JavaScript Object Property Access: Dot vs. Bracket Notation

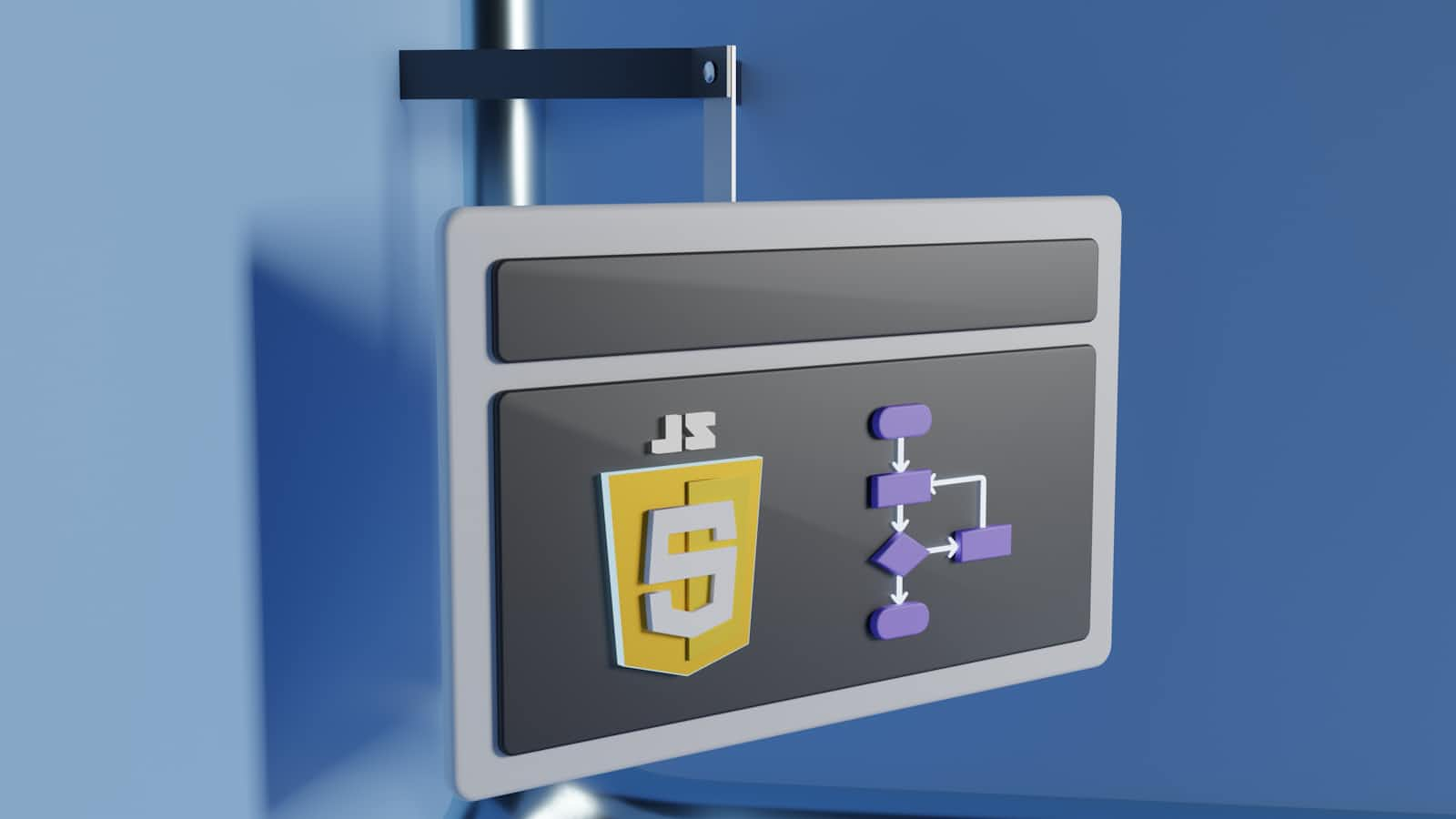
Today as a beginner while learning Javascript I faced a problem that left me confused for quite some time. I was trying to update the values of an object dynamically using a variable as the key. However, instead of updating the intended property, my code either resulted in undefined
or simply didn’t work as expected. Definitely, this is so dumb 😅. I mean I forgot about these concept that Javascript provide two ways to access the property of an object.
Initially, I thought I could access an object’s properties using object.variableName.propertyName
, similar to how we usually navigate objects in Java or other languages. But that wasn't the case! The real issue was understanding how to properly access object properties using the right notation.
