Java Map.entrySet()

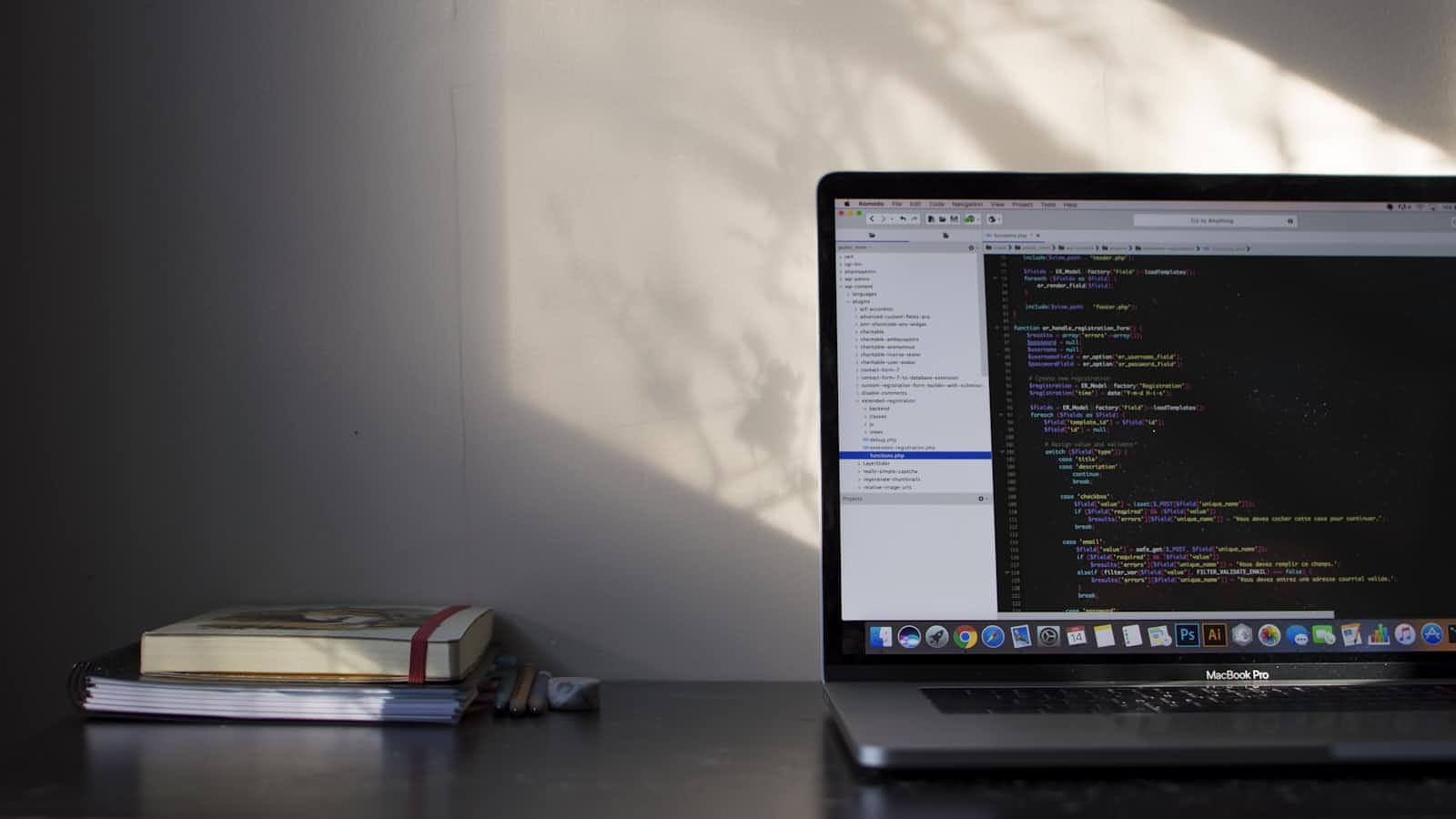
If you have ever worked with key-value pairs in Java, you’ve likely used a Map
. But what if you need to go through each entry efficiently? That’s where Map.Entry
comes in! This guide will help you understand Map.Entry
Set and how it makes iterating over a Map
easy.
What is Map.Entry
?
Map.Entry
is a built-in interface in Java that represents each key-value pair inside a Map
. It allows you to access and modify both keys and values while looping through the Map
.
How to Use entrySet()
The entrySet()
method in Java returns a set containing all key-value pairs in a Map
. This makes it easier to iterate over a Map
without needing to call get(key)
repeatedly.
Syntax:
Set<Map.Entry<K, V>> entrySet()
K: Type of the key.
V: Type of the value.
Example: Iterating Through a Map
Here’s a simple example of how to use entrySet()
to print key-value pairs:
import java.util.HashMap;
import java.util.Map;
public class MapEntrySetExample {
public static void main(String[] args) {
// Creating a HashMap
Map<String, Integer> studentMarks = new HashMap<>();
studentMarks.put("Alice", 85);
studentMarks.put("Bob", 90);
studentMarks.put("Charlie", 78);
// Using entrySet() to iterate
for (Map.Entry<String, Integer> entry : studentMarks.entrySet()) {
System.out.println(entry.getKey() + " scored " + entry.getValue());
}
}
}
Modifying Values Using entrySet()
You can update values inside a Map
while iterating over it.
for (Map.Entry<String, Integer> entry : studentMarks.entrySet()) {
if (entry.getKey().equals("Alice")) {
entry.setValue(90); // Updating Alice's marks
}
}
Using Java Streams with entrySet()
With Java 8, you can use Streams for a cleaner approach:
studentMarks.entrySet().stream()
.filter(entry -> entry.getValue() > 80)
.forEach(entry -> System.out.println(entry.getKey() + " scored " + entry.getValue()));
When Should You Use entrySet()
?
Use entrySet()
when:
You need to access both keys and values in a loop.
You want to update values while iterating.
You prefer a more efficient way than calling
get(key)
multiple times.
Mind Map: Quick Recap
To help you quickly recall the concept, here’s a simple mind map:
[ Map.Entry Set in Java ]
│
┌──────────────┴──────────────┐
│ │
[ What is it? ] [ Why use it? ]
│ │
- Represents key-value - Efficient iteration
pairs in a Map - Access both key & value
- Provides `getKey()`, - Modify values directly
`getValue()`, `setValue()` - Works well with Streams
Conclusion
The Map.Entry
interface and entrySet()
method provide a simple and effective way to work with Java Maps. Whether you use a loop or Java Streams, this method makes your code cleaner and faster.
Happy Coding! 🍻
Subscribe to my newsletter
Read articles from Amit Sangwan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amit Sangwan
Amit Sangwan
💼 Automation Engineer | AI Enthusiast | Tech Blogger Passionate about automation, AI agents, and testing. Exploring innovations in QA while sharing insights on technology and career growth. Always learning, always evolving. 🚀