30 Ways to Write More Pythonic Code and Improve Your Job Readiness (part 3 of 6)

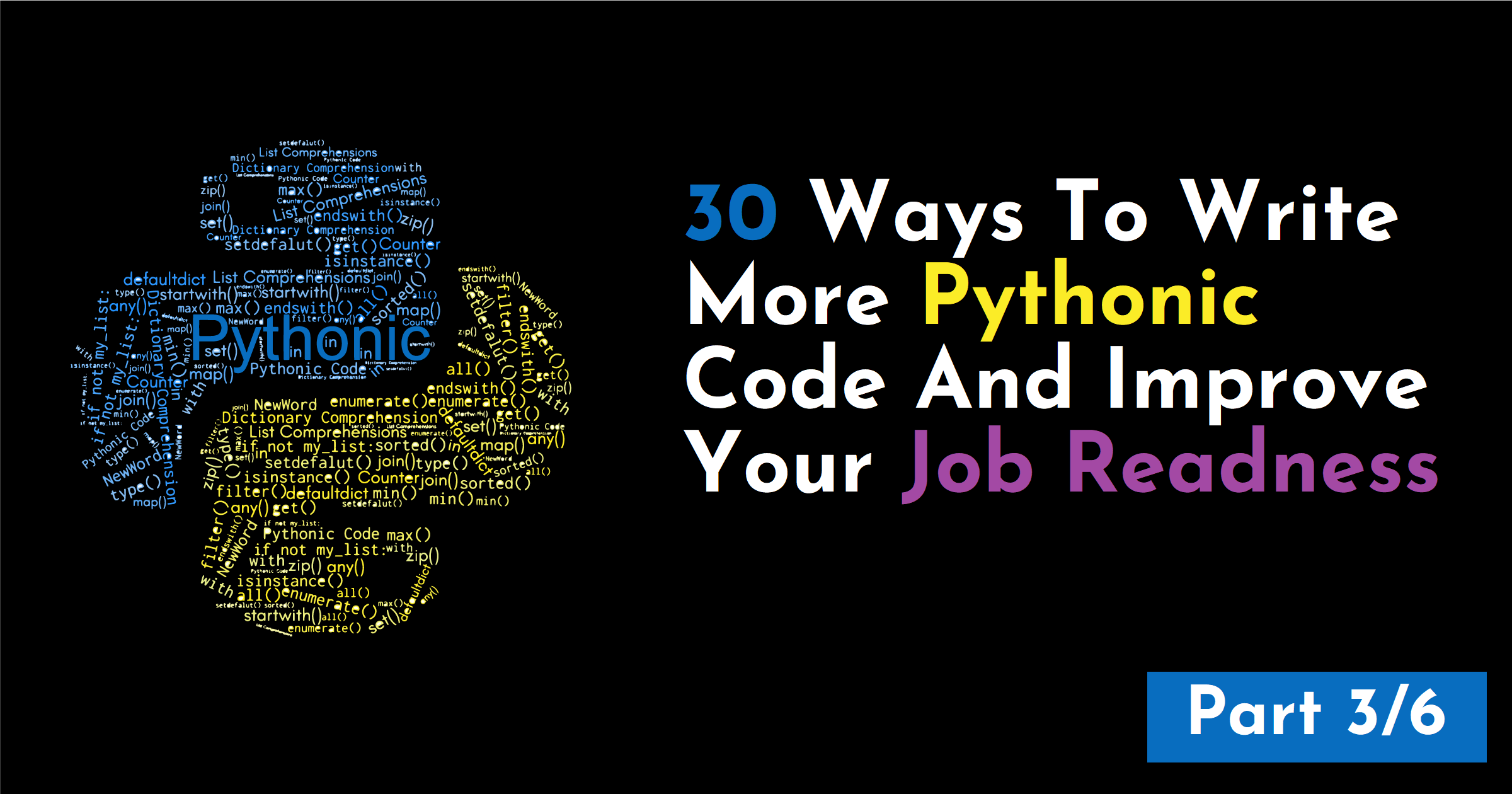
Python is known for its readability, simplicity, and elegance. But writing code that merely works isn’t enough—writing Pythonic code is what sets great Python developers apart. If you're looking to refine your coding skills and prepare for job interviews at top tech companies, mastering Pythonic coding patterns will give you a significant advantage.
