Creating a polyfill for useMemo in ReactJS
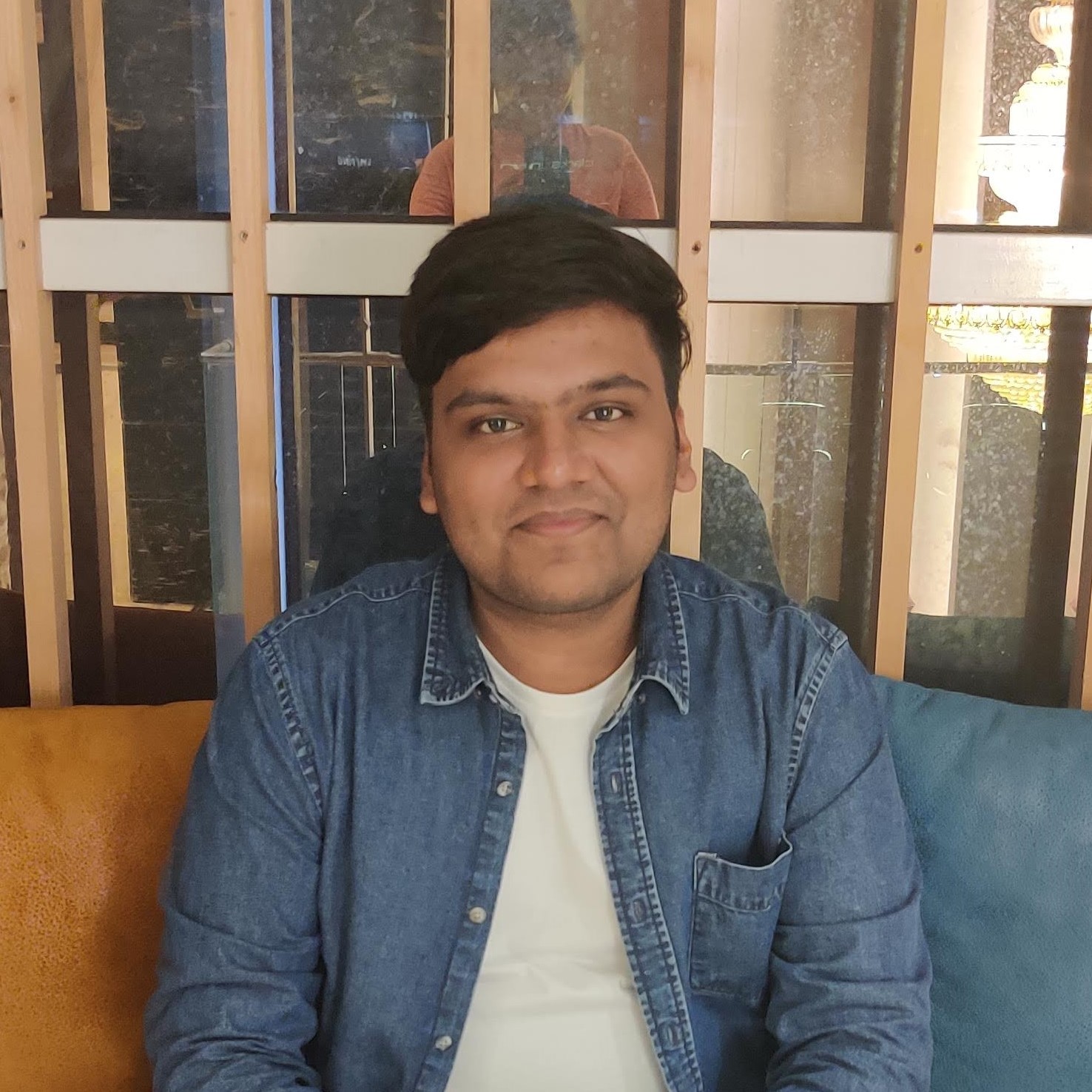
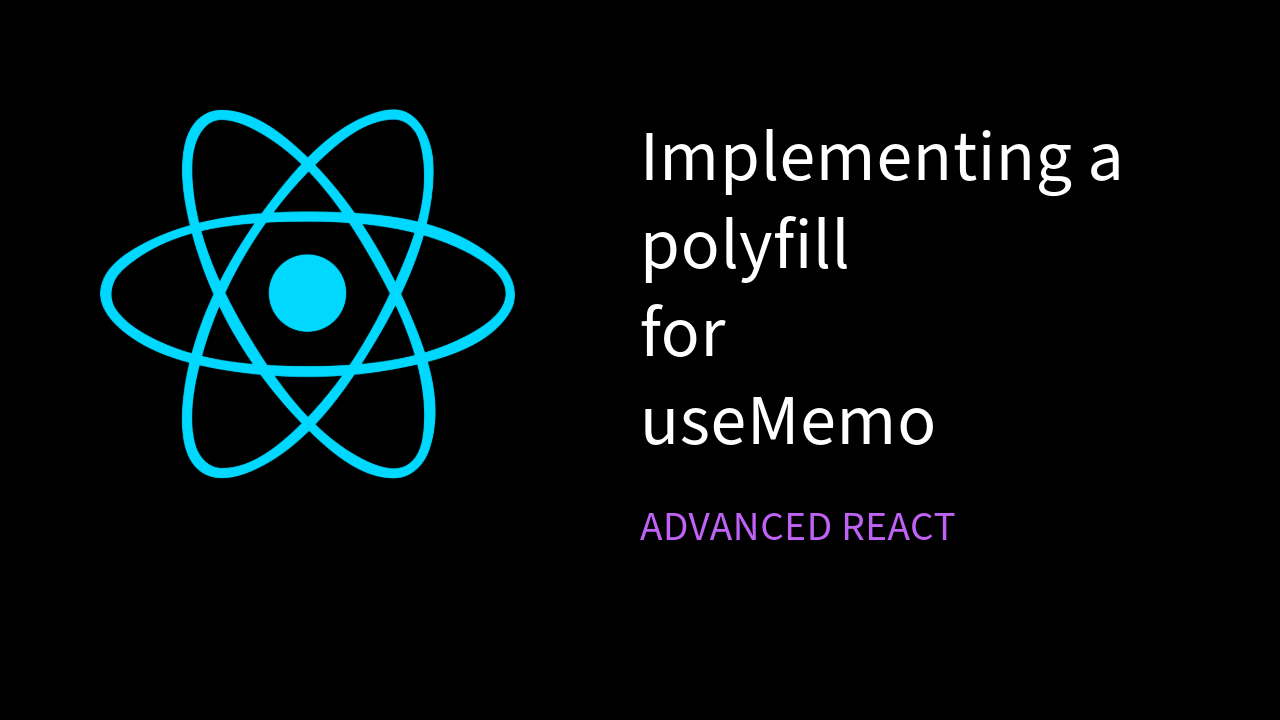
In this post, we will learn how to implement a polyfill for useMemo in ReactJS
React’s useMemo
is an essential hook for performance optimization. It helps you memoize values so that expensive computations only run when necessary. But have you ever thought about building your own version of useMemo
? Let’s break it down step by step! 🤓
🧠 What Does useMemo
Do?
useMemo
returns a memoized value that only recalculates if its dependencies change. This prevents unnecessary recalculations, especially in heavy computations.
const expensiveValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
In this example, computeExpensiveValue
only runs when a
or b
changes.
💡 Creating a Polyfill for useMemo
Let’s build a simplified version of useMemo
:
import { useRef } from 'react';
const useMemoPolyfill = (factory, dependencies) => {
const ref = useRef({ value: null, dependencies: [] });
if (
!ref.current.dependencies ||
!areDepsEqual(ref.current.dependencies, dependencies)
) {
ref.current.value = factory();
ref.current.dependencies = dependencies;
}
return ref.current.value;
};
const areDepsEqual = (oldDeps, newDeps) => {
return (
oldDeps.length === newDeps.length &&
oldDeps.every((dep, i) => dep === newDeps[i])
);
};
🛠️ Breaking It Down:
Ref Storage: We use
useRef
to store the value and dependencies.Dependency Check: We compare the old and new dependencies on each render.
Memoization: If dependencies haven’t changed, we return the cached value; otherwise, we recompute and store the new value.
📈 Why Is This Useful?
Performance Gains: Reduces redundant computations.
Interview Prep: Shows your deep understanding of React internals.
