How to Export HTML Table to Excel: A Step-by-Step Guide

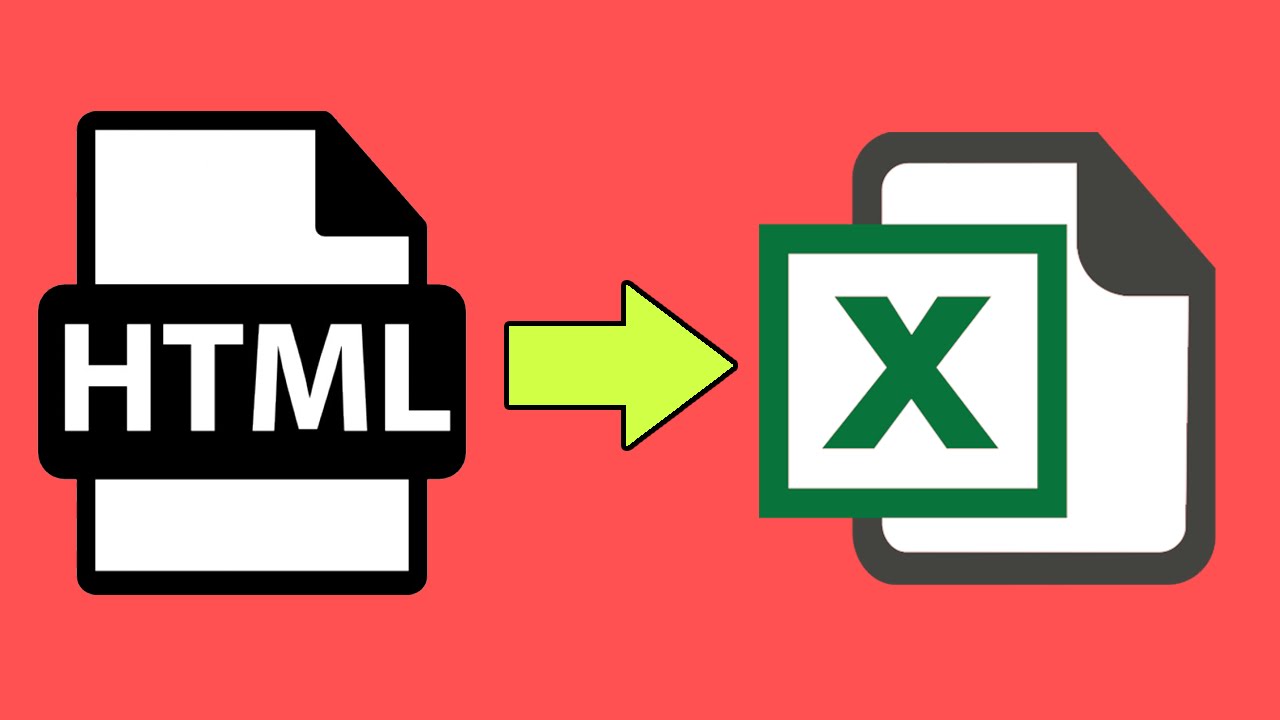
Exporting a table in HTML to Excel is an important capability for most web applications, particularly when working with large datasets. Whether you are creating a report tool or financial dashboard, or simply need to save tabular data in a structured form, exporting to Excel makes it simpler to allow users to manipulate and analyze data.
In this guide, we’ll explore multiple ways to export an HTML table to Excel, using both JavaScript and third-party libraries.
Why Export HTML Tables to Excel?
Before diving into the technical details, let’s look at why exporting an HTML table to Excel is useful:
Data Analysis – Excel allows users to sort, filter, and analyze tabular data efficiently.Easy Sharing – Excel files can be shared via email or cloud storage for collaboration.Data Backup – Storing data in Excel helps maintain records for future use.Improved Productivity – Users can quickly perform calculations, create charts, and apply formulas.
Methods to Export an HTML Table to Excel
There are several ways to export an HTML table to an Excel file. Below, we’ll discuss three popular methods:
Using JavaScript and Blob API
Using TableExport.js (A JavaScript Library)
Using PHP and CSV Format
Method 1: Export HTML Table to Excel Using JavaScript
One of the simplest ways to export an HTML table to an Excel file is by using JavaScript and the Blob
API.
Step-by-Step Code Example:
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Export Table to Excel</title>
</head>
<body>
<table id="myTable" border="1">
<tr>
<th>Name</th>
<th>Age</th>
<th>Country</th>
</tr>
<tr>
<td>John Doe</td>
<td>28</td>
<td>USA</td>
</tr>
<tr>
<td>Jane Smith</td>
<td>34</td>
<td>UK</td>
</tr>
</table>
<button onclick="exportTableToExcel('myTable', 'data')">Export to Excel</button>
<script>
function exportTableToExcel(tableID, filename = '') {
let table = document.getElementById(tableID);
let html = table.outerHTML.replace(/ /g, '%20');
let uri = 'data:application/vnd.ms-excel,' + html;
let link = document.createElement("a");
link.href = uri;
link.download = filename ? filename + ".xls" : "table.xls";
document.body.appendChild(link);
link.click();
document.body.removeChild(link);
}
</script>
</body>
</html>
Pros: Simple and doesn’t require any external library.
Cons: The format may not be perfect for complex tables.
Method 2: Using TableExport.js Library
For a more advanced approach, you can use TableExport.js
, a JavaScript library that makes exporting tables easier.
Steps to Use TableExport.js
**Include the Library
Add the following script to your HTML page:**
Implement the Export Function
Pros: More customizable and supports different file formats.
Cons: Requires an external library.
Method 3: Export HTML Table to Excel Using PHP (CSV Format)
If you're working with a server-side application, you can export an HTML table using PHP by converting the data into a CSV file.
PHP Code to Export Table as CSV:
<?php
header('Content-Type: text/csv');
header('Content-Disposition: attachment; filename="table_data.csv"');
$output = fopen('php://output', 'w');
fputcsv($output, array('Name', 'Age', 'Country'));
$data = [
['John Doe', 28, 'USA'],
['Jane Smith', 34, 'UK']
];
foreach ($data as $row) {
fputcsv($output, $row);
}
fclose($output);
?>
Pros: Works well for dynamic web applications and databases.
Cons: Outputs CSV instead of Excel format.
Conclusion
Exporting an HTML table to Excel is a useful feature for data-driven applications. With either JavaScript, a third-party library, or PHP, each approach has its merits. Select the approach that best fits your project's requirements.
If this guide helped you, please forward it to your network and spread the word about how easily one can export HTML tables into Excel!
Know More >> https://scrapelead.io/blog/web-scraping-definition-meaning-key-insights/
Subscribe to my newsletter
Read articles from ScrapeLead directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ScrapeLead
ScrapeLead
Scrape Any Website and Connect With Your Popular Apps It’s easy to connect your data to thousands of apps, including Google Sheets and Airtable. You can utilize Zapier, http://scrapelead.io’s API, and more for smooth data sharing and integration across multiple platforms.