In this third part of our analysis, we extend our evaluation by incorporating benchmarks with varying input sizes to compare the efficiency of Pythonic and non-Pythonic techniques more comprehensively. By analyzing execution times across different scales, we gain deeper insights into the trade-offs between readability, maintainability, and performance.
Missed part 1? Check it out!
Missed part 2? Check it out!
11. Using any()
and all()
Instead of Loops for Conditions
Non-Pythonic
has_positive = False
for num in numbers:
if num > 0:
has_positive = True
break
Pythonic
has_positive = any(num > 0 for num in numbers)
Why?
any()
stops at the firstTrue
value, making it more efficient.More concise and expressive.
How it works?
In the Pythonic approach, the built-in any()
function is used. It evaluates a generator expression that checks if any number in numbers
is greater than 0
. As soon as any()
finds a True
value, it short-circuits and stops further evaluations, making the code cleaner and more efficient.
Time Complexity
Both approaches have a worst-case time complexity of O(n)
and a best-case complexity of O(k)
, where k
is the index of the first positive number.
The loop with a break
is slightly faster because it avoids the function call overhead associated with any()
. The any()
function uses a generator expression, which is evaluated lazily but still involves calling the built-in function. However, in terms of readability and maintainability, any()
is the preferred approach since it is more expressive and directly conveys intent.
Benchmarking
12. Using sorted()
Instead of Sorting a List in Place
Non-Pythonic
sorted_list = my_list[:] # copy the original list to a new list
sorted_list.sort() # modifies sorted_list
Pythonic
sorted_list = sorted(my_list) # does not modify original list and creates a new sorted_list
Why**?**
sorted()
returns a new list, preserving the original list.More functional and less prone to unintended side effects.
Useful when needing both the sorted and original versions.
How it Works?
In the non-Pythonic approach, the original list is first copied using slicing (my_list[:]
) to ensure that the sorting operation does not alter the original data. Then, the .sort()
method is applied to the copied list, modifying it in place. This approach ensures that the original list remains unchanged but requires an explicit copy operation before sorting. In contrast, the Pythonic approach utilizes the built-in sorted()
function, which creates and returns a new sorted list while leaving the original list intact. Since sorted()
performs the copying and sorting in one step, it results in cleaner and more expressive code. Additionally, sorted()
supports optional parameters such as key
for custom sorting and reverse=True
for descending order, making it more flexible.
Time Complexity
Both approaches utilize Timsort, which results in an average and worst-case time complexity of O(n log n)
. However, there is a key difference in memory usage. The non-Pythonic approach first creates a copy of the list in O(n)
time and space before sorting it in place in O(n log n)
, leading to an overall space complexity of O(n)
. The Pythonic approach with sorted()
also has a space complexity of O(n)
since it generates a new sorted list, but it avoids the two-step process of copying and sorting separately, making it more streamlined. sorted()
can sometimes perform better due to optimized memory allocation and better cache locality.
Benchmarking
13. Using defaultdict
to Handle Missing Keys
Non-Pythonic
word_counts = {}
for word in words:
if word in word_counts:
word_counts[word] += 1
else:
word_counts[word] = 1
Pythonic
from collections import defaultdict
word_counts = defaultdict(int)
for word in words:
word_counts[word] += 1
Why?
defaultdict(int)
initializes missing keys automatically.Avoids explicit
if
conditions, making the code cleaner.
How it works?
The defaultdict
class from the collections
module simplifies dictionary operations by automatically assigning default values to missing keys. In this case, defaultdict(int)
initializes any missing key with 0
, eliminating the need for explicit condition checks. As words are iterated through, their corresponding counts are updated seamlessly, resulting in cleaner and more efficient code.
Time Complexity
Both the standard dictionary approach and defaultdict
have a time complexity of O(n)
, where nnn is the number of words processed. However, defaultdict
is slightly faster since it eliminates redundant dictionary lookups when checking for key existence before updating values. By removing these extra operations, defaultdict
reduces overhead and enhances performance in high-frequency dictionary operations.
Benchmarking
The difference is minor, but defaultdict
is slightly faster because it eliminates the explicit check for key existence. In the non-Pythonic approach, the if word in word_counts:
condition incurs an additional dictionary lookup, which adds to the overhead. While defaultdict
does introduce a small function call overhead, the efficiency gain from avoiding redundant lookups makes it slightly faster.
