Functions in JavaScript

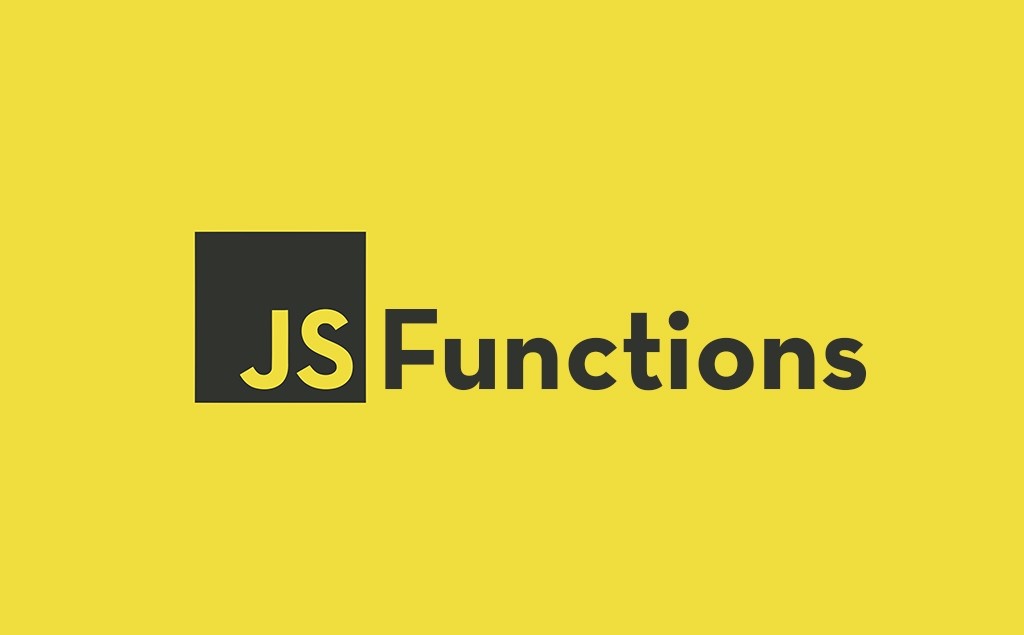
Functions are the basic building blocks of JavaScript. People often say that functions are the heart of JavaScript. To understand JavaScript well, you need to understand functions well.
What is a function ?
A function is a group of statements written to do a specific job. Why use functions? We often need to do the same task in different parts of our code. In these cases, functions are very useful. We write a function for that task and use it wherever needed. This reduces repetition and makes our code more reusable.
Function Declaration
So, how do we tell JavaScript that we want to write a function? We use the 'function' keyword. Here is the syntax for declaring a function.
function functionName() {
// function body
}
Just declaring a function doesn't make it run. We need to call the function to make it do its job. Here's an example.
// Function declaration or Function Statement
function greet(){
console.log("Good Evening");
}
// Calling the function
greet();
In the code above, calling greet() runs the function, so "Good Evening" is printed out.
A function declaration is also called a Function Statement.
Function Expression
When we create a function and assign it to a variable like any other value, it is called a function expression.
// Function expression
const greet = function(){
console.log("Good Evening");
}
// Calling the function
greet();
Let me explain the code above a bit.
The function without a name is assigned to the greet variable. Notice that the function itself is assigned, not its return value (we'll discuss return values later). Since greet holds the function, we use it to call the function.
We can name the function, but this name can't be used to call the function from outside. However, we can use this name inside the function body itself.
// Named Function expression
const greet = function greetings(){
console.log("Good Evening");
}
// Calling the function
greet();
// This will cause ReferenceError
greetings();
Function Declaration vs Expression
There are two main differences →
In a function expression, you can leave out the function name, but in a function statement, leaving out the name will cause an error saying Function declaration requires a function name.
Function statements are hoisted, but function expressions are not. If you're not familiar with hoisting, just remember that function statements can be called before they are declared, but function expressions must be declared before they are called.
greet();
foo(); // cause error
// Function expression
var foo = function(){
console.log("I'm foo");
}
// Function declaration/statement
function greet(){
console.log("Good Evening");
}
greet();
foo();
Parameters and return statement
Often, a function needs some information to do its job. This information is given to the function through parameters.
A function can also return a value if needed. When the function finishes running or hits a return statement, it goes back to the line where it was called.
See it in action below.
function add(x,y){
const result = x + y;
return result;
}
const added = add(5,9);
console.log(added); // 14
Notice how the result is returned from function add() and assigned to the variable added.
Arguments vs Parameters
Arguments are what you pass when you call a function. Parameters are what receive these arguments in the function.
In our example above, x and y are parameters, while 5 and 9 are arguments. x gets the value 5, and y gets the value 9.
First Class Functions
In JavaScript, functions are first-class citizens, which means you can use them like any other variable. These are called first-class functions.
You can assign a first-class function to a variable (as shown above).
You can pass it to other functions as an argument.
