{Scope{Scope{Scope}}} <=> Closures
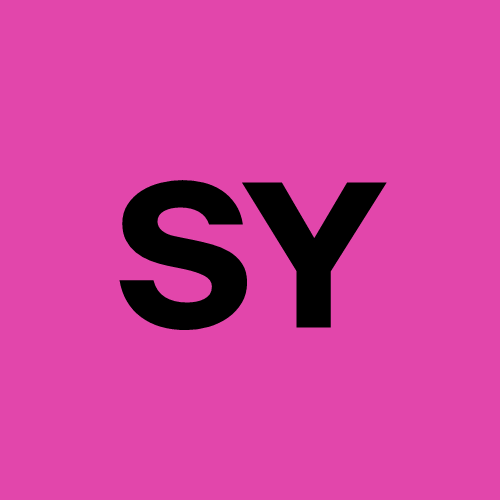
The Scope in JavaScript refers to the environment, context, or the lexical boundary of the function in which the variable is held.
Types of Scopes:
Local Scope
Global Scope
Global Scope: Variables which are declared outside the function are termed as global. These
are accessible anywhere in the script.
Local Scope: Variables which are declared inside the function are called as Local variables and these take precedence over the global variable in the local environment if they have the same name. These local variables or their values are not accessible from the global space, that is, outside of the function in which they are declared.
// Global variable
let message = "I am a global variable";
function testScope() {
// Local variable with the same name
let message = "I am a local variable";
console.log(message); // This will print the local variable value
}
testScope();
console.log(message); // This will print the global variable value
//Expected output
I am a local variable
I am a global variable
What is a Lexical Scope :
Lexical Scope refers to the location of a variable inside a function or the boundary to which it has effects and access. We have 4 kinds of Lexical scopes Global , Local , Nested , Block.
Closures :
Closures are nothing but functions which are bundled along with their lexical scopes.
let a = 10;
function outer(){
console.log(a); // this will print 100 not 10 as the value
a=7; //changed in the global Scope.
function inner(){
console.log(a); //this will print 7;
}
inner();
}
a=100;
let z = outer();
console.log(z); // this will print a as 7 because the inner function remebers
//the lexical scope of a which is the local scope.
Kindly refer to the code above.
function greet(name) {
// `name` is a variable from the outer function
return function() {
console.log("Hello, " + name); // Inner function accessing outer function's
// variable `name`
};
}
const greetPerson = greet("Alice"); // `greet("Alice")` returns the inner function
greetPerson(); // Output: "Hello, Alice"
We are able to print the value of a variable outside of the outer function and the inner function
remembers the lexical scope of a even when it is called from the outside using z;
For closures, remember that every function remembers all the lexical scopes of the variables used in
it has both local scope as well as its global scope through references of these variables.
Subscribe to my newsletter
Read articles from Satish Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
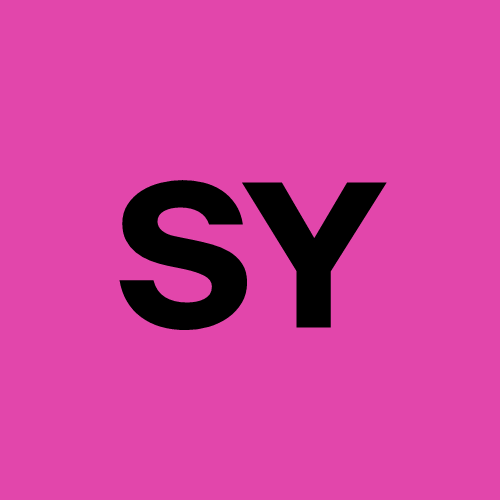