Python: Effortless File Management by Zipping Folders
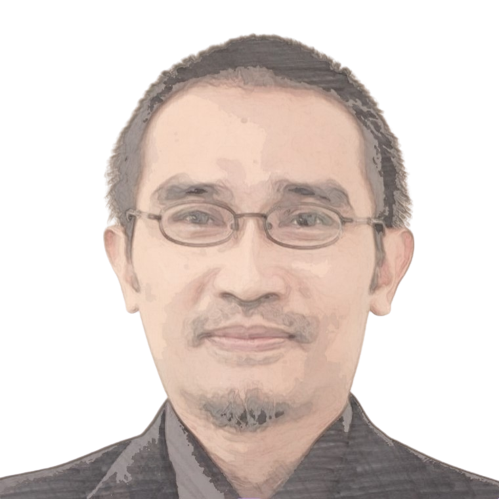
In today's digital age, managing files and folders efficiently is crucial for both personal and professional tasks. Whether you're organizing project files, archiving old documents, or simply looking to save space on your storage device, zipping folders can be a game changer. Python, with its powerful libraries and straightforward syntax, offers a simple way to automate the process of compressing multiple directories into ZIP files. In this blog post, we will explore how to write a Python script that locates folders within a specified path and compresses them based on their names, streamlining your file management tasks.
import os
import zipfile
def zip_folders_in_path(path):
# List all items in the specified directory
for item in os.listdir(path):
item_path = os.path.join(path, item)
# Check if the item is a directory
if os.path.isdir(item_path):
zip_file_path = os.path.join(path, f"{item}.zip")
# Create a zip file for the directory
with zipfile.ZipFile(zip_file_path, 'w', zipfile.ZIP_DEFLATED) as zip_file:
for foldername, subfolders, filenames in os.walk(item_path):
for filename in filenames:
file_path = os.path.join(foldername, filename)
zip_file.write(file_path, os.path.relpath(file_path, item_path))
print(f"Zipped folder: {item} into {zip_file_path}")
# Specify the path
path = r"C:\example\folders"
# Call the function
zip_folders_in_path(path)
The following code checks if a ZIP file already exists for each folder. If it does, the script will skip zipping that folder and proceed to delete the original folder instead:
import os
import zipfile
import shutil
def zip_and_delete_folders_in_path(path):
# List all items in the specified directory
for item in os.listdir(path):
item_path = os.path.join(path, item)
# Check if the item is a directory
if os.path.isdir(item_path):
zip_file_path = os.path.join(path, f"{item}.zip")
# Check if the zip file already exists
if not os.path.exists(zip_file_path):
# Create a zip file for the directory
with zipfile.ZipFile(zip_file_path, 'w', zipfile.ZIP_DEFLATED) as zip_file:
for foldername, subfolders, filenames in os.walk(item_path):
for filename in filenames:
file_path = os.path.join(foldername, filename)
zip_file.write(file_path, os.path.relpath(file_path, item_path))
print(f"Zipped folder: {item} into {zip_file_path}")
else:
print(f"Zip file already exists for folder: {item}. Skipping zipping.")
# Delete the original folder
shutil.rmtree(item_path)
print(f"Deleted folder: {item}")
# Specify the path
path = r"C:\example\folders"
# Call the function
zip_and_delete_folders_in_path(path)
Subscribe to my newsletter
Read articles from Mohamad Mahmood directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
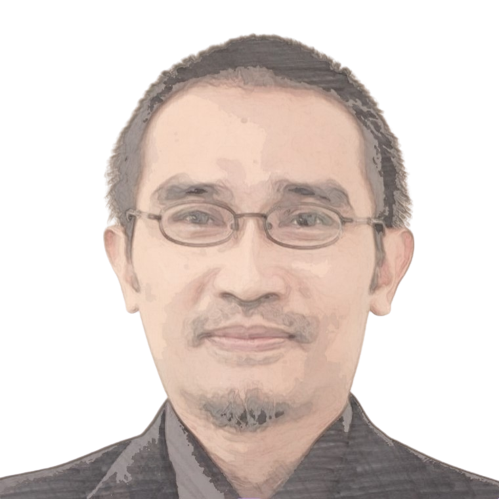
Mohamad Mahmood
Mohamad Mahmood
Mohamad's interest is in Programming (Mobile, Web, Database and Machine Learning). He studies at the Center For Artificial Intelligence Technology (CAIT), Universiti Kebangsaan Malaysia (UKM).