Interfaces vs Abstract Classes

Table of contents
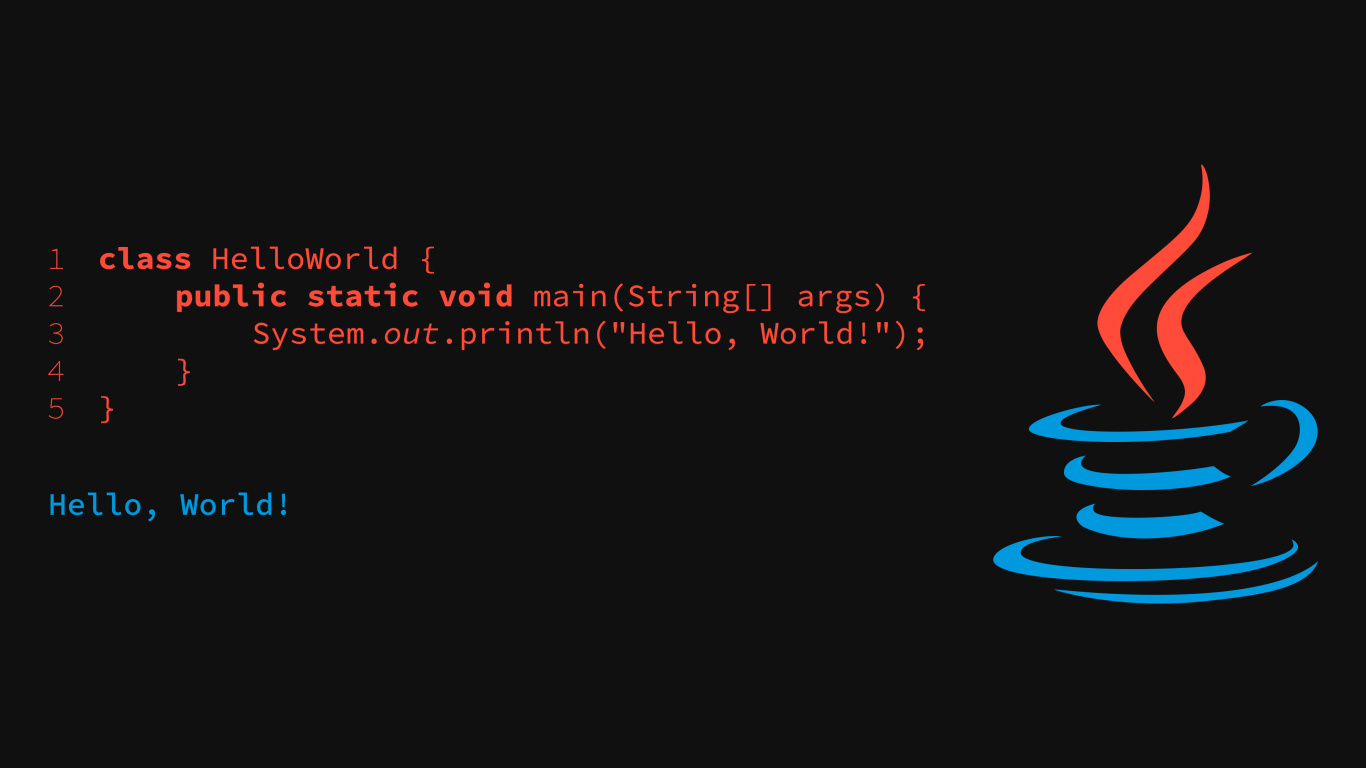
In Java, both interfaces and abstract classes are used to achieve abstraction and define contracts for classes that implement or extend them. However, they serve different purposes and have distinct use cases. This article explores the key differences between interfaces and abstract classes, provides code examples, and explains when to use each.
What is an Interface?
An interface in Java is a collection of abstract methods and static or default methods (since Java 8). It defines a contract that implementing classes must follow but does not provide method implementations (except for default and static methods).
interface Animal {
void makeSound(); // Abstract method (implicitly public and abstract)
default void sleep() {
System.out.println("Sleeping...");
}
static void info() {
System.out.println("This is an Animal interface");
}
}
Characteristics:
Cannot have instance variables (only
public static final
constants).Cannot have constructors.
All methods are implicitly
public
andabstract
(unless they aredefault
orstatic
).A class can implement multiple interfaces (multiple inheritance is supported).
Used for defining a contract that multiple classes can implement.
Example:
class Dog implements Animal {
public void makeSound() {
System.out.println("Bark!");
}
}
public class Main {
public static void main(String[] args) {
Animal dog = new Dog();
dog.makeSound();
dog.sleep(); // Default method from interface
Animal.info(); // Static method from interface
}
}
What is an Abstract Class?
An abstract class is a class that cannot be instantiated and may contain both abstract methods (without implementation) and concrete methods (with implementation). It is used to provide a common base for subclasses.
abstract class Vehicle {
int speed;
Vehicle(int speed) { // Constructor
this.speed = speed;
}
abstract void move(); // Abstract method
void stop() {
System.out.println("Vehicle stopped");
}
}
Characteristics:
Can have instance variables.
Can have constructors.
Can have both abstract and concrete methods.
Can extend only one abstract class (single inheritance).
Used for creating a base class with common functionality.
Example:
class Car extends Vehicle {
Car(int speed) {
super(speed);
}
void move() {
System.out.println("Car is moving at speed: " + speed);
}
}
public class Main {
public static void main(String[] args) {
Vehicle car = new Car(100);
car.move();
car.stop();
}
}
Key Differences Between Interface and Abstract Class
Feature | Interface | Abstract Class |
Methods | Only abstract (except default & static) | Both abstract and concrete |
Fields | Only public static final constants | Can have instance variables |
Constructors | Not allowed | Allowed |
Inheritance | Multiple inheritance supported | Only single inheritance |
Access Modifiers | Methods are implicitly public | Can have any access modifier |
Use Case | Define a contract | Provide base functionality |
When to Use Interfaces vs Abstract Classes?
Use an Interface When:
You need to define a contract for multiple classes to implement.
You require multiple inheritance.
You do not need instance variables or constructors.
The behavior should be defined but not the implementation.
Use an Abstract Class When:
You want to share code among multiple related classes.
You need constructors and instance variables.
You expect subclasses to have a base implementation.
You do not need multiple inheritance.
Combining Abstract Classes and Interfaces
In real-world applications, abstract classes and interfaces can be used together to leverage their advantages.
interface Flyable {
void fly();
}
abstract class Bird {
String name;
Bird(String name) {
this.name = name;
}
abstract void makeSound();
}
class Sparrow extends Bird implements Flyable {
Sparrow(String name) {
super(name);
}
void makeSound() {
System.out.println(name + " chirps");
}
public void fly() {
System.out.println(name + " is flying");
}
}
public class Main {
public static void main(String[] args) {
Sparrow sparrow = new Sparrow("Sparrow");
sparrow.makeSound();
sparrow.fly();
}
}
Conclusion
Interfaces and abstract classes both enable abstraction but serve different purposes. Interfaces define a contract, allowing multiple inheritance, while abstract classes provide a base for related classes with common behavior. Understanding their differences helps in designing flexible and maintainable object-oriented applications.
Use interfaces when defining capabilities that can be shared across unrelated classes, and use abstract classes when creating a shared base for related classes.
Subscribe to my newsletter
Read articles from Ali Rıza Şahin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ali Rıza Şahin
Ali Rıza Şahin
Product-oriented Software Engineer with a solid understanding of web programming fundamentals and software development methodologies such as agile and scrum.