Enum in Java

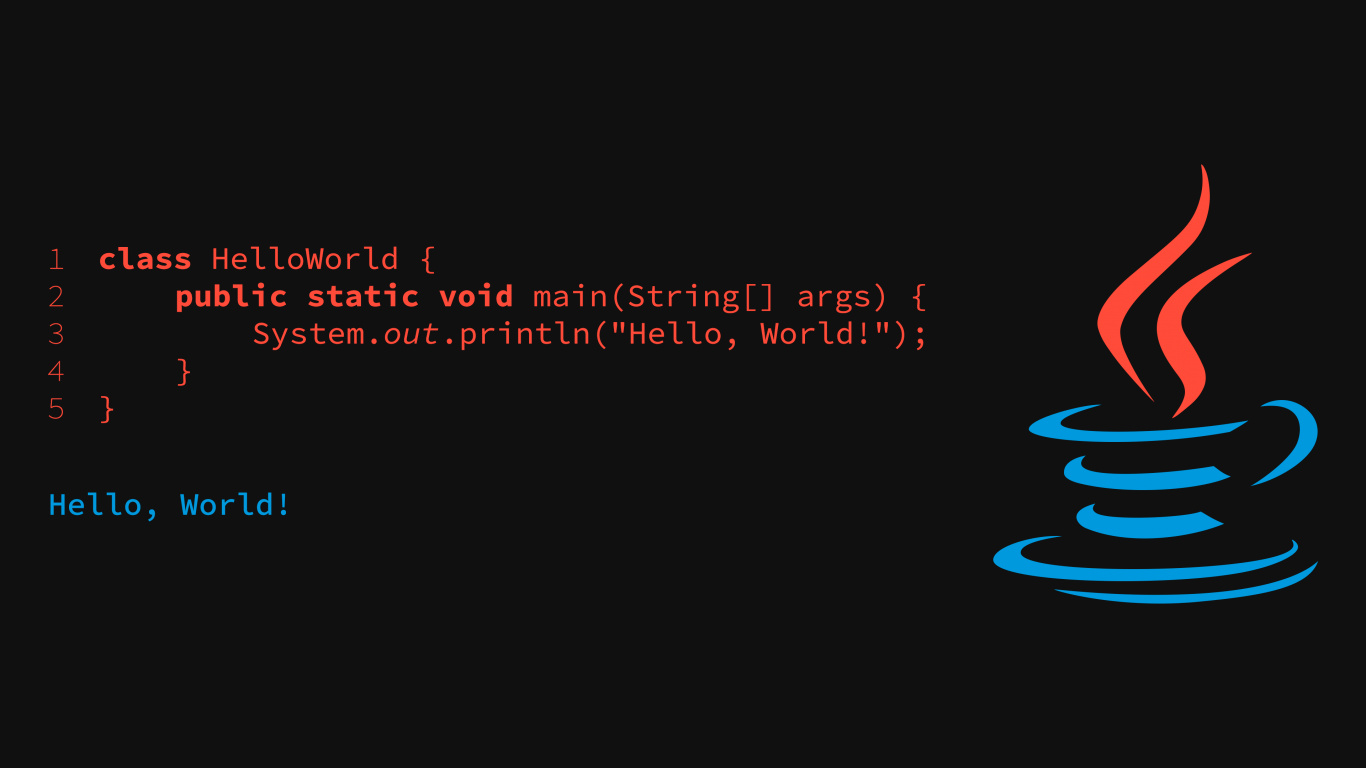
In Java, an enum
(short for enumeration) is a special data type that enables a variable to be a set of predefined constants. Enums are a powerful feature introduced in Java 5 that allows developers to create type-safe constants. Unlike traditional constants (static final
variables), enums provide additional features such as methods, fields, and constructors.
This article explores the enum
type in Java, including its usage, methods, and best practices, with code examples.
Declaring an Enum
An enum
in Java is declared using the enum
keyword. Here is a basic example:
public enum Day {
SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY;
}
In this example, Day
is an enumeration with seven constant values.