After some research, I realized that JavaScript provides two primary ways to access object properties:
1️⃣ Dot Notation (.
)
2️⃣ Bracket Notation ([]
)
This blog is my way of breaking it down for beginners who might be struggling with the same concept. Let’s dive in! 🚀
Understanding JavaScript Objects
An object in JavaScript is a collection of properties, where each property is a key-value pair. Keys are typically strings, while values can be any data type.
Example
let teaTypes = {
name: ["Green Tea", "Black Tea", "Milk Tea", "Herbal Tea"],
price: [30, 25, 35, 40], // Prices in currency units
ingredients: [
["Green tea leaves", "Hot water"],
["Black tea leaves", "Hot water"],
["Black tea leaves", "Milk", "Sugar"],
["Herbs", "Hot water"]
],
available: [true, true, true, false] // Availability status
};
This object now contains:
name
: An array of tea names.price
: An array of prices corresponding to each tea.ingredients
: A nested array listing ingredients for each tea type.available
: A boolean array indicating whether each tea is available.
Two Ways to Access Object Properties
JavaScript allows two primary ways to access properties in an object:
1️⃣ Dot Notation (object.property
)
Dot notation is the most common way to access object properties when the key is fixed and known in advance.
🔹 Example: Accessing Tea Names and Prices
console.log(teaTypes.name); // Output: ["Green Tea", "Black Tea", "Milk Tea", "Herbal Tea"]
console.log(teaTypes.price); // Output: [30, 25, 35, 40]
✅ Works well when the key is a simple, known identifier.
🚨 Limitations of Dot Notation:
Cannot be used with dynamic property names.
Fails if the property name contains spaces or special characters.
Example where dot notation fails:
let key = "price"; console.log(teaTypes.key); // ❌ Wrong! Looks for "key", not "price"
2️⃣ Bracket Notation (object["property"]
)
Bracket notation is more flexible and can handle dynamic property names.
🔹 Example: Accessing Properties Using a Variable
let key = "price";
console.log(teaTypes[key]); // Output: [30, 25, 35, 40]
✅ Works perfectly because teaTypes[key]
translates to teaTypes["price"]
.
🔹 Example: Accessing Specific Tea Information
Suppose we want to get the ingredients for the second tea (Black Tea). We can access it using:
console.log(teaTypes.ingredients[1]);
// Output: ["Black tea leaves", "Hot water"]
✅ teaTypes.ingredients[1]
retrieves the second array inside "ingredients"
.
Practical Example: Checking Tea Availability Dynamically
Let’s say we want to check if "Milk Tea"
is available. Since the availability status is stored as an array, we need to access it dynamically using an index:
let teaIndex = 2 //Index for Milk Tea
console.log(teaTypes.available[teaIndex] //Output: true
✅ Using bracket notation ensures the correct tea’s availability is checked dynamically.
🚨 Common Mistake:
console.log(teaTypes.available.teaIndex); // ❌ Wrong! Looks for "teaIndex" key ths my mistake actually.
console.log(teaTypes.available[teaIndex]); // ✅ Correct //Here, teaIndex is a variable, so we must use bracket notation.
Using reduce()
for a Dynamic Summary
The key role of bracket notation in reduce()
is to access dynamic properties or array indices inside an object. In our example, teaTypes.available[index]
dynamically checks if a tea is available before adding its price. Since index
is a variable, dot notation (teaTypes.available.index
) would fail, making bracket notation (teaTypes.available[index]
) essential for correctly processing data in loops or array methods like reduce()
.
🔹 Code: Summing Prices of Available Teas
let totalTeaPrice = teaTypes.price.reduce((sum, cost, index) =>{
// return teaTypes.available[index] ? sum + cost : sum; //Using ternary ooperator
if(teaTypes.available[index]){
sum = sum + cost
}
return sum
}, 0)
console.log(totalTeaPrice)
✅ teaTypes.available[index]
dynamically checks if the tea is available before adding its price.
Final Thoughts
Navigating JavaScript’s object property access might seem tricky at first, but once you grasp the difference between dot notation and bracket notation, things start to click.
Dot notation works great for fixed property names.
Bracket notation is essential when dealing with dynamic properties or arrays inside objects.
Using
reduce()
effectively means understanding how to access object properties dynamically.
Mastering these concepts will help you write cleaner, more efficient JavaScript—and save you from hours of debugging headaches! 🚀
Subscribe to my newsletter
Read articles from Santwan Pathak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Santwan Pathak
Santwan Pathak
"A beginner in tech with big aspirations. Passionate about web development, AI, and creating impactful solutions. Always learning, always growing."