Deep dive into V8 JS Engine
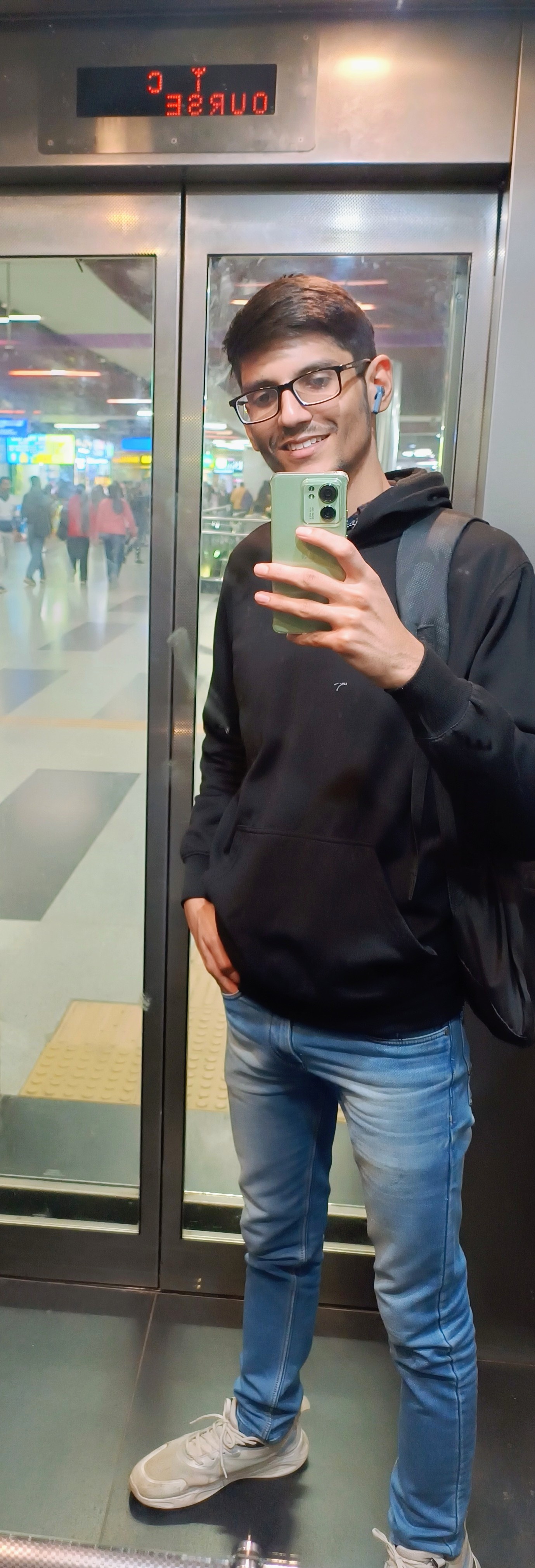
Parsing Stage:
When JavaScript code is given to the V8 engine, the first step is lexical analysis, where the code is broken into tokens.
Then, it moves to syntax analysis, where the tokens are converted into an Abstract Syntax Tree (AST).
🚀 Tech Fact: "No AST, No Execution! A SyntaxError means your code didn’t even make it past the parsing stage
Abstract Syntax Tree (AST) representation for the JavaScript code:
var a = "priyanshu";
{
"type": "VariableDeclaration",
"declarations": [
{
"type": "VariableDeclarator",
"id": {
"type": "Identifier",
"name": "a"
},
"init": {
"type": "Literal",
"value": "priyanshu",
"raw": "\"priyanshu\""
}
}
],
"kind": "var"
}
Execution Stage:
- The AST is then passed to an interpreter for execution.
Compiler vs Interpreter:
Interpreter: Executes code line by line, making the initial execution fast but overall slower.
Compiler: Translates the entire code into machine code before execution, which makes the initial process heavy but execution fast.
Is JavaScript a Compiler or Interpreter?
- JavaScript uses Just-In-Time (JIT) Compilation, meaning it compiles code at runtime, combining the benefits of both interpreters and compilers.
1. Execution Begins (Interpreter - Ignition)
After parsing, the Abstract Syntax Tree (AST) is passed to Ignition, the interpreter inside V8.
Ignition converts the AST into bytecode and begins executing it.
2. Optimization (Compiler - TurboFan)
While executing, Ignition detects frequently used functions or code patterns that can be optimized.
These "hot" functions are passed to TurboFan, the JIT (Just-In-Time) compiler.
TurboFan compiles the bytecode into highly optimized machine code.
The optimized machine code is then executed instead of the slower bytecode.
3. Deoptimization (When Needed)
If the assumptions made by TurboFan for optimization turn out to be incorrect (e.g., variable types change), the optimized machine code is discarded.
The execution falls back to the bytecode from Ignition, ensuring correctness.
Subscribe to my newsletter
Read articles from Priyanshu Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
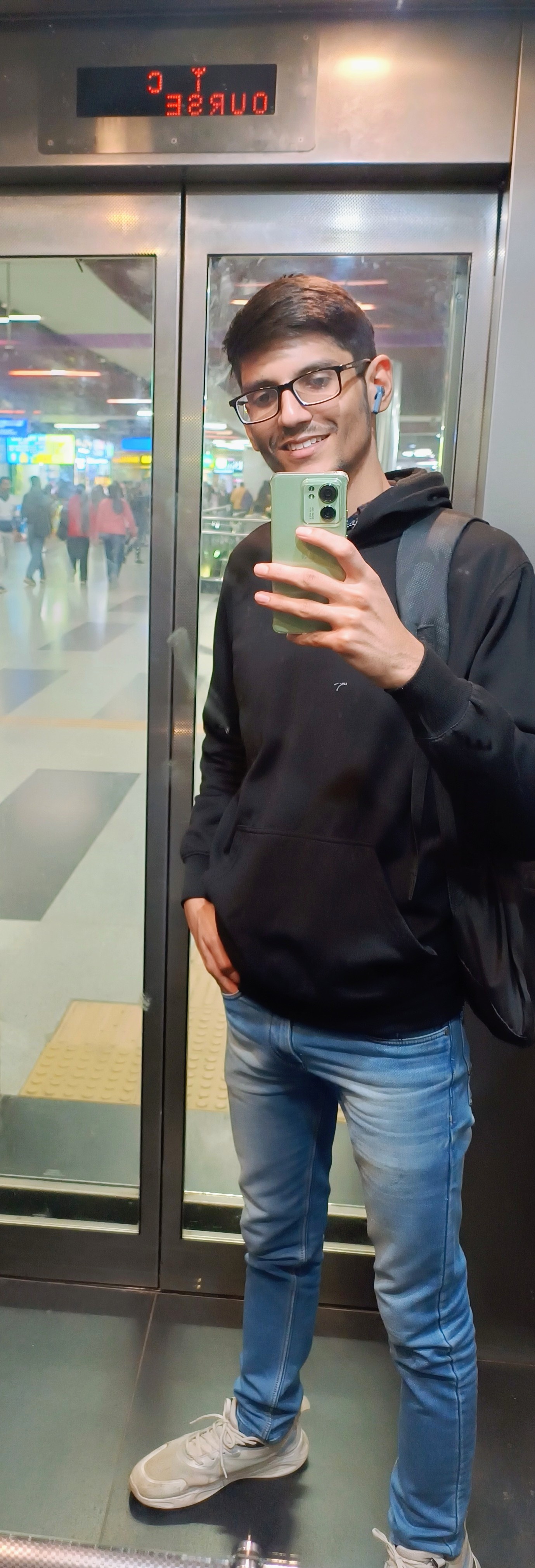