Using Enums
Enums can be used to define variables just like any other data type:
public class EnumExample {
public static void main(String[] args) {
Day today = Day.WEDNESDAY;
System.out.println("Today is: " + today);
}
}
Today is: WEDNESDAY
Enum Methods
values() Method
The values()
method returns an array of all enum constants in the order they are declared.
public class EnumValuesExample {
public static void main(String[] args) {
for (Day day : Day.values()) {
System.out.println(day);
}
}
}
SUNDAY
MONDAY
TUESDAY
WEDNESDAY
THURSDAY
FRIDAY
SATURDAY
valueOf() Method
This method returns an enum constant corresponding to a given string.
public class EnumValueOfExample {
public static void main(String[] args) {
Day day = Day.valueOf("FRIDAY");
System.out.println("Day: " + day);
}
}
Day: FRIDAY
Enum with Fields and Methods
Enums can have fields and methods, making them more powerful than traditional constants.
public enum Size {
SMALL("S"), MEDIUM("M"), LARGE("L"), EXTRA_LARGE("XL");
private String abbreviation;
Size(String abbreviation) {
this.abbreviation = abbreviation;
}
public String getAbbreviation() {
return abbreviation;
}
}
public class EnumWithFieldExample {
public static void main(String[] args) {
Size size = Size.LARGE;
System.out.println("Size: " + size + ", Abbreviation: " + size.getAbbreviation());
}
}
Size: LARGE, Abbreviation: L
Enum with Abstract Methods
Enums can define abstract methods, which must be implemented by each constant.
public enum Operation {
ADD {
@Override
public int apply(int a, int b) {
return a + b;
}
},
SUBTRACT {
@Override
public int apply(int a, int b) {
return a - b;
}
},
MULTIPLY {
@Override
public int apply(int a, int b) {
return a * b;
}
},
DIVIDE {
@Override
public int apply(int a, int b) {
return a / b;
}
};
public abstract int apply(int a, int b);
}
public class EnumWithAbstractMethodExample {
public static void main(String[] args) {
int result = Operation.ADD.apply(10, 5);
System.out.println("Addition Result: " + result);
}
}
Addition Result: 15
Enum in Switch Statement
Enums can be used in switch
statements, making code more readable and maintainable.
public class EnumSwitchExample {
public static void main(String[] args) {
Day today = Day.MONDAY;
switch (today) {
case MONDAY:
System.out.println("Start of the workweek!");
break;
case FRIDAY:
System.out.println("Weekend is coming!");
break;
case SUNDAY:
System.out.println("It's a holiday!");
break;
default:
System.out.println("Just another day.");
}
}
}
Start of the workweek!
Enum Implementing Interfaces
An enum can implement an interface to define common behavior.
interface Describable {
String getDescription();
}
public enum Status implements Describable {
SUCCESS {
public String getDescription() {
return "Operation was successful";
}
},
FAILURE {
public String getDescription() {
return "Operation failed";
}
};
}
public class EnumWithInterfaceExample {
public static void main(String[] args) {
Status status = Status.SUCCESS;
System.out.println("Status: " + status + " - " + status.getDescription());
}
}
Status: SUCCESS - Operation was successful
Conclusion
Enums in Java provide a powerful way to define constant values with additional capabilities, such as fields, methods, and constructors. They improve type safety, readability, and maintainability. By using enums properly, developers can write more robust and structured code.
We explored various aspects of enums, including:
Basic declaration and usage
Enum methods (
values()
,valueOf()
)Enum with fields and methods
Enum with abstract methods
Using enums in switch statements
Enum implementing interfaces
Understanding and effectively using enums can enhance code clarity and efficiency, making Java applications more robust and maintainable.
Subscribe to my newsletter
Read articles from Ali Rıza Şahin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ali Rıza Şahin
Ali Rıza Şahin
Product-oriented Software Engineer with a solid understanding of web programming fundamentals and software development methodologies such as agile and scrum.