Control Flow in JavaScript

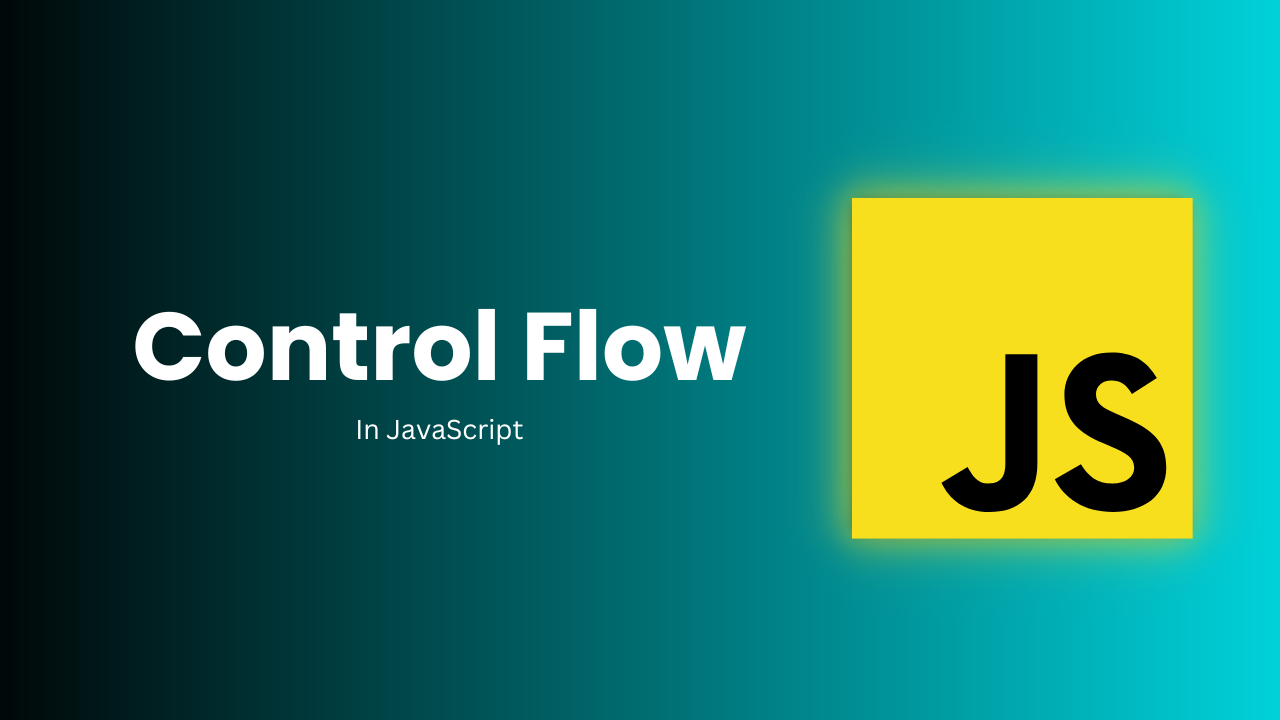
Having the control over the flow of execution of code in any programming language is very necessary to get the desired output. Just like any other programming language JavaScript also provides us the flow control statements for us. Control statements allows us to run specific block of code depending on some condition, If the condition is true some block of code is executed if not another block is executed. There are two types of control statements in JavaScript :
Conditional statements - theses are executed based on some condition. Used for decision making.
Loop/Iteration statements - theses executes specific block of code n times unless and until the condition is met. User to perform task repeatedly.
In this article we are only going to study about the conditional statements.
Conditional Statements
A conditional statements are set of commands that gets executed only if the specified condition it true
JavaScript offer us two conditional statements if-else
statement and switch
statement. This are used to make decision based on given condition, that’s why are referred as decision making statements. Let’s understand them one by one.
if-else statement
if-else statement is used to perform some action based on give condition. it has if
statement along with the condition and an optional else
block. if-else
block takes action based on the result of condition. if condition evaluates to be true
it executes if
block if it evaluates to be false
it executes else
block.
The syntax of if-else statements looks like below
// syntax
if (condition) {
statement1;
} else {
statement2;
}
note: condition should always evaluates to be either as
true
or asfalse
.
let’s take an example where you want to determine is user is able to vote based on his age. If age is greater than 18 he is eligible to vote if it is less than 18 he is not eligible to vote.
let age = 21
if (age >= 18) {
console.log("You are eligible to vote");
} else {
console.log("You are eligible to vote");
}
//output : "You are eligible to vote"
we can have multiple if-else-if-else
statements to check for multiple conditions depending on the requirement. which ever if
condition evaluates to true
, execution will go inside to that if
block.
this is also known as if-else ladder statements, it follows the below syntax
if(condition)
statement1;
else if(condition)
statement2;
else if(condition)
statement3;
...
else
statement4;
let’s take an example where we want and determine students grade based on his score.
if (score >= 90) {
console.log("A");
} else if (score >= 80) {
console.log("B");
} else if (score >= 70) {
console.log("C");
} else if (score >= 60) {
console.log("D");
} else {
console.log("F");
}
switch statement
switch
statement executes a give condition / expression and try match the result of expression to given case
labels. if it finds the match it executes that particular case
block.
syntax of switch
case looks like below
switch (expression) {
case label1:
statements1;
break;
case label2:
statements2;
break;
// …
default:
statementsDefault;
}
the switch
case
matches the expression value to case
label, if any match is found it executes that case block. If no match is found it executes the default
case.
note: In
switch
statementbreak
statement is very important for each block. if nobreak
statement is found, it executes all the case block below from where match is found till last orbreak
statement is found.break
limits the execution only to that block.
let’s take an example or traffic light, where we want perform some action based on given color of light.
let color = "Yellow";
switch(color) {
case "Red":
console.log("Stop");
break;
case "Yellow":
console.log("Go Slow");
break;
case "Green":
console.log("Go");
break;
default:
console.log("Invalid Color");
}
//output : "Go Slow"
Summary
This article discusses the importance of control statements in JavaScript, focusing specifically on conditional statements. It explores the two main types: if-else statements and switch statements. An if-else statement executes code blocks based on whether a specified condition is true or false. An if-else ladder is used for multiple condition checks. The switch statement evaluates an expression against case labels and executes the matching case block, with the break statement ensuring only the matched block is executed. Examples illustrate how these structures work in practice.
Subscribe to my newsletter
Read articles from Ganesh Ghadage directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
