Understanding SQL Queries Using the EXPLAIN Command
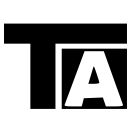
5 min read
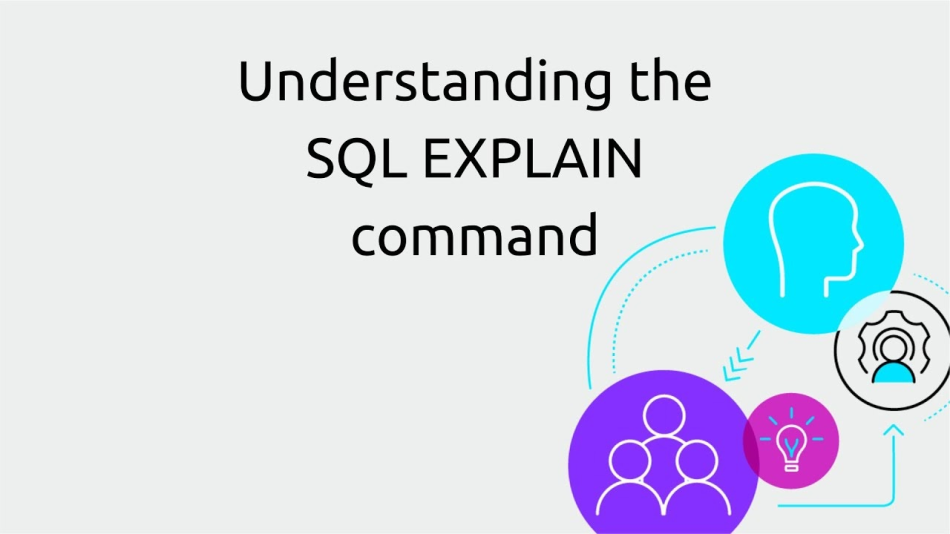
1. What is the EXPLAIN Command?
The EXPLAIN command is a powerful SQL feature that allows you to view the query execution plan. The execution plan is a breakdown of how the SQL engine will process your query, from selecting the best indexes to applying joins, filtering, and sorting. With this tool, you can spot potential bottlenecks and inefficiencies in your query.
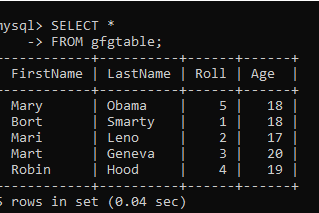
1.1 Understanding the Basics of EXPLAIN
The simplest way to use EXPLAIN is to prefix your SQL query with the EXPLAIN keyword. For example:
EXPLAIN SELECT * FROM employees WHERE department = 'HR';
Running this query doesn’t return the actual data; instead, it provides a detailed breakdown of how the SQL engine plans to execute this query.
1.2 The Output of EXPLAIN
The output of EXPLAIN generally consists of several columns, including but not limited to:
- id: Identifies the order of execution.
- select_type: Describes the type of query (e.g., SIMPLE, SUBQUERY).
- table: Indicates which table is being accessed.
- type: Shows the type of join (e.g., ALL, INDEX, REF).
- possible_keys: Lists potential indexes that could be used.
- key: Specifies the actual index chosen by the query planner.
- rows: Estimated number of rows that will be scanned.
- extra: Additional information, such as whether filesort or temporary tables are being used.
Let’s break down the importance of these fields.
1.3 Why These Fields Matter
- id: This field helps you understand the order in which your SQL statements are executed, especially for complex queries with subqueries.
- select_type: Identifies whether the query is SIMPLE, PRIMARY, or a SUBQUERY. This gives insights into the complexity of the query.
- possible_keys and key: The keys (indexes) are critical because they determine how fast the database can access the data. If no index is used, it’s a red flag for potential performance issues.
1.4 A Simple Example
Consider a simple query to fetch employees from the HR department:
EXPLAIN SELECT * FROM employees WHERE department = 'HR';
The output might look something like this:
id | select_type | table | type | possible_keys | key | rows | Extra |
1 | SIMPLE | employees | ref | department | dept | 50 | Using where |
Here, the EXPLAIN result shows that the database will use the dept index and will scan approximately 50 rows. This is an efficient query, as the database is leveraging an index to limit the number of rows it needs to evaluate.
2. Optimizing Queries with EXPLAIN
Knowing how to interpret the output of EXPLAIN is crucial for optimizing SQL queries. Let's dive deeper into specific scenarios where EXPLAIN can guide you toward optimization.
2.1 Identifying Full Table Scans
One common issue with SQL queries is full table scans, which can significantly slow down query performance, especially on large tables. A full table scan means that the database has to evaluate every row in the table to determine which rows match the query.
For instance, if you run this query:
EXPLAIN SELECT * FROM orders WHERE order_date = '2023-09-01';
And see output like this:
id | select_type | table | type | possible_keys | key | rows | Extra |
1 | SIMPLE | orders | ALL | NULL | NULL | 1000 | Using where |
In this case, the ALL type and NULL keys indicate that no index is being used, and the database will scan all 1,000 rows. Adding an index on order_date could significantly improve performance.
CREATE INDEX idx_order_date ON orders(order_date);
Running the same query again might result in:
id | select_type | table | type | possible_keys | key | rows | Extra |
1 | SIMPLE | orders | ref | idx_order_date | idx_order_date | 100 | Using where |
The query planner now uses the idx_order_date index, scanning only 100 rows instead of 1,000, which is a clear improvement in efficiency.
2.2 Optimizing Joins
When working with multiple tables, joins are inevitable. However, improper join types can also lead to performance issues. Let’s examine a join query:
EXPLAIN SELECT employees.name, departments.dept_name
FROM employees
JOIN departments ON employees.dept_id = departments.id
WHERE employees.salary > 50000;
The output might look like this:
id | select_type | table | type | possible_keys | key | rows | Extra |
1 | SIMPLE | departments | ALL | PRIMARY | NULL | 50 | |
1 | SIMPLE | employees | ref | dept_id | dept_id | 100 | Using where |
Here, the ALL join type on the departments table indicates a full table scan, which could be inefficient for large datasets. You can optimize this by adding an index on departments.id:
CREATE INDEX idx_dept_id ON departments(id);
Now, the query optimizer can use the index, reducing the rows scanned in the join process.
2.3 Avoiding Filesorts
In some cases, you might see Using filesort in the Extra column of your EXPLAIN output. This indicates that the database is sorting the results in memory, which can be inefficient for large datasets. You can avoid filesorts by indexing the columns that are used in the ORDER BY clause.
For example:
EXPLAIN SELECT * FROM employees ORDER BY name;
If the output shows Using filesort, creating an index on name will help:
CREATE INDEX idx_name ON employees(name);
Running the EXPLAIN again should show improved performance without a filesort.
3. Conclusion
Using the EXPLAIN command is essential for optimizing your SQL queries and ensuring they run efficiently. By understanding the output of EXPLAIN, such as the use of indexes, the type of joins, and potential issues like full table scans or filesorts, you can make informed decisions about query optimization. Regularly using EXPLAIN will not only improve your query performance but also deepen your understanding of how databases process SQL queries.
If you have any questions or need further clarification, feel free to leave a comment below.
Read more at : Understanding SQL Queries Using the EXPLAIN Command
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
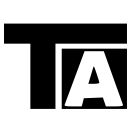
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.