Understanding JSX and How It Works in React

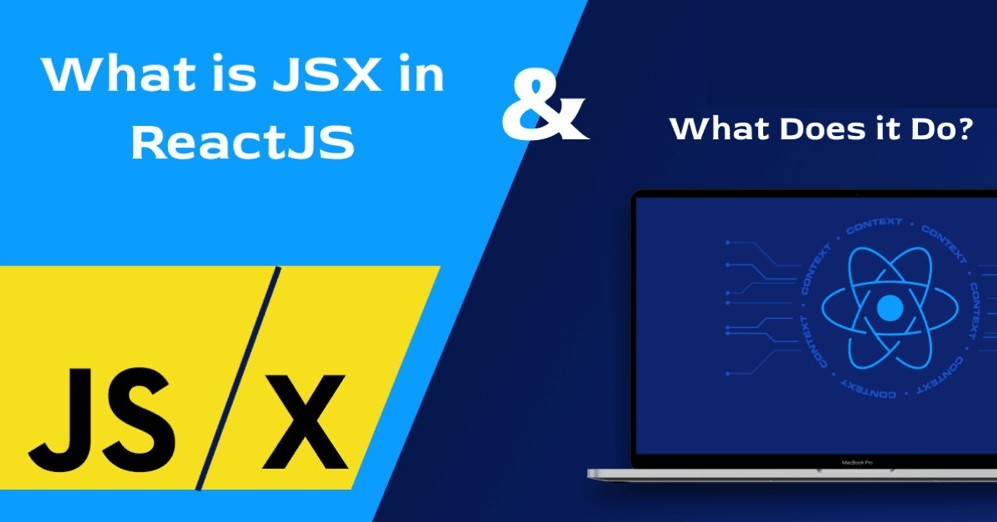
If you are learning React, you have probably encountered JSX. It might look like HTML, but it's actually a powerful JavaScript syntax extension. In this blog, we'll break down JSX, how it works, and why it makes building UI components in React easier.
What is JSX?
JSX (JavaScript XML) is a syntax extension for JavaScript that allows us to write HTML-like code inside JavaScript. It makes React code more readable and expressive by allowing developers to describe the UI structure in a way that resembles HTML.
For example, instead of using React.createElement
to build elements, we can write:
const element = <h1>Hello, World!</h1>;
This looks like HTML but is actually JavaScript under the hood.
How JSX Works Under the Hood
JSX is not understood by browsers directly. Instead, it is compiled into JavaScript using Babel before being executed. When we write JSX like this:
const element = <h1>Hello, World!</h1>;
It gets transformed into:
const element = React.createElement('h1', null, 'Hello, World!');
React.createElement
generates a JavaScript object that represents the element, and React uses this object to update the Virtual DOM efficiently.
Why Use JSX?
JSX comes with several benefits:
Improved Readability: It makes the code easier to read and write compared to using plain JavaScript.
Better Developer Experience: JSX allows us to write UI elements in a way that feels more natural.
Prevents Injection Attacks: React escapes any values embedded inside JSX, preventing cross-site scripting (XSS) attacks.
Easier Debugging: Since JSX is closer to HTML, debugging UI-related issues becomes more intuitive.
JSX Rules to Keep in Mind
While JSX is easy to use, there are some important rules to follow:
1. Elements Must Have a Single Parent
JSX expressions must return a single parent element. If you need to return multiple elements, wrap them in a <div>
or use <>
(React Fragments):
return (
<>
<h1>Title</h1>
<p>Description</p>
</>
);
2. JSX Attributes Use CamelCase
Unlike HTML, JSX attributes follow camelCase notation. For example:
<input type="text" autoComplete="off" />
3. Embedding JavaScript in JSX
We can embed JavaScript expressions inside JSX using curly braces {}
:
const name = "Yasin";
const element = <h1>Hello, {name}!</h1>;
4. Class is className
Since class
is a reserved keyword in JavaScript, JSX uses className
for CSS classes:
<div className="container">Hello</div>
JSX in React Components
JSX is commonly used inside React components. A basic functional component with JSX looks like this:
function Welcome() {
return <h1>Welcome to React!</h1>;
}
For dynamic content, we can pass props and use JSX to render them:
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
Conclusion
JSX makes writing React components intuitive and expressive. It allows developers to write UI elements in a way that closely resembles HTML while leveraging JavaScript’s power. By understanding how JSX works under the hood, you can write better and more efficient React applications.
If you are new to React, keep practicing JSX, and you'll see how it simplifies front-end development!
🚀 Happy coding!
Subscribe to my newsletter
Read articles from Yasin Sarkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Yasin Sarkar
Yasin Sarkar
Front-End Developer. I create dynamic web applications using HTML, Tailwind CSS, JavaScript, React, and Next.js. I share my knowledge on social media to help others enhance their tech skills. An Open Source Enthusiast and Writer.