Insert and Extract Images in Word Tables Using Python – Guide

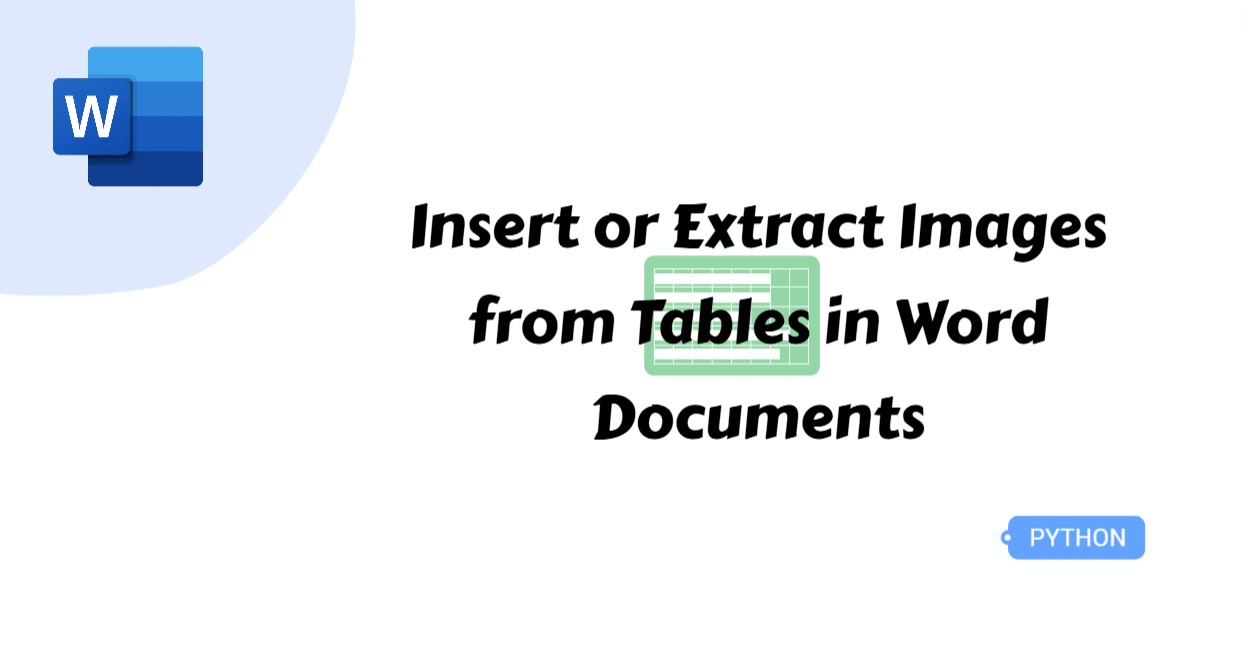
Tables are a crucial element in Word documents, often used to structure data clearly. In many cases, you may need to insert images, such as company logos or product samples, into table cells. Conversely, extracting these images for archiving or further use can also be essential. Python provides a powerful way to automate both tasks, making document processing more efficient. In this article, we’ll explore how to insert and extract images in Word tables using Python, helping you streamline your workflow and boost productivity.
Python Libraries to Insert and Extract Images in Word Tables
When using Python for inserting or extracting images in Word tables, leveraging the right libraries can significantly improve efficiency. Open-source options like python-docx can handle basic image operations, but they come with limitations—it can extract images from Word documents but not directly from tables.
For more advanced functionality, enterprise-level libraries such as Spire.Doc and Aspose.Words are better choices. These libraries not only support precise image manipulation in Word tables but also offer additional features like converting Markdown to Word, finding and replacing text in Word files, and more.
Considering their capabilities, this article will use Spire.Doc to demonstrate how to insert and extract images in Word tables. You can install it easily with the following command: pip install spire.doc.
