Polyfills - Making life easy in Javascript

Table of contents
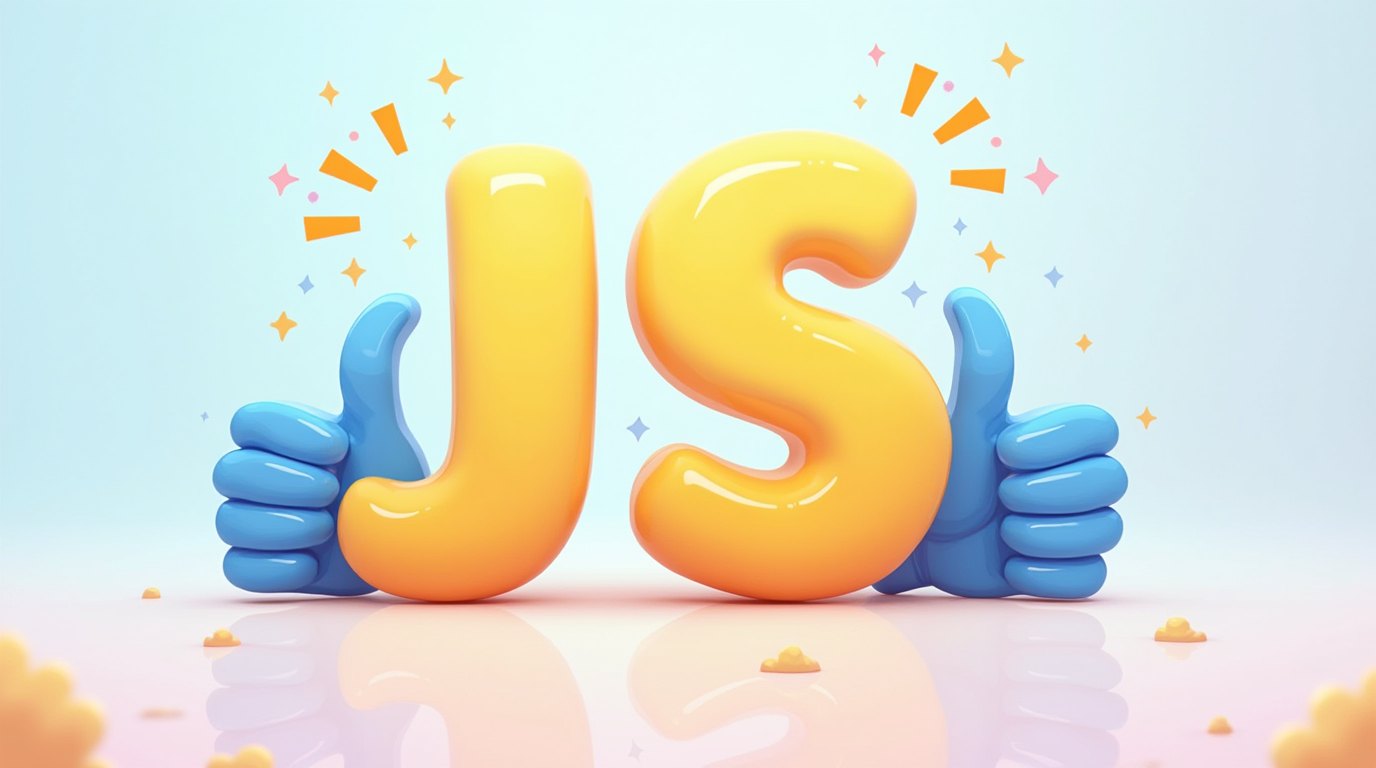
On a random Sunday morning, while sipping your cappuccino, gazing at a beautiful grey Indian cuckoo bird, you reminisce about the old days with your friends who are now in Canada. You decide to pack your bags and give them a surprise visit by taking the first flight from India to Canada. You land at the airport and decide to book a cab to travel to your buddy’s place. However, while accessing your mobile phone, you realize the battery is at 2% and about to die soon. Thankfully, you have an adapter in hand which safely converts the electricity current at the Canadian airport for your Indian mobile consumption.
