Comparing Bulk Write and Simple Write in MongoDB: Choosing the Optimal Approach for Large Data Ingestion
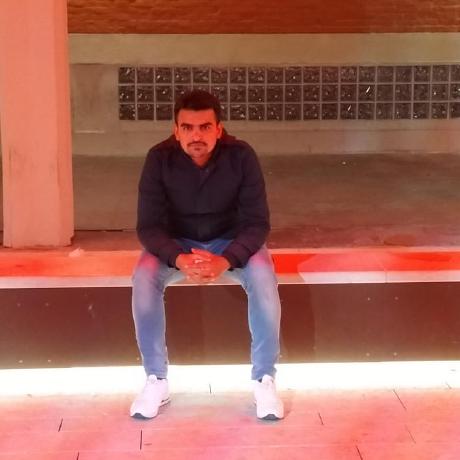

When working with MongoDB to handle significant amounts of data, the choice between bulk write and simple write operations becomes a critical factor in optimizing performance and resource utilization. Each method has its strengths and trade-offs, and understanding their characteristics can help developers choose the best strategy for their specific use case.
Simple Write Operations
Simple writes involve executing individual write commands, such as insertOne()
, updateOne()
, or deleteOne()
, for each document. This approach is straightforward, easy to implement, and suitable for smaller datasets or operations requiring high granularity.
Advantages of Simple Write Operations
Ease of Debugging: Errors are easier to pinpoint as operations are processed sequentially.
Fine-grained Control: Each operation can be tailored to specific conditions, making it ideal for complex or conditional writes.
Low Overhead: Best suited for scenarios where the volume of data is minimal.
Disadvantages of Simple Write Operations
Performance Bottlenecks: For large datasets, executing individual writes can cause significant performance degradation due to frequent network round trips and overhead.
Lack of Batch Optimization: MongoDB cannot optimize write operations at scale, leading to inefficiencies.
Bulk Write Operations
Bulk write operations allow developers to perform multiple write commands in a single call, such as insertMany()
or bulkWrite()
. This method is highly efficient for handling large datasets.
Advantages of Bulk Write Operations
Improved Performance: Combining multiple operations into a single network call reduces latency and minimizes resource contention.
Atomicity: Bulk writes support atomic operations within ordered batches, ensuring either all or none of the operations in a batch are executed.
Scalability: Ideal for high-throughput applications or scenarios requiring ingestion of large volumes of data.
Disadvantages of Bulk Write Operations
Complexity: Debugging can be more challenging as multiple operations are executed simultaneously.
Memory Overhead: Larger batch sizes can consume significant server resources, requiring careful tuning.
Performance Considerations
To illustrate the impact of these approaches, consider the following examples:
Simple Write Example
const data = Array.from({ length: 10000 }, (_, i) => ({ key: `value_${i}` })); data.forEach(async (doc) => { await collection.insertOne(doc); });
This approach results in 10,000 individual write operations.
Each operation incurs network latency, making it inefficient for large datasets.
Bulk Write Example
const data = Array.from({ length: 10000 }, (_, i) => ({ key: `value_${i}` })); await collection.insertMany(data);
- A single command writes all 10,000 documents, significantly reducing network calls.
Best Practices for Bulk Write Operations
Batch Size Management
Keep batch sizes between 500 and 1,000 documents to balance performance and memory usage.
MongoDB imposes a 16MB limit on document size per batch.
Error Handling
Use the
ordered: false
option for non-critical writes to allow the operation to proceed even if some documents fail.Example:
const bulkOps = [ { insertOne: { document: { key: "value1" } } }, { updateOne: { filter: { key: "value2" }, update: { $set: { key: "new_value" } } } }, ]; await collection.bulkWrite(bulkOps, { ordered: false });
Monitoring and Tuning
Monitor write performance using tools like MongoDB Atlas Performance Advisor or database profiling.
Optimize indexes before bulk writes to ensure minimal query execution time.
Validation and Schema Design
- Use validation rules or ORMs like Mongoose to ensure data consistency during bulk operations.
Conclusion
For scenarios involving large data ingestion in MongoDB, bulk write operations offer substantial performance advantages over simple writes. They minimize network overhead, improve scalability, and reduce latency. However, simple writes remain useful for smaller datasets or cases requiring precise control over individual operations.
By implementing best practices such as batch size management, proper error handling, and monitoring, developers can leverage MongoDB's bulk write capabilities to efficiently handle large-scale data operations while maintaining reliability and performance.
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
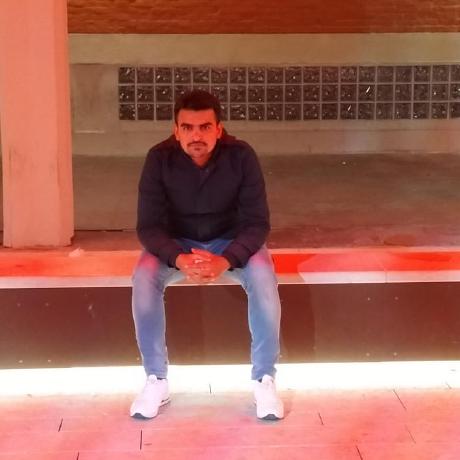
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.