🚀 Wrapping Up
By creating a polyfill, you get hands-on experience with how React optimizes rendering. Try playing around with this hook in your projects to see its impact!
Subscribe to my newsletter
Read articles from Anmol Kansal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
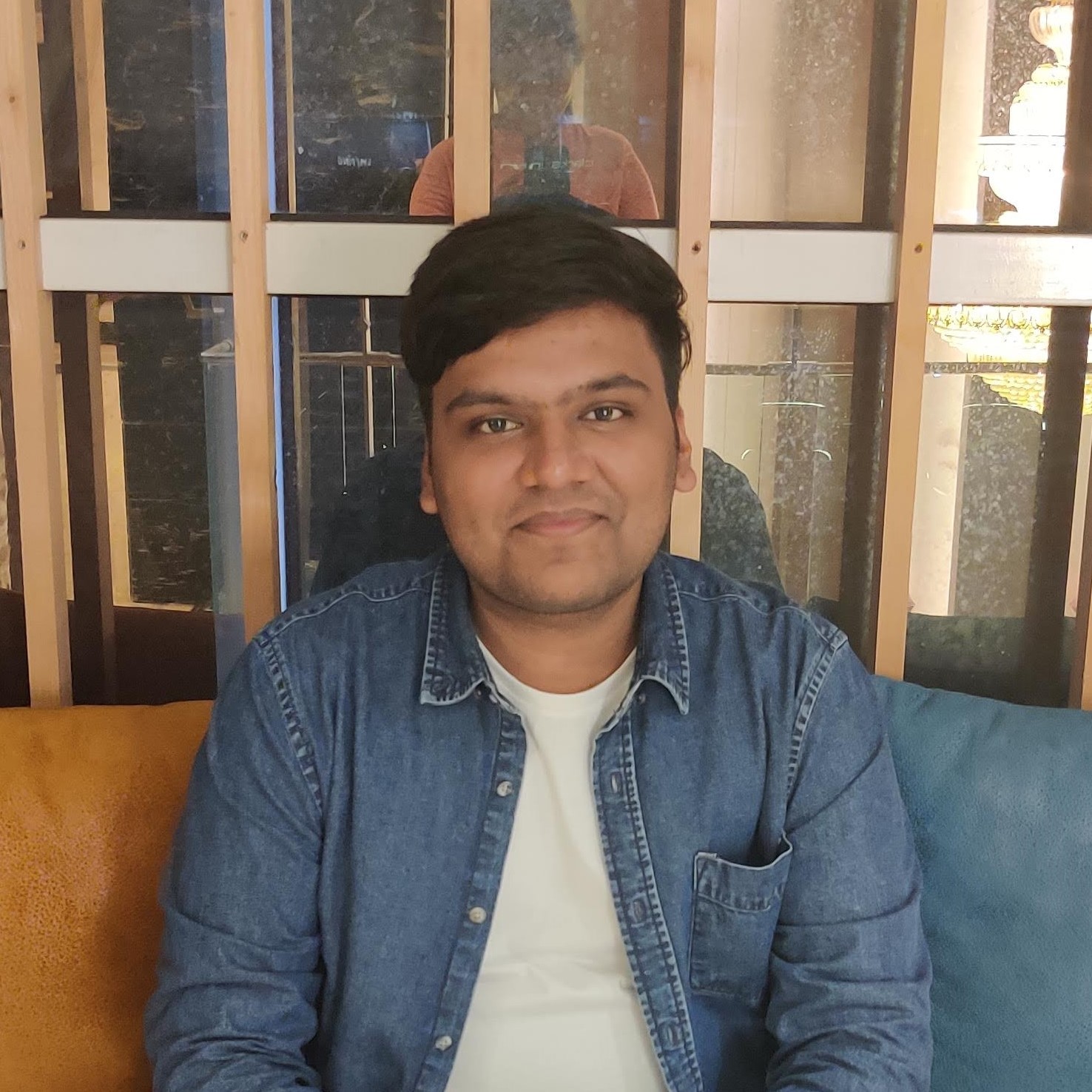
Anmol Kansal
Anmol Kansal
Web Development allows me to bring my ideas to life. Passionate about Web Development and Proficient in Data Structures and Algorithms, I like to solve challenging problems.