Terraform Associate: Protect Sensitive Input Variables

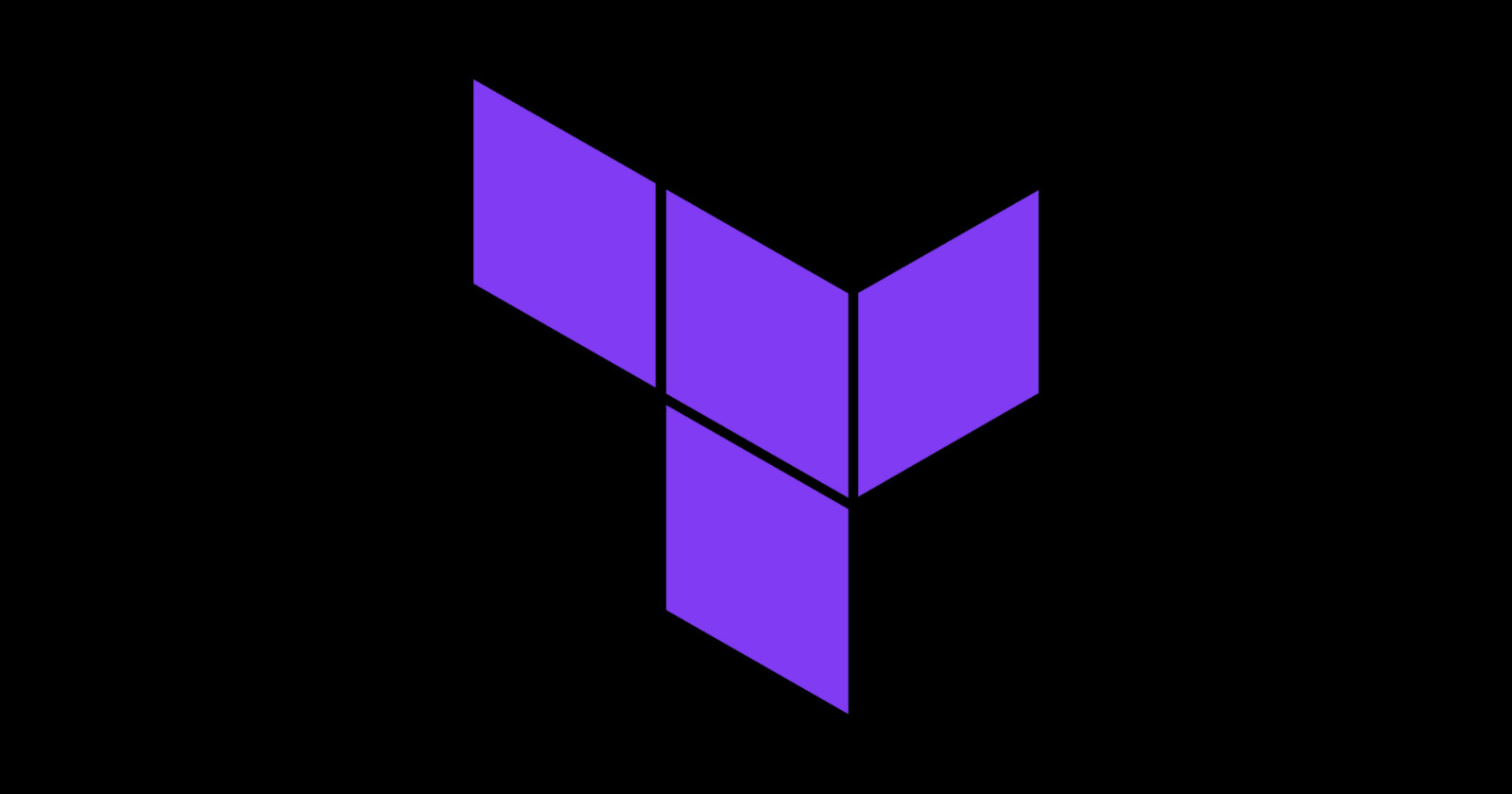
When managing infrastructure with Terraform, handling sensitive information securely is critical. Credentials like database passwords, API keys, and private keys must be protected to prevent accidental exposure. Terraform provides multiple mechanisms to safeguard sensitive input variables, ensuring that these secrets do not appear in logs, output, or version control. In this blog post, we’ll explore several best practices and techniques for protecting sensitive input variables in Terraform.
1. Marking Variables as Sensitive
Terraform allows you to mark input variables as sensitive so that their values are not displayed in the command-line output or logs. This is done by setting the sensitive
attribute to true
when declaring a variable.
