Limiting The Length Of A String
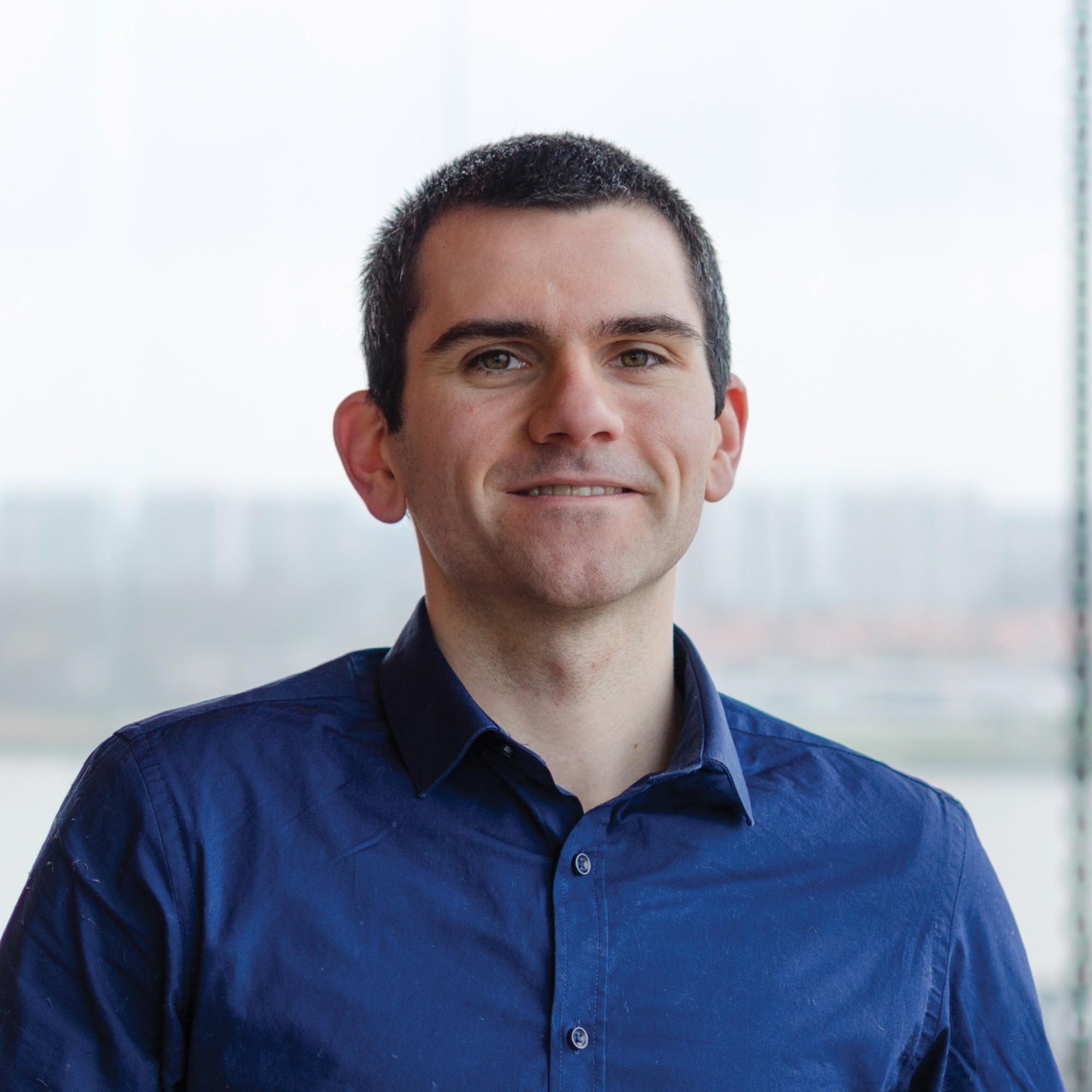
There are cases where you want to make sure that a string has only a certain max length, this is something you mainly want to do if the string comes from an external source. Trying to save this in a database or other system where the size has a certain maximum would result in errors if not handled. I recently had this situation, and I thought of two possible solutions, which I want to compare here.
The first way that comes to mind is the classic approach with a Math.max to calculate the length of the substring, which results in the following code:
input.substring(0, Math.min(input.length(), 20);
I did however uncover another approach when I was looking at some possible solutions online, one that uses the String.format function:
String.format("%.20s", input);
While the second approach is shorter, I think it is less understandable. The first approach makes the intent (of having a shorter string) much more clear by explicitly calling the substring method. While I agree that the extra call to the Math.min is a bit cumbersome, it shouldn't be a problem. It would of course be even better if there was a method called 'trimToSize(20)', but this simply doesn't exist.
