Left Join Coming to .NET 10

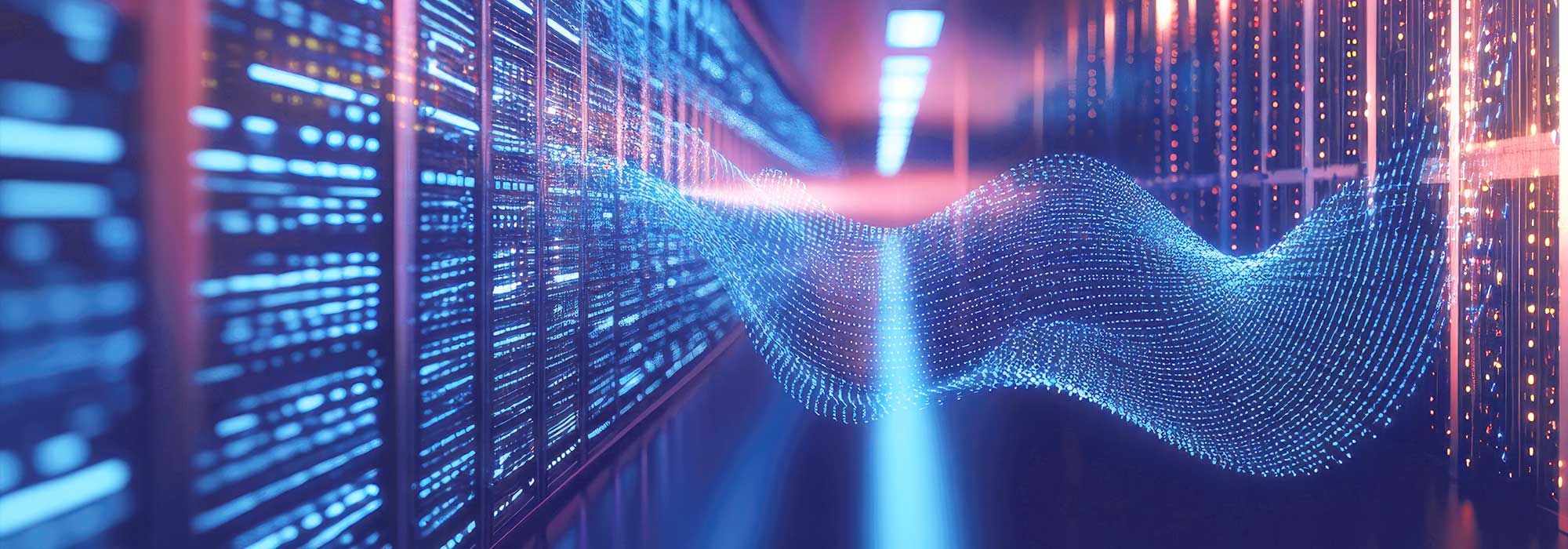
.NET 10 is looking to be an exciting release, bringing new enhancements to LINQ, including the much-anticipated Left Join support. This feature simplifies querying operations, making data manipulation more intuitive and efficient.
A Left Join is a type of SQL join that returns all records from the left table and the matched records from the right table. If there’s no match, NULL values are returned for the right table’s columns. This is particularly useful when dealing with data that might not have corresponding entries in another collection.
How Left Join Works in LINQ (Before .NET 10)
Prior to .NET 10, achieving a Left Join in LINQ required workarounds using GroupJoin
followed by SelectMany
, or using the DefaultIfEmpty()
method. Here’s how you would do it:
var leftJoinResult = from customer in customers
join order in orders on customer.Id equals order.CustomerId into orderGroup
from order in orderGroup.DefaultIfEmpty()
select new
{
CustomerName = customer.Name,
OrderId = order?.Id,
OrderTotal = order?.Total
};
This method works but is a bit verbose and unintuitive compared to SQL’s LEFT JOIN
.
The New Left Join in .NET 10
With .NET 10, Left Join will have first-class support in LINQ, making queries cleaner and more readable. The new syntax will resemble something like this:
var leftJoinResult = customers.LeftJoin(
orders,
customer => customer.Id,
order => order.CustomerId,
(customer, order) => new
{
CustomerName = customer.Name,
OrderId = order?.Id,
OrderTotal = order?.Total
});
Real-World Use Case
Let’s say you’re building a customer dashboard where you want to list all customers and their most recent orders. Even customers with no orders should be displayed. The new Left Join simplifies this query significantly:
var customerOrders = customers.LeftJoin(
orders,
c => c.Id,
o => o.CustomerId,
(c, o) => new
{
c.Name,
OrderId = o?.Id,
OrderDate = o?.Date
});
This is much cleaner and provides better clarity compared to earlier LINQ workarounds.
The Left Join feature in .NET 10 is a significant step forward for LINQ. It brings SQL-style joins to C# in a more intuitive way, improving both developer experience and code efficiency. Whether you're working with databases, in-memory collections, or API data, this new feature is a game-changer.
Subscribe to my newsletter
Read articles from Patrick Kearns directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
