MongoDB Indexes: A Comprehensive Guide
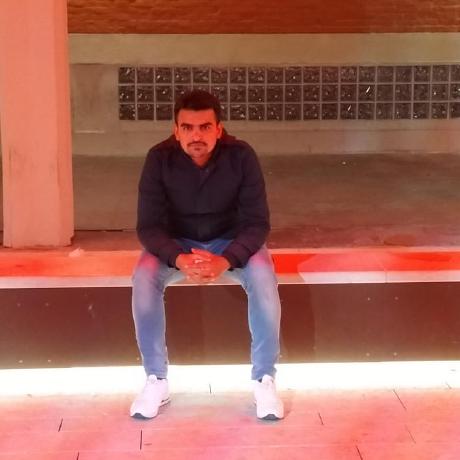

Indexes are a fundamental aspect of database optimization, enabling efficient querying and improving the overall performance of MongoDB applications. This article delves into what MongoDB indexes are, how they work, best practices, and implementation examples. It also references official MongoDB documentation for further reading.
What is an Index in MongoDB?
An index in MongoDB is a data structure that stores a subset of data from a collection in a way that facilitates efficient retrieval. Much like an index in a book, MongoDB indexes allow queries to locate data without scanning the entire collection, significantly speeding up read operations.
MongoDB creates a default index on the _id
field of every collection to ensure uniqueness and provide fast access to documents by their primary key. However, developers often need additional indexes tailored to their specific query patterns.
How Indexes Work
When you query a MongoDB collection, the database engine must scan the documents to find matches. Without an index, MongoDB performs a collection scan, checking every document. While this works for small datasets, it becomes inefficient as the data grows. Indexes allow MongoDB to quickly locate matching documents, reducing the need for full collection scans.
Types of Indexes in MongoDB
Single Field Index
Indexes a single field in ascending or descending order.
Example:
{ field1: 1 }
or{ field1: -1 }
.
Compound Index
Combines multiple fields into a single index.
Useful for queries involving multiple fields.
Example:
{ field1: 1, field2: -1 }
.
Multikey Index
Created on arrays, allowing MongoDB to index each array element.
Example:
{ arrayField: 1 }
.
Text Index
Enables text search capabilities.
Example:
{ field: "text" }
.
Hashed Index
Supports hashed sharding by indexing hashed values.
Example:
{ field: "hashed" }
.
Wildcard Index
Indexes all fields or a subset of fields dynamically.
Example:
{ "$**": 1 }
.
Creating Indexes in MongoDB
Using the MongoDB Shell
Indexes can be created using the createIndex()
method.
// Single field index
db.collection.createIndex({ field1: 1 });
// Compound index
db.collection.createIndex({ field1: 1, field2: -1 });
// Text index
db.collection.createIndex({ description: "text" });
// Hashed index
db.collection.createIndex({ userId: "hashed" });
Using MongoDB Compass
Navigate to the desired collection.
Go to the "Indexes" tab.
Click "Create Index" and configure the index options.
Querying with Indexes
Indexes automatically enhance the performance of queries matching indexed fields. MongoDB uses the query planner to decide whether to utilize an index based on the query.
db.collection.find({ field1: "value" });
To confirm index usage, leverage the explain()
method:
db.collection.find({ field1: "value" }).explain("executionStats");
Best Practices for MongoDB Indexes
Understand Query Patterns
- Analyze frequent queries and create indexes that align with them.
Use Compound Indexes Wisely
- Place the most selective fields first in the index definition.
Avoid Over-Indexing
- Indexes consume storage and slow down write operations.
Monitor Index Usage
- Use the
getIndexes()
method and the MongoDB Atlas Performance Advisor.
- Use the
Optimize Multikey Indexes
- Avoid creating multikey indexes on large arrays to prevent performance degradation.
Regularly Evaluate Indexes
- Remove unused indexes to optimize storage and performance.
Leverage TTL Indexes
- Use time-to-live (TTL) indexes for expiring documents.
db.collection.createIndex({ createdAt: 1 }, { expireAfterSeconds: 3600 });
Challenges and Considerations
Index Cardinality
- Low cardinality fields (e.g., boolean values) are less effective for indexing.
Write Overhead
- Each index adds overhead to write operations.
Storage Impact
- Indexes consume additional disk space.
Further Reading
Conclusion
Indexes are a powerful tool for optimizing MongoDB queries, but they must be used judiciously. By understanding query patterns, selecting appropriate index types, and following best practices, you can ensure your database remains performant and efficient. For advanced scenarios, consult the official MongoDB documentation and tools like the Performance Advisor in MongoDB Atlas.
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
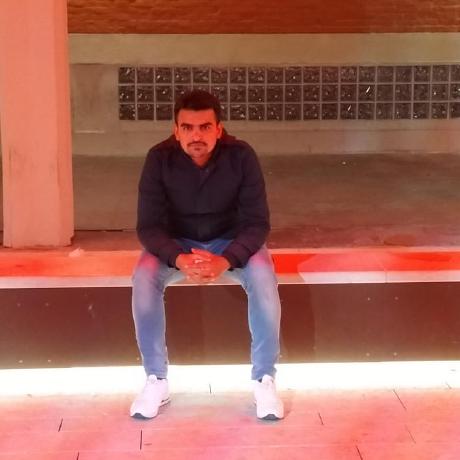
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.