Beyond the cleanliness and readability of the code, there also is the impact on performance. So I decided to run 2 simple tests:
Truncate a string that is already short enough.
Truncate a string that is too long.
Each test performs the operation 10.000 times. In order to calculate the min, max and average values, I run test test 1000 times.
SUBSTR | SHORT | LONG | FORMAT | SHORT | LONG | |
Min: | 8 | 17 | Min: | 10 | 18 | |
Max: | 37 | 48 | Max: | 43 | 58 | |
Avg: | 11 | 25 | Avg: | 12 | 25 |
All these measurements are in milliseconds, and as you can see. There isn't really much difference, but the substring approach seems to be a bit faster. Note that this is when you execute this operation 10.000 times, so the gains are very limited.
However, in my opinion this means that the substring approach is in all ways superior to the format approach. That is of course, if you don't want to add extra padding or trailing letters to your string.
Subscribe to my newsletter
Read articles from Chris Vesters directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
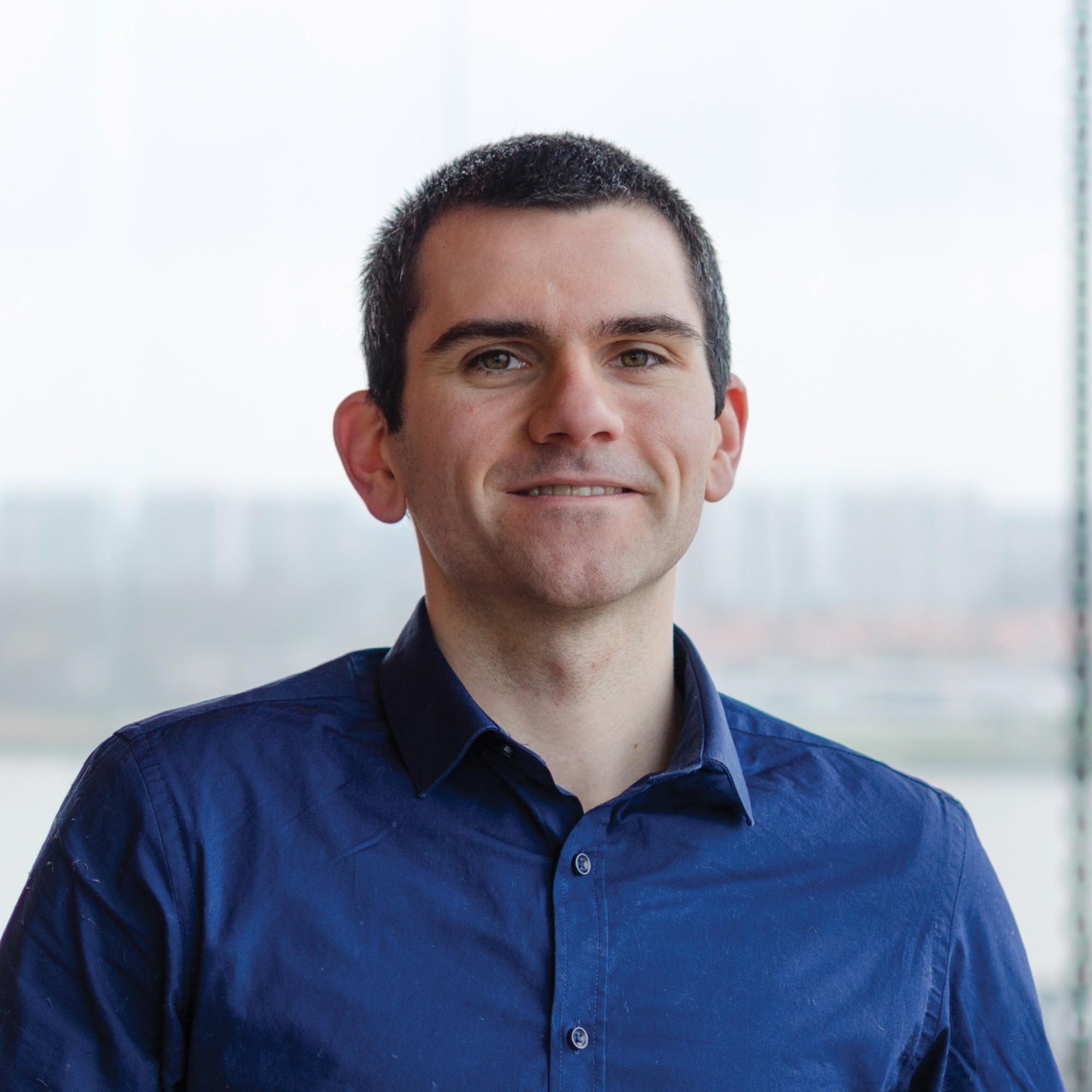