The Comprehensive Guide to Software & Web Development: From Conception to Deployment
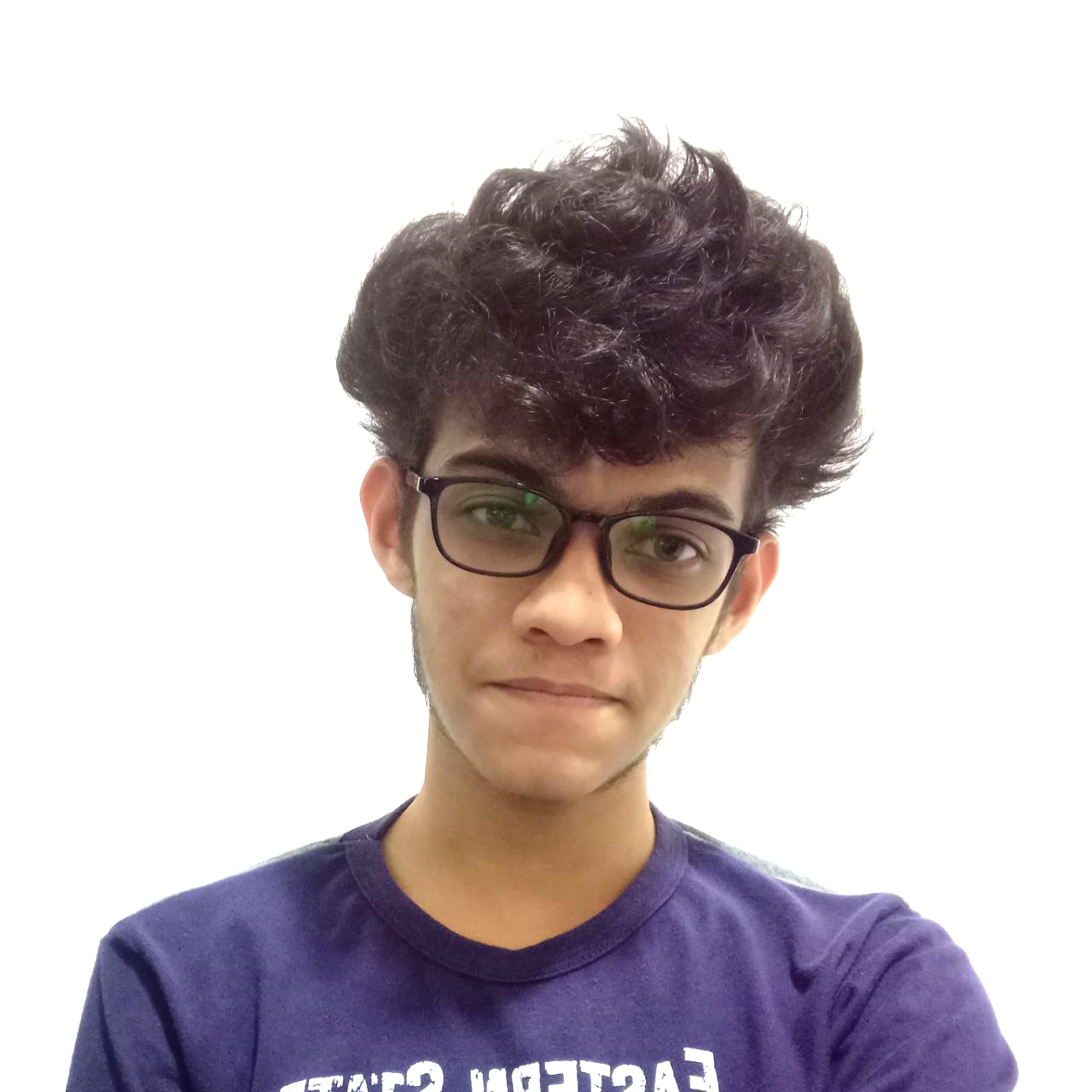
Table of contents
- Introduction: Understanding the Software Development Ecosystem
- Part 1: The Software Development Lifecycle (SDLC)
- Part 2: The Technology Stack: Building Blocks of Modern Software
- Part 3: Advanced Development Concepts
- Part 4: Emerging Trends and Technologies
- Part 5: Best Practices and Career Development
- Conclusion: The Journey of Software Development
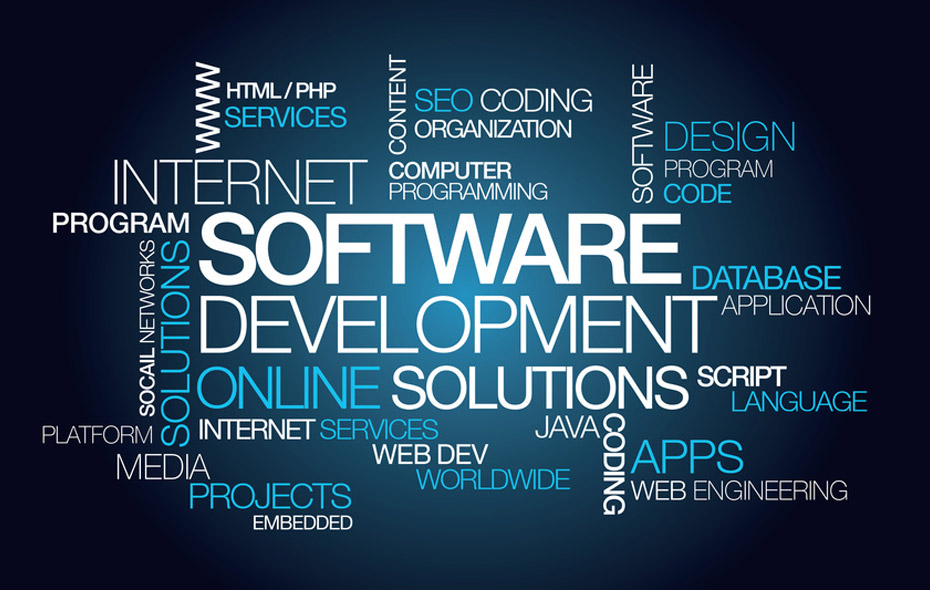
Introduction: Understanding the Software Development Ecosystem
Software development is much like constructing a complex building. It requires careful planning, solid foundations, skilled craftspeople, and ongoing maintenance. Whether you're building a simple website or an enterprise-level application, understanding how all the pieces fit together is essential before diving into specific languages or frameworks.
This guide will walk you through the entire software development lifecycle, from initial concept to deployment and beyond, giving you a comprehensive understanding of how modern software is built, deployed, and maintained.
Note to Readers: I've included code examples throughout most sections to illustrate key concepts. If you're completely new to programming and aren't familiar with coding syntax yet, feel free to skip these technical portions. you can still gain valuable insights from the conceptual overviews alone.
