Callback functions and their features

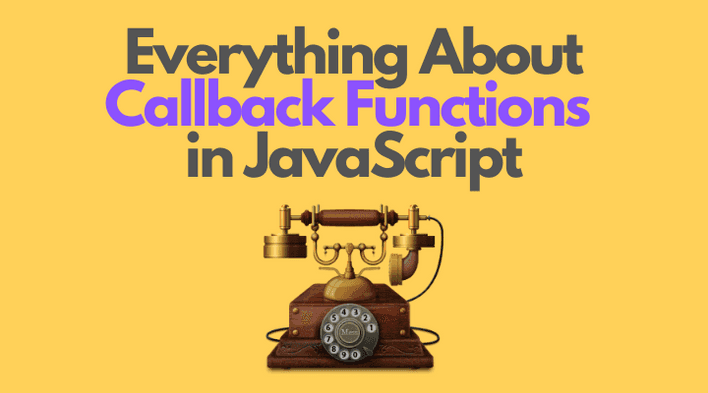
Understanding Callback Functions in JavaScript
1. What is a Callback Function?
A callback function is a function that is passed as an argument to another function and is executed later when needed.
Why are Callbacks Important?
Callbacks allow JavaScript to handle asynchronous operations, such as:
✅ Fetching data from a server
✅ Reading files
✅ Handling user interactions
✅ Running code after a delay
2. How to Use a Callback Function?
Let’s start with a simple example:
Example: Basic Callback Function
function greet(name, callback) {
console.log("Hello, " + name);
callback(); // Executing the callback function
}
function sayGoodbye() {
console.log("Goodbye!");
}
greet("Raveena", sayGoodbye);
🔹 Here, sayGoodbye
is passed as a callback to greet
. After printing "Hello, Raveena"
, it executes sayGoodbye()
.
Output:
Hello, Raveena
Goodbye!
3. Callbacks in Asynchronous JavaScript
JavaScript executes code line by line, but some operations take time (e.g., fetching data). Instead of blocking execution, callbacks wait for the task to complete and then execute.
Example: Using Callbacks in setTimeout
console.log("Start");
setTimeout(() => {
console.log("Callback executed after 2 seconds");
}, 2000); // Executes after 2 seconds
console.log("End");
🔹 Here, setTimeout
waits for 2 seconds before executing the callback function.
Output:
Start
End
Callback (executed after 2 seconds)
4. Callbacks in API Requests
Callbacks are commonly used when fetching data from a server.
Example: Fetching Data Using a Callback
function fetchData(callback) {
fetch("https://jsonplaceholder.typicode.com/posts/1")
.then(response => response.json())
.then(data => callback(data))
.catch(error => console.log("Error:", error));
}
fetchData((data) => {
console.log("Fetched Data:", data);
});
🔹 The fetchData
function gets data from an API and calls the callback function when the data is received.
5. The Problem of Callback Hell
If you nest too many callbacks, the code becomes unreadable and difficult to maintain. This is called Callback Hell.
Example: Callback Hell
function step1(callback) {
setTimeout(() => {
console.log("Step 1 completed");
callback();
}, 1000);
}
function step2(callback) {
setTimeout(() => {
console.log("Step 2 completed");
callback();
}, 1000);
}
function step3(callback) {
setTimeout(() => {
console.log("Step 3 completed");
callback();
}, 1000);
}
// Nested callbacks (Callback Hell)
step1(() => {
step2(() => {
step3(() => {
console.log("All steps completed");
});
});
});
🔹 The code is deeply nested, making it hard to read.
Output:
Step 1 completed
Step 2 completed
Step 3 completed
All steps completed
6. How to Avoid Callback Hell?
JavaScript provides better alternatives to callbacks:
✅ Promises → Allow chaining of asynchronous operations
✅ Async/Await → Makes asynchronous code look like synchronous code
We will learn about these promises and async/await in a complete new article.
Conclusion
✔ Callbacks allow functions to be executed later, making JavaScript handle asynchronous tasks effectively.
✔ Too many nested callbacks (Callback Hell) make the code difficult to manage.
Subscribe to my newsletter
Read articles from Raveena Putta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
