Mastering Behavioral Design Pattern in Go

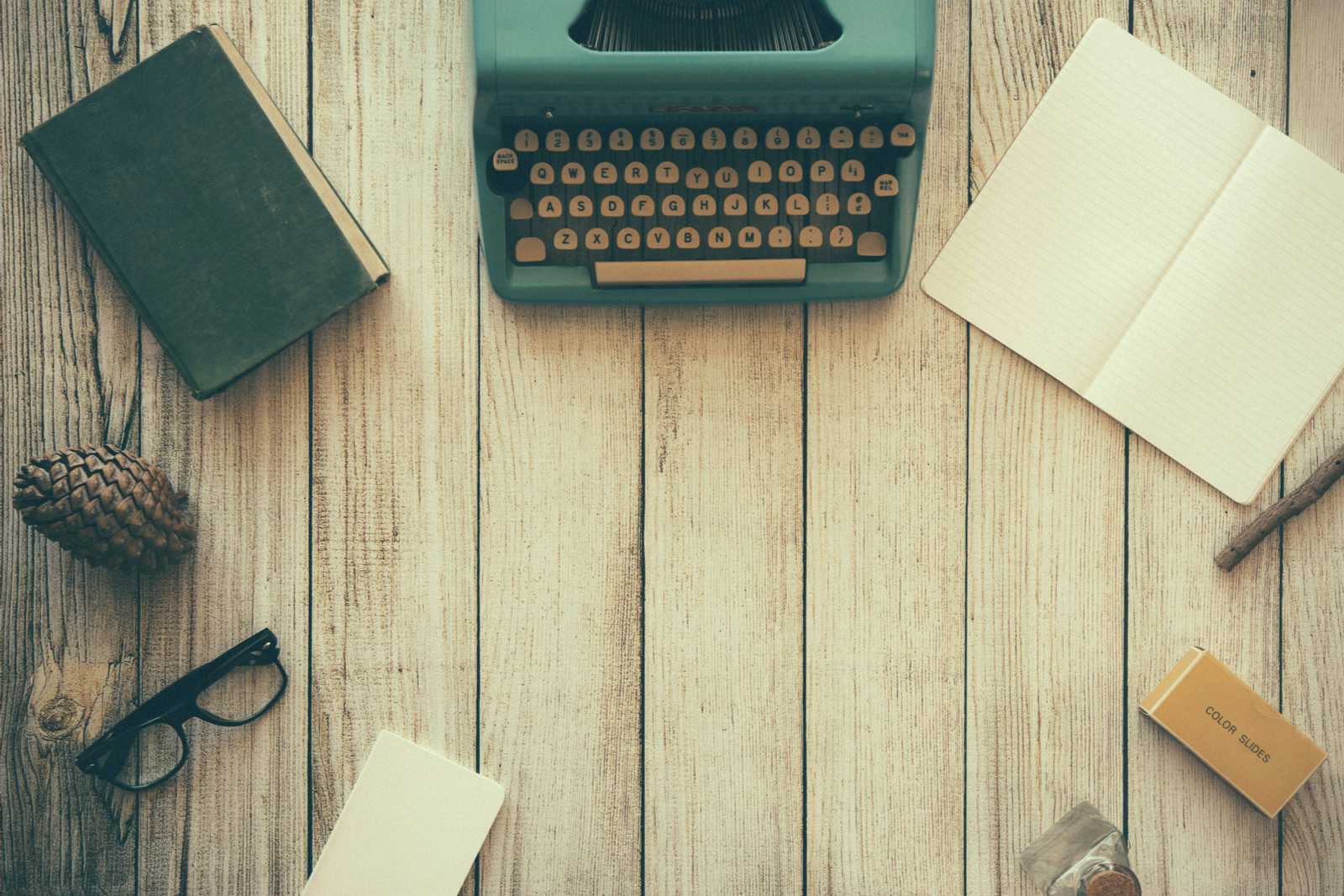
Behavioral patterns focus on how objects interact and communicate with each other. They help define clear communication and delegation mechanisms while keeping the code loosely coupled.
Common Behavioral Design Patterns
1. Strategy Pattern
The Strategy Pattern is a behavioral design pattern that allows you to define a family of algorithms, encapsulate each one, and make them interchangeable. This pattern enables a client to select an algorithm at runtime instead of implementing multiple conditions in the code.
