All about FUNCTIONS in JS

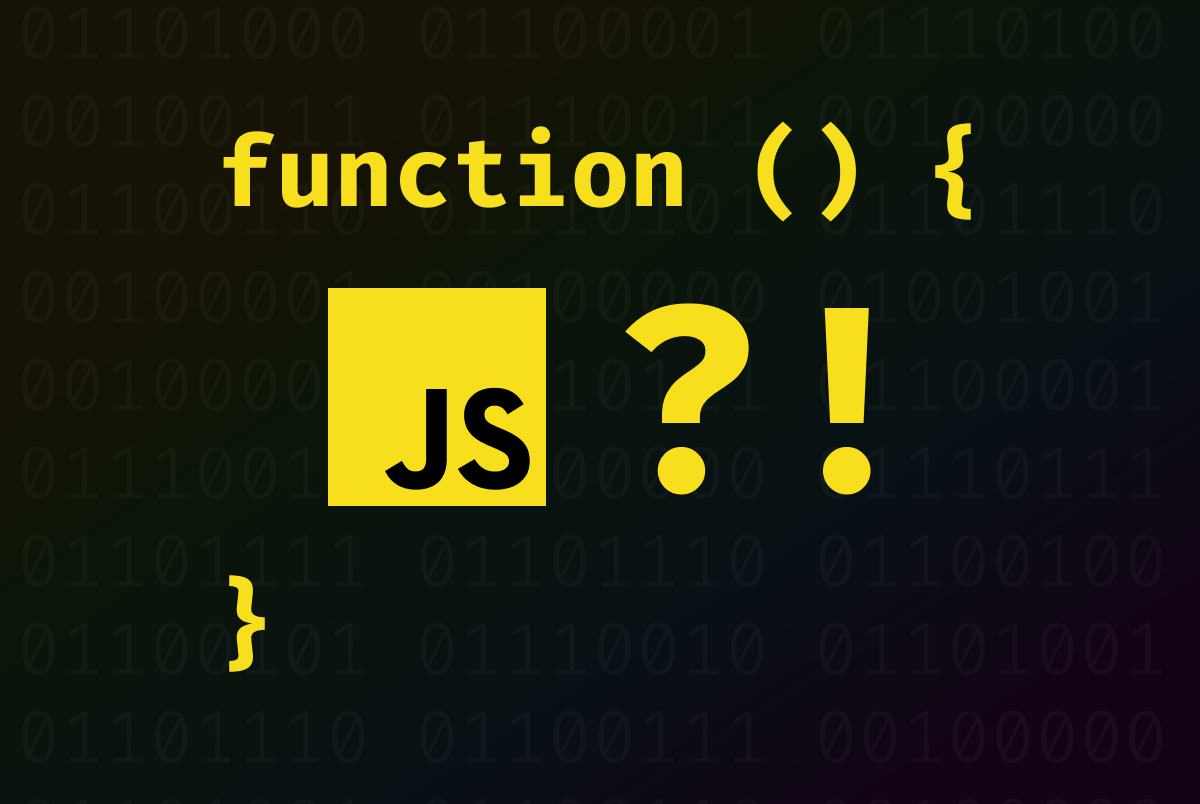
Functions in JavaScript
Functions are reusable blocks of code that perform a specific task when called. They help in making code modular, readable, and maintainable. JavaScript provides multiple ways to define functions, each serving different use cases.
Ways to Create a Function in JavaScript
1. Function Declaration (Named Function)
A function declaration is the most commonly used method to define a function in JavaScript. It consists of the function
keyword followed by the function name, parameters (if any), and a block of code inside {}
.
Example:
function greet() {
console.log("Hello, welcome to JavaScript!");
}
greet(); // Output: Hello, welcome to JavaScript!
Key Features:
✔ Hoisted: You can call the function before its declaration in the code.
✔ Reusable: Can be used multiple times by calling its name.
✔ Readable: The function name clearly describes what it does.
Hoisting Example:
Hello(); // This works even before the function is declared
function sayHello() {
console.log("Hello, this function is hoisted!");
}
Even though we called sayHello()
before defining it, JavaScript moves function declarations to the top during execution.
2. Function Expression (Anonymous Function)
A function expression involves storing a function inside a variable. These functions are anonymous (do not have a name) and can be invoked using the variable name.
Example:
const greet = function() {
console.log("Hello from function expression!");
};
greet(); // Output: Hello from function expression!
Key Features:
✔ Not Hoisted: Unlike function declarations, function expressions cannot be called before they are defined.
✔ Useful for Callbacks(click the link to learn about callbacks): Since they are assigned to variables, they can be easily passed as arguments to other functions.