14. Using max()
and min()
Instead of Loops
Non-Pythonic
max_value = numbers[0]
for num in numbers:
if num > max_value:
max_value = num
Pythonic
max_value = max(numbers)
Why?
max()
is optimized and much faster than a loop.Cleaner and more readable.
How it works?
Instead of manually iterating through a list to find the maximum or minimum value, the built-in max()
and min()
functions efficiently perform this operation internally. These functions traverse the list once and return the highest or lowest value, respectively. They also support a key
argument, allowing for custom comparison logic when working with complex objects or nested data.
Time Complexity
Both approaches have a time complexity of O(n)
since they require scanning the entire list once to determine the maximum or minimum value. While the manual loop and max()
perform the same number of comparisons, max()
is generally more optimized due to its internal implementation in C, reducing the overhead associated with explicit loops in Python.
Benchmarking
15. Using map()
Instead of a Loop for Transformation
Non-Pythonic
squared = []
for x in numbers:
squared.append(x ** 2)
Pythonic
squared = list(map(lambda x: x ** 2, numbers))
Why?
map()
is optimized in C and often more efficient.Can improve readability in some cases.
(However, list comprehensions are usually preferred over map()
).
How it works?
The map()
function applies a transformation function to each element of an iterable, producing a map object, which can then be converted into a list. In this example, map(lambda x: x ** 2, numbers)
applies the squaring operation to every number in the list without the need for an explicit loop. This approach is more concise, avoids manual iteration, and leverages Python’s built-in optimizations.
Time Complexity
Both the manual loop and map()
have a time complexity of O(n) because each approach processes every element in the list exactly once. However, their actual performance differs due to execution overhead. The loop iterates through the list and appends squared values, benefiting from Python’s internal list resizing optimizations. map()
, on the other hand, applies a function call to each element, which introduces additional overhead, especially when using a lambda function. Since function calls in Python are relatively expensive, this can make map()
slower than a direct loop in some cases. Despite map()
being implemented in C, its repeated function calls often negate its expected speed advantage.
Benchmarking
Time Complex And Bechmarking Analisys
Technique | Time Complexity (Non-Pythonic) | Time Complexity (Pythonic) | Best Approach |
Using any() for condition checking | O(n) (best case O(k)) | O(n) (best case O(k)) | Non-Pythonic (slightly faster - avoids the function call overhead) |
Using sorted() for sorting | O(n log n) | O(n log n) | Pythonic (avoids manual copying) |
Using defaultdict for counting | O(n) | O(n) | Pythonic (avoids redundant checks and improves efficiency) |
Using max() for finding maximum | O(n) | O(n) | Pythonic (more optimized due to C implementation) |
Using map() for transformation | O(n) | O(n) | Non-Pythonic (map() can be slower due to function calls) |
Conclusion
The analysis of Pythonic and non-Pythonic approaches to common programming patterns highlights a critical balance between readability, maintainability, and performance. While Pythonic techniques often improve code expressiveness and clarity, their impact on execution speed varies depending on implementation details.
Short-circuiting with
any()
demonstrates improved code clarity but comes with a slight function call overhead. In cases where performance is paramount, a manual loop with abreak
may still be preferable.Sorting with
sorted()
offers a more functional approach compared to in-place.sort()
, reducing the risk of unintended side effects while maintaining the same time complexity.Using
defaultdict
simplifies dictionary operations by eliminating redundant checks, providing both cleaner code and minor performance gains.The built-in
max()
function showcases the advantage of Python's internal optimizations, outperforming a manual loop due to its C-based implementation.The
map()
function presents an interesting trade-off. While it aligns with functional programming principles, its repeated function call overhead may make list comprehensions or explicit loops the better choice in many scenarios.
While Pythonic and non-Pythonic approaches generally share the same time complexity, C-based optimizations in functions like max()
and sorted()
often make Pythonic methods more efficient, whereas map()
may suffer from function call overhead. The choice between the two depends on context—Pythonic methods enhance readability and maintainability, making them ideal for collaborative projects, while non-Pythonic techniques may offer slight performance advantages in critical scenarios. Understanding these trade-offs allows developers to balance efficiency and code clarity for optimal software design.
See you in the 4th article of a series of 6.
Subscribe to my newsletter
Read articles from Leo Bcheche directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
