From Figma to React Native: A Step-by-Step Guide for Seamless UI Development

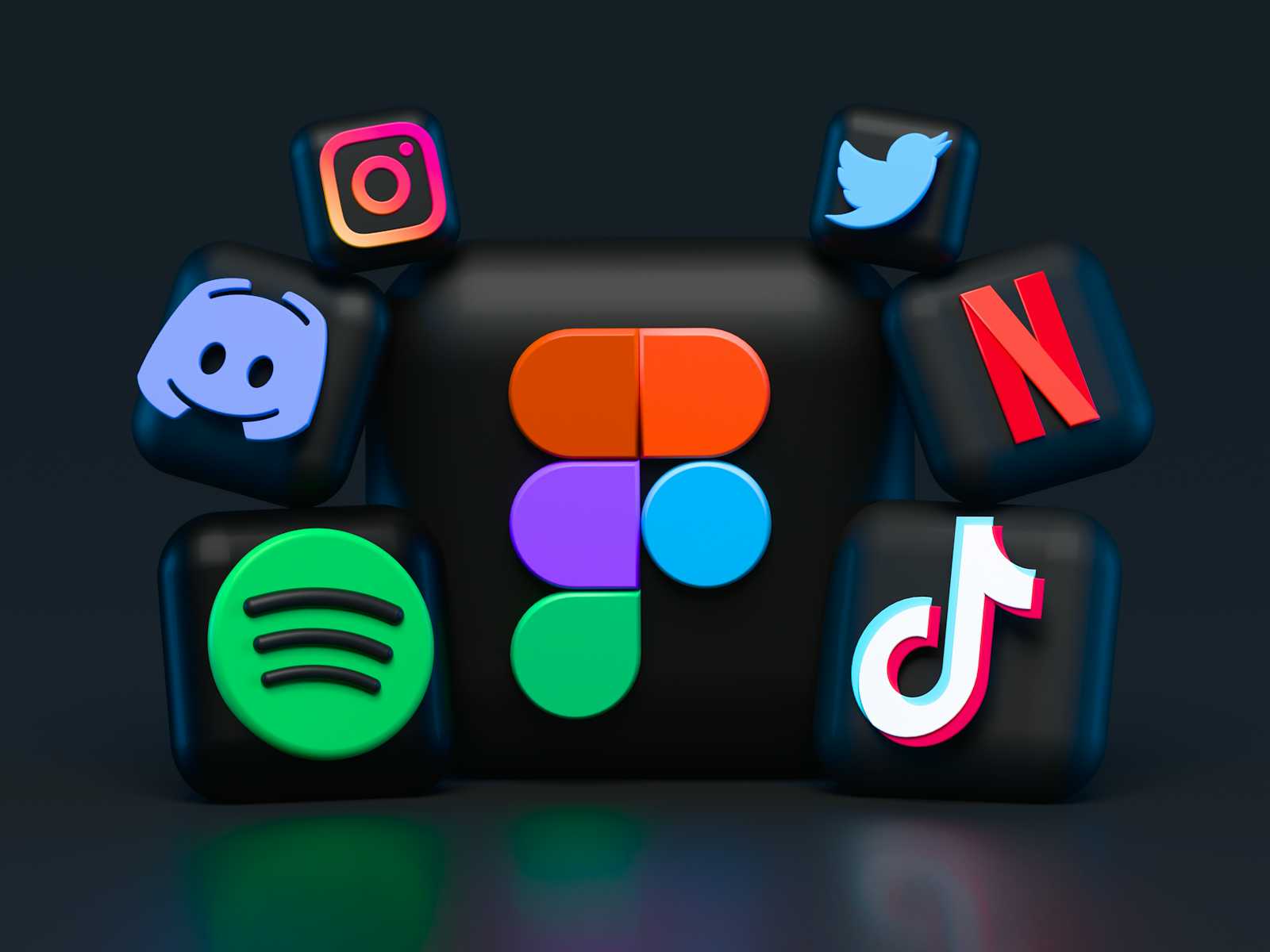
Introduction
Have you ever designed a stunning UI in Figma but struggled to translate it into a functional React Native application? You're not alone. The process of converting static designs into interactive mobile applications can be daunting. How do you ensure pixel-perfect accuracy? What are the best practices for making this transition smooth and efficient? In this guide, we'll take you through a step-by-step process to help you bridge the gap between design and development seamlessly.