It promotes Open/Closed Principle, meaning new strategies (algorithms) can be added without modifying existing code.
When to Use the Strategy Pattern?
When you have multiple ways to perform a task and want to switch between them dynamically.
When you want to remove complex
if-else
orswitch-case
conditions.When different behaviours should be easily interchangeable.
Implementation in GO
package main
import "fmt"
// PaymentStrategy defines a common interface for all payment methods
type PaymentStrategy interface {
Pay(amount float64)
}
// CreditCardPayment is a concrete strategy
type CreditCardPayment struct{}
func (c *CreditCardPayment) Pay(amount float64) {
fmt.Printf("Paid $%.2f using Credit Card\n", amount)
}
// PayPalPayment is another concrete strategy
type PayPalPayment struct{}
func (p *PayPalPayment) Pay(amount float64) {
fmt.Printf("Paid $%.2f using PayPal\n", amount)
}
// BitcoinPayment is another concrete strategy
type BitcoinPayment struct{}
func (b *BitcoinPayment) Pay(amount float64) {
fmt.Printf("Paid $%.2f using Bitcoin\n", amount)
}
// PaymentContext holds a payment strategy
type PaymentContext struct {
strategy PaymentStrategy
}
// SetStrategy allows changing the payment method dynamically
func (p *PaymentContext) SetStrategy(strategy PaymentStrategy) {
p.strategy = strategy
}
// ExecutePayment delegates payment to the selected strategy
func (p *PaymentContext) ExecutePayment(amount float64) {
p.strategy.Pay(amount)
}
func main() {
// Create a payment context
paymentContext := &PaymentContext{}
// Use Credit Card payment strategy
paymentContext.SetStrategy(&CreditCardPayment{})
paymentContext.ExecutePayment(100.0)
// Switch to PayPal payment strategy
paymentContext.SetStrategy(&PayPalPayment{})
paymentContext.ExecutePayment(50.0)
// Switch to Bitcoin payment strategy
paymentContext.SetStrategy(&BitcoinPayment{})
paymentContext.ExecutePayment(30.0)
}
2. Observer Pattern
The Observer Pattern is a behavioral design pattern that allows one object (the Subject) to notify multiple other objects (Observers) about state changes. This pattern is useful when you want to implement a publisher-subscriber model without tightly coupling the components.
When to Use the Observer Pattern?
When multiple objects need to be notified of changes in another object.
When implementing event-driven programming (e.g., UI event listeners, real-time notifications).
When reducing dependencies between objects, making the system more scalable.
Implementation in GO
A news publisher system where subscribers (observers) get notified whenever a new article is published.
package main
import "fmt"
// Observer interface that all subscribers must implement
type Observer interface {
Update(news string)
}
// Subject interface defining methods for registering, unregistering, and notifying observers
type Subject interface {
Register(observer Observer)
Unregister(observer Observer)
NotifyAll()
}
// NewsPublisher is the concrete subject (publisher)
type NewsPublisher struct {
observers []Observer // List of subscribers
news string
}
// Register adds a new observer
func (p *NewsPublisher) Register(observer Observer) {
p.observers = append(p.observers, observer)
}
// Unregister removes an observer
func (p *NewsPublisher) Unregister(observer Observer) {
for i, obs := range p.observers {
if obs == observer {
p.observers = append(p.observers[:i], p.observers[i+1:]...)
break
}
}
}
// NotifyAll sends the latest news update to all registered observers
func (p *NewsPublisher) NotifyAll() {
for _, observer := range p.observers {
observer.Update(p.news)
}
}
// PublishNews updates the news and notifies observers
func (p *NewsPublisher) PublishNews(news string) {
fmt.Println("\nPublishing News:", news)
p.news = news
p.NotifyAll()
}
// EmailSubscriber receives news updates via email
type EmailSubscriber struct {
email string
}
func (e *EmailSubscriber) Update(news string) {
fmt.Printf("Email sent to %s: %s\n", e.email, news)
}
// SMSSubscriber receives news updates via SMS
type SMSSubscriber struct {
phoneNumber string
}
func (s *SMSSubscriber) Update(news string) {
fmt.Printf("SMS sent to %s: %s\n", s.phoneNumber, news)
}
func main() {
publisher := &NewsPublisher{}
// Create subscribers
emailSubscriber := &EmailSubscriber{email: "user@example.com"}
smsSubscriber := &SMSSubscriber{phoneNumber: "+123456789"}
// Register subscribers
publisher.Register(emailSubscriber)
publisher.Register(smsSubscriber)
// Publish news updates
publisher.PublishNews("Go 1.22 Released!")
publisher.PublishNews("Observer Pattern in Go Explained!")