For topics you'd like to explore further, I encourage you to use online resources like Google or AI assistants for additional explanations. Knowing how to independently research solutions is actually a crucial skill in software development! This approach of learning, researching, and problem-solving mirrors the real-world development process that professionals use daily.
Part 1: The Software Development Lifecycle (SDLC)
Before examining specific technologies, it's important to understand the overall process of creating software:
1.1 Planning & Requirements Gathering
Every software project begins with identifying a problem and planning a solution. This phase includes:
Market Research: Understanding user needs and pain points
Stakeholder Interviews: Gathering requirements from clients or end-users
Feasibility Studies: Determining if the project is technically and financially viable
Project Scope Definition: Setting clear boundaries for what the software will and won't do
Real-world example: A healthcare provider wants to streamline appointment scheduling. During the planning phase, the development team interviews doctors, administrative staff, and patients to understand pain points in the current system and define requirements for the new solution.
1.2 Design Phase
Once requirements are clear, architects and designers create blueprints for the software:
System Architecture: Deciding the overall structure and technologies
Database Design: Planning how data will be organized and stored
UI/UX Design: Creating wireframes and prototypes of user interfaces
Security Planning: Identifying potential threats and designing safeguards
Real-world example: For the healthcare scheduling system, architects decide to build a cloud-based solution with a web frontend and mobile app. UI designers create wireframes showing appointment calendars, reminder systems, and patient profiles.
1.3 Development Phase
This is where actual coding begins:
Setting Up Development Environment: Configuring tools and version control
Frontend Development: Building user interfaces and interactive elements
Backend Development: Creating server-side logic and database interactions
Testing Environment: Establishing systems to verify code quality
Real-world example: Developers write code for the appointment system, creating interfaces for patients to book appointments and for staff to manage schedules. They build APIs to connect these interfaces with the database and external systems like SMS notification services.
1.4 Testing Phase
Testing ensures the software works correctly and meets requirements:
Unit Testing: Testing individual components in isolation
Integration Testing: Verifying that components work together
User Acceptance Testing (UAT): Having end-users validate the software
Performance Testing: Checking system behavior under various conditions
Real-world example: QA testers verify that appointments can be booked, modified, and canceled properly. They test how the system handles conflicts, how it performs under load (simulating many users booking at once), and validate that reminder notifications are sent correctly.
1.5 Deployment Phase
Once testing is complete, the software is released to users:
Production Environment Setup: Configuring servers and databases
Data Migration: Moving existing data to the new system
Deployment Strategies: Choosing between big-bang or phased rollouts
Training: Preparing users for the new system
Real-world example: The healthcare provider decides on a phased rollout, first implementing the system in a single department before expanding to others. IT staff configure production servers, migrate existing appointment data, and train staff on using the new system.
1.6 Maintenance Phase
Software development doesn't end at deployment:
Bug Fixes: Addressing issues reported by users
Feature Updates: Adding new capabilities based on feedback
Performance Optimization: Improving speed and efficiency
Security Patches: Addressing vulnerabilities
Real-world example: After deployment, the team fixes a bug where appointment reminders were sending at incorrect times. They also add a new feature allowing patients to join virtual appointments directly from the app, based on user feedback.
Part 2: The Technology Stack: Building Blocks of Modern Software
Now that we understand the overall process, let's examine the specific technologies that power software applications.
2.1 Frontend Development: What Users See and Touch
Frontend development creates the interface users interact with. It encompasses:
2.1.1 Core Technologies
- HTML (HyperText Markup Language): The skeleton that structures web content
<section class="appointment-card">
<h2>Your Upcoming Appointment</h2>
<div class="appointment-details">
<p>Dr. Sarah Johnson</p>
<p>March 15, 2025 - 10:30 AM</p>
<p>General Checkup</p>
</div>
<button class="reschedule-btn">Reschedule</button>
<button class="cancel-btn">Cancel</button>
</section>
- CSS (Cascading Style Sheets): The skin that styles and positions elements
.appointment-card {
background-color: white;
border-radius: 8px;
box-shadow: 0 2px 8px rgba(0, 0, 0, 0.1);
padding: 24px;
margin-bottom: 16px;
}
.appointment-details {
margin: 16px 0;
color: #444;
}
.reschedule-btn {
background-color: #4a90e2;
color: white;
border: none;
padding: 8px 16px;
border-radius: 4px;
margin-right: 8px;
}
.cancel-btn {
background-color: white;
color: #e25c4a;
border: 1px solid #e25c4a;
padding: 8px 16px;
border-radius: 4px;
}
- JavaScript: The muscles that add interactivity and dynamic behavior
document.querySelector('.reschedule-btn').addEventListener('click', function() {
// Open reschedule modal
const modal = document.getElementById('reschedule-modal');
modal.style.display = 'block';
// Load available time slots from API
fetchAvailableTimeSlots(doctorId).then(slots => {
populateTimeSlotOptions(slots);
});
});
document.querySelector('.cancel-btn').addEventListener('click', function() {
if (confirm('Are you sure you want to cancel this appointment?')) {
cancelAppointment(appointmentId)
.then(response => {
showNotification('Appointment successfully canceled');
removeAppointmentFromUI(appointmentId);
})
.catch(error => {
showError('Failed to cancel appointment. Please try again.');
});
}
});
2.1.2 Frontend Frameworks and Libraries
Rather than building everything from scratch, developers use frameworks to accelerate development:
React: A JavaScript library for building user interfaces (maintained by Facebook)
Vue.js: A progressive framework for building UIs
Angular: A platform and framework for building single-page applications
Svelte: A compiler that converts your components into highly efficient JavaScript
Example of a React component for an appointment card:
function AppointmentCard({ appointment, onReschedule, onCancel }) {
const { doctor, date, time, type } = appointment;
const handleCancelClick = () => {
if (window.confirm('Are you sure you want to cancel this appointment?')) {
onCancel(appointment.id);
}
};
return (
<div className="appointment-card">
<h2>Your Upcoming Appointment</h2>
<div className="appointment-details">
<p>{doctor.name}</p>
<p>{formatDate(date)} - {time}</p>
<p>{type}</p>
</div>
<button
className="reschedule-btn"
onClick={() => onReschedule(appointment.id)}
>
Reschedule
</button>
<button
className="cancel-btn"
onClick={handleCancelClick}
>
Cancel
</button>
</div>
);
}
2.1.3 Mobile App Development
For mobile applications, there are several approaches:
Native Development:
iOS: Swift or Objective-C
Android: Kotlin or Java
Cross-Platform Development:
React Native: Using React to build native mobile apps
Flutter: Google's UI toolkit for building natively compiled applications
Xamarin: Microsoft's platform for building Android and iOS apps with .NET
Example of a React Native component:
import React from 'react';
import { View, Text, StyleSheet, TouchableOpacity, Alert } from 'react-native';
const AppointmentCard = ({ appointment, onReschedule, onCancel }) => {
const confirmCancel = () => {
Alert.alert(
"Cancel Appointment",
"Are you sure you want to cancel this appointment?",
[
{ text: "No", style: "cancel" },
{ text: "Yes", onPress: () => onCancel(appointment.id) }
]
);
};
return (
<View style={styles.card}>
<Text style={styles.title}>Your Upcoming Appointment</Text>
<View style={styles.details}>
<Text style={styles.text}>{appointment.doctor}</Text>
<Text style={styles.text}>{appointment.date} - {appointment.time}</Text>
<Text style={styles.text}>{appointment.type}</Text>
</View>
<View style={styles.buttonContainer}>
<TouchableOpacity
style={styles.rescheduleButton}
onPress={() => onReschedule(appointment.id)}
>
<Text style={styles.rescheduleText}>Reschedule</Text>
</TouchableOpacity>
<TouchableOpacity
style={styles.cancelButton}
onPress={confirmCancel}
>
<Text style={styles.cancelText}>Cancel</Text>
</TouchableOpacity>
</View>
</View>
);
};
const styles = StyleSheet.create({
card: {
backgroundColor: 'white',
borderRadius: 8,
padding: 24,
marginBottom: 16,
shadowColor: '#000',
shadowOffset: { width: 0, height: 2 },
shadowOpacity: 0.1,
shadowRadius: 4,
elevation: 2,
},
// Additional styles...
