Hydration in Modern Web Apps: What JavaScript Frameworks Don't Tell You

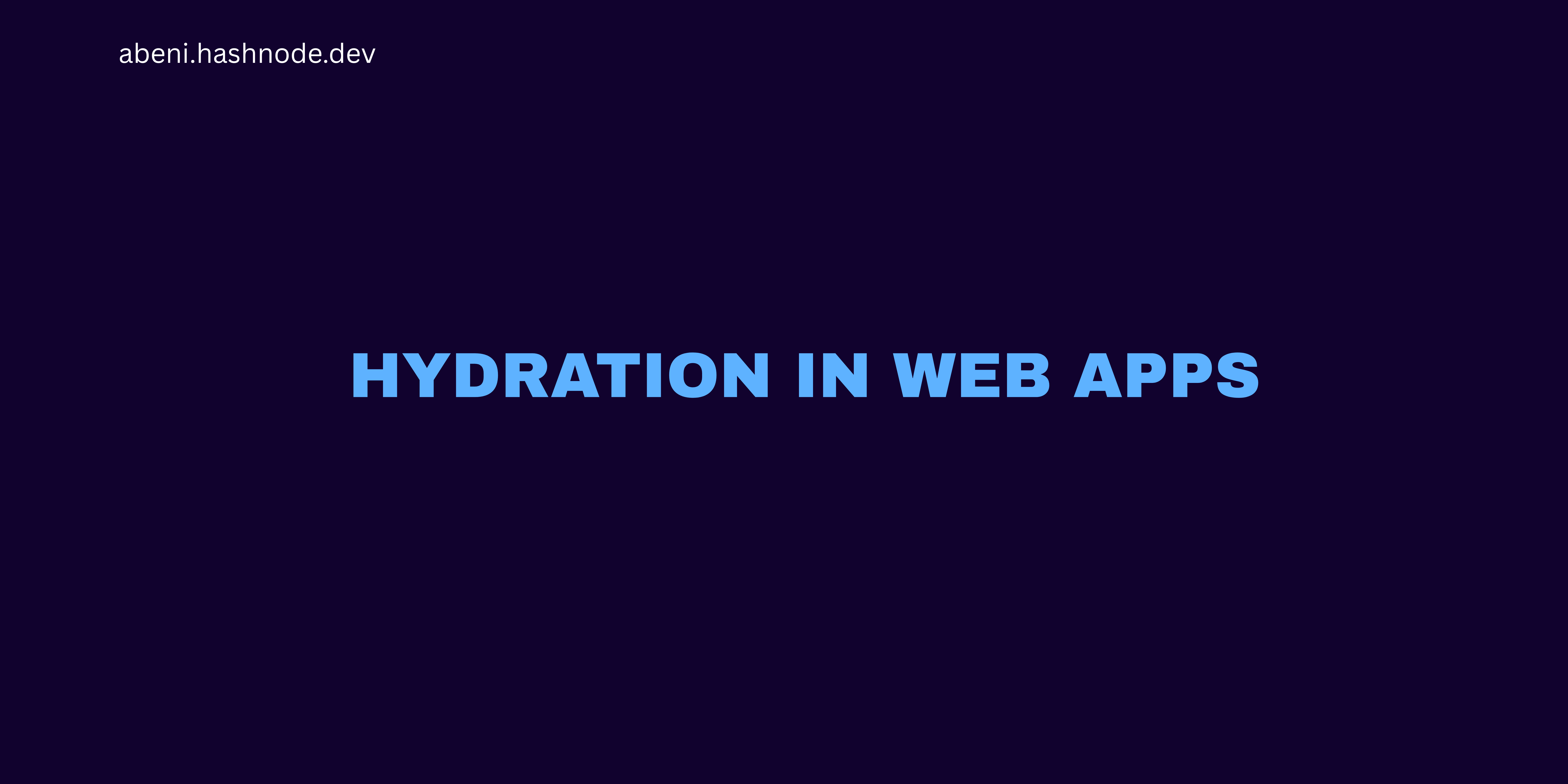
At some point, you’ve likely encountered a web page that feels refreshingly fast as it loads—and others where you wait, staring at a blank screen or a spinner. The difference? Often, it boils down to how web apps handle hydration. Surprisingly, while frameworks like React, Next.js, Vue, and Svelte have taken the web development world by storm, they don't always pull back the curtain on the silent complexities of hydration. Why does it exist, and what’s actually happening under the hood? Let’s dive in.
