🚀 JavaScript Operators and Expressions: A Complete Guide 🎯

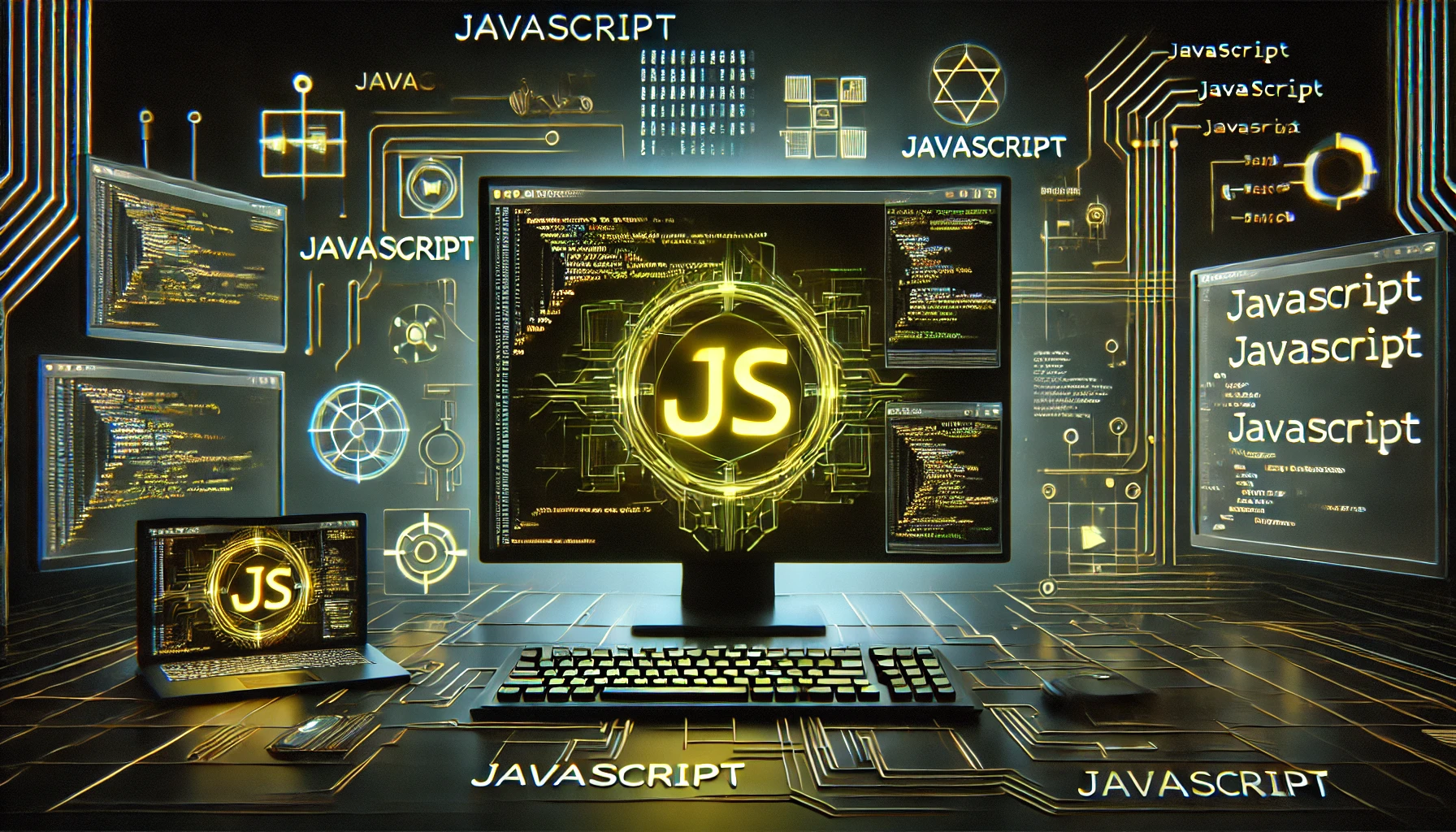
🌟 What are Operators in JavaScript?
Operators in JavaScript are symbols that perform operations on values and variables. They help in performing mathematical, logical, comparison, and other operations.
🔹 Types of Operators in JavaScript
JavaScript has several types of operators:
1️⃣ Arithmetic Operators 2️⃣ Assignment Operators 3️⃣ Comparison Operators 4️⃣ Logical Operators 5️⃣ Bitwise Operators 6️⃣ String Operators 7️⃣ Ternary Operators
🔥 1️⃣ Arithmetic Operators (Used for mathematical calculations)
let a = 10;
let b = 5;
console.log(a + b); // Addition -> 15
console.log(a - b); // Subtraction -> 5
console.log(a b); // Multiplication -> 50
console.log(a / b); // Division -> 2
console.log(a % b); // Modulus -> 0
console.log(a **\ b); // Exponentiation -> 100000
🎯 2️⃣ Assignment Operators (Assign values to variables)
let x = 10;
x += 5; // x = x + 5 -> 15
x -= 2; // x = x - 2 -> 13
x *\= 3; // x = x 3 -> 39
x /= 3; // x = x / 3 -> 13
✅ 3️⃣ Comparison Operators (Compare values and return true/false)
console.log(10 == "10"); // true (loose equality, converts types)
console.log(10 === "10"); // false (strict equality, checks type too)
console.log(10 != 5); // true
console.log(10 > 5); // true
console.log(10 < 5); // false
🔥 4️⃣ Logical Operators (Used for combining conditions)
let isJSFun = true;
let isEasy = false;
console.log(isJSFun && isEasy); // false (AND operator)
console.log(isJSFun || isEasy); // true (OR operator)
console.log(!isJSFun); // false (NOT operator)
🎯 5️⃣ Bitwise Operators (Operate at the binary level)
console.log(5 & 1); // AND -> 1
console.log(5 | 1); // OR -> 5
console.log(5 ^ 1); // XOR -> 4
console.log(~5); // NOT -> -6
🔥 6️⃣ String Operators (Used to concatenate strings)
let firstName = "Vivek";
let lastName = "Varshney";
console.log(firstName + " " + lastName); // "Vivek Varshney"
🎯 7️⃣ Ternary Operator (Shorter if-else statement)
let age = 20;
let status = age >= 18 ? "Adult" : "Minor";
console.log(status); // "Adult"
🚀 Conclusion
Operators and expressions are the backbone of JavaScript logic. Understanding them helps in writing efficient and powerful code! 🔥
🎯 What's Next?
In the next blog, we will explore JavaScript Functions and Scope in detail. Stay tuned! 🚀
📌 Read More on: vivekwebdev.hashnode.dev 🚀
Subscribe to my newsletter
Read articles from Vivek varshney directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vivek varshney
Vivek varshney
Full-Stack Web Developer | Blogging About Tech, React.js, Angular, Node.js & Web Development. Turning Ideas into Code & Helping Businesses Grow!