});
export default AppointmentCard;
2.2 Backend Development: The Engine Behind the Scenes
While frontend focuses on user interaction, backend handles business logic, data storage, and processing.
2.2.1 Server-Side Languages and Frameworks
Node.js (JavaScript):
Express.js: Minimalist web framework
NestJS: Progressive framework for building efficient server-side applications
// Express.js example for appointment API endpoints
const express = require('express');
const router = express.Router();
const AppointmentController = require('../controllers/appointment.controller');
const authMiddleware = require('../middleware/auth');
// Get all appointments for a patient
router.get('/patients/:patientId/appointments',
authMiddleware.verifyToken,
AppointmentController.getPatientAppointments
);
// Book a new appointment
router.post('/appointments',
authMiddleware.verifyToken,
AppointmentController.createAppointment
);
// Cancel an appointment
router.delete('/appointments/:id',
authMiddleware.verifyToken,
AppointmentController.cancelAppointment
);
module.exports = router;
Python:
Django: Full-featured framework with "batteries included"
Flask: Lightweight, flexible framework
# Flask example for appointment routes
from flask import Blueprint, request, jsonify
from flask_jwt_extended import jwt_required, get_jwt_identity
from app.services.appointment_service import AppointmentService
from app.schemas.appointment_schema import AppointmentSchema
appointment_bp = Blueprint('appointments', __name__)
appointment_service = AppointmentService()
@appointment_bp.route('/appointments', methods=['POST'])
@jwt_required()
def create_appointment():
current_user = get_jwt_identity()
data = request.get_json()
result = appointment_service.create_appointment(
patient_id=current_user['id'],
doctor_id=data['doctor_id'],
date=data['date'],
time=data['time'],
appointment_type=data['type']
)
return jsonify(AppointmentSchema().dump(result)), 201
@appointment_bp.route('/appointments/<int:appointment_id>', methods=['DELETE'])
@jwt_required()
def cancel_appointment(appointment_id):
current_user = get_jwt_identity()
appointment_service.cancel_appointment(
appointment_id=appointment_id,
user_id=current_user['id']
)
return '', 204
Java:
Spring Boot: Framework for creating stand-alone, production-grade applications
Jakarta EE: Enterprise platform for Java applications
Ruby:
Ruby on Rails: Convention over configuration framework
Sinatra: Lightweight alternative to Rails
PHP:
Laravel: Elegant framework with expressive syntax
Symfony: Reusable PHP components and framework
C#:
- ASP.NET Core: Cross-platform, high-performance framework
2.2.2 Databases: Storing and Managing Data
SQL (Relational) Databases:
MySQL: Open-source relational database
PostgreSQL: Advanced open-source relational database
SQL Server: Microsoft's relational database solution
Oracle: Enterprise database system
Example of SQL schema for our healthcare system:
CREATE TABLE patients (
id SERIAL PRIMARY KEY,
first_name VARCHAR(100) NOT NULL,
last_name VARCHAR(100) NOT NULL,
email VARCHAR(255) UNIQUE NOT NULL,
phone VARCHAR(20),
date_of_birth DATE,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
CREATE TABLE doctors (
id SERIAL PRIMARY KEY,
first_name VARCHAR(100) NOT NULL,
last_name VARCHAR(100) NOT NULL,
specialty VARCHAR(100) NOT NULL,
email VARCHAR(255) UNIQUE NOT NULL,
phone VARCHAR(20),
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
CREATE TABLE appointments (
id SERIAL PRIMARY KEY,
patient_id INTEGER REFERENCES patients(id),
doctor_id INTEGER REFERENCES doctors(id),
appointment_date DATE NOT NULL,
appointment_time TIME NOT NULL,
appointment_type VARCHAR(100) NOT NULL,
status VARCHAR(20) DEFAULT 'scheduled',
notes TEXT,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
UNIQUE(doctor_id, appointment_date, appointment_time)
);
CREATE INDEX idx_appointments_patient_id ON appointments(patient_id);
CREATE INDEX idx_appointments_doctor_id ON appointments(doctor_id);
CREATE INDEX idx_appointments_date ON appointments(appointment_date);
NoSQL Databases:
MongoDB: Document-oriented database
Redis: In-memory data structure store
Cassandra: Wide-column store designed for scalability
Firebase Firestore: Cloud-based NoSQL database
Example of MongoDB schema for our healthcare system:
// Patient collection
{
"_id": ObjectId("6054b1a2e87dcf001f9e4c2a"),
"firstName": "John",
"lastName": "Smith",
"email": "john.smith@example.com",
"phone": "+1-555-123-4567",
"dateOfBirth": ISODate("1985-06-15"),
"medicalHistory": [
{
"condition": "Asthma",
"diagnosedDate": ISODate("2010-03-12")
}
],
"createdAt": ISODate("2024-03-09T14:22:10.123Z")
}
// Doctor collection
{
"_id": ObjectId("6054b8a7e87dcf001f9e4c2b"),
"firstName": "Sarah",
"lastName": "Johnson",
"specialty": "Cardiology",
"email": "sarah.johnson@example.com",
"phone": "+1-555-987-6543",
"availability": [
{
"day": "Monday",
"slots": ["9:00", "9:30", "10:00", "10:30", "11:00"]
},
{
"day": "Wednesday",
"slots": ["13:00", "13:30", "14:00", "14:30", "15:00"]
}
],
"createdAt": ISODate("2024-03-09T14:50:15.456Z")
}
// Appointment collection
{
"_id": ObjectId("6054c1d9e87dcf001f9e4c2c"),
"patientId": ObjectId("6054b1a2e87dcf001f9e4c2a"),
"doctorId": ObjectId("6054b8a7e87dcf001f9e4c2b"),
"date": ISODate("2025-03-15T00:00:00.000Z"),
"time": "10:30",
"type": "General Checkup",
"status": "scheduled",
"notes": "Follow-up on recent lab results",
"createdAt": ISODate("2024-03-09T15:30:05.789Z")
}
2.2.3 APIs: The Communication Bridge
APIs (Application Programming Interfaces) connect frontend and backend, allowing them to exchange data.