How to Insert Images into Word Tables with Python
Now that the preparations are complete, let's dive into the process. Adding images to a Word table enhances visual appeal, making the table more engaging and easier to understand. This is especially useful for including company logos, product images, or other visual elements that support the content. With Spire.Doc, you can use the Spire.TableCell.Paragraphs[index].AppendPicture() method to insert images into specific table cells effortlessly. Below, we’ll walk through the detailed steps to accomplish this.
Steps to insert images into a Word table:
Create an object of the Document class.
Read a source Word file using the Document.LoadFromFile() method.
Get a section with the Document.Sections[] property and get a table with the Section.Tables[] property.
Access a certain cell through the Table.Row[index].Cells[index] property.
Insert an image into the cell using the TableCell.Paragraphs[index].AppendPicture() method.
Save the updated Word document with the Document.SaveToFile() method.
The following code example shows how to insert an image to the 3rd cell of the 2nd row in the first table of a Word document:
from spire.doc import *
from spire.doc.common import *
# Create an object of the Document class
doc = Document()
# Load a Word document
doc.LoadFromFile("/Doc1.docx")
# Get the first section
section = doc.Sections[0]
# Get the first table in the section
table = section.Tables[0]
# Add an image to the 3rd cell of the second row in the table
cell = table.Rows[1].Cells[2]
picture = cell.Paragraphs[0].AppendPicture("/image.png")
# Set image width and height
picture.Width = 100
picture.Height = 100
# Save the result document
doc.SaveToFile("/AddImagesToTable.docx", FileFormat.Docx2013)
doc.Close()
How to Extract Images from Word Tables Using Python
Extracting images from a Word table is a bit more complex than inserting them. You need to iterate through each cell, check its paragraphs, and scan all objects to ensure no image is missed. However, there's no need to worry—we'll walk through the process step by step.
To make things easier, this section provides detailed explanations along with a Python code sample, helping you understand and implement the extraction process efficiently. Let’s dive in!
Steps to extract all images from a Word table:
Create an instance of the Document class and read a source file using the Document.LoadFromFile() method.
Get a section through the Document.Sections[] property and get a table with the Section.Tables[] property.
Iterate through all rows in the table. Loop through each cell in each row, and all paragraphs in each cell. Iterate through all child objects in each paragraph.
Check if the child object is of DocPicture type.
Retrieve the image bytes of the DocPicture object using the DocPicture.ImageBytes property and append them to a list.
Save the image bytes as image files in the list.
from spire.doc import *
from spire.doc.common import *
# Create an object of the Document class
doc = Document()
# Load a Word document
doc.LoadFromFile("/AddImagesToTable.docx")
# Get the first section
section = doc.Sections[0]
# Get the first table in the section
table = section.Tables[0]
# Create a list to store image bytes
image_data = []
# Iterate through all rows in the table
for i in range(table.Rows.Count):
row = table.Rows[i]
# Iterate through all cells in each row
for j in range(row.Cells.Count):
cell = row.Cells[j]
# Iterate through all paragraphs in each cell
for k in range(cell.Paragraphs.Count):
paragraph = cell.Paragraphs[k]
# Iterate through all child objects in each paragraph
for o in range(paragraph.ChildObjects.Count):
child_object = paragraph.ChildObjects[o]
# Check if the current child object is of DocPicture type
if isinstance(child_object, DocPicture):
picture = child_object
# Get the image bytes
bytes = picture.ImageBytes
# Append the image bytes to the list
image_data.append(bytes)
# Save the image bytes in the list to image files
for index, item in enumerate(image_data):
image_Name = f"/AllImages/Image-{index}.png"
with open(image_Name, 'wb') as imageFile:
imageFile.write(item)
doc.Close()
The Conclusion
In this article, we’ve explored how to insert and extract images from Word tables using Python. With the step-by-step guide and code examples provided, you should now be able to handle both tasks efficiently. Try out these methods in your own projects and streamline your Word document processing!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