// Unsubscribe SMS subscriber
publisher.Unregister(smsSubscriber)
publisher.PublishNews("New Go Concurrency Features!")
}
3. Command Pattern
The Command Pattern is a behavioral design pattern that encapsulates a request as an object. This allows parameterizing clients with different requests, queueing requests, and logging request history.
In simpler terms, this pattern helps decouple the sender (invoker) from the receiver (Executor) by encapsulating operations into command objects.
When to Use the Command Pattern?
Need to queue, log, or undo operations.
Want to parameterize objects with operations (e.g., GUI menu items).
Need to decouple the sender and receiver of a request.
Implementing macro commands where one command consists of multiple smaller commands.
Implementation in GO
Let’s implement a remote control system where we can execute, undo, and queue commands.
package main
import "fmt"
// Command interface that all commands must implement
type Command interface {
Execute()
Undo()
}
// Light represents a receiver (the actual business logic)
type Light struct {
isOn bool
}
// TurnOn turns the light on
func (l *Light) TurnOn() {
l.isOn = true
fmt.Println("Light is ON")
}
// TurnOff turns the light off
func (l *Light) TurnOff() {
l.isOn = false
fmt.Println("Light is OFF")
}
// LightOnCommand is a concrete command to turn the light on
type LightOnCommand struct {
light *Light
}
func (c *LightOnCommand) Execute() {
c.light.TurnOn()
}
func (c *LightOnCommand) Undo() {
c.light.TurnOff()
}
// LightOffCommand is a concrete command to turn the light off
type LightOffCommand struct {
light *Light
}
func (c *LightOffCommand) Execute() {
c.light.TurnOff()
}
func (c *LightOffCommand) Undo() {
c.light.TurnOn()
}
// RemoteControl acts as an invoker, triggering commands
type RemoteControl struct {
command Command
}
func (r *RemoteControl) SetCommand(command Command) {
r.command = command
}
func (r *RemoteControl) PressButton() {
r.command.Execute()
}
func (r *RemoteControl) PressUndo() {
r.command.Undo()
}
func main() {
light := &Light{}
// Create command objects
lightOn := &LightOnCommand{light: light}
lightOff := &LightOffCommand{light: light}
// Remote control (Invoker)
remote := &RemoteControl{}
// Turn light ON
remote.SetCommand(lightOn)
remote.PressButton()
// Undo turning light ON
remote.PressUndo()
// Turn light OFF
remote.SetCommand(lightOff)
remote.PressButton()
// Undo turning light OFF
remote.PressUndo()
}
4. Chain of Responsibility Pattern
The Chain of Responsibility (CoR) Pattern is a behavioral design pattern that allows multiple handlers to process a request sequentially. Each handler in the chain decides either to process the request or pass it to the next handler in the chain.
When to Use the Chain of Responsibility Pattern?
Need to process a request through multiple handlers without tightly coupling them.
Want to allow multiple objects a chance to handle a request dynamically at runtime.
Need to avoid excessive conditional logic (
if-else
orswitch-case
) for request handling.Implementing a middleware pipeline (e.g., HTTP request processing).
