Arrays

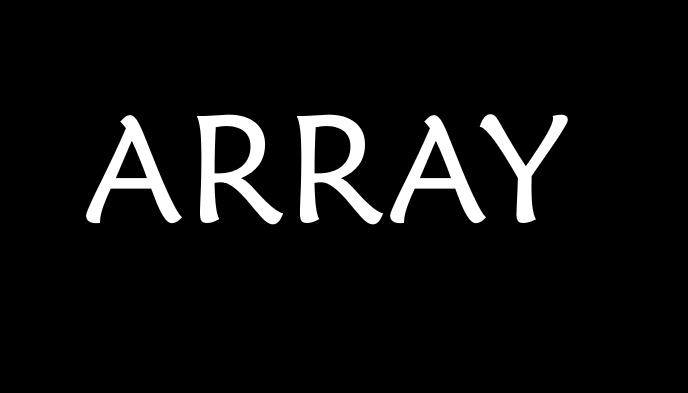
Arrays
It can store multiple values in a single variable. It can also store mix values of different data types.
Its value is not stored directly into the memory, reference is stored in the memory.
Arrays are zero indexed. First element is at index 0, second is at 1, and so on.
Ways to declare an array
let language = ["Html", "css", "Javascript"]
let color = new Array("Green", "Blue", "Red", "Yellow")
Accessing and modifying elements of array
let language = ["Html", "css", "Javascript"]
console.log(language[0]); // Html
console.log(language[1]); // css
console.log(language[2]); // Javascript
language[1] = "Bootstrap";
console.log(language) // ["Html", "Bootstrap", "Javascript"]
Some built-in properties and methods of array
length - return the number of elements present in the array.
let language = ["Html", "css", "Javascript"] console.log(language.length); // output: 3
forEach() - use to traverse on each element of array, does not return any value.
let language = ['Html', 'css', 'Javascript', 'Django', 'PHP', 'Laravel'] language.forEach((item) => { console.log(item); // print array element one by one in console window })
push() - it add elements in the last of the array and returns number of elements present in array after adding new element.
let language = ["Html", "css", "Javascript"] let count = language.push("Django"); console.log(language) // ['Html', 'css', 'Javascript', 'Django'] console.log(count) // 4 // we can also add more than one items at a time in the end of array language.push("PHP", "Laravel"); console.log(language); // ['Html', 'css', 'Javascript', 'Django', 'PHP', 'Laravel']
pop() - it delete the last element of the array and return it.
let language = ['Html', 'css', 'Javascript', 'Django', 'PHP', 'Laravel'] let deletedItem = language.pop(); console.log(language); // ['Html', 'css', 'Javascript', 'Django', 'PHP'] console.log(deletedItem); // Laravel
unshift() - it add elements in the start of the array and returns number of elements.
let language = ["Html", "css", "Javascript"] let count = language.unshift("Django"); console.log(language) // ['Django', 'Html', 'css', 'Javascript'] console.log(count) // 4 // we can also add more than one items at a time in the start of array language.unshift("PHP", "Laravel"); console.log(language); // ['PHP', 'Laravel', 'Django','Html', 'css', 'Javascript']
shift() - it removes first item and return the deleted item.
let language = ['PHP', 'Laravel', 'Django','Html', 'css', 'Javascript'] let deletedItem = language.shift(); console.log(language); // ['Laravel', 'Django','Html', 'css', 'Javascript'] console.log(deletedItem); // PHP
splice() - it is use to remove or replace or add elements in existing array and returns the deleted items, if no item is deleted it returns empty array.
syntax: splice(start, deleteCount, item1, item2, /* …, */ itemN)
let language = ['PHP', 'Laravel', 'Django','Html', 'css', 'Javascript']; let deletedLanguage = language.splice(4); // delete items from index 4 till end of the array console.log(language); // ['PHP', 'Laravel', 'Django','Html'] console.log(deletedLanguage); // ['css', 'Javascript']
let language = ['PHP', 'Laravel', 'Django','Html', 'css', 'Javascript']; let deletedLanguage = language.splice(3,2); // delete 2 items starting from index 3 console.log(language); // ['PHP', 'Laravel', 'Django', 'Javascript'] console.log(deletedLanguage); // ['Html', 'css']
let language = ['PHP', 'Laravel', 'Django','Html', 'css', 'Javascript']; let deletedLanguage = language.splice(4,1,"Bootstrap","Tailwind"); // delete 1 item starting from index 4 and add two items Bootstrap and Tailwind at index 4 console.log(language); // ['PHP', 'Laravel', 'Django', 'Html', 'Bootstrap', 'Tailwind', 'Javascript'] console.log(deletedLanguage); // ['css']
includes() - it determine whether array has certain value and based on that return true or false.
let number = [2, 3, 5, 7]; let isPresent = number.includes(5); // true console.log(isPresent);
indexOf() - it returns the first index of element in given array, if element not present it returns -1.
let number = [2, 3, 5, 2, 3, 7]; let idx = number.indexOf(3); // 3 is present at index 1 and 4 console.log(idx); // 1 (first position of 3)
map() - it creates a new array with the results of provided function on every element in calling array.
let arr1 = [2, 6, 1, 9]; let map1 = arr1.map((x) => x*2 ); console.log(map1); // [4, 12, 2, 18]
filter() - filter the elements from given array that pass the provided function and store it in array and return it.
let language = ['PHP', 'Laravel', 'Django','Html', 'css', 'Javascript']; let languageFilter = language.filter( (word) => word.length > 4 ); console.log(languageFilter);
sort() - use to sort the array.
let arr1 = [1, 30, 4, 201, 100]; arr1.sort(); // lexicographical (dictionary order) sorting console.log(arr1); // [1, 100, 201, 30, 4]
let arr1 = [1, 30, 4, 201, 100]; // a-b < 0 (a < b, a comes before b) // a-b > 0 (a > b, b comes before a) // a-b = 0 (a = b, position remains unchanged) arr1.sort((a,b) => a-b ); //sort the array in ascending order console.log(arr1); // [1, 4, 30, 100, 201]
reduce() - traverse on each element of array, in starting accumulator value is intialValue, and currentValue is current item of array. each item accumulator value gets updated in last it is returned.
let arr = [1, 2, 3, 4]; let initialValue = 0; let sumWithInitial = arr.reduce( (accumulator,currentValue) => accumulator + currentValue, initialValue ); console.log(sumWithInitial); // 10 // accumulator = initialValue = 0 and currentValue = arr[0] = 1, accumulator = 0(accumulator) + 1(currentValue) = 1 // accumulator = 1 and currentValue = arr[1] = 2, accumulator = 1 + 2 = 3 // accumulator = 3 and currentValue = arr[2] = 3, accumulator = 3 + 3 = 6 // accumulator = 6 and currentValue = arr[3] = 4, accumulator = 6 + 4 = 10 // sumWithInitial = 10
Subscribe to my newsletter
Read articles from Ayushi Anand directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
