Implementing Server-Side Rendering (SSR) with Spring Boot
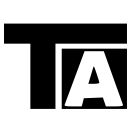
4 min read
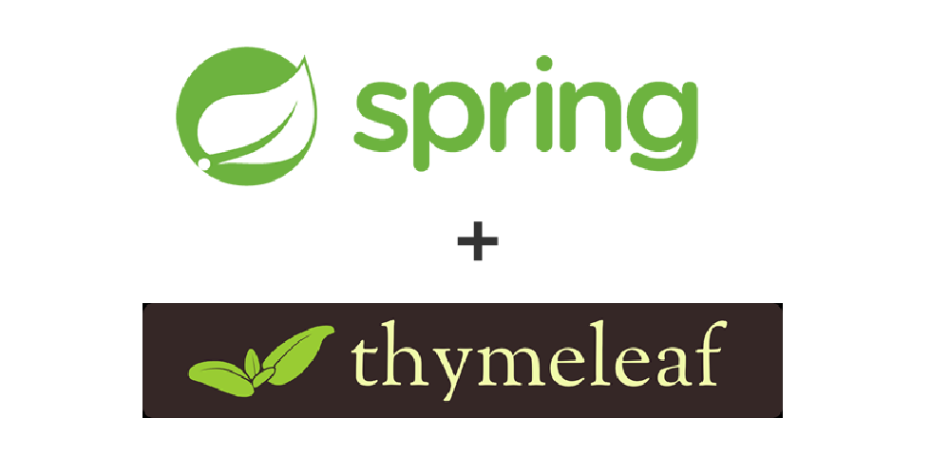
1. What is Server-Side Rendering (SSR)?
Server-Side Rendering refers to the process of rendering web pages on the server instead of the browser. When the server processes a request, it generates an HTML file that is sent to the client's browser, where the content is displayed immediately. This approach contrasts with client-side rendering, where JavaScript fetches data and renders it in the browser.
2. Setting Up Server-Side Rendering in Spring Boot
Now, let’s dive into how to implement SSR using Spring Boot. We'll use Thymeleaf, a powerful server-side templating engine that integrates seamlessly with Spring Boot to render dynamic HTML on the server.
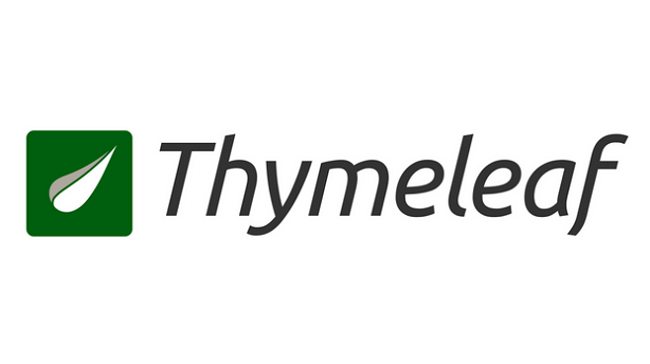
2.1 Spring Boot and Thymeleaf Setup
To get started, you need to include the necessary dependencies in your Spring Boot project. If you’re using Maven, add the following dependencies to your pom.xml:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Thymeleaf enables us to write dynamic HTML pages that the server will process before sending the final HTML to the client.
2.2 Creating the Controller
Next, we need to create a Spring Boot controller that will handle HTTP requests and return the server-rendered HTML. Here’s a basic example of a controller:
@Controller
public class HomeController {
@GetMapping("/home")
public String home(Model model) {
model.addAttribute("message", "Welcome to Server-Side Rendering with Spring Boot!");
return "home";
}
}
In this example, the home method processes GET requests for /home and adds a message to the model. The home.html view will be rendered server-side and sent to the client.
2.3 Creating the View Template
Next, create the Thymeleaf view in the src/main/resources/templates/ directory. The home.html file might look like this:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Server-Side Rendering with Spring Boot</title>
</head>
<body>
<h1 th:text="${message}"></h1>
</body>
</html>
When a user visits /home, the server will generate an HTML page with the message "Welcome to Server-Side Rendering with Spring Boot!" embedded directly into the HTML.
2.4 Running the Application and Viewing Results
Run the Spring Boot application and navigate to http://localhost:8080/home. You’ll see the rendered HTML page:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Server-Side Rendering with Spring Boot</title>
</head>
<body>
<h1>Welcome to Server-Side Rendering with Spring Boot!</h1>
</body>
</html>
The key point here is that this HTML is generated on the server and sent directly to the browser, allowing the page to be rendered immediately without relying on client-side JavaScript.
3. Advanced Techniques for Server-Side Rendering in Spring Boot
Let’s now explore some advanced techniques to optimize SSR in Spring Boot.
3.1 Using Conditional Rendering in Thymeleaf
Thymeleaf supports powerful conditional rendering capabilities, allowing us to dynamically include or exclude sections of HTML based on server-side logic. For example:
<p th:if="${isLoggedIn}">Welcome back, user!</p>
<p th:unless="${isLoggedIn}">Please log in to continue.</p>
In this example, the message displayed depends on whether the isLoggedIn attribute is true or false.
3.2 Rendering Lists with Thymeleaf
Another useful technique is rendering lists of data. Imagine you have a list of products you want to display:
@GetMapping("/products")
public String products(Model model) {
List<String> productList = Arrays.asList("Product A", "Product B", "Product C");
model.addAttribute("products", productList);
return "products";
}
And in the products.html template, you can loop through the list and display the items:
<ul>
<li th:each="product : ${products}" th:text="${product}"></li>
</ul>
When visiting /products, the server renders the full HTML with the product list, which the browser displays immediately.
4. Conclusion
Implementing server-side rendering with Spring Boot offers several advantages, especially in terms of SEO and user experience. By leveraging Spring Boot’s integration with Thymeleaf, we can efficiently render dynamic HTML on the server, delivering content-rich web pages to clients with minimal load times.
However, SSR also comes with challenges, particularly in managing server load and handling complex state synchronization between server and client. Understanding these trade-offs will help you decide if SSR is the right choice for your project.
If you have any questions or would like to discuss further, feel free to leave a comment below! I’d be happy to help clarify any concepts or provide additional examples.
Read more at : Implementing Server-Side Rendering (SSR) with Spring Boot
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
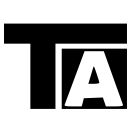
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.