Step 1: Understanding the Figma Design Structure
Before jumping into development, it's crucial to dissect the Figma design properly.
1.1 Organizing Components and Layers
Figma allows designers to use frames, components, and auto-layouts. Ensuring that the design is well-structured will make it easier to convert to React Native.
1.2 Extracting Design Elements
Use the Figma inspect tool to extract colors, fonts, spacing, and other design details. This helps developers replicate the design accurately.
1.3 Exporting Assets
Export images and icons in the appropriate formats such as SVG or PNG. Optimize assets to ensure performance efficiency in React Native.
Step 2: Setting Up the React Native Environment
Now that you have the design elements, it's time to set up the React Native project.
2.1 Installing Dependencies
Ensure you have Node.js, React Native CLI (or Expo for a managed workflow), and the necessary libraries installed:
npx react-native init MyApp # For bare React Native setup
Or if using Expo:
npx create-expo-app MyApp
2.2 Choosing a UI Library
To speed up development, you can use UI component libraries like React Native Paper or NativeBase that provide pre-built components styled according to Material Design or other UI paradigms.
Step 3: Converting Figma Components to React Native Components
This is where the magic happens—converting static UI into dynamic code.
3.1 Mapping Figma Components to Code
Buttons & Inputs →
<Button />
&<TextInput />
Typography →
<Text />
Containers →
<View />
3.2 Implementing the Design Using Flexbox
React Native uses Flexbox for layout. Define styles in StyleSheet
objects to maintain consistency:
import { View, Text, StyleSheet } from 'react-native';
const Header = () => (
<View style={styles.container}>
<Text style={styles.title}>Welcome</Text>
</View>
);
const styles = StyleSheet.create({
container: { padding: 20, backgroundColor: '#f8f9fa' },
title: { fontSize: 24, fontWeight: 'bold' }
});
export default Header;
Step 4: Adding Navigation and Interactivity
A well-designed UI isn’t just about looks—it must also function seamlessly.
4.1 Setting Up React Navigation
Use React Navigation to manage screens and transitions.
npm install @react-navigation/native
Example of a simple stack navigator:
import { createStackNavigator } from '@react-navigation/stack';
import { NavigationContainer } from '@react-navigation/native';
const Stack = createStackNavigator();
export default function App() {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
4.2 Implementing State Management
For managing global state, consider using Context API, Redux, or Zustand.
import { useState } from 'react';
const [count, setCount] = useState(0);
Step 5: Optimizing Performance and Responsiveness
A great UI is not only beautiful but also smooth and responsive.
5.1 Implementing Responsive Design
Use Dimensions API and libraries like react-native-responsive-screen
to ensure adaptability across devices.
import { Dimensions } from 'react-native';
const { width, height } = Dimensions.get('window');
5.2 Enhancing Performance
Optimize images using react-native-fast-image
Reduce unnecessary re-renders with memoization techniques
Conclusion
Converting a Figma design into a React Native application requires careful planning and execution. By following these steps—understanding the design, setting up the environment, implementing UI components, and optimizing performance—you can ensure a smooth transition from design to code. The key takeaway? Good communication between designers and developers is essential to achieve a seamless UI/UX experience.
Ready to bring your Figma designs to life? Start coding today and watch your designs transform into fully functional apps!
Subscribe to my newsletter
Read articles from James Smith directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

James Smith
James Smith
A highly skilled Mobile App Developer with expertise in both Android and iOS app development. With years of experience building intuitive, scalable, and high-performance applications, James specializes in creating seamless user experiences across mobile platforms. Proficient in a wide range of programming languages and frameworks, including Java, Kotlin, Swift, and Flutter, James delivers custom solutions tailored to client needs. Known for a keen eye for detail and problem-solving abilities, he consistently develops apps that meet the latest industry standards while ensuring optimal functionality. Whether working independently or as part of a team, James brings technical expertise, creativity, and a passion for innovation to every project.