REST (Representational State Transfer):
Architectural style using HTTP methods (GET, POST, PUT, DELETE)
Resources identified by URLs
Example REST API responses:
// GET /api/patients/1234/appointments
{
"appointments": [
{
"id": "6054c1d9e87dcf001f9e4c2c",
"doctor": {
"id": "6054b8a7e87dcf001f9e4c2b",
"name": "Dr. Sarah Johnson",
"specialty": "Cardiology"
},
"date": "2025-03-15",
"time": "10:30",
"type": "General Checkup",
"status": "scheduled"
}
],
"total": 1,
"page": 1,
"perPage": 10
}
GraphQL:
Query language for APIs
Clients specify exactly what data they need
Example GraphQL query and response:
# Query
query {
patient(id: "1234") {
firstName
lastName
appointments {
id
doctor {
name
specialty
}
date
time
type
}
}
}
# Response
{
"data": {
"patient": {
"firstName": "John",
"lastName": "Smith",
"appointments": [
{
"id": "6054c1d9e87dcf001f9e4c2c",
"doctor": {
"name": "Sarah Johnson",
"specialty": "Cardiology"
},
"date": "2025-03-15",
"time": "10:30",
"type": "General Checkup"
}
]
}
}
}
WebSockets:
Persistent connections for real-time communication
Used for chat applications, live notifications, etc.
Example WebSocket implementation for appointment notifications:
// Server-side (Node.js with Socket.io)
const io = require('socket.io')(server);
io.on('connection', socket => {
// Authenticate user
socket.on('authenticate', async (token) => {
const user = await verifyToken(token);
if (user) {
// Associate socket with user ID
socket.join(`user-${user.id}`);
console.log(`User ${user.id} connected`);
}
});
});
// When an appointment status changes
function notifyAppointmentChange(appointment) {
const { patientId, doctorId, status } = appointment;
// Notify patient
io.to(`user-${patientId}`).emit('appointment:updated', {
appointmentId: appointment.id,
status: status,
message: `Your appointment has been ${status}`
});
// Notify doctor
io.to(`user-${doctorId}`).emit('appointment:updated', {
appointmentId: appointment.id,
patientName: `${appointment.patient.firstName} ${appointment.patient.lastName}`,
status: status
});
}
// Client-side (JavaScript)
const socket = io.connect('https://api.healthcare-app.com');
// Authenticate when connected
socket.on('connect', () => {
const token = localStorage.getItem('authToken');
socket.emit('authenticate', token);
});
// Listen for appointment updates
socket.on('appointment:updated', (data) => {
console.log(`Appointment update: ${data.message}`);
// Update UI with new appointment status
updateAppointmentInUI(data.appointmentId, data.status);
// Show notification to user
showNotification(data.message);
});
2.3 DevOps: Building the Bridge Between Development and Operations
DevOps practices streamline the development-to-deployment pipeline.
2.3.1 Version Control Systems
Git: Distributed version control system
GitHub/GitLab/Bitbucket: Platforms for hosting Git repositories and collaboration
Example Git workflow:
# Create a new feature branch
git checkout -b feature/appointment-reminders
# Make changes to code and commit them
git add src/services/notification-service.js
git commit -m "Add SMS reminders for upcoming appointments"
# Push changes to remote repository
git push origin feature/appointment-reminders
# After code review and approval, merge to main branch
git checkout main
git pull
git merge feature/appointment-reminders
git push origin main
2.3.2 Continuous Integration/Continuous Deployment (CI/CD)
CI/CD automates testing and deployment, ensuring rapid, reliable releases.
Example GitHub Actions workflow for our healthcare app:
# .github/workflows/deploy.yml
name: Deploy Healthcare App
on:
push:
branches: [ main ]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Use Node.js
uses: actions/setup-node@v2
with:
node-version: '18'
- name: Install dependencies
run: npm ci
- name: Run tests
run: npm test
deploy:
needs: test
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Use Node.js
uses: actions/setup-node@v2
with:
node-version: '18'
- name: Install dependencies
run: npm ci
- name: Build
run: npm run build
- name: Deploy to AWS
uses: aws-actions/configure-aws-credentials@v1
with:
aws-access-key-id: ${{ secrets.AWS_ACCESS_KEY_ID }}
aws-secret-access-key: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
aws-region: us-east-1
- name: Deploy to S3 and invalidate CloudFront
run: |
aws s3 sync ./build s3://healthcare-app-production --delete
aws cloudfront create-invalidation --distribution-id ${{ secrets.CLOUDFRONT_DISTRIBUTION_ID }} --paths "/*"
2.3.3 Containerization and Orchestration
Docker: Platform for developing, shipping, and running applications in containers
Kubernetes: Container orchestration system for automating deployment and scaling
Example Docker configuration for our healthcare app backend:
# Dockerfile
FROM node:18-alpine
WORKDIR /app
# Copy package.json and install dependencies
COPY package*.json ./
RUN npm ci --only=production
# Copy application code
COPY . .
