Getting Started with .NET Authorization and AWS Verified Permissions

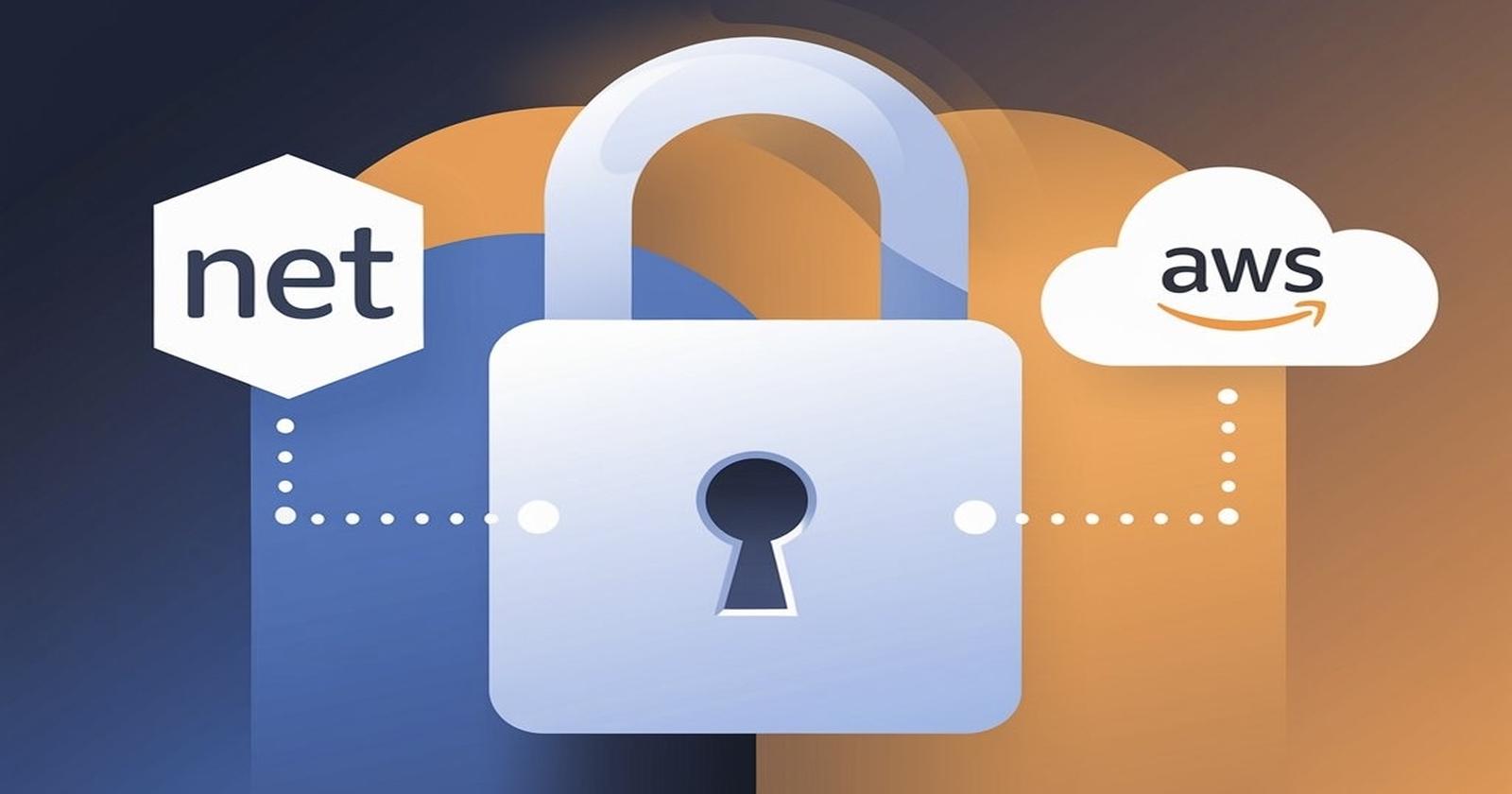
Are you a .NET developer struggling to build secure applications with complex authorization rules? Managing permissions, especially in applications with many users and shared resources, can quickly become a nightmare. Fortunately, there's a better way!
This article introduces Amazon Verified Permissions (AVP), a scalable, fine-grained authorization and permissions management service for custom applications. AVP helps you build secure applications faster by externalizing authorization and centralizing policy management. Using the Cedar policy language, you can define granular permissions to protect your application's resources effectively.
Why This Matters: AVP decouples authorization from application logic, making it easier to manage permissions, audit access, and implement Zero Trust principles. It lets you define policy-based access controls using roles and attributes for context-aware access control.
In this article, we'll cover:
Setting up a policy store in Amazon Verified Permissions.
Understanding the fundamentals of the Cedar policy language.
Integrating AVP with a .NET application.
Prerequisites
Before you get started, make sure you have the following:
AWS Account: An active AWS account.
.NET Development Environment: The .NET SDK installed and a basic understanding of .NET development.
AWS CLI (Optional): The AWS CLI can be helpful for scripting and automation.
Familiarity with IAM (Optional): Basic knowledge of AWS Identity and Access Management (IAM) is beneficial.