Implementation in GO
A request validation system where a request goes through a series of handlers (authentication, logging, and execution).
package main
import "fmt"
// Request struct represents a client request
type Request struct {
user string
payload string
}
// Handler interface for processing requests
type Handler interface {
SetNext(handler Handler)
Handle(request *Request)
}
// BaseHandler struct provides default implementation of SetNext
type BaseHandler struct {
next Handler
}
// SetNext assigns the next handler in the chain
func (h *BaseHandler) SetNext(handler Handler) {
h.next = handler
}
// PassToNext calls the next handler if it exists
func (h *BaseHandler) PassToNext(request *Request) {
if h.next != nil {
h.next.Handle(request)
}
}
// AuthHandler checks user authentication
type AuthHandler struct {
BaseHandler
}
func (a *AuthHandler) Handle(request *Request) {
if request.user == "" {
fmt.Println("Authentication Failed: No user provided")
return
}
fmt.Println("Authentication Passed")
a.PassToNext(request)
}
// LogHandler logs the request
type LogHandler struct {
BaseHandler
}
func (l *LogHandler) Handle(request *Request) {
fmt.Println("Logging Request:", request.payload)
l.PassToNext(request)
}
// ExecuteHandler processes the request
type ExecuteHandler struct {
BaseHandler
}
func (e *ExecuteHandler) Handle(request *Request) {
fmt.Println("Executing Request:", request.payload)
}
func main() {
// Create handlers
auth := &AuthHandler{}
logger := &LogHandler{}
executor := &ExecuteHandler{}
// Build the chain: auth -> logger -> executor
auth.SetNext(logger)
logger.SetNext(executor)
// Create request and process it
request := &Request{user: "JohnDoe", payload: "Process Data"}
auth.Handle(request) // Starts the chain
fmt.Println("\nProcessing request without authentication:")
request2 := &Request{user: "", payload: "Unauthorized Access"}
auth.Handle(request2)
}
5. State Pattern
The State Pattern is a behavioral design pattern that allows an object to change its behavior when its internal state changes. Instead of using conditionals (if-else
or switch-case
), the state-specific behavior is encapsulated into separate state objects.
When to Use the State Pattern?
Need an object’s behavior to change dynamically based on its state.
Want to avoid complex conditional logic (
if-else
chains for different states).Need to encapsulate state-specific behavior into separate classes for better maintainability.
Implementing finite state machines (e.g., ATMs, traffic lights, vending machines).
Implementation in GO
A Vending Machine that has different states (Ready, HasMoney, Dispensing).
package main
import "fmt"
// State interface that defines methods for the vending machine states
type State interface {
InsertMoney(amount int)
DispenseItem()
}
// VendingMachine represents the context with a current state
type VendingMachine struct {
state State
balance int
}
// SetState changes the vending machine's current state
func (vm *VendingMachine) SetState(state State) {
vm.state = state
}
// InsertMoney delegates to the current state
func (vm *VendingMachine) InsertMoney(amount int) {
vm.state.InsertMoney(amount)
}
// DispenseItem delegates to the current state
func (vm *VendingMachine) DispenseItem() {
vm.state.DispenseItem()
}
// ReadyState allows money insertion
type ReadyState struct {
vendingMachine *VendingMachine
}
func (s *ReadyState) InsertMoney(amount int) {
fmt.Printf("Money inserted: $%d\n", amount)
s.vendingMachine.balance += amount
s.vendingMachine.SetState(&HasMoneyState{vendingMachine: s.vendingMachine})
}
func (s *ReadyState) DispenseItem() {
fmt.Println("Insert money first!")
}
// HasMoneyState allows item dispensing
type HasMoneyState struct {
vendingMachine *VendingMachine
}
func (s *HasMoneyState) InsertMoney(amount int) {
fmt.Println("Already have enough money. Dispense item first.")
}
func (s *HasMoneyState) DispenseItem() {
if s.vendingMachine.balance < 10 {
fmt.Println("Not enough money! Insert at least $10.")
return
}
fmt.Println("Dispensing item...")
s.vendingMachine.balance -= 10
s.vendingMachine.SetState(&ReadyState{vendingMachine: s.vendingMachine})
}
func main() {
vm := &VendingMachine{state: &ReadyState{}}
// Trying to dispense without inserting money
vm.DispenseItem() // Insert money first!
// Insert money and try again
vm.InsertMoney(10) // Money inserted: $10
vm.DispenseItem() // Dispensing item...
// Insert money again and try to dispense
vm.InsertMoney(5) // Money inserted: $5
vm.DispenseItem() // Not enough money! Insert at least $10
}
6. Template Method Pattern
The Template Method Pattern is a behavioral design pattern that defines the skeleton of an algorithm in a base class but allows subclasses to override specific steps of the algorithm without changing its structure.
