args,kwargs, and Function Arguments in Python.

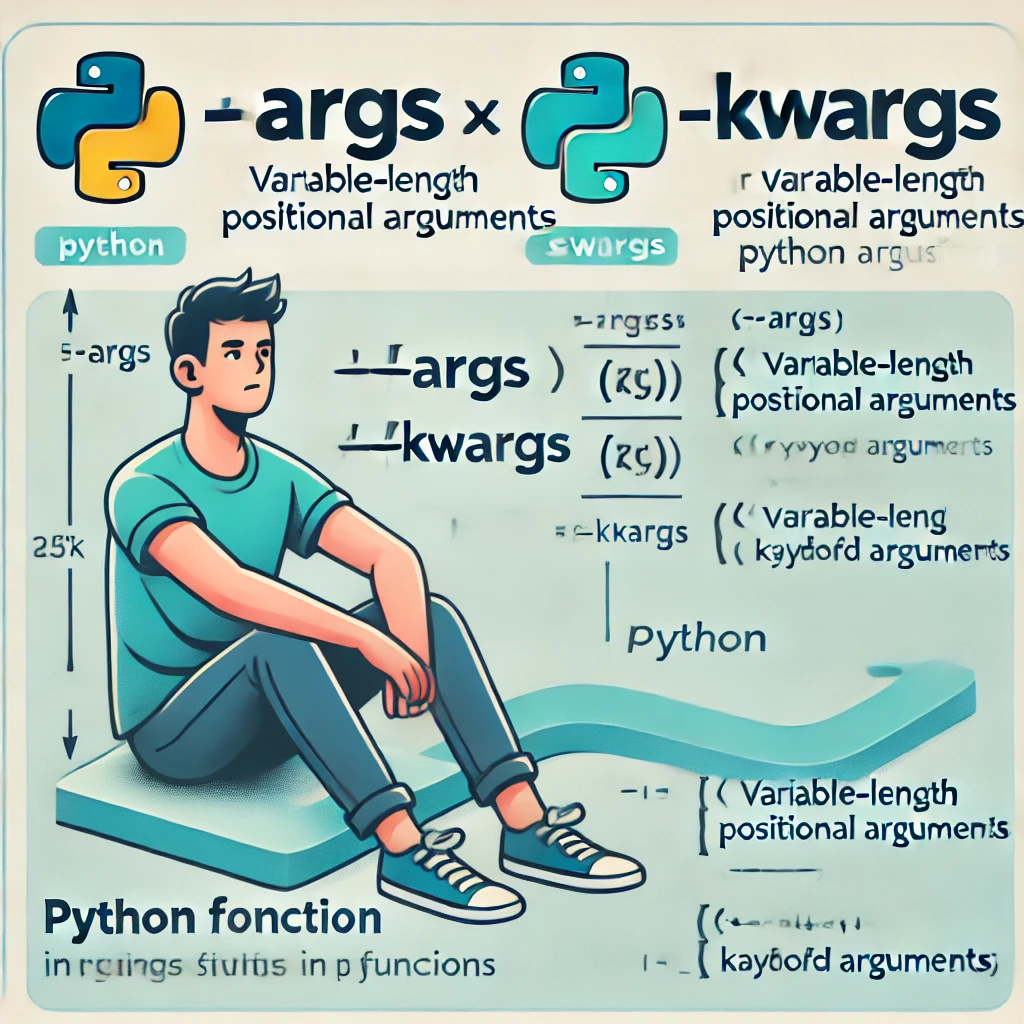
In Python, functions are the building blocks of reusable code. But what if you want to create a function that can handle a variable number of arguments? That's where *args
and **kwargs
come into play. In this blog post, we'll explore these concepts, along with the difference between positional and keyword arguments, and how to use the splat operator effectively.
Positional vs Keyword Arguments
Positional arguments are passed to a function based on their position. The order matters.
def greet(name, age):
print(f"Hello {name}, you are {age} years old.")
greet("Alice", 25) # "Hello Alice, you are 25 years old."
The Keyword arguments are passed with a keyword (i.e., the parameter name). The order doesn't matter.
greet(age=25, name="Alice") # Same output as above
What is the Splat Operator exactly?
The splat operator, is used to unpack iterables and dictionaries. Eg:
Single Asterisk (*
)
This is used to unpack iterables (e.g., lists, tuples) into positional arguments.
def add(a, b, c):
return a + b + c
numbers = [1, 2, 3]
print(add(*numbers)) # Output: 6
Double Asterisk (**
)
This is used to unpack dictionaries into keyword arguments.
def greet(name, age):
print(f"Hello {name}, you are {age} years old.")
person = {"name": "Alice", "age": 25}
greet(**person) # Output: Hello Alice, you are 25 years old.
*args
for Variable Positional Arguments
*args
collects extra positional arguments as a tuple. It’s Useful when you don't know how many arguments will be passed.
def sum_numbers(*args):
return sum(args)
print(sum_numbers(1, 2, 3)) # Output: 6
print(sum_numbers(10, 20, 30, 40)) # Output: 100
**kwargs
for Variable Keyword Arguments
The **kwargs
allows a function to accept any number of keyword arguments. It’s also Useful for handling named arguments dynamically.
def print_info(**kwargs):
for key, value in kwargs.items():
print(f"{key}: {value}")
print_info(name="Alice", age=25, city="New York")
# Output:
# name: Alice
# age: 25
# city: New York
Combining the *args
and **kwargs
def func(*args, **kwargs):
print("Positional arguments:", args)
print("Keyword arguments:", kwargs)
func(1, 2, 3, name="Alice", age=25)
# Output:
# Positional arguments: (1, 2, 3)
# Keyword arguments: {'name': 'Alice', 'age': 25}
Avoid overusing
*args
and**kwargs
as it can make code less readable.Always document your functions clearly when using these features.
Use meaningful variable names like
*values
or**options
instead of*args
and**kwargs
when appropriate.
Conclusion:
Understanding *args
, **kwargs
, and the difference between positional and keyword arguments is essential for writing flexible and reusable Python code. Whether you're building functions, decorators, or working with OOP, these tools will help you handle variable inputs with ease. Try experimenting with these concepts in your own projects and see how they can simplify your code!
Subscribe to my newsletter
Read articles from Lawani Elyon John directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lawani Elyon John
Lawani Elyon John
As a student at Babcock University, I've built a foundational understanding of HTML, CSS, and JavaScript.