Debouncing and Throttling in Javascript
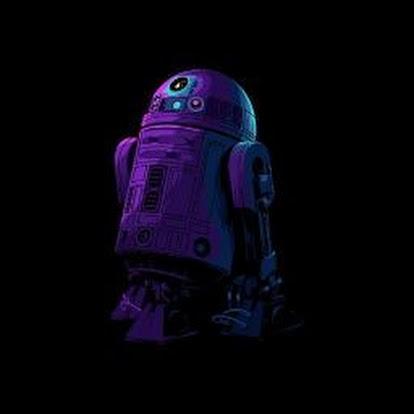
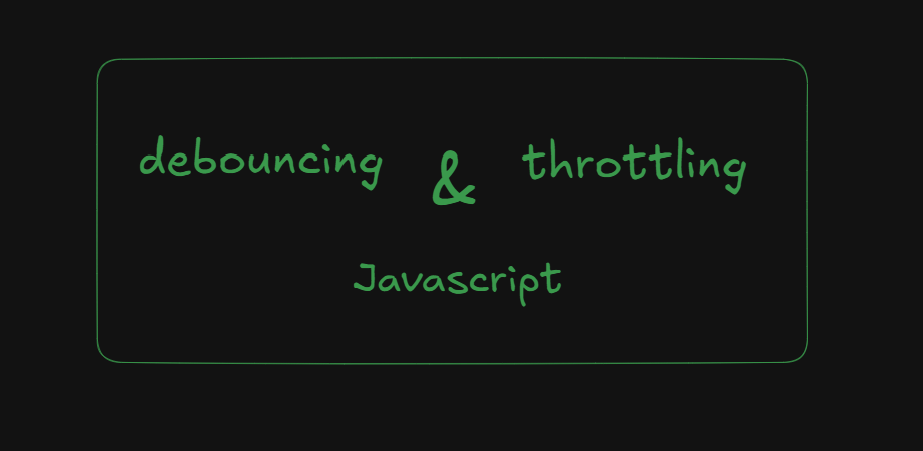
These are some of the main concept or techniques to help and control the frequency of function execution to make the application more efficient.
Understanding the problem
Imagine a search bar that makes an API call with every keystroke. Or a window resize event that triggers a complex layout recalculation every pixel change.
These scenarios can lead to problems like :
Excessive API calls, that can waste the resources of server. Performance lag, that is noticeable to user.
How debouncing and throttling can solve this problem ? and what actually is this debouncing and throttling , lets catch this jargons.
Debouncing
Debouncing delays the execution of a function until a certain amount of time has passed since the last time the event was triggered. If the event occurs before the timeout again the timer resets.
Lets see that example of search bar
function debounce(func, delay) {
let timeout;
return function(...args) {
clearTimeout(timeout);
timeout = setTimeout(() => {
func.apply(this, args);
}, delay);
};
}
A
function handleSearchInput(event) {
console.log("Searching for:", event.target.value);
callingapi(); // perform api call here
}
const debouncedSearch = debounce(handleSearchInput, 300);
const searchInput = document.getElementById("search");
searchInput.addEventListener("input", debouncedSearch);
The function debounce
here takes the function func
and delay delay
as the parameters. It returns a new function that, when called, clears any existing timeout and sets a new one.
On every keystroke searchInput.addEventListener("input", debouncedSearch);
this line of code calls the debouncesearch function again and again. now when user performs any keystroke on the search bar, the debouce function checks if there is a timeout still running then it resets the timer. if the debounce function is not called between the delay then it calls the original handleSearchInput
function.
Throttling
Throttling is very simple to understand , it just prevents to perform an API call for a certain. Or we can say it as like Throttling limits the execution of a function to a maximum of once per specified time interval .
The major use case of this technique is to limit the number of API calls.
Lets see the example :
function throttle(func, interval) {
let lastTime = 0;
return function(...args) {
const now = Date.now();
if (now - lastTime >= interval) {
func.apply(this, args);
lastTime = now;
}
};
}
function handleApiCall() {
console.log("Calling...");
// Perform api call here
}
const throttledScroll = throttle(handleScroll, 2000);
button.addEventListener("click", throttledApicall);
Here throttle
is a function to perform throttling which takes a function (func
) and an interval (interval
) as arguments.
It returns a new function that, when called, checks if the specified interval has passed since the last execution. If the interval has passed, the original function (func
) is executed, and the lastTime
variable is updated.
Debouncing vs. Throttling: when and where to use
Debouncing: Use this when you need to wait for a period of inactivity of any event before execution of a function.
Throttling: Use this when you need to limit the frequency of function execution, even if the event continues to occur.
These topics are one of the important concepts or technique to learn and make your application efficient and smooth.
Subscribe to my newsletter
Read articles from Aniket Vishwakarma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
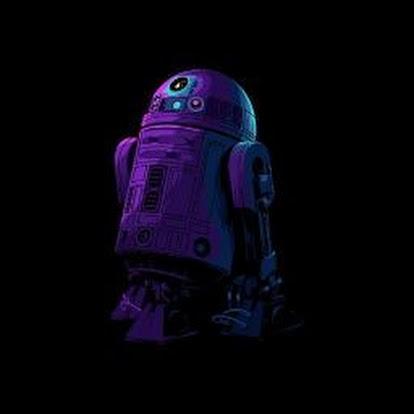
Aniket Vishwakarma
Aniket Vishwakarma
I am currently a final year grad, grinding, learning and solving problems by leveraging technology and my soft skills