Arrow function vs Normal function in JavaScript
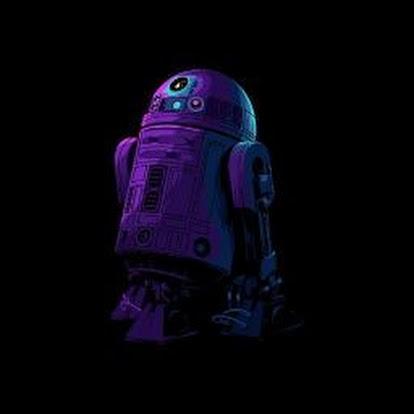
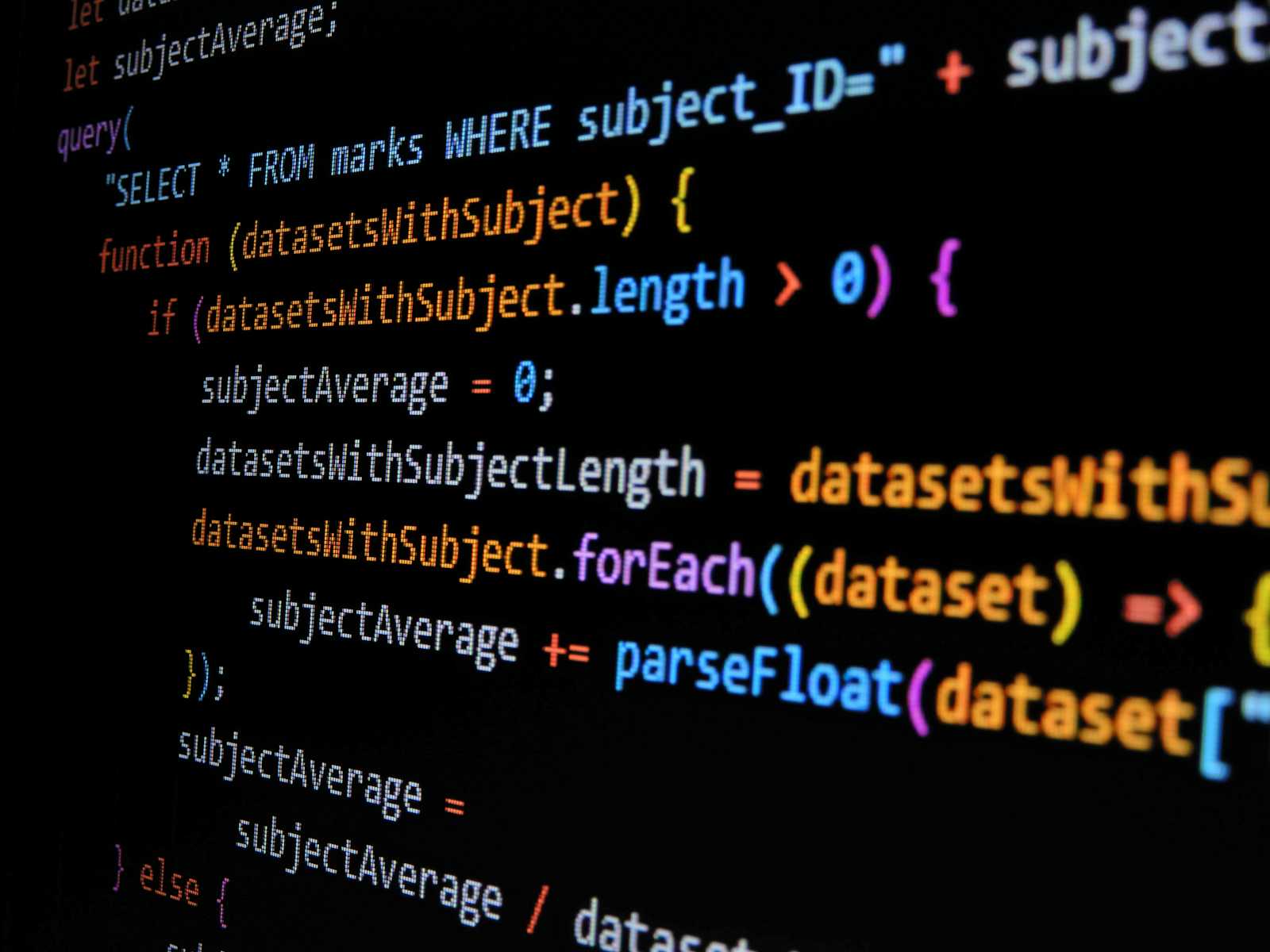
Javascript provides two ways to define functions : Normal function with function keyword and arrow function with =>
syntax . While both serve similar purposes, they have key differences . Lets discuss these differences one by one.
