Easy Steps to Master Java Collections for Newbies
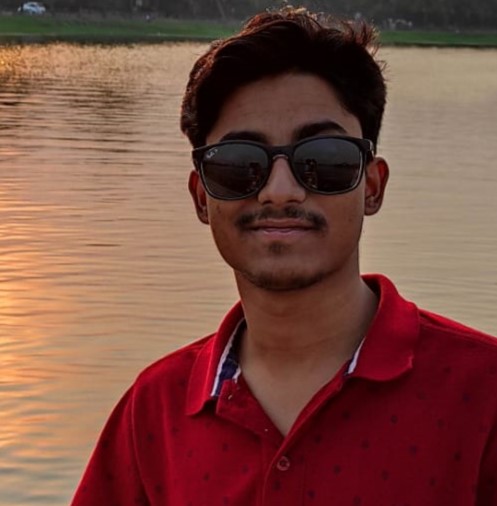
Table of contents
- 1️⃣ What is the Java Collection Framework?
- 2️⃣ Core Interfaces in Java Collections (Where I Got Confused 😅)
- 3️⃣ Lists – When You Need an Ordered Collection
- 4️⃣ Sets – When You Need Unique Elements
- 5️⃣ Maps – When You Need Key-Value Pairs
- 6️⃣ Choosing the Right Collection (What I Learned)
- 7️⃣ Iterating Over Collections (Different Ways to Loop)
- 8️⃣ Real-World Examples (How I Used These Collections)
- In a nutshell 🥜
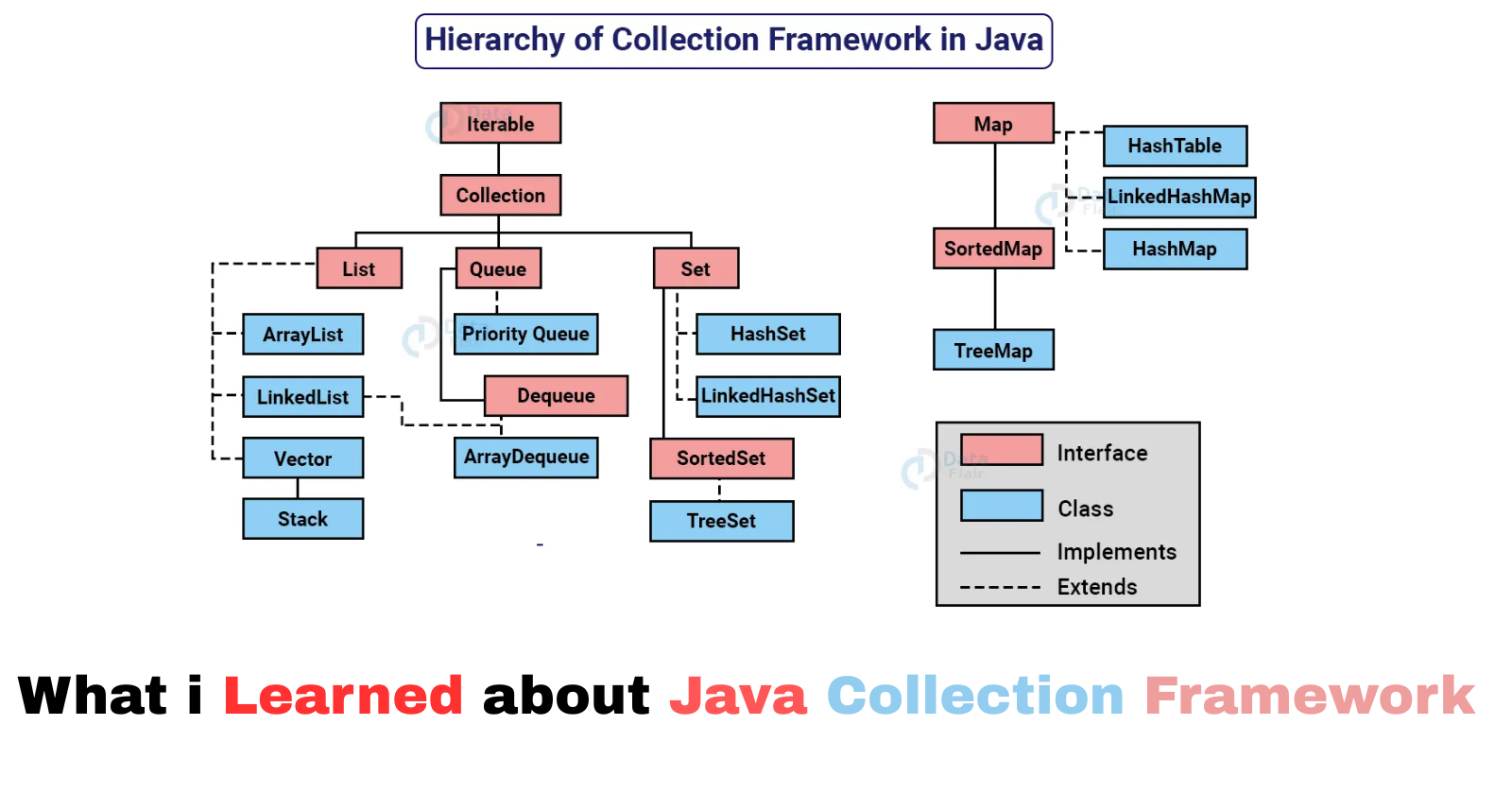
When I first started learning Java Collections, I was honestly overwhelmed by the number of interfaces and classes like ArrayList, LinkedList, HashSet, TreeMap... so many options! At first, I thought, "Do I really need all these different data structures?" But as I started learning about it, I realized that choosing the right collection can make a huge difference in performance, efficiency, and code simplicity. So, if you're just starting to learn about the Java Collection Framework (JCF), this guide is for you! I’ll share what I’ve learned, where I got stuck, and how I finally understood Collections in Java.
📌 Check out all my Java Collection practice codes here 👉 GitHub Repo
1️⃣ What is the Java Collection Framework?
In simple terms, Java Collections provide pre-built data structures that help us store and manage objects efficiently. Instead of writing our own lists, sets, or maps, Java provides them ready-to-use in the java.util
package.
Why Should You Care About Collections?
✔️ Saves time – No need to implement data structures from scratch.
✔️ Performance optimized – Different implementations for different use cases.
✔️ Scalability – Collections grow dynamically, unlike arrays.
✔️ Interoperability – Works well with Java’s built-in APIs.
When I first used ArrayList, I thought, “This feels like an advanced array.” But then I found out about LinkedList, HashSet, and TreeMap—each of them solves different problems better than an array.
