Day 14: JavaScript Asynchronous Programming - Callbacks, Promises & Async/Await 🚀

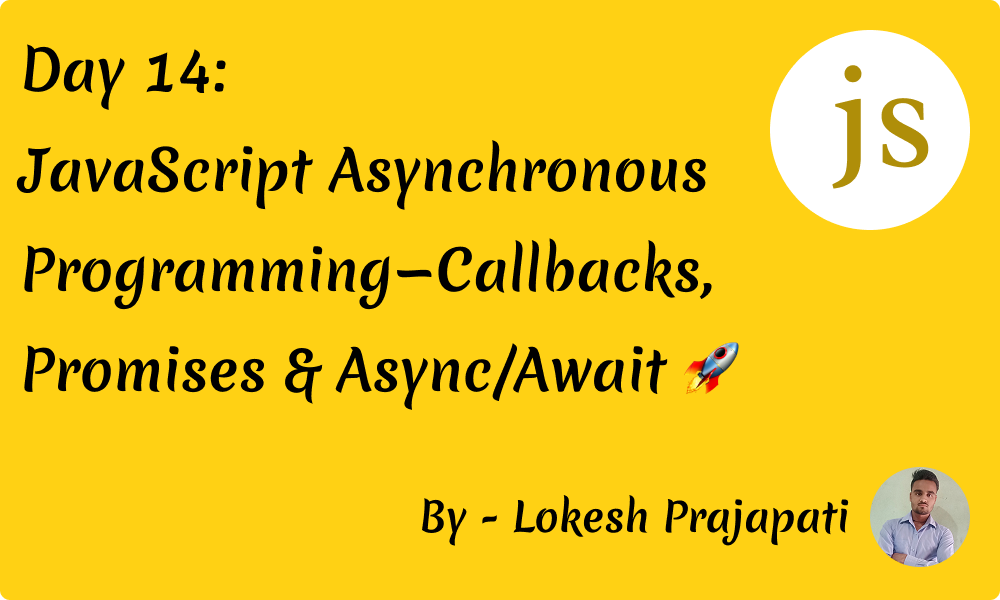
Asynchronous programming is a crucial part of modern JavaScript development. Whether you’re handling API requests, reading files, or interacting with databases, understanding async techniques like callbacks, promises, and async/await is essential.
In this post, we’ll explore these concepts step by step with clear explanations and real-life code examples. 🌟
1. Understanding Asynchronous Programming 🧠
JavaScript is single-threaded, meaning it can only execute one task at a time. However, asynchronous programming allows JavaScript to handle multiple tasks concurrently without blocking the main thread.
For example, when you request data from an API, JavaScript doesn’t pause your entire application. Instead, it continues executing other tasks and processes the API response once it arrives. 🔄
2. Callbacks — The Old School Way 📞
A callback is a function that is passed as an argument to another function and executed later.
Example of Callback Function:
function fetchData(callback) {
setTimeout(() => {
console.log("Data fetched!");
callback("Sample Data");
}, 2000);
}
function processData(data) {
console.log("Processing data:", data);
}
fetchData(processData); // Output after 2 seconds: Data fetched! Processing data: Sample Data
✅ Pros: Simple and straightforward for small tasks.
❌ Cons: Leads to callback hell when nesting multiple callbacks.
Callback Hell Example:
setTimeout(() => {
console.log("Step 1 Completed");
setTimeout(() => {
console.log("Step 2 Completed");
setTimeout(() => {
console.log("Step 3 Completed");
}, 1000);
}, 1000);
}, 1000);
👉 This messy nesting structure makes the code hard to read and maintain. 😵
3. Promises — The Modern Approach 💬
A Promise is an object that represents the eventual completion (or failure) of an asynchronous operation.
Promise States:
Pending: Initial state, neither fulfilled nor rejected.
Fulfilled: Operation completed successfully. ✅
Rejected: Operation failed. ❌
Example of Promise:
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
let success = true; // Change to 'false' to simulate an error
if (success) {
resolve("Data fetched successfully!");
} else {
reject("Error fetching data!");
}
}, 2000);
});
}
fetchData()
.then((result) => console.log(result))
.catch((error) => console.error(error));
✅ Pros: Cleaner and better for chaining operations.
❌ Cons: Still requires .then()
and .catch()
chaining, which can get complex.
4. Async/Await — The Cleanest Approach ✨
async
and await
provide a modern way to write asynchronous code that looks and behaves like synchronous code.
Key Rules:
Use
async
before a function to make it return a promise. 🔥Use
await
to pause execution until a promise resolves. 🛑
Example of Async/Await:
async function fetchData() {
try {
let response = await new Promise((resolve) => {
setTimeout(() => resolve("Data fetched successfully!"), 2000);
});
console.log(response);
} catch (error) {
console.error("Error:", error);
}
}
fetchData();
✅ Pros: Cleaner, more readable code. Ideal for complex workflows.
❌ Cons: Requires proper error handling using try-catch
. ⚠️
5. Real-Life Example: Fetching API Data 🌍
Imagine you are fetching user data from an API. Let’s see how this works using all three methods:
Using Callbacks:
function fetchUserData(callback) {
setTimeout(() => {
callback({ name: "John Doe", age: 30 });
}, 2000);
}
fetchUserData((user) => console.log("User Data:", user));
Using Promises:
function fetchUserData() {
return new Promise((resolve) => {
setTimeout(() => {
resolve({ name: "John Doe", age: 30 });
}, 2000);
});
}
fetchUserData().then(user => console.log("User Data:", user));
Using Async/Await:
async function fetchUserData() {
let user = await new Promise((resolve) => {
setTimeout(() => resolve({ name: "John Doe", age: 30 }), 2000);
});
console.log("User Data:", user);
}
fetchUserData();
6. Which Method Should You Use? 🤔
✅ Use Callbacks
for simple one-time asynchronous tasks.
✅ Use Promises
when chaining multiple async tasks.
✅ Use Async/Await
for clean, readable, and maintainable code.
For modern JavaScript development, Async/Await
is highly recommended as it simplifies async code significantly. 🔥
7. Conclusion 🏁
Mastering asynchronous programming is key to becoming an efficient JavaScript developer. Understanding callbacks, promises, and async/await will help you build scalable and error-free applications. Start practicing these concepts in real-life scenarios to gain confidence. 💪
Have any questions or tips? Feel free to share in the comments below! 😊
Subscribe to my newsletter
Read articles from Lokesh Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lokesh Prajapati
Lokesh Prajapati
🚀 JavaScript | React | Shopify Developer | Tech Blogger Hi, I’m Lokesh Prajapati, a passionate web developer and content creator. I love simplifying JavaScript, React, and Shopify development through easy-to-understand tutorials and real-world examples. I’m currently running a JavaScript Basics to Advanced series on Medium & Hashnode, helping developers of all levels enhance their coding skills. My goal is to make programming more accessible and practical for everyone. Follow me for daily coding tips, tricks, and insights! Let’s learn and grow together. 💡🚀 #JavaScript #React #Shopify #WebDevelopment #Coding #TechBlogger #LearnToCode