JavaScript’s Data Type Questions #3

Table of contents
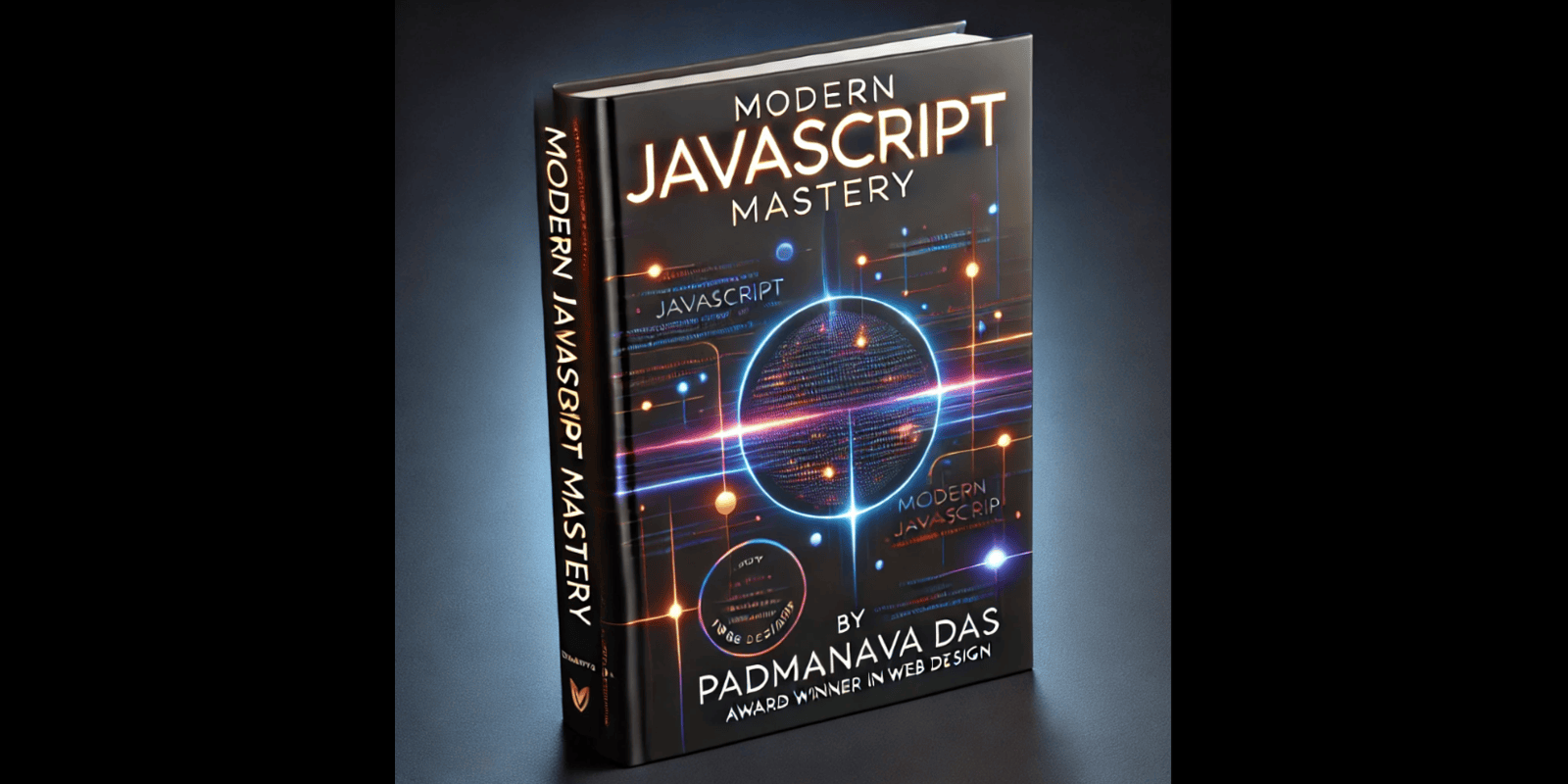
Hi Friends!
Kamon acho!
Q: What does it mean that JavaScript is dynamically typed?
Ans: In JavaScript, you can assign a value of one type to a variable and later assign a different type.
let name = "Padmanava";
name = 42; // No error
Number & BigInt
Q: What is the safe integer range in JavaScript?
Ans: JavaScript’s Number
type has a safe range from -(2^53 - 1)
to (2^53 - 1)
.
If you need larger numbers, use BigInt
.
let bigNum = 9007199254740991n; // BigInt
NaN (Not-a-Number)
Q: What happens when you perform arithmetic with NaN?
Ans: Any arithmetic operation involving NaN
results in NaN
, except NaN ** 0
, which is 1
.
console.log(NaN + 5); // NaN
console.log(NaN * 2); // NaN
console.log(NaN / 2); // NaN
console.log(NaN ** 0); // 1
Template Literals ( )
Q: What makes template literals better than regular strings?
Ans: They allow multi-line strings, variable interpolation using ${}
, and are more modern than ""
or ''
.
let name = "Padmanava";
console.log(`Hello, ${name}!`); // "Hello, Padmanava!"
null
vs undefined
Q: How are null
and undefined
different?
Ans: null
is an intentional absence of a value, while undefined
means a variable is declared but not assigned.
let a = null;
let b;
console.log(a); // null
console.log(b); // undefined
Equality Operators (==
vs ===
)
Q: How does ==
differ from ===
?
Ans: ==
compares values without checking types, while ===
checks both values and types.
console.log(5 == "5"); // true (loose comparison)
console.log(5 === "5"); // false (strict comparison)
🎭 The typeof
Operator
Q: What is the result of typeof null
?
Ans: It returns "object"
due to an old JavaScript bug that was never fixed.
console.log(typeof null); // "object"
Boolean Conversion in Arithmetic
Q: How do true
and false
behave in arithmetic?
Ans: true
→ 1
and false
→ 0
.
console.log(5 + true); // 6 (true → 1)
console.log(5 - false); // 5 (false → 0)
console.log(true + true); // 2
String & Number Operations
Q: When does +
perform concatenation instead of addition?
Ans: If at least one operand is a string, +
concatenates. Otherwise, it performs addition.
console.log(5 + "5"); // "55" (string concatenation)
console.log(5 - "5"); // 0 (number subtraction)
null
and undefined
in Arithmetic
Q: How do null
and undefined
behave in arithmetic?
Ans: null
converts to 0
, while undefined
converts to NaN
.
console.log(5 + null); // 5 (null → 0)
console.log(5 + undefined); // NaN (undefined → NaN)
Final Takeaways
✔ JavaScript is dynamically typed, meaning variable types can change.
✔ null
is intentional emptiness, undefined
means uninitialized.
✔ ==
checks values, ===
checks values and types.
✔ NaN
propagates in math, but NaN ** 0 === 1
.
✔ typeof null === "object"
is an old JavaScript quirk.
✔ +
concatenates if a string is involved, otherwise it performs addition.
✔ Boolean values convert to numbers in arithmetic (true
→ 1, false
→ 0).
Subscribe to my newsletter
Read articles from Padmanava Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Padmanava Das
Padmanava Das
From Republic of Bharat