This pattern is useful when multiple classes share a common structure but need custom implementations for certain steps.
When to Use the Template Method Pattern?
Need to define a common algorithm structure but allow customization of specific steps.
Avoid code duplication when multiple classes have similar workflows.
Ensure a standardized sequence of steps in different implementations.
Useful in frameworks where base classes define workflows, and users implement specific steps.
Implementation in GO
package main
import "fmt"
// Beverage interface defines the template method
type Beverage interface {
BoilWater()
Brew()
PourInCup()
AddCondiments()
}
// Template struct embedding common steps
type Template struct{}
func (t *Template) BoilWater() {
fmt.Println("Boiling water")
}
func (t *Template) PourInCup() {
fmt.Println("Pouring into cup")
}
// Tea struct following the template
type Tea struct {
Template
}
func (t *Tea) Brew() {
fmt.Println("Steeping the tea")
}
func (t *Tea) AddCondiments() {
fmt.Println("Adding lemon")
}
// Coffee struct following the template
type Coffee struct {
Template
}
func (c *Coffee) Brew() {
fmt.Println("Brewing the coffee")
}
func (c *Coffee) AddCondiments() {
fmt.Println("Adding sugar and milk")
}
// PrepareBeverage is the template method executing the common sequence
func PrepareBeverage(b Beverage) {
b.BoilWater()
b.Brew()
b.PourInCup()
b.AddCondiments()
fmt.Println()
}
func main() {
fmt.Println("Making Tea:")
PrepareBeverage(&Tea{})
fmt.Println("Making Coffee:")
PrepareBeverage(&Coffee{})
}
7. Iterator Pattern
The Iterator Pattern is a behavioral design pattern that provides a way to access elements of a collection sequentially without exposing its underlying representation (e.g., arrays, lists, maps).
This pattern helps in iterating over complex data structures without modifying their structure, keeping the code clean, extensible, and decoupled.
When to Use the Iterator Pattern?
Need a consistent way to traverse a collection without exposing its internal structure.
Want to provide different iteration strategies (e.g., forward, backward, filtering).
Need to decouple iteration logic from the collection implementation.
Useful when working with custom data structures that don't support standard iteration.
Implementation in GO
package main
import "fmt"
// Book represents an item in the collection
type Book struct {
Title string
}
// Iterator interface defines methods for iteration
type Iterator interface {
HasNext() bool
Next() *Book
}
// BookCollection holds a list of books
type BookCollection struct {
books []*Book
}
// BookIterator implements the Iterator interface
type BookIterator struct {
collection *BookCollection
index int
}
// HasNext checks if there are more elements
func (b *BookIterator) HasNext() bool {
return b.index < len(b.collection.books)
}
// Next returns the next element and moves the iterator forward
func (b *BookIterator) Next() *Book {
if !b.HasNext() {
return nil
}
book := b.collection.books[b.index]
b.index++
return book
}
// CreateIterator returns a new iterator for the collection
func (bc *BookCollection) CreateIterator() Iterator {
return &BookIterator{collection: bc}
}
func main() {
// Creating a collection of books
books := &BookCollection{
books: []*Book{
{Title: "The Go Programming Language"},
{Title: "Clean Code"},
{Title: "Design Patterns"},
},
}
// Creating an iterator for the collection
iterator := books.CreateIterator()
// Iterating over the collection
for iterator.HasNext() {
book := iterator.Next()
fmt.Println("Book:", book.Title)
}
}
8. Mediator Pattern
The Mediator Pattern is a behavioral design pattern that helps reduce direct dependencies between objects by introducing a mediator object that facilitates communication.
Instead of objects communicating directly, they send messages to the mediator, which handles and routes the communication.
When to Use the Mediator Pattern?
Need to reduce coupling between objects that frequently interact.
Want to centralize complex communication logic instead of spreading it across multiple objects.
Useful when multiple objects interact in a many-to-many relationship (e.g., chat applications, UI components).
Need to simplify object interactions in event-driven systems.
