Day 13: JavaScript Debugging - Finding & Fixing Bugs 🐞🔍

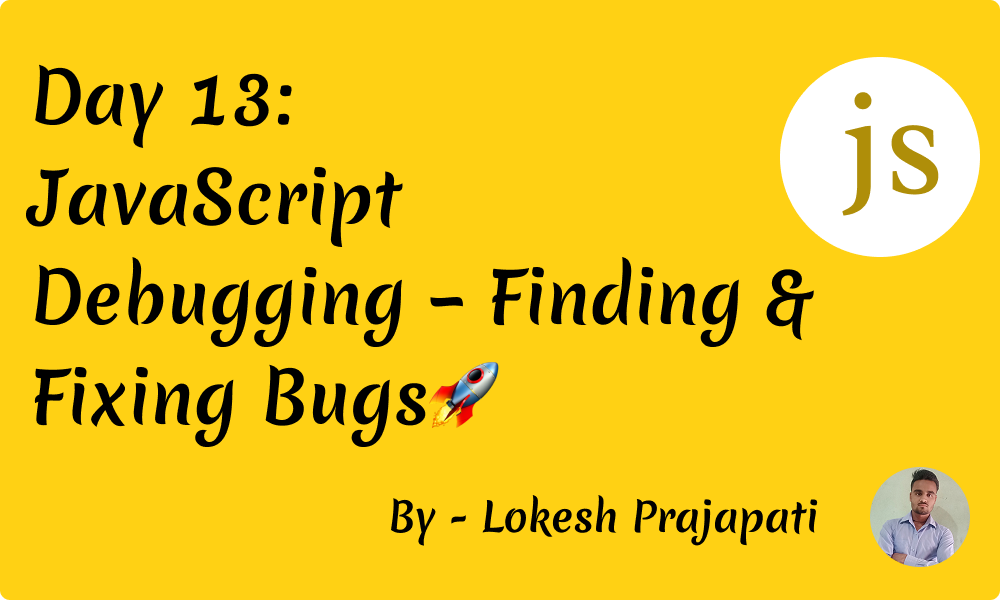
Debugging is an essential skill for every JavaScript developer. Even experienced programmers encounter bugs, and knowing how to effectively find and fix them can save you hours of frustration. In this post, we’ll cover practical debugging techniques, common JavaScript errors, and real-world examples to help you become a better developer. 🚀
1. Understanding Bugs in JavaScript 🤔
Bugs in JavaScript can occur due to various reasons, including syntax errors, logical mistakes, incorrect API usage, or unhandled asynchronous operations. Some common types of bugs are:
❌ Syntax Errors: Typos, missing brackets, or incorrect syntax.
🚨 Reference Errors: Accessing variables that don’t exist.
⚠️ Type Errors: Performing operations on incompatible data types.
🔄 Logic Errors: The program runs but doesn’t produce the expected output.
2. Essential Debugging Tools 🛠️
1️⃣ Using console.log()
(Quick & Easy Debugging)
The simplest and most common debugging technique is using console.log()
to print variable values and execution flow.
Example:
function addNumbers(a, b) {
console.log("a:", a, "b:", b); // Debugging output
return a + b;
}
console.log(addNumbers(5, "10")); // Expected 15, but got '510'
👉 Fix: Ensure both a
and b
are numbers before adding.
function addNumbers(a, b) {
return Number(a) + Number(b);
}
2️⃣ Using Browser Developer Tools 🖥️
Modern browsers like Chrome and Firefox provide built-in Developer Tools (DevTools). You can open DevTools by pressing:
Windows/Linux:
F12
orCtrl + Shift + I
Mac:
Cmd + Option + I
Steps to Use DevTools for Debugging:
Open DevTools and go to the “Console” tab to check for errors.
Use the “Sources” tab to inspect and set breakpoints in your JavaScript code.
Monitor network requests in the “Network” tab.
3. Setting Breakpoints for Better Debugging 🎯
Breakpoints allow you to pause code execution at specific lines and inspect variable values in real time.
Example:
function multiply(x, y) {
debugger; // Execution will pause here in DevTools
return x * y;
}
console.log(multiply(3, 4));
👉 Open DevTools → Go to “Sources” → Run the function → See values at the debugger
statement.
4. Common JavaScript Bugs & How to Fix Them 🛑
1️⃣ Uncaught ReferenceError
console.log(myVar); // ReferenceError: myVar is not defined
Fix: Always define variables before using them.
let myVar = 10;
console.log(myVar);
2️⃣ Uncaught TypeError
let str = "hello";
console.log(str.toUpper()); // TypeError: str.toUpper is not a function
Fix: Use the correct method name (toUpperCase()
).
console.log(str.toUpperCase()); // HELLO
3️⃣ Logical Errors (Wrong Output) 🧠
function isEven(num) {
return num % 2 === 1; // Wrong condition ❌
}
console.log(isEven(4)); // Expected false, but got true
Fix: The correct condition should be num % 2 === 0
.
function isEven(num) {
return num % 2 === 0;
}
5. Debugging Asynchronous Code (Promises & Async/Await) ⏳
When dealing with asynchronous operations like API calls, debugging can be tricky.
Common Issue: Forgetting .catch()
in Promises
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data));
// No error handling if the request fails ❌
Fix: Always use .catch()
to handle errors.
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error("Fetch error:", error));
Using Try-Catch with Async/Await ⚡
async function fetchData() {
try {
let response = await fetch('https://api.example.com/data');
let data = await response.json();
console.log(data);
} catch (error) {
console.error("Error fetching data:", error);
}
}
fetchData();
6. Best Practices for Debugging JavaScript ✅
✅ Use console.log()
for quick debugging, but remove it before production. ✅ Use debugger
and DevTools for in-depth analysis. ✅ Handle errors properly using try-catch
and .catch()
in promises. ✅ Write unit tests to catch errors before deployment. ✅ Follow coding standards and best practices to reduce the chances of bugs. ✅ Read error messages carefully—they often tell you exactly what’s wrong! 🧐
Conclusion 🎉
Debugging is an essential part of JavaScript development. By using tools like console.log()
, breakpoints, and DevTools, and following best practices, you can efficiently find and fix bugs in your code. Keep practicing these debugging techniques, and over time, you'll become more confident in handling JavaScript errors effectively. 🚀
💡 Have a debugging tip or trick? Share it in the comments! 💬
Subscribe to my newsletter
Read articles from Lokesh Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lokesh Prajapati
Lokesh Prajapati
🚀 JavaScript | React | Shopify Developer | Tech Blogger Hi, I’m Lokesh Prajapati, a passionate web developer and content creator. I love simplifying JavaScript, React, and Shopify development through easy-to-understand tutorials and real-world examples. I’m currently running a JavaScript Basics to Advanced series on Medium & Hashnode, helping developers of all levels enhance their coding skills. My goal is to make programming more accessible and practical for everyone. Follow me for daily coding tips, tricks, and insights! Let’s learn and grow together. 💡🚀 #JavaScript #React #Shopify #WebDevelopment #Coding #TechBlogger #LearnToCode