What is Hydration?
In simple terms, hydration is the process where the JavaScript framework reconciles a server-rendered HTML page by attaching event listeners, data, and interactivity. When your app is server-side rendered (SSR), it starts as static HTML that displays quickly. However, for interactivity—dropdowns, forms, buttons, modals, you name it—the framework needs to "hydrate" that HTML with JavaScript.
Let’s break it down:
Server-side Rendering (SSR): Generates the HTML on the server, sent to the browser.
Hydration: JavaScript kicks in on the client-side to activate that HTML.
For a mental model, imagine a freshly painted mural. While the picture looks complete (SSR), it’s missing the gloss coat that makes it shine (Hydration).
Why Hydration Matters
The necessity of hydration lies in combining the best of two worlds—speedy, SEO-friendly server rendering with the interactivity of client-side rendering. Here are some key benefits:
Initial Page Load Speed: Users see content faster.
Search Engine Optimization (SEO): Search engines like static HTML.
Dynamic Interactivity: Enables advanced, app-like functionality.
Yet, it’s not without challenges, which often go unnoticed.
Under the Hood: How Hydration Works
When hydration begins, the client-side JavaScript parses your server-rendered HTML and carefully recreates the DOM tree precisely as generated on the server. For frameworks like React, this involves comparing a “virtual DOM” representation to ensure minimal updates are made. If something doesn’t line up correctly, errors like content flickering or broken interactivity can occur.
Here’s a flow of how hydration works:
Initial HTML: Delivered from the server.
Script Loading: JavaScript assets download.
DOM Parsing: Client-side script parses the HTML DOM.
Reconciliation: Frameworks like React compare server-side DOM to virtual DOM.
Event Attachments: Event listeners are bound to relevant elements.
Hydration Complete: Your web app is now fully interactive.
Visualizing Hydration
Server-Side Rendering: HTML is painted on the browser.
Hydration Process: Hydration converts static HTML to a living app.
<!-- Server-rendered HTML example before hydration -->
<button id="my-button">Click Me</button>
<script src="bundle.js"></script>
// Hydration attaches event listener after JS is parsed
const button = document.getElementById('my-button');
button.addEventListener('click', () => alert('Hello!'));
Common Pitfalls of Hydration
Hydration isn’t without its downsides. Here are some common issues developers face:
Slow Hydration: Large JavaScript bundles can delay interactivity.
Over-Hydration: Unnecessarily hydrating static content.
Hydration Mismatches: When server-rendered HTML doesn’t match client data.
Optimization Techniques
Partial Hydration: Hydrate only specific components, e.g., text remains static but buttons are interactive.
Progressive Hydration: Incrementally hydrate as the user scrolls or interacts.
Islands Architecture: Divide the page into self-contained interactive “islands” (used by Astro).
Resumability: Skip the hydration process by saving and resuming the application state (e.g., Qwik).
Code Example: Optimization
Here’s an example using React with partial hydration:
import dynamic from 'next/dynamic';
const InteractiveComponent = dynamic(() => import('./InteractiveComponent'), {
ssr: false, // Skip server-side rendering for this component
});
export default function Page() {
return (
<div>
<h1>Static Content</h1>
<InteractiveComponent />
</div>
);
}
This ensures only critical components are hydrated, optimizing performance.
Over all, Hydration is one of those low-key marvels of modern web apps. The next time your front-end feels sluggish, pause and ask, “What’s my hydration game like?”
Subscribe to my newsletter
Read articles from Abenezer Teshome directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abenezer Teshome
Abenezer Teshome
MERN Stack & Next.js Enthusiast A computer science student sharing my development journey and connecting with fellow devs to grow together.