Implementation in GO
A Chat Room where multiple users communicate via a mediator (chat room) instead of directly messaging each other.
package main
import "fmt"
// Mediator interface defines a communication contract
type Mediator interface {
SendMessage(sender string, message string)
}
// ChatRoom is the concrete mediator that facilitates communication
type ChatRoom struct {
users map[string]*User
}
// NewChatRoom creates a new chat room instance
func NewChatRoom() *ChatRoom {
return &ChatRoom{users: make(map[string]*User)}
}
// RegisterUser adds a user to the chat room
func (c *ChatRoom) RegisterUser(user *User) {
c.users[user.name] = user
user.chatRoom = c
}
// SendMessage allows users to send messages via the chat room
func (c *ChatRoom) SendMessage(sender string, message string) {
for name, user := range c.users {
if name != sender { // Do not send message back to the sender
user.ReceiveMessage(sender, message)
}
}
}
// User represents a participant in the chat room
type User struct {
name string
chatRoom Mediator
}
// SendMessage sends a message via the mediator
func (u *User) SendMessage(message string) {
fmt.Printf("%s sends: %s\n", u.name, message)
u.chatRoom.SendMessage(u.name, message)
}
// ReceiveMessage is called by the chat room to deliver messages
func (u *User) ReceiveMessage(sender string, message string) {
fmt.Printf("%s received from %s: %s\n", u.name, sender, message)
}
func main() {
// Create chat room (mediator)
chatRoom := NewChatRoom()
// Create users
alice := &User{name: "Alice"}
bob := &User{name: "Bob"}
charlie := &User{name: "Charlie"}
// Register users in the chat room
chatRoom.RegisterUser(alice)
chatRoom.RegisterUser(bob)
chatRoom.RegisterUser(charlie)
// Users communicate via the chat room
alice.SendMessage("Hello, everyone!")
bob.SendMessage("Hi Alice!")
}
9. Memento Pattern
The Memento Pattern is a behavioral design pattern that allows an object to save and restore its state without exposing its internal structure.
It is mainly used for undo/redo functionality in applications, where an object’s previous state needs to be stored and retrieved later.
When to Use the Memento Pattern?
Need to implement undo/redo functionality in applications.
Want to store and restore an object’s state without breaking encapsulation.
Useful in applications like text editors, game state saving, and transactional memory systems.
Implementation in GO
A text editor that allows users to write text and undo changes using the Memento Pattern.
package main
import "fmt"
// Memento stores the state of the editor
type Memento struct {
state string
}
// Editor represents a text editor with undo functionality
type Editor struct {
content string
}
// Save creates a memento with the current state
func (e *Editor) Save() Memento {
return Memento{state: e.content}
}
// Restore sets the editor's state from a memento
func (e *Editor) Restore(m Memento) {
e.content = m.state
}
// Write updates the editor content
func (e *Editor) Write(text string) {
e.content = text
}
// Show displays the current content
func (e *Editor) Show() {
fmt.Println("Content:", e.content)
}
// Caretaker manages memento history
type Caretaker struct {
history []Memento
}
// Backup saves the current state
func (c *Caretaker) Backup(e *Editor) {
c.history = append(c.history, e.Save())
}
// Undo restores the last saved state
func (c *Caretaker) Undo(e *Editor) {
if len(c.history) == 0 {
fmt.Println("No undo available")
return
}
lastState := c.history[len(c.history)-1]
c.history = c.history[:len(c.history)-1] // Remove last state
e.Restore(lastState)
}
func main() {
editor := &Editor{}
caretaker := &Caretaker{}
editor.Write("Hello, World!")
editor.Show()
caretaker.Backup(editor) // Save state
editor.Write("Hello, Go!")
editor.Show()
caretaker.Undo(editor) // Restore previous state
editor.Show()
}
10. Visitor Pattern
The Visitor Pattern is a behavioral design pattern that allows adding new operations to existing object structures without modifying their code.
Instead of modifying the objects, a separate visitor object is used to perform operations on them. This is useful when you want to extend functionality without changing the existing structure.
When to Use the Visitor Pattern?
Need to add new behaviors to a complex object structure without modifying its classes.
Want to separate algorithms from object structure for better maintainability.
Useful when working with complex data structures like ASTs, file systems, or UI hierarchies.
