Understanding Asynchronous JavaScript: A Beginner's Guide

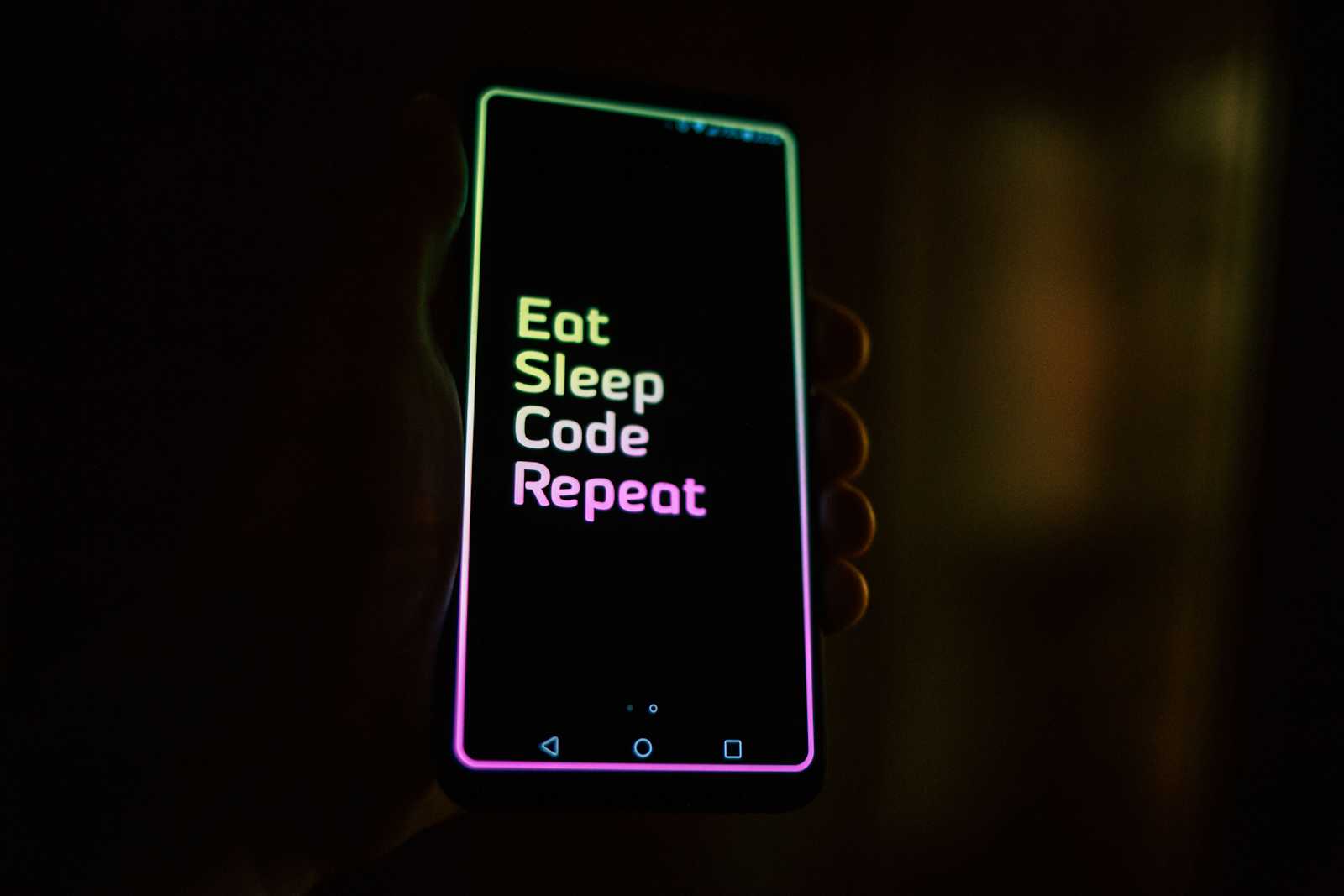
Synchronous JavaScript
Synchronous code in JavaScript runs on a single thread, meaning each operation must complete before the next one begins. This sequential execution ensures that tasks are processed one at a time.
