Developing Smart Contracts on CrossFi Chain

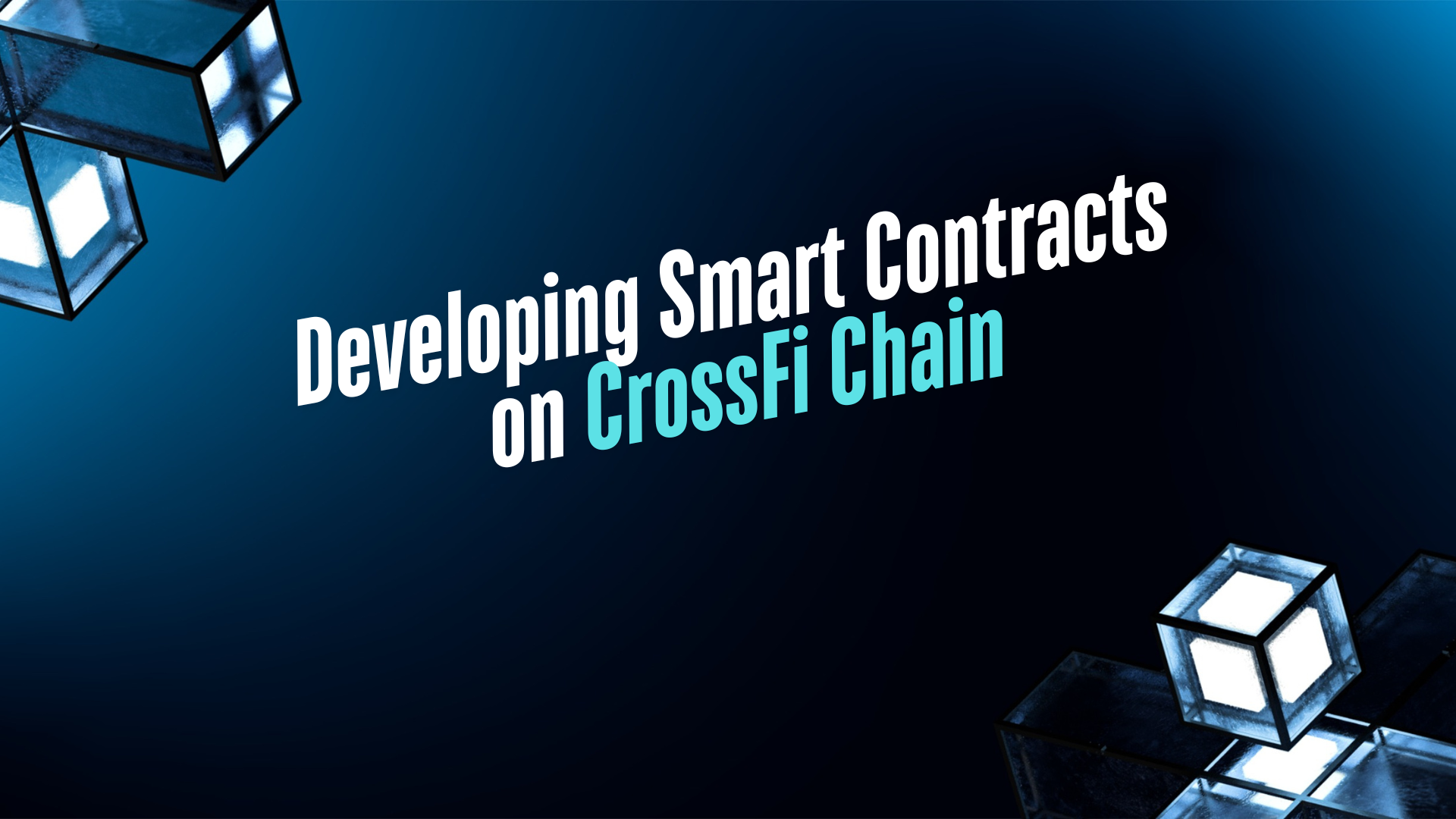
CrossFi Chain is an EVM-compatible blockchain, allowing developers to build and deploy Solidity-based smart contracts with ease. Whether you're creating DeFi protocols, NFT platforms, or decentralized marketplaces, this guide will help you set up your development environment, write your first contract, and deploy it on the CrossFi Testnet.
๐ Prerequisites
Before we begin, ensure you have the following installed:
โ
Node.js
โ
Hardhat (for Solidity development)
โ
MetaMask (for managing CrossFi tokens)
โ
A CrossFi Testnet account with test tokens
๐ง Setting Up Hardhat
First, create a new Hardhat project:
mkdir crossfi-smart-contract && cd crossfi-smart-contract
npm init -y
npm install --save-dev hardhat
npx hardhat
Select "Create a JavaScript project" when prompted.
๐ Writing a Smart Contract
Create a new file contracts/SimpleStorage.sol
and add:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 private storedValue;
function set(uint256 _value) public {
storedValue = _value;
}
function get() public view returns (uint256) {
return storedValue;
}
}
โ๏ธ Configuring Hardhat for CrossFi
Update hardhat.config.js
to use CrossFi Testnet:
require("@nomiclabs/hardhat-ethers");
module.exports = {
solidity: "0.8.20",
networks: {
crossfiTestnet: {
url: "https://rpc-testnet.crossfi.org",
accounts: ["0xYOUR_PRIVATE_KEY"]
}
}
};
๐ก Replace "0xYOUR_PRIVATE_KEY"
with your actual CrossFi testnet private key.
Compiling and Deploying the Contract
Compile the smart contract:
npx hardhat compile
Deploy the contract by creating a new script scripts/deploy.js
:
const hre = require("hardhat");
async function main() {
const SimpleStorage = await hre.ethers.getContractFactory("SimpleStorage");
const contract = await SimpleStorage.deploy();
console.log("Contract deployed to:", contract.target);
}
main().catch((error) => {
console.error(error);
process.exit(1);
});
Run the deployment script:
npx hardhat run scripts/deploy.js --network crossfiTestnet
๐ฏ After deployment, note down the contract address!
๐ก Interacting with the Smart Contract
Use a simple script scripts/interact.js
to interact with the deployed contract:
const hre = require("hardhat");
async function main() {
const contractAddress = "0xYourDeployedContractAddress";
const SimpleStorage = await hre.ethers.getContractFactory("SimpleStorage");
const contract = SimpleStorage.attach(contractAddress);
await contract.set(42);
const value = await contract.get();
console.log("Stored Value:", value.toString());
}
main().catch((error) => {
console.error(error);
process.exit(1);
});
Run the script:
npx hardhat run scripts/interact.js --network crossfiTestnet
๐ Next Steps
๐ฏ Deploying the client side interface.
Subscribe to my newsletter
Read articles from Abdullahi Raji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