1.Syntax Difference
Normal Function
Javascript uses function keyword to define normal functions
function normalFunction(name){
console.log("hello ", name)
}
normalFunction("Aniket");
Arrow Function
For Arrow functions javascript allows to use const keyword to write the function name followed by =
and parenthesis ( )
to pass the arguments and then =>
arrow sign (with no spaces between them).
const arrowFunction = (name) => {
console.log("hello ", name)
}
arrowFunction("Aniket")
Now in both the functions the output is going to be the same but since you are using const in the arrow functions syntax then there will hoisting properties of const will be applied to this function i.e now you can not use the function before defining it.
Let’s see an example :
normalFunction("aniket") //No error
function normalFunction(name){
console.log("Hello ", name)
}
arrowFunction("aniket") //Will cause error
const arrowFunction = (name) => {
console.log("Hello ", name)
}
2. this
Behavior
In Normal function ( this depends on how the function is called ) and in Arrow function ( this is lexically inherited )
Let’s see it with example :
const obj = {
value: 10,
normalFunction: function() {
console.log(this.value); // here 'this' refers to obj
},
arrowFunction: () => {
console.log(this.value); // here 'this' refers to the global object (undefined in strict mode)
}
};
obj.normalFunction(); // Output: 10
obj.arrowFunction(); // Output: undefined (in strict mode) or depends on the environment (e.g. window in browsers)
In the above example you can see that in arrow function the behavior of this is undefined and depends on the environment .Try running the code in debugger mode for better understanding.
3. arguments
Object
Normal functions have access to the arguments object but in case of arrow function the argument object is different and not includes the argument array.
function normalFunction() {
console.log({argsfromnormal: arguments});
}
const arrowFunction = () => {
console.log({argsfromarrow : arguments});
};
normalFunction(1, 2, 3); // Output: Arguments(3) [1, 2, 3]
arrowFunction(1, 2, 3); // logs big arguments object
4. Duplicate parameters
In normal functions the duplicate functions are allowed and function uses the value of the last used duplicate parameter, while in arrow functions using duplicate parameters give you error.
function normalFuncWithDuplicateParams(a, a, b) {
console.log(a, b);
}
normalFuncWithDuplicateParams(1, 2, 3); // Output: 2, 3
const arrowFuncWithDuplicateParams = (c, c, d) => {
console.log(c, d);
}
arrowFuncWithDuplicateParams(4, 5, 6); // throws SyntaxError: Duplicate parameter name not allowed in this context
5. Constructors
In javascript you can only use normal function to write constructors with this keyword, when you use arrow function to write constructor in javascript it throws you error.
function normalConstructor(name) {
this.name = name;
}
const normal = new normalConstructor("Aniket");
console.log(normal.name); // Output: Aniket
const arrowConstructor = (name) => {
this.name = name;
};
const arrow = new arrowConstructor("Aniket"); // Error: PersonArrow is not a constructor
These are some of the main difference between arrow function and normal function in javascript. Do run these sample code in debugger mode in you IDE to get a more clearer perspective.
Subscribe to my newsletter
Read articles from Aniket Vishwakarma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
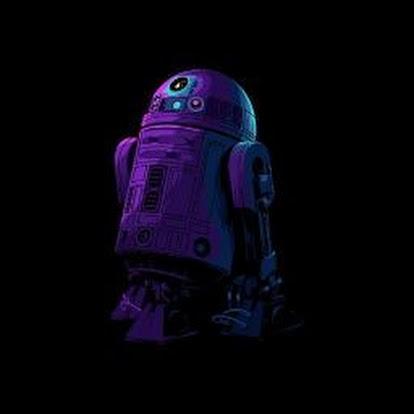
Aniket Vishwakarma
Aniket Vishwakarma
I am currently a final year grad, grinding, learning and solving problems by leveraging technology and my soft skills