Spring Boot with Lombok: Simplifying Boilerplate Code
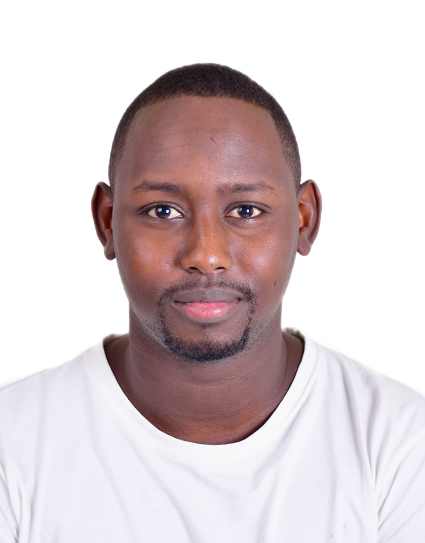

1. Introduction
Spring Boot is widely used for building Java-based web applications due to its simplicity and efficiency. However, Java’s verbosity often leads to writing repetitive boilerplate code, such as getters, setters, constructors, and toString()
methods.
This is where Project Lombok comes in. Lombok is a Java library that helps reduce boilerplate code through annotations, making development faster and more readable. It seamlessly integrates with Spring Boot, improving code maintainability.
In this blog, we’ll explore the benefits of Lombok in Spring Boot, see how to use it with practical code examples, and understand why it's a valuable addition to your projects.
2. Benefits of Lombok in Spring Boot
Lombok provides several advantages when working with Spring Boot applications:
2.1 Reduces Boilerplate Code
Java developers often write repetitive methods like getters, setters, constructors, equals, and hashCode methods. Lombok automates these using simple annotations.
2.2 Improves Readability
With Lombok, classes are cleaner and easier to read since you don’t have to manually write standard methods.
2.3 Enhances Maintainability
When adding new fields to a class, you don’t need to update multiple methods. Lombok ensures that methods like toString()
and equals()
remain up to date.
2.4 Improves Performance and Reduces Errors
Since Lombok generates methods at compile time, it improves performance and avoids manual errors from manually written boilerplate code.
3. Code Examples
Let’s explore how to use Lombok in a Spring Boot project.
3.1 Adding Lombok to a Spring Boot Project
First, add the Lombok dependency in your pom.xml
if using Maven:
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.28</version> <!-- Check for the latest version -->
<scope>provided</scope>
</dependency>
3.2 Lombok Annotations in Action
Let’s see how Lombok simplifies a typical entity class.
Without Lombok
public class User {
private Long id;
private String name;
private String email;
public User() {}
public User(Long id, String name, String email) {
this.id = id;
this.name = name;
this.email = email;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", name='" + name + '\'' +
", email='" + email + '\'' +
'}';
}
}
With Lombok
Using Lombok, the same class can be reduced to:
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data
@NoArgsConstructor
@AllArgsConstructor
public class User {
private Long id;
private String name;
private String email;
}
Here’s what these annotations do:
@Data
→ Generates getters, setters, toString, equals, hashCode, and constructor.@NoArgsConstructor
→ Generates a no-argument constructor.@AllArgsConstructor
→ Generates a constructor with all fields.
3.3 Lombok in a Spring Boot REST Controller
Lombok works well in Spring Boot applications. Here’s an example of a simple UserController.
import org.springframework.web.bind.annotation.*;
import java.util.ArrayList;
import java.util.List;
@RestController
@RequestMapping("/users")
public class UserController {
private final List<User> users = new ArrayList<>();
@PostMapping
public User addUser(@RequestBody User user) {
users.add(user);
return user;
}
@GetMapping
public List<User> getUsers() {
return users;
}
}
3.4 Lombok with JPA Entities
For Spring Boot JPA Entities, we should use Lombok carefully. Here’s how it integrates with Hibernate.
import jakarta.persistence.*;
import lombok.*;
@Entity
@Table(name = "users")
@Getter
@Setter
@NoArgsConstructor
@AllArgsConstructor
@ToString
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
}
@Getter
&@Setter
→ Generates getter and setter methods.@Entity
&@Table
→ Marks it as a JPA entity.@ToString
→ Generates atoString()
method but excludes relationships like@OneToMany
to avoid infinite recursion.
3.5 Lombok Logging
Lombok also helps in logging without explicitly declaring a logger:
import lombok.extern.slf4j.Slf4j;
import org.springframework.stereotype.Service;
@Slf4j
@Service
public class UserService {
public void logMessage() {
log.info("Lombok logging is working!");
}
}
4. Conclusion
Lombok is a powerful tool for simplifying Java development, especially in Spring Boot projects. It eliminates redundant code, improves maintainability, and enhances readability without impacting performance.
Key Takeaways:
✔ Lombok reduces boilerplate code with annotations like @Data
, @Getter
, and @Setter
.
✔ It integrates seamlessly with Spring Boot REST APIs and JPA Entities.
✔ Helps with logging using @Slf4j
for easier log management.
✔ Enhances productivity by making Java code more concise and readable.
By incorporating Lombok into your Spring Boot project, you can write cleaner, more efficient, and maintainable code. 🚀 Happy coding!
Subscribe to my newsletter
Read articles from Muhire Josué directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
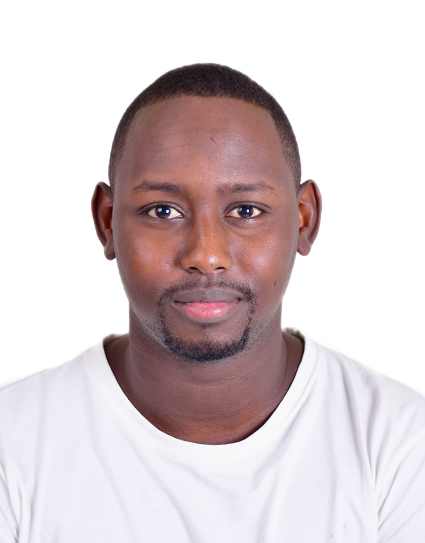
Muhire Josué
Muhire Josué
I am a backend developer, interested in writing about backend engineering, DevOps and tooling.