2️⃣ Core Interfaces in Java Collections (Where I Got Confused 😅)
The Java Collection Framework is built around a few main interfaces, and each of them has different implementations.
Interface | Behavior | Common Implementations |
List | Ordered, allows duplicates | ArrayList , LinkedList , Vector |
Set | Unique elements, unordered | HashSet , TreeSet , LinkedHashSet |
Queue | FIFO (First In, First Out) | PriorityQueue , ArrayDeque |
Map | Key-value pairs, unique keys | HashMap , TreeMap , LinkedHashMap |
When I first saw this, I was like, “Why so many options? Can't I just use an ArrayList for everything?”
Turns out, no—each one is designed for specific needs. Let’s break them down!
3️⃣ Lists – When You Need an Ordered Collection
A List is great when you need to store elements in a specific order and allow duplicates.
🔹 ArrayList
– Fast for Searching, Slow for Insert/Delete
List<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
System.out.println(list); // Output: [Apple, Banana]
✅ Fast lookups (O(1)
).
❌ Slow insertions/deletions (O(n)
).
🔹 LinkedList
– Fast Insert/Delete, Slower Access
List<String> linkedList = new LinkedList<>();
linkedList.add("Dog");
linkedList.add("Cat");
System.out.println(linkedList); // Output: [Dog, Cat]
✅ Fast insert/delete (O(1)
).
❌ Slow random access (O(n)
).
When to Use?
ArrayList
if you need fast random access.LinkedList
if you frequently insert/delete elements.
4️⃣ Sets – When You Need Unique Elements
A Set is great when you don’t want duplicate values.
🔹 HashSet
– Unordered, Fast Lookups
Set<Integer> set = new HashSet<>();
set.add(10);
set.add(20);
set.add(10); // Duplicate will be ignored
System.out.println(set); // Output: [10, 20]
✅ Fast lookups (O(1)
).
❌ No guaranteed order.
🔹 TreeSet
– Sorted Set
Set<Integer> treeSet = new TreeSet<>();
treeSet.add(5);
treeSet.add(3);
treeSet.add(8);
System.out.println(treeSet); // Output: [3, 5, 8]
✅ Sorted order (O(log n)
).