Example of Function Expression in Callbacks:
setTimeout(function() {
console.log("Executed after 2 seconds");
}, 2000);
In this example, an anonymous function is passed to setTimeout
, which executes after 2 seconds.
3. Arrow Function (ES6)
Arrow functions provide a concise syntax for writing functions. They are best suited for small functions and do not have their own this
context.
Example:
const greet = () => {
console.log("Hello from arrow function!");
};
greet(); // Output: Hello from arrow function!
Key Features:
✔ Shorter Syntax: Reduces the amount of code needed to define a function.
✔ No this
Binding: Useful when working with this
inside objects or classes.
✔ Implicit Return: If the function has only one statement, it automatically returns the result without using {}
and return
.
Example of Implicit Return:
const add = (a, b) => a + b;
console.log(add(5, 3)); // Output: 8
Here, return
is implicitly used, making the function even more concise.
When NOT to Use Arrow Functions:
When you need
this
context (e.g., in event listeners or object methods).For defining object methods because
this
will refer to the outer scope.
const person = {
name: "Raveena",
greet: () => {
console.log("Hello, " + this.name); // `this` is undefined here!
}
};
person.greet(); // Output: Hello, undefined
In the above example, this
does not refer to person
because arrow functions do not have their own this
.
4. Immediately Invoked Function Expression (IIFE)
IIFE is a function that runs as soon as it is defined. It is useful for avoiding global variable pollution and for executing code that needs to run once.
Example:
(function() {
console.log("I am an IIFE!");
})(); // Output: I am an IIFE!
Key Features:
✔ Executes Immediately: No need to call it separately.
✔ Encapsulated Scope: Variables inside IIFE do not affect the global scope.
✔ Used for Initializing Code: Often used to set up configurations or one-time operations.
Example of IIFE with Parameters:
(function(name) {
console.log("Hello, " + name);
})("Raveena"); // Output: Hello, Raveena
This allows passing values to IIFE like regular functions.
5. Function with Parameters and Return Values
A function can accept parameters (inputs) and return a value to make it reusable for different cases.
Example:
function add(a, b) {
return a + b;
}
console.log(add(5, 3)); // Output: 8
Key Features:
✔ Reusable: Instead of writing 5 + 3
multiple times, you can call add(5, 3)
.
✔ Flexible: You can use different numbers without changing the function itself.
✔ Returns a Value: The function gives back a result that can be stored or used.
Example of Using Default Parameters:
function greet(name = "Guest") {
console.log("Hello, " + name);
}
greet(); // Output: Hello, Guest
greet("Raveena"); // Output: Hello, Raveena
If no argument is passed, "Guest"
is used as the default value.
Conclusion
JavaScript provides multiple ways to define functions, each with its own advantages:
Function Type | Description | Hoisted? | Best Used For |
Function Declaration | Traditional way to define named functions | ✅ Yes | General-purpose functions |
Function Expression | Function assigned to a variable (anonymous) | ❌ No | Callbacks, event handlers |
Arrow Function | Shorter syntax for functions (ES6) | ❌ No | Short functions, avoiding this |
IIFE | Executes immediately upon definition | ❌ No | Encapsulating code, one-time execution |
Function with Parameters | Accepts inputs and returns values | ✅ Yes | Reusable logic |
When to Use Which Function?
✔ Use function declarations when you need traditional, reusable functions.
✔ Use function expressions when you need anonymous functions or callbacks.
✔ Use arrow functions for short, one-line functions or when avoiding this
.
✔ Use IIFE when you want to execute a function immediately and prevent global variable pollution.
Important topics to know about
1. Pure Functions
Definition: A function that always produces the same output for the same input and has no side effects.
Example:
function add(a, b) { return a + b; } console.log(add(2, 3)); // Always returns 5
Key Characteristics:
No modification of external variables
Always returns a value
2. Impure Functions
Definition: A function that interacts with external data or produces side effects (modifies variables, logs data, makes API calls).
Example:
let count = 0; function increment() { count++; console.log(count); } increment(); // Modifies external variable (impure)
Why are they considered bad?
- They can lead to unpredictable behaviour and are harder to test.
3. Higher-Order Functions
Definition: A function that either takes another function as an argument or returns a function.
Example (Using
map
as a higher-order function):const numbers = [1, 2, 3, 4]; const doubled = numbers.map(num => num * 2); console.log(doubled); // [2, 4, 6, 8]
Example (Returning a function):
function multiplier(factor) { return function (num) { return num * factor; }; } const double = multiplier(2); console.log(double(5)); // 10
Benefits:
Increases code reusability
Makes functions more flexible
Subscribe to my newsletter
Read articles from Raveena Putta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