# Set environment variables
ENV NODE_ENV=production
ENV PORT=3000
# Expose the port the app runs on
EXPOSE 3000
# Start the application
CMD ["node", "src/index.js"]
Kubernetes deployment configuration:
# kubernetes/deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: healthcare-api
spec:
replicas: 3
selector:
matchLabels:
app: healthcare-api
template:
metadata:
labels:
app: healthcare-api
spec:
containers:
- name: healthcare-api
image: healthcare-registry.azurecr.io/healthcare-api:latest
ports:
- containerPort: 3000
env:
- name: DATABASE_URL
valueFrom:
secretKeyRef:
name: healthcare-secrets
key: database-url
- name: JWT_SECRET
valueFrom:
secretKeyRef:
name: healthcare-secrets
key: jwt-secret
resources:
limits:
cpu: "500m"
memory: "512Mi"
requests:
cpu: "200m"
memory: "256Mi"
readinessProbe:
httpGet:
path: /health
port: 3000
initialDelaySeconds: 5
periodSeconds: 10
2.3.4 Cloud Services and Infrastructure as Code
Modern applications often leverage cloud services:
AWS (Amazon Web Services): Leading cloud provider with vast service offerings
Azure: Microsoft's cloud computing service
Google Cloud Platform (GCP): Google's suite of cloud services
Infrastructure as Code (IaC) tools automate cloud resource provisioning:
Terraform: Multi-cloud infrastructure provisioning tool
AWS CloudFormation: AWS-specific IaC service
Azure Resource Manager: Azure's deployment and management service
Example Terraform configuration for our healthcare application:
# main.tf
provider "aws" {
region = "us-east-1"
}
# VPC and networking
resource "aws_vpc" "healthcare_vpc" {
cidr_block = "10.0.0.0/16"
tags = {
Name = "healthcare-vpc"
Environment = "production"
}
}
# Database
resource "aws_db_instance" "healthcare_db" {
allocated_storage = 20
storage_type = "gp2"
engine = "postgres"
engine_version = "14.5"
instance_class = "db.t3.medium"
db_name = "healthcare"
username = var.db_username
password = var.db_password
parameter_group_name = "default.postgres14"
skip_final_snapshot = true
vpc_security_group_ids = [aws_security_group.db_sg.id]
db_subnet_group_name = aws_db_subnet_group.healthcare_db_subnet.name
tags = {
Name = "healthcare-db"
Environment = "production"
}
}
# ECS Cluster for API containers
resource "aws_ecs_cluster" "healthcare_api_cluster" {
name = "healthcare-api-cluster"
setting {
name = "containerInsights"
value = "enabled"
}
tags = {
Name = "healthcare-api-cluster"
Environment = "production"
}
}
# S3 bucket for frontend static assets
resource "aws_s3_bucket" "frontend_bucket" {
bucket = "healthcare-app-frontend"
tags = {
Name = "Healthcare Frontend"
Environment = "production"
}
}
# CloudFront distribution for frontend
resource "aws_cloudfront_distribution" "frontend_distribution" {
origin {
domain_name = aws_s3_bucket.frontend_bucket.bucket_regional_domain_name
origin_id = "S3Origin"
}
enabled = true
is_ipv6_enabled = true
default_root_object = "index.html"
# Additional CloudFront configuration...
tags = {
Name = "healthcare-frontend-distribution"
Environment = "production"
}
}
Part 3: Advanced Development Concepts
3.1 Authentication and Authorization
Securing applications is critical, especially for sensitive data like healthcare information.
3.1.1 Authentication Strategies
JWT (JSON Web Tokens): Compact, self-contained tokens for secure information transmission
OAuth 2.0: Industry-standard protocol for authorization
OpenID Connect: Identity layer on top of OAuth 2.0
Passwordless Authentication: Email links, SMS codes, or biometrics
Example JWT implementation:
// Authentication controller (Node.js)
const jwt = require('jsonwebtoken');
const bcrypt = require('bcrypt');
const User = require('../models/user.model');
exports.login = async (req, res) => {
try {
const { email, password } = req.body;
// Find user by email
const user = await User.findOne({ email });
if (!user) {
return res.status(401).json({ message: 'Invalid credentials' });
}
// Check password
const isPasswordValid = await bcrypt.compare(password, user.password);
if (!isPasswordValid) {
return res.status(401).json({ message: 'Invalid credentials' });
}
// Generate JWT token
const token = jwt.sign(
{ id: user._id, email: user.email, role: user.role },
process.env.JWT_SECRET,
{ expiresIn: '8h' }
);
// Return token and user info
res.status(200).json({
token,
user: {
id: user._id,
name: `${user.firstName} ${user.lastName}`,
email: user.email,
role: user.role
}
});
} catch (error) {
res.status(500).json({ message: 'Server error', error: error.message });
}
};
3.1.2 Authorization and Access Control
RBAC (Role-Based Access Control): Permissions based on user roles
ABAC (Attribute-Based Access Control): Permissions based on attributes of users, resources, and environment
Example RBAC middleware:
// Role-based authorization middleware
const authorize = (...allowedRoles) => {
return (req, res, next) => {
// User must be authenticated first
if (!req.user) {
return res.status(401).json({ message: 'Unauthorized' });
}
// Check if user's role is in the allowed roles
if (allowedRoles.length && !allowedRoles.includes(req.user.role)) {
return res.status(403).json({ message: 'Forbidden - Insufficient permissions' });
}
// User has required role, proceed to next middleware/controller
next();
};
};
// Usage in routes
router.get('/patients',
authMiddleware.verifyToken,
authorize('doctor', 'admin', 'nurse'),
PatientController.getAllPatients
);
router.post('/admin/users',
authMiddleware.verifyToken,
authorize('admin'),
UserController.createUser
);
3.2 Performance Optimization
High-performing applications provide better user experiences and lower operating costs.
3.2.1 Frontend Performance
Code Splitting: Loading code only when needed
// React code splitting example using dynamic imports import React, { lazy, Suspense } from 'react'; // Instead of regular import // import AppointmentCalendar from './components/AppointmentCalendar'; // Use lazy loading const AppointmentCalendar = lazy(() => import('./components/AppointmentCalendar')); function DoctorDashboard() { return ( <div> <h1>Doctor Dashboard</h1> <Suspense fallback={<div>Loading calendar...</div>}> <AppointmentCalendar /> </Suspense> </div> ); }
Tree Shaking: Eliminating unused code during bundling
Lazy Loading: Loading images and components only when visible
Minimizing Bundle Size: Optimizing dependencies and assets
Caching Strategies: Leveraging browser caching effectively
3.2.2 Backend Performance
Database Optimization:
Indexing for faster queries
Query optimization and execution planning
Connection pooling
Caching:
In-memory caching (Redis, Memcached)
Content Delivery Networks (CDNs)
Response caching
// Redis caching example in Node.js
const express = require('express');
const redis = require('redis');
const { promisify } = require('util');
const app = express();
const client = redis.createClient();
const getAsync = promisify(client.get).bind(client);
const setAsync = promisify(client.set).bind(client);
// Middleware to check cache before hitting database
const cacheMiddleware = async (req, res, next) => {
const cacheKey = `doctor:${req.params.doctorId}:availability`;
try {
// Check if data exists in cache
const cachedData = await getAsync(cacheKey);
if (cachedData) {
console.log('Cache hit');
return res.json(JSON.parse(cachedData));
}
// Add response data to req object to be used later
req.cacheKey = cacheKey;
next();
} catch (error) {
console.error('Cache error:', error);
next();
}
};
// Route with caching
app.get('/api/doctors/:doctorId/availability', cacheMiddleware, async (req, res) => {
try {
// Fetch data from database
const availability = await DoctorService.getAvailability(req.params.doctorId);
// Store in cache for 5 minutes (300 seconds)
if (req.cacheKey) {
await setAsync(req.cacheKey, JSON.stringify(availability), 'EX', 300);
}
res.json(availability);
} catch (error) {
res.status(500).json({ error: error.message });
}
});
Horizontal Scaling:
Adding more server instances
Load balancing across instances
Asynchronous Processing:
Using message queues for background tasks
Implementing webhooks for non-critical notifications
3.2.3 Performance Monitoring and Analysis
Real User Monitoring (RUM): Measuring actual user experiences
Application Performance Monitoring (APM): Tracking server and application metrics
Profiling: Identifying performance bottlenecks in code
3.3 Security Best Practices
Security is particularly crucial for applications handling sensitive data like healthcare information.