❌ Slower than HashSet
.
5️⃣ Maps – When You Need Key-Value Pairs
Maps are perfect when you want to map a unique key to a value.
🔹 HashMap
– Fast Lookups, Unordered
Map<String, Integer> map = new HashMap<>();
map.put("Alice", 25);
map.put("Bob", 30);
System.out.println(map.get("Alice")); // Output: 25
✅ Fast access (O(1)
).
❌ No order guarantee.
🔹 TreeMap
– Sorted by Keys
Map<String, Integer> treeMap = new TreeMap<>();
treeMap.put("Banana", 2);
treeMap.put("Apple", 5);
System.out.println(treeMap); // Output: {Apple=5, Banana=2}
✅ Sorted keys (O(log n)
).
❌ Slower than HashMap
.
6️⃣ Choosing the Right Collection (What I Learned)
Need | Best Choice |
Fast lookups | HashMap , HashSet |
Ordered list | ArrayList , LinkedList |
Sorted data | TreeSet , TreeMap |
Unique elements | HashSet , TreeSet |
Thread safety | ConcurrentHashMap , Vector |
At first, I tried using ArrayList for everything, but after experimenting, I realized:
For large datasets:
HashMap
is faster than looping through anArrayList
.For fast removal:
LinkedList
is better thanArrayList
.For ensuring uniqueness:
HashSet
is better than filtering aList
.
7️⃣ Iterating Over Collections (Different Ways to Loop)
🔹 Using a For-Each Loop
for (String item : list) {
System.out.println(item);
}
🔹 Using an Iterator
Iterator<String> it = list.iterator();
while (it.hasNext()) {
System.out.println(it.next());
}
🔹 Using Streams (Java 8+)
list.stream().forEach(System.out::println);
8️⃣ Real-World Examples (How I Used These Collections)
📌 User Management System → ArrayList
for users, HashMap
for profiles.
📌 E-Commerce Cart → LinkedList
for the checkout queue.
📌 Leaderboard System → TreeMap
for ranking users.
In a nutshell 🥜
Java Collections simplify data management by providing pre-built data structures like Lists, Sets, Maps, and Queues. Instead of manually handling arrays, we can use optimized, scalable, and flexible collections.
✔ Lists (ArrayList
, LinkedList
) → Ordered, allows duplicates.
✔ Sets (HashSet
, TreeSet
) → Unique elements, no duplicates.
✔ Maps (HashMap
, TreeMap
) → Key-value pairs, fast lookups.
✔ Queues (PriorityQueue
, ArrayDeque
) → FIFO operations.
💡 Choosing the right collection is key!
Need fast lookups? →
HashMap
/HashSet
Need ordered data? →
ArrayList
/LinkedHashMap
Need sorted data? →
TreeSet
/TreeMap
Need thread safety? →
ConcurrentHashMap
Understanding when to use what makes Java programming efficient & scalable! 🚀 Check out my practice codes here 👉 GitHub Repo
If you're just starting with Java Collections, I’d love to hear which one confused you the most! Let’s discuss in the comments. 🚀😊
Subscribe to my newsletter
Read articles from Arkadipta Kundu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
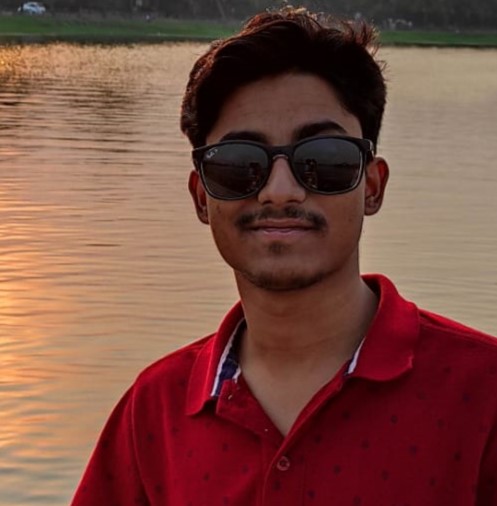
Arkadipta Kundu
Arkadipta Kundu
I’m a Computer Science undergrad from India with a passion for coding and building things that make an impact. Skilled in Java, Data Structures and Algorithms (DSA), and web development, I love diving into problem-solving challenges and constantly learning. Right now, I’m focused on sharpening my DSA skills and expanding my expertise in Java development.