any value. They sit at the bottom of the type hierarchy.
never
: represents values that never occur. It's used in scenarios where something is impossible to happen.
Combining types
We can create new types by combining types using:
unions
|
- assigning multiple possible types for a single variableintersections
&
- combines 2 exisiting types
Generics
Generics are used to create reusable dynamic types e.g.
// without generics
function printStuff(s: string, t: string): string {
const newST = s + t
console.log(newST)
return newST
}
printStuff("Casa", "Blanca")
function printStuff2(s: number, t: number): number {
const newST = s + t
console.log(newST)
return newST
}
printStuff(1,2)
// with generics
function printStuffWithGenerics<T>(s: T, t: T): T {
const newST = s + t
console.log(newST)
return newST
}
printStuffWithGenerics<string>("Casa", "Blanca")
printStuffWithGenerics<number>(1,2)
Utility types
Utility types are built-in generic types that help manipulate and transform types in TypeScript
Partial
- Makes all properties optionalRequired
- Makes all properties requiredReadonly
- Makes all properties read-onlyPick
- Selects specific properties from a typeOmit
- Removes specific properties from a typeRecord
- Creates an object type with specific keys and value typesExclude
- Removes a type from a uniontype Status = "active" | "pending" | "archived"; type ActiveStatus = Exclude<Status, "archived">; // "active" | "pending"
Extract
- Keeps only matching types in a uniontype Status = "active" | "pending" | "archived"; type ActiveStatus = Exclude<Status, "archived">; // "active" | "pending"
NonNullable
- Removesnull
andundefined
from a typeReturnType
- Gets the return type of a function
Type Inferencing
Typescript can infer types from the value assigned to a variable. The TypeScript compiler will ensure that you don’t perform any invalid operations on it.
Type guards
Type guards are used to determine the type of a value at runtime
instanceof
: checks if an object is an instance of a classtypeof
: used to check the type of a variabletype predicates: Type predicates are functions that return a boolean value e.g.
isString(x)
Type assertions
Type assertions override TypeScript’s inferred type. They allow us to explicitly tell the compiler the type we want to resolve to, overriding the static type checking behavior using as
and as const
e.g.
let value: string | number = "Hello, TS!";
let strLength: number = (value as string).length;
const pronouns = ["me", "you", "us"] as const;
// TypeScript infers: readonly ["me", "you", "us"]
pronouns.push("it"); // ❌ Error: Property 'push' does not exist on type 'readonly ["me", "you", "us"]'
Sample Q & As
What is type inferencing?
TS infers the type of a variable from the assigned value
What is the difference between an interface and a type?
Both
interface
andtype
in TypeScript allow you to define the shape of an object.An
interface
is primarily used to define object structures and supports extension via inheritance. Can be used to enforce certain implementations on classes.A
type
can represent objects, primitives, unions, intersections, and tuples. Supports unions and intersections. Doesn’t support inheritance.
How do you define an optional property in an interface?
Using a
?
Explain the difference between
unknown
andany
. When would you use each?unknown
can hold any value, but you cannot perform operations on it until you explicitly check its type;any
can hold any value, but disables type checking.What are
readonly
properties, and how do they work in TypeScript?immutable properties that cannot be reassigned, just read
How can you create a utility type that removes specific properties from an object?
Using
Omit
,Extract
,Exclude
orNonNullable
Explain function overloading in TypeScript with an example.
defining multiple signatures for a function while maintaining a single implementation.
What are conditional types, and how do they work?
Conditional types allow you to create dynamic types based on conditions using the ternary syntax.
T extends U ? X : Y
How can you ensure strict null checks in your TypeScript project?
enable strict null checking in
tsconfig.json
Note, mention: Strinct null check prevents assigning
null
orundefined
to values unless explicitly allowed.What’s the difference between a void, null and undefined?
null
- represents intentional absence of a valuevoid
- for a function returns nothingundefined
- represents missing value
Practice solving problems on:
Leet code: https://leetcode.com/
Coding Dojo: https://codingdojo.org
Subscribe to my newsletter
Read articles from Shalon N. Ngigi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
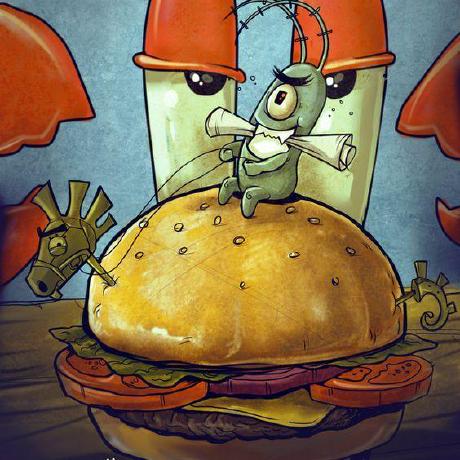