3.3.1 Common Security Vulnerabilities
OWASP Top 10: Understanding common web application security risks
Injection (SQL, NoSQL, Command)
Broken Authentication
Sensitive Data Exposure
XML External Entities (XXE)
Broken Access Control
Security Misconfiguration
Cross-Site Scripting (XSS)
Insecure Deserialization
Using Components with Known Vulnerabilities
Insufficient Logging & Monitoring
3.3.2 Security Implementation
Input Validation and Sanitization:
// Express validator example const { body, validationResult } = require('express-validator'); app.post('/api/appointments', [ body('doctorId').isMongoId().withMessage('Invalid doctor ID'), body('patientId').isMongoId().withMessage('Invalid patient ID'), body('date').isISO8601().withMessage('Invalid date format'), body('time').matches(/^([0-1]?[0-9]|2[0-3]):[0-5][0-9]$/) .withMessage('Time must be in format HH:MM'), body('type').isString().trim().isLength({ min: 3, max: 100 }) .withMessage('Appointment type must be between 3 and 100 characters') ], (req, res, next) => { const errors = validationResult(req); if (!errors.isEmpty()) { return res.status(400).json({ errors: errors.array() }); } next(); }, AppointmentController.createAppointment );
HTTPS Everywhere: Securing data in transit
Content Security Policy (CSP): Preventing XSS and data injection
Security Headers: Implementing HTTP security headers
Rate Limiting: Preventing abuse and brute force attacks
// Rate limiting middleware with Express const rateLimit = require('express-rate-limit'); // Limit login attempts const loginLimiter = rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 5, // 5 attempts per window message: 'Too many login attempts, please try again after 15 minutes' }); app.post('/api/login', loginLimiter, AuthController.login); // General API rate limiter const apiLimiter = rateLimit({ windowMs: 60 * 1000, // 1 minute max: 60, // 60 requests per minute message: 'Too many requests, please try again later' }); app.use('/api/', apiLimiter);
Regular Security Audits: Scanning code and dependencies for vulnerabilities
3.4 Testing and Quality Assurance
Thorough testing ensures reliable, high-quality software.
3.4.1 Testing Types
Unit Testing: Testing individual components in isolation
// Jest unit test for appointment service const AppointmentService = require('../services/appointment.service'); const AppointmentModel = require('../models/appointment.model'); // Mock the model jest.mock('../models/appointment.model'); describe('AppointmentService', () => { beforeEach(() => { jest.clearAllMocks(); }); test('should create a new appointment', async () => { // Arrange const appointmentData = { patientId: '6054b1a2e87dcf001f9e4c2a', doctorId: '6054b8a7e87dcf001f9e4c2b', date: '2025-03-15', time: '10:30', type: 'General Checkup' }; const expectedAppointment = { ...appointmentData, _id: '6054c1d9e87dcf001f9e4c2c', status: 'scheduled', createdAt: new Date() }; AppointmentModel.create.mockResolvedValue(expectedAppointment); // Act const result = await AppointmentService.createAppointment(appointmentData); // Assert expect(AppointmentModel.create).toHaveBeenCalledWith(appointmentData); expect(result).toEqual(expectedAppointment); }); });
Integration Testing: Testing interactions between components
// Supertest integration test for appointment API const request = require('supertest'); const app = require('../app'); const mongoose = require('mongoose'); const jwt = require('jsonwebtoken'); describe('Appointment API', () => { let authToken; beforeAll(async () => { // Connect to test database await mongoose.connect(process.env.TEST_DB_URI); // Create test token authToken = jwt.sign( { id: '6054b1a2e87dcf001f9e4c2a', role: 'patient' }, process.env.JWT_SECRET, { expiresIn: '1h' } ); }); afterAll(async () => { await mongoose.connection.close(); }); test('should create a new appointment', async () => { const appointmentData = { doctorId: '6054b8a7e87dcf001f9e4c2b', date: '2025-03-15', time: '10:30', type: 'General Checkup' }; const response = await request(app) .post('/api/appointments') .set('Authorization', `Bearer ${authToken}`) .send(appointmentData) .expect(201); expect(response.body).toHaveProperty('_id'); expect(response.body.status).toBe('scheduled'); expect(response.body.patientId).toBe('6054b1a2e87dcf001f9e4c2a'); }); });
End-to-End Testing: Testing complete user flows
// Cypress E2E test for appointment booking describe('Appointment Booking', () => { beforeEach(() => { // Log in user cy.login('patient@example.com', 'password123'); // Visit appointment booking page cy.visit('/appointments/new'); }); it('should allow booking an appointment', () => { // Select doctor cy.get('[data-testid="doctor-select"]').click(); cy.contains('Dr. Sarah Johnson').click(); // Select date and time cy.get('[data-testid="date-picker"]').click(); cy.contains('15').click(); cy.get('[data-testid="time-slot"]').contains('10:30 AM').click(); // Select appointment type cy.get('[data-testid="appointment-type"]').select('General Checkup'); // Add notes cy.get('[data-testid="appointment-notes"]') .type('Follow-up on recent lab results'); // Submit form cy.get('[data-testid="book-appointment-btn"]').click(); // Verify success cy.contains('Appointment booked successfully').should('be.visible'); cy.contains('March 15, 2025').should('be.visible'); cy.contains('10:30 AM').should('be.visible'); cy.contains('Dr. Sarah Johnson').should('be.visible'); }); });
Performance Testing: Testing system behavior under load
Security Testing: Identifying security vulnerabilities
3.4.2 Test-Driven Development (TDD)
TDD involves writing tests before implementing features, following this cycle:
Write a failing test
Write minimum code to pass the test
Refactor the code while maintaining test success
3.4.3 Testing Tools and Frameworks
JavaScript: Jest, Mocha, Cypress, Selenium
Python: pytest, unittest, Selenium
Java: JUnit, TestNG, Mockito
C#: NUnit, xUnit, MSTest
3.5 Accessibility and Inclusive Design
Building software that works for everyone, including people with disabilities.
