Understanding REST API, RPC, and gRPC: Choosing the Right Communication Method
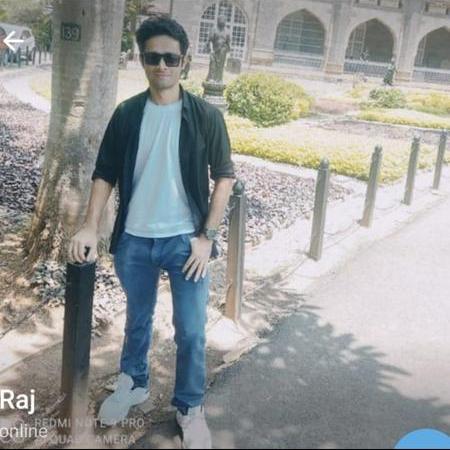

In modern software development, applications need to communicate with each other efficiently. Whether it's a mobile app talking to a backend server, microservices exchanging data, or distributed systems working together, the method of communication plays a crucial role.
REST API and gRPC are two widely used communication methods, but they are fundamentally different in how they operate. Let’s break them down.
REST API: An Architectural Style, Not an Implementation
Many people think of REST (Representational State Transfer) as a technology, but it's actually just an architectural style—a set of guidelines for designing networked applications. It defines principles such as:
Client-Server Separation: The client (frontend) and server (backend) operate independently.
Statelessness: Each request contains all the necessary information; the server does not remember previous requests.
Resource-Based Design: Data is represented as resources, each identified by a unique URL (e.g.,
/users/1
).Standard Methods: Uses HTTP methods like
GET
,POST
,PUT
, andDELETE
to interact with resources.Request:
GET /users/1 HTTP/1.1
Host: example.com
Content-Type: application/json
Response:
{
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com"
}
Limitations of REST
While REST APIs are widely used and easy to understand, they come with some downsides:
Overhead: Each request carries redundant headers and uses text-based JSON, which is not the most efficient format.
Latency: Since REST relies on multiple HTTP requests for different resources, it can be slow in large applications.
Lack of Strong Typing: REST doesn’t enforce strict data structures, leading to potential inconsistencies.
This is where RPC (Remote Procedure Call) and its modern version, gRPC, come into play.
RPC: The Concept Behind gRPC
RPC (Remote Procedure Call) is a communication model where one program can directly call a function on another computer as if it were a local function.
Think of it like calling a friend on the phone and asking them to perform a task for you. Instead of you doing it yourself, they execute the task and tell you the result.
In technical terms, RPC allows a client to send a request to a remote server, which executes the requested function and returns the result.
Example of a Basic RPC Call:
Imagine a function called getUser(1)
that fetches user data from a remote server. Instead of making an HTTP request with GET /users/1
, the client just calls:
user = getUser(1) # Feels like a local function call but runs on another server
This simplicity and efficiency are what make RPC powerful. Now, let’s see how gRPC builds on this concept.
gRPC: A Modern, High-Performance RPC Framework
gRPC (Google Remote Procedure Call) is an advanced communication framework designed to overcome the inefficiencies of REST APIs. It is based on the Remote Procedure Call (RPC) model but enhances it with modern technologies to provide faster, more efficient, and more scalable service-to-service communication.
Key Features of gRPC
Binary Data Transmission
Unlike REST, which uses JSON (a text-based format), gRPC uses Protocol Buffers (ProtoBuf)—a compact and efficient binary format. This significantly reduces the size of transmitted data, leading to faster and more efficient communication.Strongly Typed API Contracts
With REST, the data format is flexible but lacks strict structure enforcement. gRPC, on the other hand, defines strict contracts (schemas) using ProtoBuf, ensuring that both client and server follow the same structure. This reduces errors and enhances reliability.Built-in Streaming Capabilities
REST APIs typically follow a request-response model, where the client makes a request and waits for the server's response. gRPC introduces streaming, allowing continuous data flow between client and server. This is useful in scenarios like real-time data updates, video streaming, or chat applications.Multiplexing with HTTP/2
While REST APIs typically use HTTP/1.1, gRPC is built on HTTP/2, which offers multiplexing—allowing multiple requests and responses to be sent over a single connection. This improves efficiency and reduces network latency.Multi-Language Support
gRPC is designed to work across different programming languages. With REST, developers manually write client-side code to interact with APIs. In gRPC, the schema automatically generates client and server code for multiple languages like Python, Java, C++, Go, and more. This simplifies development in polyglot (multi-language) environments.Ideal for Microservices Architecture
Microservices need to communicate frequently, and using REST APIs for this can lead to inefficiencies. gRPC is optimized for fast, lightweight, and reliable microservices communication, making it a preferred choice for large-scale, distributed systems.
Conclusion
While REST remains popular due to its simplicity and ease of use, gRPC is a more powerful and efficient alternative for applications requiring high-speed, scalable, and structured communication.
If your project involves real-time interactions, microservices, or performance-sensitive applications, gRPC is a better choice than REST!
Subscribe to my newsletter
Read articles from Raj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
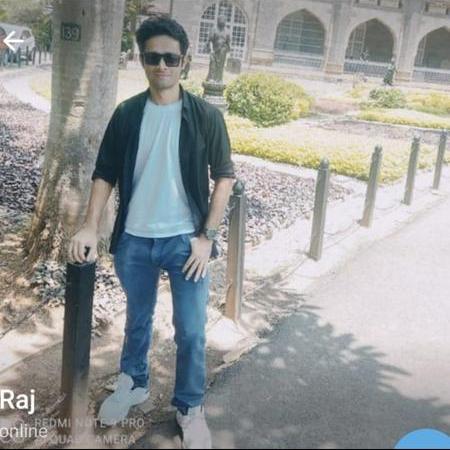