Similarly to the adapter, polyfills are used to give functionality to a browser which it was lacking. A polyfill is any piece of code, whether a function, object, or snippet, that provides functionality missing in a browser, mimicking how that feature would work if it were natively supported. The technical definition from MDN web docs is “A polyfill is a piece of code (usually JavaScript on the Web) used to provide modern functionality on older browsers that do not natively support it.” Polyfill acts like a "converter" to make something compatible with an environment it wasn’t originally designed for. Therefore, it "fills in" the gaps in the browser’s capabilities, much like your converter adapts the charger to the new environment.
Examples
Let’s make a polyfill that already exists to understand how they actually work. There is an array method called Array.protoype.includes
which helps to check if the argument element (‘2’ in this case) is included in the elements of the array, and returns a boolean. To demonstrate:
let array = [1, 2, 3]
console.log(array.includes(2)) // Output: true
Now, let’s suppose we install a browser which doesn’t include this array method. In order to have the browser run this method, we need to make our own polyfill of Array.prototype.includes
to mimic its functionality. Let’s make the polyfill below:
if (!Array.prototype.includes) {
Array.prototype.includes = function(searchElement) {
for (let i = 0; i < this.length; i++) {
if (this[i] === searchElement) {
return true;
}
}
return false;
}
}
We check if the array method includes
does not exist, we create a function accepting a parameter and assign that function to the method. this
acts like a pointer that points to the array calling the method, letting the polyfill know which array’s data to work with.
Very well, let’s make another one. Array.prototype.pop
is a method which, when executed, removes the last element of the array and returns that element.
array = [1, 2, 3]
console.log(array.pop()); //Output = 3
Although pop
is an inbuilt native javascript method and is technically not considered a polyfill, we’ll still make one for educational purposes for the sake of deeper understanding. Here’s what our polyfill looks like:
// To nullify the already existing array method to create a new one
Array.prototype.pop = null;
if (!Array.prototype.pop) {
Array.prototype.pop = function() {
// Check if the array is empty
if (this.length === 0) {
return undefined;
}
// Get the last element
const lastElement = this[this.length - 1];
// Reduce the length by 1, effectively removing the last element
this.length = this.length - 1;
// Return the removed element
return lastElement;
};
}
array = [1, 2, 3]
console.log(array.pop());
Creating your own polyfill
Decide what feature you want to be implemented that is missing in your browser: Let’s say we want to create an object which returns the first key-value pair inside of it.
Check if the feature already exists: Use Google or an AI tool (like ChatGPT or Perplexity) to figure out if the feature (Object in our case) that you want to implement already exists or not.
Write the polyfill if the feature is missing: Since there is no object that returns the first key-value pair inside an object, we can create a polyfill for that:
if (!Object.prototype.firstPair) { Object.prototype.firstPair = function() { // Ensure 'this' is an object and not null if (this == null) { throw new TypeError('firstPair called on null or undefined'); } // Get all enumerable own keys const keys = Object.keys(this); // Check if there are any keys if (keys.length === 0) { return undefined; // Return undefined for an empty object } // Return the first key-value pair as an array [key, value] const firstKey = keys[0]; return [firstKey, this[firstKey]]; } }
Test your polyfill: Write out some test cases also, including edge cases, to make sure your polyfill works as intended.
const realMadrid = {Asensio: "Defender", Valverde: "Midfielder"} console.log(realMadrid.firstPair()) //Output = [ 'Asensio', 'Defender' ]
This works even in environments without native
Object.firstPair()
.
You can basically follow this simple flowchart next time you want to identify whether you want to create your own polyfill:
Summary
In this article, we learnt how polyfills play an integral part in web development, helping us implement personalized features for browsers. We can create features to make old browsers support modern web browser features or implement our own features in modern web environments. Also, we saw some examples of already existing polynomials and a custom one. Moreover, we saw how to decide why, when and how to create a polyfill. To conclude, now that you know everything about polynomials, I would recommend you to go and create your own and have fun with it!
Subscribe to my newsletter
Read articles from Sartaj Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