Defining a Sensitive Variable
Create a variable in your configuration file (e.g., variables.tf
) and mark it as sensitive:
variable "db_password" {
description = "The database password"
type = string
sensitive = true
}
Using Sensitive Variables in Outputs
Even when outputting sensitive data, Terraform can mask these values:
output "database_password" {
value = var.db_password
sensitive = true
}
When you run:
terraform output
The output will be masked:
database_password = <sensitive>
Note: While marking a variable as
sensitive
prevents it from being printed in outputs and logs, it does not prevent the value from being stored in the Terraform state file. Additional measures are needed to secure the state file.
2. Passing Sensitive Variables Securely
a) Using Environment Variables
One of the safest methods to provide sensitive values is through environment variables. Terraform will automatically use environment variables prefixed with TF_VAR_
to assign values to corresponding variables.
Example
For a variable named db_password
:
export TF_VAR_db_password="my-secure-password"
terraform apply
This approach ensures that sensitive values are not hardcoded in your configuration or .tfvars
files.
b) Using a .tfvars
File (And Excluding It from Version Control)
You can also store sensitive data in a separate .tfvars
file, such as secrets.tfvars
, and make sure this file is excluded from your version control system.
Example: secrets.tfvars
db_password = "supersecret123"
Run Terraform with the variable file:
terraform apply -var-file="secrets.tfvars"
Secure Your .tfvars
Files
Add these files to your .gitignore
to prevent accidental commits:
# Ignore variable files containing secrets
*.tfvars
Warning: If you commit a
.tfvars
file containing sensitive information, immediately rotate those credentials.
c) Integrating with Secret Managers
For enhanced security, retrieve sensitive data directly from a secure vault like AWS Secrets Manager, HashiCorp Vault, or Azure Key Vault. This approach eliminates the need to store secrets in your Terraform code.
Example: Using AWS Secrets Manager
data "aws_secretsmanager_secret_version" "db_password" {
secret_id = "my-db-password"
}
variable "db_password" {
description = "The database password"
type = string
sensitive = true
default = data.aws_secretsmanager_secret_version.db_password.secret_string
}
By fetching secrets at runtime, you keep sensitive information out of your configuration files entirely.
3. Encrypting the Terraform State File
Even if you mark variables as sensitive, their values are still stored in the Terraform state file (terraform.tfstate
). Securing this file is vital to protect sensitive data.
a) Use an Encrypted Remote Backend
Storing your state file remotely in a secure, encrypted backend can significantly reduce the risk of exposing sensitive data.
Example: AWS S3 with Encryption
terraform {
backend "s3" {
bucket = "my-terraform-state-bucket"
key = "terraform.tfstate"
region = "us-east-1"
encrypt = true
kms_key_id = "arn:aws:kms:us-east-1:123456789012:key/my-kms-key"
}
}
This configuration ensures that the state file is encrypted at rest using AWS KMS.
b) Restrict Access to the State File
Limit access: Only authorized users should have access to the remote backend.
Enable auditing and logging: Monitor who accesses your state file for added security.
4. Best Practices for Handling Sensitive Variables
Always use
sensitive = true
: Prevent secrets from being printed in logs or outputs.Prefer environment variables: Set sensitive values using environment variables (
TF_VAR_
) instead of hardcoding them.Store secrets outside of version control: Use
.tfvars
files for sensitive data, but ensure they are excluded from Git.Leverage secret managers: Integrate with AWS Secrets Manager, HashiCorp Vault, or similar services to fetch secrets at runtime.
Encrypt and secure the state file: Use encrypted backends and restrict access to your Terraform state.
Conclusion
Protecting sensitive input variables in Terraform is a crucial aspect of infrastructure security. By marking variables as sensitive, securely passing values through environment variables or secret managers, and encrypting your state file, you can significantly reduce the risk of exposing critical data.
Implement these best practices to keep your secrets safe and maintain a secure Terraform environment. Happy, secure Terraforming!
Reference
Terraform Sensitive Variables Documentation
Learn how to mark variables as sensitive in Terraform to prevent secrets from appearing in logs and outputs.Terraform Backends Configuration
Explore how to configure remote backends with encryption and access restrictions to secure your Terraform state file.Using Environment Variables in Terraform
Understand how Terraform utilizes environment variables (TF_VAR_) to assign values to variables securely, avoiding hardcoding sensitive information.Integrating Terraform with Secret Managers
Discover how to fetch secrets at runtime from tools like HashiCorp Vault, AWS Secrets Manager, or Azure Key Vault to keep sensitive data out of your configuration files.Terraform State Security Best Practices
Dive into best practices for managing and protecting your Terraform state file, ensuring that sensitive information remains secure at all times.
Subscribe to my newsletter
Read articles from Chintan Boghara directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Chintan Boghara
Chintan Boghara
Exploring DevOps ♾️, Cloud Computing ☁️, DevSecOps 🔒, Site Reliability Engineering ⚙️, Platform Engineering 🛠️, Machine Learning Operations 🤖, and AIOps 🧠