Setting Up Amazon Verified Permissions
Access the AWS Management Console: Sign in to the AWS Management Console and navigate to the Amazon Verified Permissions service. Search for "Verified Permissions" in the search bar.
Create a Policy Store: In the Verified Permissions console, choose the option to create a new policy store. A policy store is a central repository for your authorization policies. Give your policy store a descriptive name.
(Optional) Define a Schema: Define a schema for your policy store. The schema defines the structure of your application's entities (users, resources, etc.) and their attributes. Defining a schema allows you to define the shape of the principal, resource, and context that you will use in your policies.
Understanding Cedar Policies
What is Cedar?: Cedar is an open-source policy language used by AVP to define permissions. It's designed to be expressive and analyzable.
Key Components:
Principals: The user or entity requesting access.
Actions: The operation being performed (e.g., read, write, delete).
Resources: The AWS resources being accessed (e.g., databases, application).
Context: Additional information about the request (e.g., time of day, location).
Policy Types:
Permit Policies: Grant permissions.
Forbid Policies: Explicitly deny permissions.
Example Policy: Here's a simple example of a Cedar policy:
permit ( principal == User::"alice", action == Action::"read", resource == Document::"doc1" );
- This policy allows the user "alice" to read the document "doc1". Cedar Policy is based on
principal
,action
andresource
. Each of the arguments are compared with data and then the result isallow
ordeny
depending on the condition met or not.
- This policy allows the user "alice" to read the document "doc1". Cedar Policy is based on
Integrating AVP with a .NET Application
Install the AWS SDK for .NET: Add the AWSSDK.VerifiedPermissions NuGet package to your .NET project. You can do this using the .NET CLI:
dotnet add package AWSSDK.VerifiedPermissions
Configure AWS Credentials: Set up your AWS credentials using environment variables, IAM roles, or the AWS configuration file. The easiest way is to configure your AWS credentials using the AWS CLI:
aws configure
Create a Policy Decision Point (PDP): Implement a PDP in your .NET application using the Amazon Verified Permissions API to evaluate authorization requirements and obtain authorization results. In the PDP, you need to make use of
IsAuthorized
API to check if a user is allowed to perform an action on a resource.Make Authorization Requests: Use the
IsAuthorized
API to check if a user is allowed to perform an action on a resource. This will call Amazon Verified Permissions, evaluate the policies and return a decision to your app.
//Example C# code snippet (this is illustrative - adapt to your library)
using Amazon.VerifiedPermissions;
using Amazon.VerifiedPermissions.Model;
public async Task<bool> IsUserAuthorized(string userId, string action, string resourceId)
{
var client = new AmazonVerifiedPermissionsClient();
var request = new IsAuthorizedRequest
{
PolicyStoreId = "YOUR_POLICY_STORE_ID", // Replace with your Policy Store ID
Principal = new EntityIdentifier
{
EntityType = "User",
EntityId = userId
},
Action = new ActionIdentifier
{
ActionType = "Action",
ActionId = action
},
Resource = new EntityIdentifier
{
EntityType = "Document",
EntityId = resourceId
}
};
var response = await client.IsAuthorizedAsync(request);
return response.Decision == DecisionType.Allow;
}
//Example usage
public async Task<IActionResult> GetDocument(string documentId)
{
//Assume User ID
string userId = "alice";
//Call Amazon Verified Permissions
bool isAuthorized = await IsUserAuthorized(userId, "read", documentId);
if (isAuthorized)
{
//Allow the action
//... Code to retrieve and return the document
return Ok("Document Content");
}
else
{
//Deny the action
return Forbid();
}
}
- Implement Custom Authorization Policy Provider for ASP.NET Core Apps: You can make use of Amazon Verified Permissions API to evaluate authorization requirements and obtain authorization result. This can be done by creating a custom
AuthorizationHandler
in ASP.NET Core.
Testing and Debugging
Evaluation Simulator: Use the AVP console's evaluation simulator to test your policies and ensure they work as expected. You can simulate requests to evaluate how policies behave under different scenarios.
Logging: Implement detailed logging in your .NET application to track authorization requests and decisions. Use structured logging to send authorization data to your logging system.
CloudTrail Integration: Integrate with AWS CloudTrail to record all access requests, which can help with security and auditing. This will help to track changes and access permissions of your app.
Conclusion
In this article, you learned how to get started with .NET Authorization and AWS Verified Permissions. We covered setting up a policy store, understanding the Cedar policy language, and integrating AVP with a .NET application.
Benefits of AVP: By using Amazon Verified Permissions for .NET authorization, you can improve security, simplify policy management, and enhance auditability. You can define, manage, and enforce authorization policies consistently, reducing the risk of authorization-related vulnerabilities and errors.
Next Steps
Explore the AWS Verified Permissions documentation
Experiment with Cedar policies and test different scenarios.
Integrate AVP into your .NET applications and start leveraging its capabilities.
Explore advanced topics like Attribute-Based Access Control (ABAC) and Role-Based Access Control (RBAC) models.
References:
https://www.cedarpolicy.com/en
https://docs.aws.amazon.com/verifiedpermissions/latest/userguide/what-is-avp.html
Subscribe to my newsletter
Read articles from Elmar Guevarra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
