Day 9: JavaScript this Keyword – How It Works & Common Mistakes 🚀

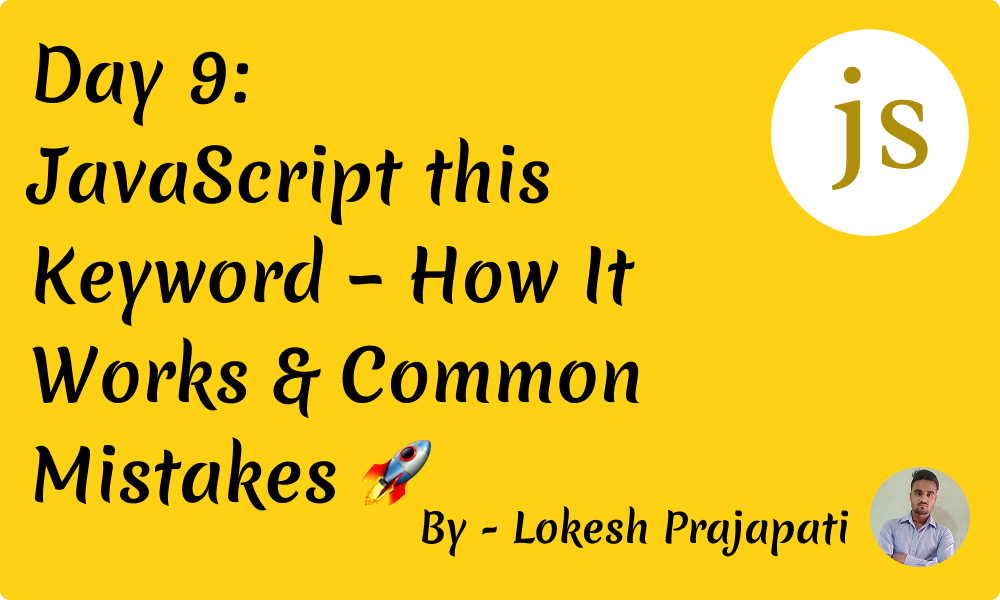
Introduction
Welcome, guys! Today, we are diving deep into one of the most important concepts in JavaScript — the this
keyword. Many developers struggle with it, but by the end of this lesson, you’ll have a solid understanding of how it works, when to use it, and how to avoid common mistakes. Let’s get started! 🚀
What is "this"
in JavaScript?
In JavaScript, this
is a special keyword that refers to the context in which a function is executed. The value of this
depends on how and where the function is called.
Think of this
as a pointer that changes based on the execution context.
How this
Works in Different Scenarios
1️⃣ Global Context
When this
is used in the global scope, it refers to the global object.
console.log(this); // In browsers, it refers to the `window` object
🔹 In the browser, this
refers to window
.
🔹 In Node.js, this
refers to an empty object ({}
) in the module.
2️⃣ Inside an Object Method
When this
is used inside an object method, it refers to the object that owns the method.
const person = {
name: "Lokesh",
greet: function () {
console.log("Hello, my name is " + this.name);
},
};
person.greet(); // Output: Hello, my name is Lokesh
✅ Since greet()
is called on person
, this
refers to person
.
3️⃣ In a Constructor Function
When this
is used inside a constructor function, it refers to the newly created object.
function Person(name) {
this.name = name;
}
const user = new Person("Lokesh");
console.log(user.name); // Output: Lokesh
✅ this
refers to the instance of Person
that is created.
4️⃣ Arrow Functions & this
Arrow functions do not have their own this
. Instead, they inherit this
from their surrounding lexical scope.
const person = {
name: "Lokesh",
greet: () => {
console.log("Hello, my name is " + this.name);
},
};
person.greet(); // Output: Hello, my name is undefined
🚨 Why? Because arrow functions take this
from their surrounding scope, which in this case is the global object.
✅ Fix: Use a regular function instead of an arrow function:
greet: function() {
console.log("Hello, my name is " + this.name);
}
5️⃣ this
Inside an Event Listener
When a function is used as an event listener, this
refers to the element that received the event.
document.getElementById("btn").addEventListener("click", function () {
console.log(this); // Refers to the button element
});
✅ Here, this
refers to the button that was clicked.
6️⃣ this
in setTimeout()
By default, when using setTimeout()
, this
refers to the global object (window
in browsers).
const person = {
name: "Lokesh",
greet: function () {
setTimeout(function () {
console.log("Hello, " + this.name);
}, 1000);
},
};
person.greet(); // Output: Hello, undefined
🚨 Why? The function inside setTimeout()
is not called as a method of person
, so this
becomes window
.
✅ Fix: Use an arrow function.
setTimeout(() => {
console.log("Hello, " + this.name);
}, 1000);
✅ Arrow functions inherit this
from greet()
's execution context.
Common Mistakes and How to Avoid Them
❌ 1. Losing this
in a Callback
const person = {
name: "Lokesh",
greet: function () {
setTimeout(function () {
console.log("Hello, " + this.name);
}, 1000);
},
};
person.greet(); // Output: Hello, undefined
✅ Fix: Use .bind(this)
.
setTimeout(function () {
console.log("Hello, " + this.name);
}.bind(this), 1000);
❌ 2. Using Arrow Functions Incorrectly
const person = {
name: "John",
greet: () => {
console.log("Hello, " + this.name);
},
};
person.greet(); // Output: Hello, undefined
✅ Fix: Use a regular function.
greet: function() {
console.log("Hello, " + this.name);
}
When to Use this
🔹 Use this
in objects and classes to refer to the current instance.
🔹 Avoid this
in nested functions unless you use .bind()
or arrow functions.
🔹 Remember arrow functions inherit this
from their surrounding scope.
Conclusion 🎯
Understanding this
is crucial for mastering JavaScript. The key takeaway is that this
depends on how a function is called, not where it is defined.
🚀 Recap: ✅ this
refers to different objects in different contexts. ✅ Arrow functions don’t have their own this
. ✅ Always be mindful of how a function is executed.
Have you ever faced issues with this
? Drop a comment below and let’s discuss! 🎉
Subscribe to my newsletter
Read articles from Lokesh Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lokesh Prajapati
Lokesh Prajapati
🚀 JavaScript | React | Shopify Developer | Tech Blogger Hi, I’m Lokesh Prajapati, a passionate web developer and content creator. I love simplifying JavaScript, React, and Shopify development through easy-to-understand tutorials and real-world examples. I’m currently running a JavaScript Basics to Advanced series on Medium & Hashnode, helping developers of all levels enhance their coding skills. My goal is to make programming more accessible and practical for everyone. Follow me for daily coding tips, tricks, and insights! Let’s learn and grow together. 💡🚀 #JavaScript #React #Shopify #WebDevelopment #Coding #TechBlogger #LearnToCode