3.5.1 Core Accessibility Principles
Perceivable: Information must be presentable in ways all users can perceive
Operable: Interface components must be operable by all users
Understandable: Information and operation must be understandable
Robust: Content must be robust enough to work with various user agents/assistive technologies
3.5.2 Implementing Accessibility
Semantic HTML: Using appropriate HTML elements
<!-- Poor accessibility example --> <div class="button" onclick="bookAppointment()">Book Now</div> <!-- Good accessibility example --> <button type="button" onclick="bookAppointment()">Book Now</button>
ARIA Attributes: Enhancing accessibility when HTML is insufficient
<div role="dialog" aria-labelledby="dialog-title" aria-describedby="dialog-desc" aria-modal="true"> <h2 id="dialog-title">Confirm Appointment</h2> <p id="dialog-desc">Please confirm your appointment details below.</p> <!-- Dialog content --> <button aria-label="Close dialog" class="close-button">×</button> </div>
Keyboard Navigation: Ensuring functionality without a mouse
Color Contrast: Making text readable for people with low vision
Screen Reader Support: Providing text alternatives for non-text content
Part 4: Emerging Trends and Technologies
The software development landscape continuously evolves. Here are some current trends shaping the industry:
4.1 Artificial Intelligence and Machine Learning
AI/ML is transforming software development:
Predictive Analytics: Forecasting patient appointment no-shows
Natural Language Processing: Automating clinical documentation
Computer Vision: Analyzing medical images
Recommendation Systems: Suggesting potential diagnoses
Example of using TensorFlow.js for patient no-show prediction:
// TensorFlow.js model to predict appointment no-shows
import * as tf from '@tensorflow/tfjs';
class NoShowPredictor {
constructor() {
this.model = null;
}
async loadModel() {
this.model = await tf.loadLayersModel('https://storage.googleapis.com/healthcare-models/no-show-model/model.json');
}
async predictNoShowRisk(appointmentData) {
if (!this.model) {
await this.loadModel();
}
// Process input data
const features = this.preprocessData(appointmentData);
// Make prediction
const prediction = this.model.predict(features);
const riskScore = await prediction.data();
return {
riskScore: riskScore[0],
riskLevel: this.getRiskLevel(riskScore[0])
};
}
preprocessData(appointmentData) {
// Convert appointment data into tensor
// Features: patient age, days in advance booked, history of no-shows, etc.
const featureArray = [
appointmentData.patientAge / 100, // Normalize age
appointmentData.daysInAdvance / 30, // Normalize days
appointmentData.previousNoShows / 5, // Normalize history
appointmentData.isFirstVisit ? 1 : 0,
appointmentData.hasInsurance ? 1 : 0
];
return tf.tensor2d([featureArray]);
}
getRiskLevel(score) {
if (score < 0.3) return 'low';
if (score < 0.7) return 'medium';
return 'high';
}
}
4.2 Progressive Web Apps (PWAs)
PWAs combine the best of web and mobile apps:
Offline Functionality: Working without internet connection
Push Notifications: Engaging users with timely reminders
Installation: Adding to home screen like native apps
Responsive Design: Adapting to any device
Service worker example for offline functionality:
// service-worker.js
const CACHE_NAME = 'healthcare-app-v1';
const URLS_TO_CACHE = [
'/',
'/index.html',
'/styles/main.css',
'/scripts/main.js',
'/images/logo.png',
'/offline.html'
];
// Install service worker and cache assets
self.addEventListener('install', event => {
event.waitUntil(
caches.open(CACHE_NAME)
.then(cache => {
console.log('Cache opened');
return cache.addAll(URLS_TO_CACHE);
})
);
});
// Intercept fetch requests
self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request)
.then(response => {
// Return cached response if found
if (response) {
return response;
}
// Clone the request
const fetchRequest = event.request.clone();
return fetch(fetchRequest)
.then(response => {
// Check for valid response
if (!response || response.status !== 200 || response.type !== 'basic') {
return response;
}
// Clone the response
const responseToCache = response.clone();
// Cache the fetched response
caches.open(CACHE_NAME)
.then(cache => {
cache.put(event.request, responseToCache);
});
return response;
})
.catch(() => {
// If fetch fails, return offline page
if (event.request.mode === 'navigate') {
return caches.match('/offline.html');
}
});
})
);
});
4.3 Serverless Architecture
Serverless computing allows developers to focus on code without managing infrastructure:
Function as a Service (FaaS): AWS Lambda, Azure Functions, Google Cloud Functions
Benefits: Automatic scaling, reduced operational costs, faster time to market
Use Cases: Event processing, scheduled tasks, API backends
AWS Lambda example for appointment reminders:
// AWS Lambda function for sending appointment reminders
const AWS = require('aws-sdk');
const sns = new AWS.SNS();
const dynamoDB = new AWS.DynamoDB.DocumentClient();
exports.handler = async (event) => {
try {
// Get appointments for tomorrow
const tomorrow = new Date();
tomorrow.setDate(tomorrow.getDate() + 1);
const tomorrowStr = tomorrow.toISOString().split('T')[0];
const params = {
TableName: 'appointments',
IndexName: 'date-index',
KeyConditionExpression: '#date = :date',
ExpressionAttributeNames: {
'#date': 'date'
},
ExpressionAttributeValues: {
':date': tomorrowStr
}
};
const result = await dynamoDB.query(params).promise();
// Send SMS reminders for each appointment
const reminderPromises = result.Items.map(appointment => {
const { patientPhone, patientName, time, doctorName } = appointment;
const message = `Hi ${patientName}, this is a reminder of your appointment with ${doctorName} tomorrow at ${time}. Reply Y to confirm or call our office to reschedule.`;
const snsParams = {
Message: message,
PhoneNumber: patientPhone,
MessageAttributes: {
'AWS.SNS.SMS.SMSType': {
DataType: 'String',
StringValue: 'Transactional'
}
}
};
return sns.publish(snsParams).promise();
});
await Promise.all(reminderPromises);
return {
statusCode: 200,
body: JSON.stringify({ message: `Sent ${result.Items.length} reminders` })
};
} catch (error) {
console.error('Error sending reminders:', error);
return {
statusCode: 500,
body: JSON.stringify({ error: 'Failed to send reminders' })
};
}
};
4.4 Microservices and API-First Development
Breaking applications into smaller, specialized services:
Microservices Architecture: Independent services with specific responsibilities
API Gateways: Centralizing access to microservices
Service Mesh: Managing service-to-service communication
Event-Driven Architecture: Services communicating via events
Example microservices architecture for healthcare application:
┌─────────────────────┐
│ API Gateway │
└─────────────────────┘
│
┌─────────┼─────────────────────────┐
│ │ │
▼ ▼ ▼
┌─────────────────┐ ┌─────────────────┐ ┌─────────────────┐
│ Authentication │ │ Appointment │ │ Notification │
│ Service │ │ Service │ │ Service │
└─────────────────┘ └─────────────────┘ └─────────────────┘
│ ▲
│ │
▼ │
┌─────────────────┐ │
│ Patient │─────────┘
│ Service │
└─────────────────┘
│
▼
┌─────────────────┐
│ Medical Records │
│ Service │
└─────────────────┘
4.5 WebAssembly (Wasm)
WebAssembly is enabling high-performance web applications:
Near-Native Performance: Running compiled code at near-native speed
Language Support: C, C++, Rust, and others targeting the web
Use Cases: Image processing, games, data visualization, simulations
Part 5: Best Practices and Career Development
5.1 Software Development Best Practices
5.1.1 Clean Code Principles
Readability: Code should be easy to understand
DRY (Don't Repeat Yourself): Avoid duplication
SOLID Principles: Guidelines for maintainable object-oriented code
Meaningful Names: Clear, descriptive naming conventions
5.1.2 Documentation
Code Comments: Explaining why, not what
API Documentation: Describing endpoints, parameters, and responses
README Files: Providing project overview and setup instructions
Architecture Documentation: Explaining system design decisions
5.1.3 Collaboration and Communication
Code Reviews: Peer feedback for quality assurance
Pair Programming: Two developers working together on the same task
Knowledge Sharing: Tech talks, documentation, mentoring
Agile Methodologies: Scrum, Kanban, and other collaborative frameworks
5.2 Career Development for Software Developers
5.2.1 Learning Paths
Frontend Development: HTML, CSS, JavaScript, framework specialization
Backend Development: Server-side languages, databases, APIs
Full-Stack Development: Combining frontend and backend expertise
DevOps Engineering: CI/CD, infrastructure, deployment automation
Mobile Development: Native or cross-platform mobile applications
Specialized Roles: AI/ML, security, data engineering, etc.
