The abstract Keyword in Java

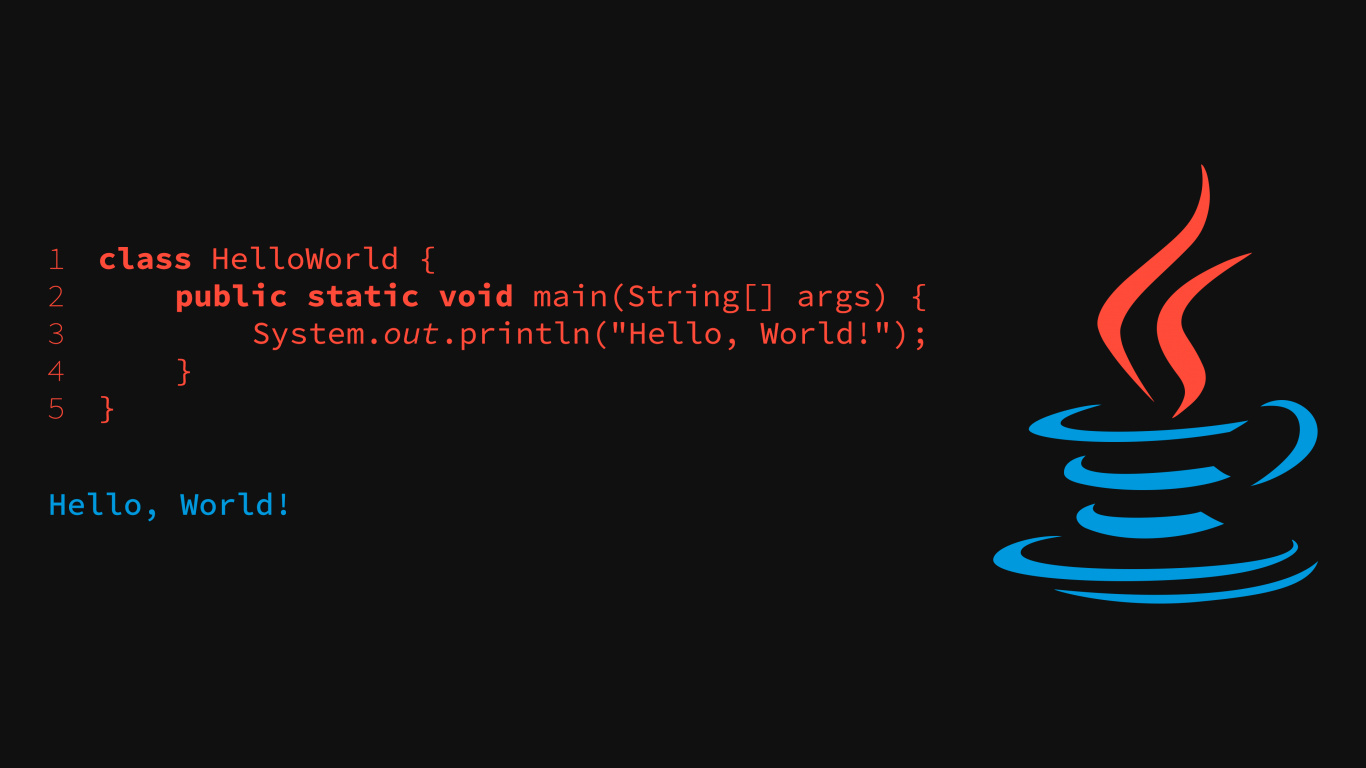
The abstract
keyword in Java is used to define abstract classes and abstract methods. An abstract class cannot be instantiated and is meant to be subclassed by concrete classes. Abstract methods, declared within an abstract class, do not have a body and must be implemented by subclasses.
This article explores the usage, benefits, and best practices of the abstract
keyword in Java with code examples.
Abstract Classes
An abstract class in Java is a class that cannot be instantiated directly. It may contain abstract methods (methods without a body) and concrete methods (methods with a body). Abstract classes are typically used to define a common base class for related classes.
Syntax
abstract class Animal {
abstract void makeSound(); // Abstract method
void sleep() { // Concrete method
System.out.println("Sleeping...");
}
}
Example
class Dog extends Animal {
@Override
void makeSound() {
System.out.println("Bark!");
}
}
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog();
myDog.makeSound(); // Output: Bark!
myDog.sleep(); // Output: Sleeping...
}
}
Abstract Methods
An abstract method is a method that has no implementation in the abstract class. Subclasses must provide their own implementation.
Example
abstract class Vehicle {
abstract void start();
}
class Car extends Vehicle {
@Override
void start() {
System.out.println("Car is starting...");
}
}
Rules for Abstract Classes and Methods
An abstract class cannot be instantiated.
It can contain both abstract and concrete methods.
If a class contains at least one abstract method, it must be declared abstract.
A subclass that extends an abstract class must implement all its abstract methods or be declared abstract itself.
Abstract methods cannot be
static
,final
, orprivate
.
Why Use Abstract Classes?
Code Reusability: Common functionality can be defined in the abstract class.
Polymorphism: Abstract classes allow different implementations for the same method signature.
Enforcing Structure: They provide a blueprint for subclasses.
Differences Between Abstract Classes and Interfaces
Feature | Abstract Class | Interface |
Methods | Can have abstract and concrete methods | Can only have abstract methods (until Java 8) |
Fields | Can have instance variables | Only constants (static and final) |
Constructors | Can have constructors | Cannot have constructors |
Inheritance | Single inheritance allowed | Multiple inheritance possible |
Access Modifiers | Can be public, protected, or private | All methods are public by default |
Example: Combining Abstract Class and Interface
interface Flyable {
void fly();
}
abstract class Bird {
abstract void makeSound();
}
class Sparrow extends Bird implements Flyable {
@Override
void makeSound() {
System.out.println("Chirp chirp!");
}
@Override
public void fly() {
System.out.println("Sparrow is flying...");
}
}
public class Main {
public static void main(String[] args) {
Sparrow sparrow = new Sparrow();
sparrow.makeSound(); // Output: Chirp chirp!
sparrow.fly(); // Output: Sparrow is flying...
}
}
Conclusion
The abstract
keyword in Java is a powerful tool for defining blueprint-like structures for classes. By using abstract classes and methods effectively, you can create flexible, reusable, and maintainable object-oriented designs. Understanding when to use abstract classes versus interfaces will help you design robust Java applications.
Subscribe to my newsletter
Read articles from Ali Rıza Şahin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ali Rıza Şahin
Ali Rıza Şahin
Product-oriented Software Engineer with a solid understanding of web programming fundamentals and software development methodologies such as agile and scrum.