Implementation in GO
package main
import (
"fmt"
"math"
)
// Shape interface accepts a visitor
type Shape interface {
Accept(visitor Visitor)
}
// Circle struct
type Circle struct {
Radius float64
}
// Square struct
type Square struct {
Side float64
}
// Visitor interface declares a visit method for each shape
type Visitor interface {
VisitCircle(c *Circle)
VisitSquare(s *Square)
}
// AreaCalculator is a concrete visitor that calculates the area
type AreaCalculator struct{}
// VisitCircle calculates the area of a circle
func (a *AreaCalculator) VisitCircle(c *Circle) {
area := math.Pi * c.Radius * c.Radius
fmt.Printf("Circle Area: %.2f\n", area)
}
// VisitSquare calculates the area of a square
func (a *AreaCalculator) VisitSquare(s *Square) {
area := s.Side * s.Side
fmt.Printf("Square Area: %.2f\n", area)
}
// Accept methods allow shapes to accept a visitor
func (c *Circle) Accept(visitor Visitor) {
visitor.VisitCircle(c)
}
func (s *Square) Accept(visitor Visitor) {
visitor.VisitSquare(s)
}
func main() {
// Create shapes
circle := &Circle{Radius: 5}
square := &Square{Side: 4}
// Create visitor
areaCalculator := &AreaCalculator{}
// Let visitor perform operations
circle.Accept(areaCalculator)
square.Accept(areaCalculator)
}
11. Interpreter Pattern
The Interpreter Pattern is a behavioral design pattern that defines a grammar for a language and provides an interpreter to process and evaluate expressions written in that language.
It is commonly used in parsing expressions, command execution, compilers, and rule engines.
When to Use the Interpreter Pattern?
Need to interpret and evaluate expressions or sentences from a defined language or grammar.
Useful for parsing mathematical expressions, SQL queries, or programming language commands.
When implementing domain-specific languages (DSLs).
Implementation in Go
package main
import (
"fmt"
"strconv"
"strings"
)
// Expression interface represents an expression that can be interpreted
type Expression interface {
Interpret() int
}
// Number represents a numeric value
type Number struct {
value int
}
// Interpret returns the number itself
func (n *Number) Interpret() int {
return n.value
}
// Add represents an addition operation
type Add struct {
left, right Expression
}
// Interpret evaluates the addition of left and right expressions
func (a *Add) Interpret() int {
return a.left.Interpret() + a.right.Interpret()
}
// Subtract represents a subtraction operation
type Subtract struct {
left, right Expression
}
// Interpret evaluates the subtraction of right from left
func (s *Subtract) Interpret() int {
return s.left.Interpret() - s.right.Interpret()
}
// ParseExpression parses a simple expression like "5 + 3 - 2"
func ParseExpression(input string) Expression {
tokens := strings.Split(input, " ")
var stack []Expression
for _, token := range tokens {
switch token {
case "+":
right := stack[len(stack)-1]
stack = stack[:len(stack)-1]
left := stack[len(stack)-1]
stack = stack[:len(stack)-1]
stack = append(stack, &Add{left, right})
case "-":
right := stack[len(stack)-1]
stack = stack[:len(stack)-1]
left := stack[len(stack)-1]
stack = stack[:len(stack)-1]
stack = append(stack, &Subtract{left, right})
default:
value, _ := strconv.Atoi(token)
stack = append(stack, &Number{value})
}
}
return stack[0]
}
func main() {
expression := "5 + 3 - 2"
fmt.Println("Expression:", expression)
parsedExpression := ParseExpression(expression)
result := parsedExpression.Interpret()
fmt.Println("Result:", result)
}
Conclusion
Behavioral design patterns play a crucial role in defining how objects interact while ensuring loose coupling and maintainability. These patterns enhance flexibility by allowing systems to evolve without major rewrites, making them scalable and adaptable.
By understanding and applying behavioral design patterns, developers can build robust, reusable, and efficient solutions that streamline object communication and improve code quality. Whether it’s handling events (Observer), managing state transitions (State), or defining reusable algorithms (Template Method), these patterns provide powerful tools to tackle complex software design challenges.
Mastering behavioral patterns will significantly improve your problem-solving skills, making you a more effective software engineer. 🚀 Keep exploring, keep coding! 💡
Thank you for following along through this journey. I hope you found it helpful and informative. Feel free to reach out to Gyanesh Sharma
Subscribe to my newsletter
Read articles from Gyanesh Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