5.2.2 Staying Current
Continuous Learning: Online courses, books, tutorials
Open Source Contribution: Working on public projects
Technical Communities: Meetups, conferences, forums
Side Projects: Building personal applications to experiment with new technologies
5.2.3 Building a Portfolio
GitHub Profile: Showcasing your code and contributions
Personal Website: Highlighting your skills and projects
Technical Blog: Sharing knowledge and insights
Project Documentation: Demonstrating your communication skills
Conclusion: The Journey of Software Development
Software development is both an art and a science, requiring technical expertise, creativity, and continuous learning. As you progress in your career, remember that technology evolves rapidly, but fundamental principles remain constant:
Understand User Needs: Build software that solves real problems
Embrace Simplicity: Complex solutions create complex problems
Prioritize Quality: Invest in testing, security, and maintenance
Collaborate Effectively: Communication often matters more than code
Never Stop Learning: The most valuable skill is adaptability
Whether you're building a simple website or an enterprise-level healthcare system, the principles in this guide provide a foundation for creating software that is reliable, maintainable, and valuable to users.
The journey of software development is challenging but rewarding. Each line of code you write is an opportunity to solve problems, improve lives, and shape the future of technology.
Subscribe to my newsletter
Read articles from Rashindu Tharinda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
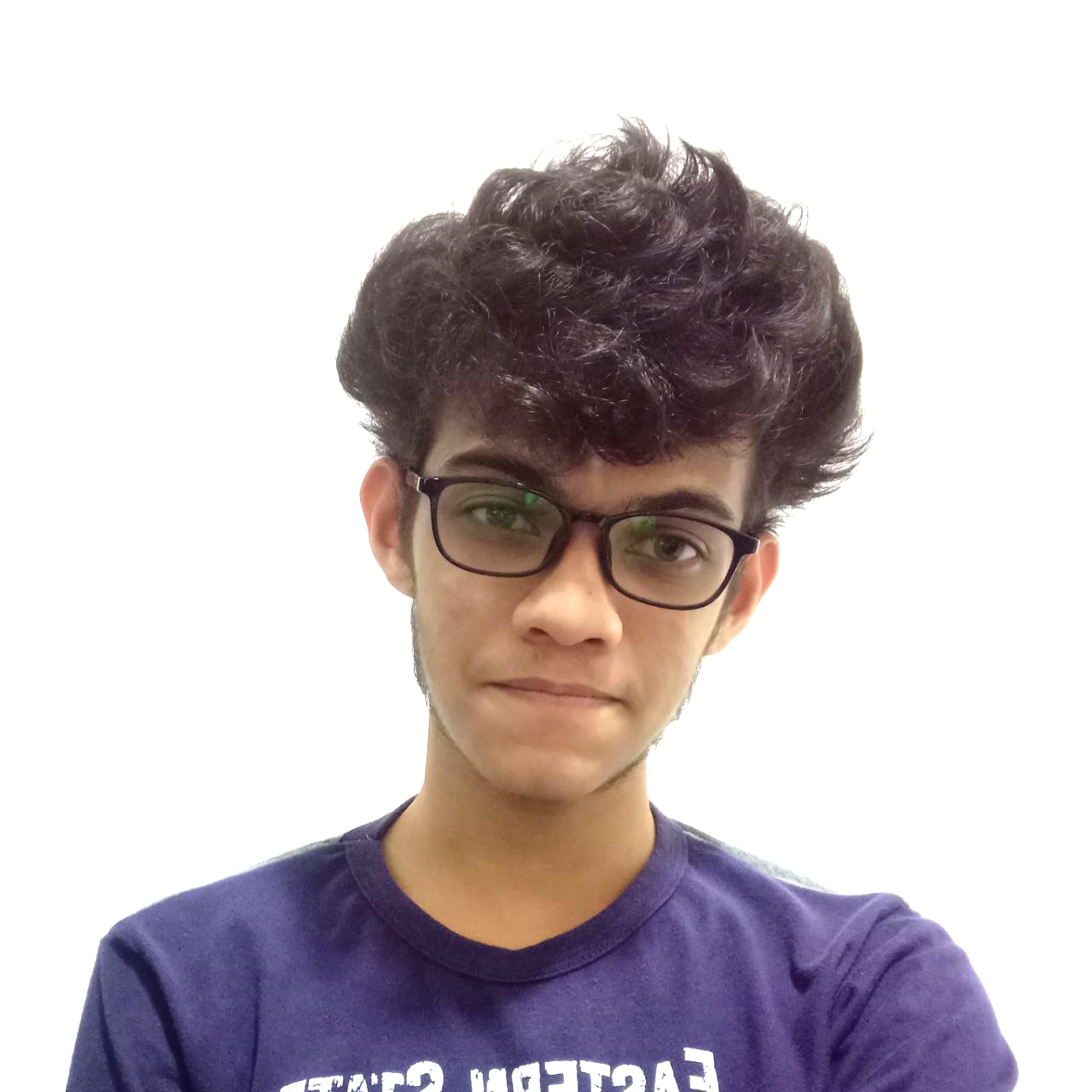