๐ JavaScript Variables and Data Types: A Complete Guide ๐ฏ

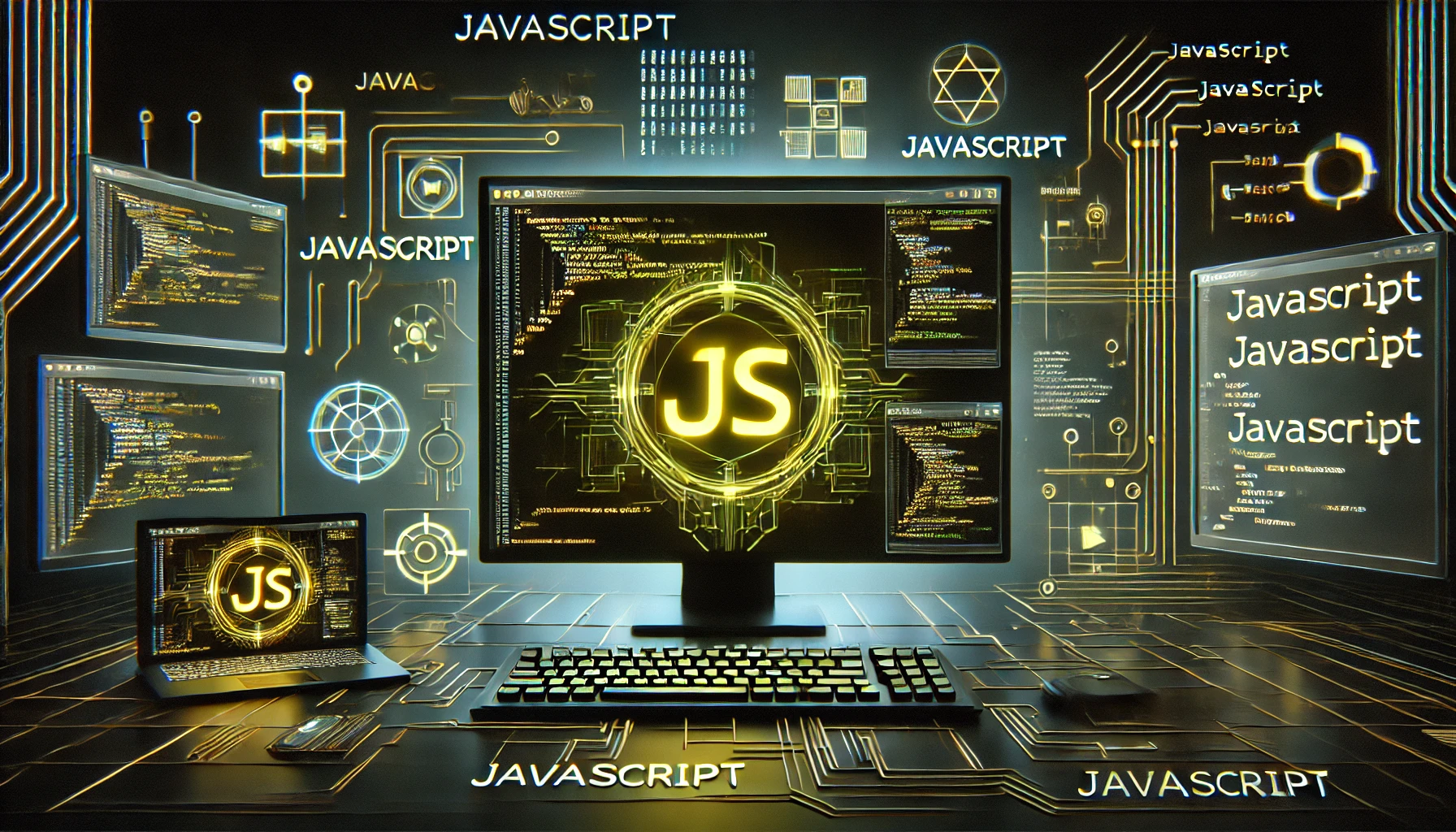
๐ Understanding Variables in JavaScript
A variable is a container for storing data values. In JavaScript, we use var, let, and const to declare variables.
๐น Declaring Variables
var name = "Vivek"; // Old way (Not recommended) let age = 24; // Preferred for changing values const country = "India"; // Preferred for constant values
๐ Key Differences:
โ
var โ Function-scoped, can be redeclared & updated (not recommended).
โ
let โ Block-scoped, can be updated but not redeclared.
โ
const โ Block-scoped, cannot be updated or redeclared.
๐ฅ JavaScript Data Types
JavaScript has two main categories of data types:
1๏ธโฃ Primitive Data Types (Stored by value)
๐น String โ Represents text. let message = "Hello, JavaScript!";
๐น Number โ Represents both integers & floating points. let score = 99.5;
๐น Boolean โ Represents true or false. let isJavaScriptFun = true;
๐น Undefined โ A variable without an assigned value. let x; // x is undefined
๐น Null โ Represents an intentional absence of value. let y = null;
๐น Symbol (ES6) โ Unique and immutable values. const sym = Symbol("unique");
๐น BigInt (ES11) โ Large integers. let bigNumber = 12345678901234567890n;
2๏ธโฃ Non-Primitive (Reference) Data Types (Stored by reference)
๐น Object โ Collection of key-value pairs. let person = { name: "Vivek", age: 25 };
๐น Array โ Ordered list of values. let colors = ["red", "green", "blue"];
๐น Function โ Block of reusable code. function greet() { return "Hello!"; }
๐ฏ Dynamic Typing in JavaScript
JavaScript is dynamically typed, meaning you donโt have to specify the type of variable explicitly. let data = "Hello"; // String data = 42; // Now it's a Number!
๐ Conclusion
Variables and data types are the foundation of JavaScript programming. Understanding them helps in writing efficient and error-free code! ๐ฅ
๐ฏ What's Next?
In the next blog, we will explore JavaScript Operators and Expressions in detail. Stay tuned! ๐
๐ Read More on: vivekwebdev.hashnode.dev ๐
Subscribe to my newsletter
Read articles from Vivek varshney directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vivek varshney
Vivek varshney
Full-Stack Web Developer | Blogging About Tech, React.js, Angular, Node.js & Web Development. Turning Ideas into Code & Helping Businesses Grow!