Mistakes and best practices with async & await patterns in C#

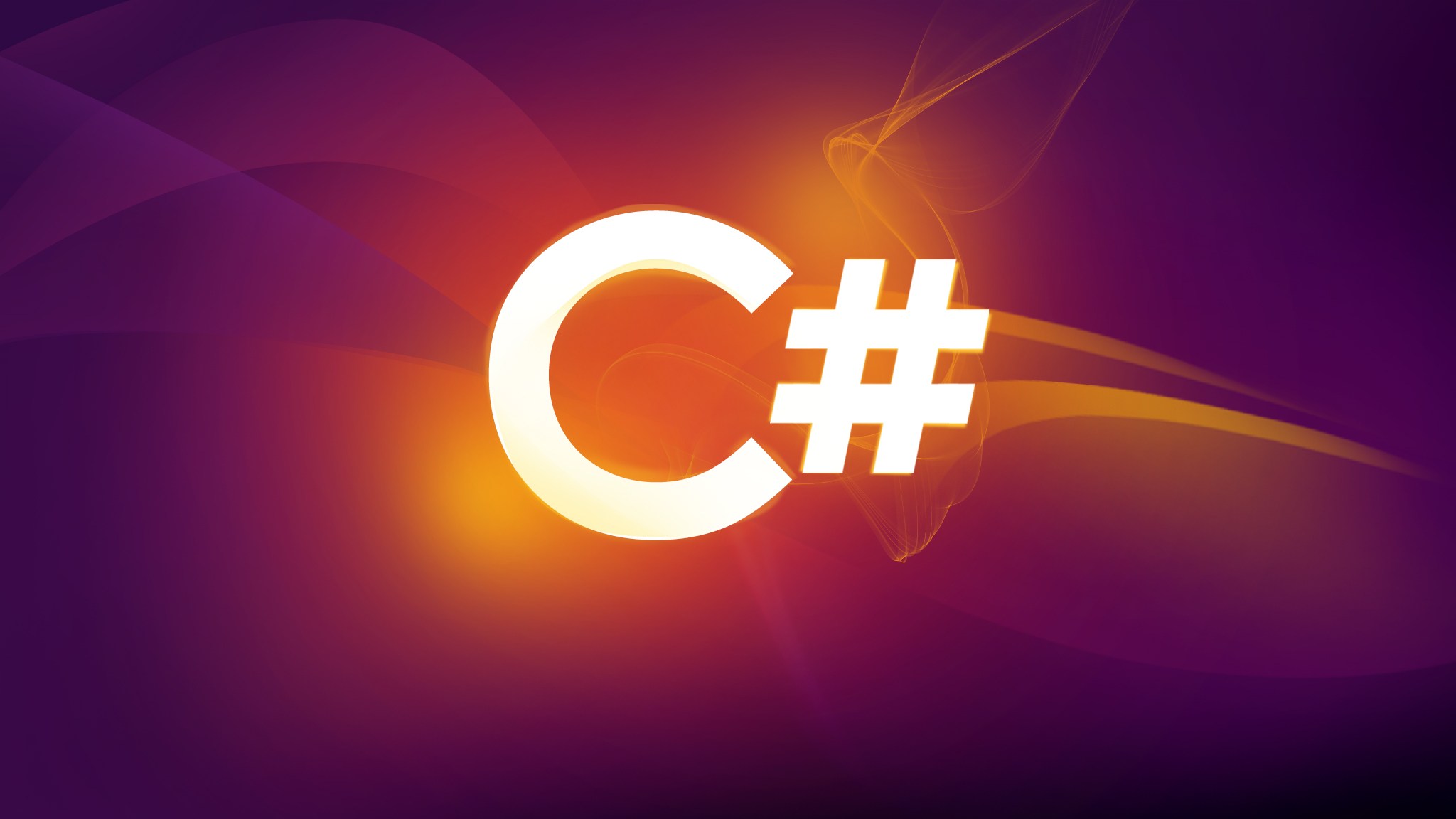
Asynchronous programming is an essential component of modern software development, particularly in C# and the .NET framework, due to its capability to greatly enhance application responsiveness and resource efficiency.
One of the most frequent and critical errors involves using async void
methods. Although convenient, async void
lacks proper exception handling capabilities. This means if an exception occurs within such a method, it can lead to unhandled exceptions, causing the application to terminate unexpectedly or behave unpredictably. A best practice is always to use async Task
or async Task<T>
instead, as this enables robust exception handling and better control over asynchronous workflows.
Another prevalent mistake is forgetting to await asynchronous methods correctly. Omitting the await
keyword can cause asynchronous operations to execute in parallel unintentionally, potentially leading to race conditions or unpredictable execution order. Properly awaiting tasks ensures operations complete in the intended sequence, maintaining the logical flow and predictability of your code.
A further issue arises when mixing synchronous and asynchronous code incorrectly, particularly when using .Result
or .Wait()
in an asynchronous context. Such synchronous calls within asynchronous methods can cause thread-pool starvation or even deadlocks, especially in environments such as UI applications or web services. The recommended practice is to maintain asynchronous methods end-to-end through your application's stack, consistently using await
and avoiding synchronous blockers entirely.
Implementing cancellation tokens is another important best practice in asynchronous programming. Cancellation tokens allow developers to gracefully handle task cancellations, enhancing the responsiveness and robustness of applications. Proper implementation ensures that tasks can be stopped promptly without resource leaks or incomplete state handling, particularly in applications involving long-running operations.
Lastly, developers should be cautious of exception handling patterns within asynchronous methods. Catching exceptions within tasks and correctly propagating them to calling methods is crucial. Using try-catch blocks around asynchronous operations helps manage errors effectively, ensuring your application can recover or provide meaningful feedback when something goes wrong.
By understanding these common mistakes and best practices in async and await patterns, developers can write clearer, safer, and more maintainable asynchronous code, significantly improving overall application stability and user experience
Subscribe to my newsletter
Read articles from Patrick Kearns directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
