Variables and Datatypes in JavaScript

Table of contents
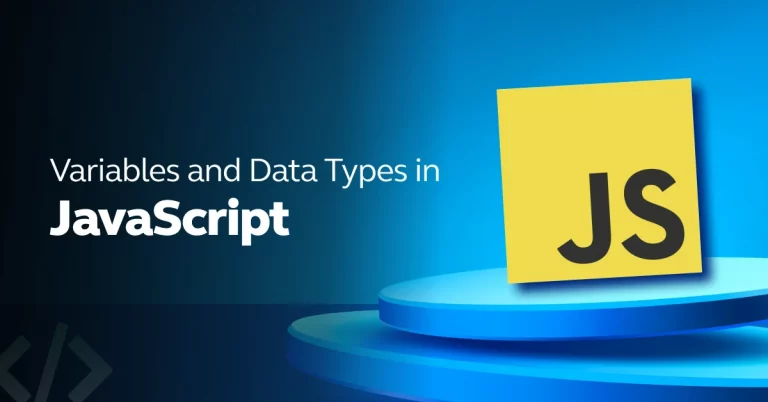
JavaScript is dynamically typed, meaning variables don’t have fixed types. Variables store values, and JavaScript has different data types to classify them.
JavaScript provides three ways to declare variables:
var
– Function-scoped, can be redeclared (not recommended).let
– Block-scoped, can be reassigned.const
– Block-scoped, cannot be reassigned.var x = 10; // Old way let y = 20; // Preferred for reassignments const z = 30; // Cannot change
JavaScript Data Types
Two main categories:
Primitive Types (stored by value):
String →
"Hello"
Number →
42
,3.14
,NaN
Boolean →
true
,false
BigInt → For very large numbers
Symbol → Unique identifiers
Undefined → No assigned value
Null → Intentional absence of value
📌 Example:
let name = "John"; // String
let age = 25; // Number
let isStudent = true; // Boolean
Non-Primitive (Reference) Types (stored by reference):
Object →
{ name: "Alice", age: 30 }
Array →
[1, 2, 3]
Function →
() => "Hello!"
📌 Example:
let person = { name: "Alice", age: 30 }; // Object
let numbers = [1, 2, 3, 4]; // Array
Type Checking
JavaScript allows us to check the type of the datatype that we are using by using the following command and the returned value will be the datatype that we are using.
Use
typeof
to check types:
console.log(typeof "Hello"); // "string"
console.log(typeof 42); // "number"
console.log(typeof null); // "object" (quirk)
Conclusion
Prefer
let
andconst
overvar
as it may cause some confusion.Use
typeof
to check data types.
Subscribe to my newsletter
Read articles from Raveena Putta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