You can return it from other functions.
function foo(func){
func();
}
function bar(){
console.log("I'm bar");
}
foo(bar);
Notice function bar is passed to the function foo and function foo receives it in the func variable and calls it.
Anonymous Function
Functions without a name are called anonymous. You can see this in the function expression.
Anonymous functions are used when we treat functions like any other variable or when a function is connected to event listeners.
Here's an example.
function foo(func){
func();
}
foo( function(){
console.log("I'm bar");
} );
Notice how an anonymous function is passed to the function foo.
Higher-order Function
A function that takes another function as an argument or returns another function is called a Higher-order Function.
Why do we use it?
Higher-order functions help us organize our code. They make our code more flexible and easier to maintain. Ready for some code?
Let's write some code →
function morning(name){
return `Good morning, ${name}`;
}
function evening(name){
return `Good evening, ${name}`;
}
function happyBirthday(name){
return `Happy Birthday, ${name}`;
}
function greet(name, func){
return func(name);
}
console.log(greet("Faheem",morning));
console.log(greet("Jack",evening));
console.log(greet("Nat",happyBirthday));
Let's break down this example step by step -
First, notice that greet is a higher-order function because it takes another function as an argument.
See how our code is organized. We have different greeting messages in small functions. These functions are passed to greet(), which calls them and returns the message to the caller.
In the example above, the functions morning(), evening(), and happyBirthday() are examples of callback functions because they are passed to another function (greet in this case) and then called by that function greet.
Function returning another function
Higher-order functions can return another function.
function calculateTotalPrice(money){
return function (){
return money + money *0.1;
}
}
const addTaxestoo = calculateTotalPrice(100);
console.log(addTaxestoo());
// calling in a single line
console.log(calculateTotalPrice(100)())
Notice, calculateTotalPrice() is a higher-order function because it returns another function. Also, see how to call the returned function in one line.
Arrow Function
Arrow functions were introduced in ES6, providing a simpler and shorter way to create functions. Here's how to create a regular function with arguments that returns a value →
function add(num1, num2) {
const result = num1 + num2;
return result;
}
To turn this into an arrow function, here's how you do it →
const add = (num1, num2) => {
const result = num1 + num2;
return result;
}
Key Points About Arrow Functions
No arguments Object →
The traditional function has an arguments
object that you can use inside the function →
function add(num1, num2) {
console.log(arguments.length);
}
add(1,2); // 2 will be printed
On the other hand, arrow functions don't have an arguments object. If you try to use it, you'll get a ReferenceError →
let add = (num1, num2) => {
console.log(arguments.length);
}
add(1,2); // ReferenceError: arguments is not defined
No this binding →
This is the biggest difference between an arrow function and a regular function. Let's explore it further.
In regular functions, the this variable is created, which refers to the object that calls them. Let's check out the code below →
const obj = {
name: 'john',
age: 21,
print: function() {
console.log(this.name)
}
}
obj.print(); // john
The this keyword refers to obj, the object that calls the method. Let's look at another example.
var name = 'alex';
const obj = {
name: 'john',
age: 21,
print: function() {
function foo() {
console.log(this.name);
}
foo();
}
}
obj.print(); // alex
It prints undefined because print is called by the obj object, but no object calls foo, so it points to the Window object.
With arrow functions, the function does not automatically create a this variable.
var z = 56;
const obj = {
name: 'john',
age: 21,
print: () => {
console.log(this.name)
}
}
obj.print(); // undefined
As a result, any reference to this will point to what this was before the function was created. So, this will refer to the Window object. Since the window object doesn't have a name variable, it prints undefined. If you try console.log(this.z) instead, you will get 56. Let's try another example →
const obj = {
name: 'john',
age: 21,
print: function() {
var name = 'alex';
let foo = () => {
console.log(this.name);
}
foo();
}
}
obj.print(); // john
The reason it prints john is that this refers to what this was before the function was made. Before foo, this pointed to the obj object, which has a name variable, so john is printed. If we change the print function to an arrow function, it will print undefined.
Implicit return
Arrow function with a single expression automatically returns the result of that expression without needing the return keyword.
const add = (num1, num2) => num1 + num2;
console.log(add(2,3)); // prints 5
Arrow functions are now a key part of modern JavaScript because they are simple and clear. They have a short syntax, avoid issues with the this context, and make your code easier to read and manage. Although they aren't perfect for every situation (like methods in classes that need their own this context), arrow functions are a useful tool in your JavaScript skills.
Conclusion
Functions are essential in JavaScript and act as its basic parts. This article looks at different types of functions, like function declarations, expressions, and arrow functions, showing their differences and uses. It also covers topics like parameters, return statements, and first-class functions, giving insights into anonymous and higher-order functions. The details of arrow functions, like implicit return and not having their own this binding, are explained to help understand modern JavaScript practices.
Thanks for reading this far. If you enjoyed it, please share your feedback.
Subscribe to my newsletter
Read articles from Rana Vikram Sinha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rana Vikram Sinha
Rana Vikram Sinha
Just a dude with his internet connection👨💻