Example of Synchronous Code
function sum(n) {
let ans = 0;
for (let i = 1; i <= n; i++) {
ans += i;
}
console.log(ans);
}
In this example, the sum
function calculates the sum of numbers from 1 to n
. The loop iterates through each number, adding it to the ans
variable. The console.log(ans)
statement waits for the loop to finish before executing, demonstrating the synchronous nature of the code.
I/O Heavy Operations
I/O (Input/Output) heavy operations involve significant interaction between the program and hardware components, such as reading or writing large files. These operations often require waiting for data transfer between the program and external sources like disks, networks, databases, or other devices.
Synchronous I/O Example
const fs = require("fs");
var a = fs.readFileSync("a.txt", "utf-8"); // Code execution is delayed here
console.log("hello");
In this synchronous example, fs.readFileSync
is used to read the contents of "a.txt". The execution of the code is paused until the file is completely read. Only after this operation is finished does the program proceed to log "hello" to the console. This delay is due to the blocking nature of synchronous I/O operations.
Asynchronous I/O Example
const fs = require("fs");
fs.readFile("a.txt", "utf-8", (err, data) => {
if (err) throw err;
console.log(data);
});
console.log("hello");
In the asynchronous version, fs.readFile
is used. Here, the execution does not wait for the file to be read. Instead, a callback function is provided, which is executed once the reading is complete. This allows "hello" to be logged to the console immediately, without waiting for the file operation to finish. The callback function handles the file content once it is available, demonstrating the non-blocking nature of asynchronous I/O operations.
A Real-world example:
Boil some water
Do some laundry
Send a package via mail
Ways to do this
One by one
Switch between them
Start all of them together and handle whichever finishes first.
const fs = require("fs")
fs.readFile("a.txt", "utf-8", function (err, contents){
console.log(contents)
})
fs.readFile("b.txt", "utf-8", function (err, contents){
console.log(contents)
})
fs.readFile("c.txt", "utf-8", function (err, contents){
console.log(contents)
})
In the example above, the code will not block because each fs
function is running concurrently, which is the most efficient way. In simple terms, we are telling the fs.readFile
function to call back the provided function in the argument whenever it is done.
Async JS Architecture
function timeout () {
console.log("Button clicked")
}
//Expensive I/O Operations
setTimeout(timeout, 1500);
console.log("Hi there")
let c = 0
//Expensive CPU operation
for(let i = 0; i<10000000000; i++){
c = c + 1
}
console.log("Completed")
The out.put will be somewhat like this
Hi there
#program delayed here because of expensive loop
Completed
Button Clicked
Code Explanation
Function Definition:
function timeout() { console.log("Button clicked"); }
This function,
timeout
, simply logs "Button clicked" to the console.Asynchronous Operation:
setTimeout(timeout, 1500);
The
setTimeout
function is used to schedule thetimeout
function to run after 1500 milliseconds (1.5 seconds). This task is pushed to the Web APIs section and runs in the background.Synchronous Operations:
console.log("Hi there");
This line immediately logs "Hi there" to the console.
Expensive CPU Operation:
let c = 0; for (let i = 0; i < 10000000000; i++) { c = c + 1; }
This loop is a CPU-intensive operation that runs synchronously, incrementing
c
a large number of times. It blocks the main thread until it completes.Completion Log:
console.log("Completed");
After the loop finishes, "Completed" is logged to the console.
Execution Flow
Initial Execution: The
setTimeout
function is called, and thetimeout
function is scheduled to run after 1.5 seconds. Meanwhile, "Hi there" is logged immediately.Blocking Operation: The loop runs, blocking the main thread due to its synchronous nature. This causes a delay in executing any other tasks.
Callback Queue: Once the loop completes, "Completed" is logged. The
timeout
function, which was waiting in the callback queue, is then executed, logging "Button clicked".
Event Loop and Queues
Callback Queue: This queue holds tasks that are ready to be executed after the current synchronous code completes. In this case, the
timeout
function waits here until the loop finishes.Microtask Queue: This queue handles high-priority tasks like Promises and
process.nextTick()
. Tasks in the microtask queue are executed before those in the callback queue, ensuring that critical operations are handled promptly.
Promises in JavaScript
A Promise is an object that represents an asynchronous operation that may complete in the future, either resolving successfully or failing with an error.
Promisifying setTimeout
To achieve a delay using Promises, you can create a promisified version of setTimeout
:
function setTimeoutPromisified(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
function callback() {
console.log("3 seconds have passed");
}
setTimeoutPromisified(3000).then(callback);
In this example, setTimeoutPromisified
returns a Promise that resolves after a specified number of milliseconds. The callback
function is executed once the Promise resolves, after 3 seconds.
If you create a Promise instance like this:
let p = setTimeoutPromisified(3000);
console.log(p);
This will log an instance of the Promise class: { <pending> }
, indicating that the Promise is still pending and has not yet resolved.
Using Promises with a Custom Function
Consider this code:
function waitFor3S(resolve) {
setTimeout(resolve, 3000);
}
function setTimeoutPromisified() {
return new Promise(waitFor3S);
}
function main() {
console.log("main is called");
}
setTimeoutPromisified().then(main);
Here, waitFor3S
is a function that takes resolve
as an argument and calls it after 3 seconds. The setTimeoutPromisified
function returns a Promise that uses waitFor3S
. When the Promise resolves, the main
function is executed.
Example with a Custom Function
function random(resolve) {
setTimeout(resolve, 3000);
}
let p = new Promise(random);
function callback() {
console.log("promise succeeded");
}
p.then(callback);
In this example, the random
function is passed to the Promise constructor. It calls resolve
after 3 seconds, triggering the callback
function via .then()
.
Promisifying File Operations
Here's an example of a promisified function that trims spaces from a file's content:
const fs = require("fs");
function trim(resolve, reject) {
fs.readFile("a.txt", "utf-8", (err, file) => {
if (err) {
return reject("Error reading file: " + err);
}
let trimmedFile = file.trim();
fs.writeFile("b.txt", trimmedFile, (err) => {
if (err) {
return reject("Error writing file: " + err);
}
resolve();
});
});
}
const clear = new Promise(trim);
clear
.then(() => {
console.log("Files are cleared");
})
.catch(err => {
console.error("Error:", err);
});
In this example, the trim
function reads a file, trims its content, and writes the trimmed content to another file. If any error occurs during reading or writing, the Promise is rejected with an error message. If successful, the Promise resolves, and the message "Files are cleared" is logged. The .catch()
method handles any errors that occur during the process.
Async Await functions
First, let's see what a callback hell is.
Consider this code:
setTimeout(function() {
conosle.log("hi")
setTimeout(function() {
console.log("hello")
setTimeout(function() {
console.log("hello there")
}, 5000);
}, 3000);
}, 1000);
This code is messy, but it prints "hi" after 1 second, then prints "hello" after 3 seconds, and finally "hello there" after 5 seconds.
Now, the promisified version of this would be
function setTimeoutPromisified(duration) {
return new Promise(function(resolve) {
setTimeout(resolve, duration);
});
}
setTimeoutPromisified(1000).then(function() {
console.log("hi");
setTimeoutPromisified(3000).then(function() {
console.log("hello");
setTimeoutPromisified(5000).then(function() {
console.log("hi there");
});
});
});
to improve it further we can use Promise Chaining.
function setTimeoutPromisified(duration) {
return new Promise(function(resolve) {
setTimeout(resolve, duration);
});
}
setTimeoutPromisified(1000).then(function() {
console.log("hi");
return setTimeoutPromisified(3000).then(function() {
console.log("hello")
return setTimeoutPromisified(5000).then(function() {
console.log("hi there")
})
})
})
Async await syntax
It's just a simplified version of promise chaining to make the code more readable.
function setTimeoutPromisified(duration) {
return new Promise(function(resolve) {
setTimeout(resolve, duration);
});
}
async function solve() {
await setTimeoutPromisified(1000);
console.log("hi")
await setTimeoutPromisified(3000);
console.log("hello");
await setTimeoutPromisified(5000);
console.log("hi there"
}
solve()
It may look like execution stops at each await call, but the function continues asynchronously while waiting for the promise to resolve..
Conclusion
Asynchronous JavaScript helps prevent thread blocking. If a task takes a long time to run, it operates in the background, letting the program continue with other code.
Promises are objects that represent a value that will be available in the future—either a resolved value or a reason for failure (error).
Subscribe to my newsletter
Read articles from Abdullah Farhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
