Bash Loops and Conditionals

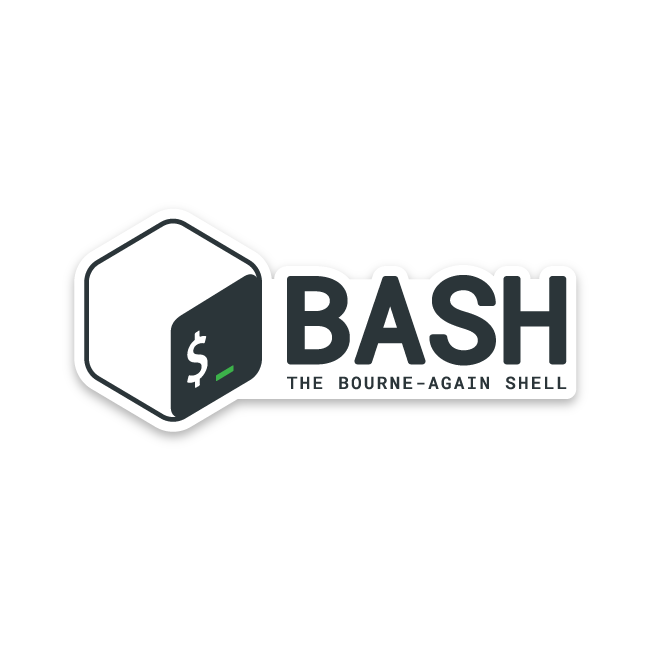
Bash, the default shell in many Linux distributions, offers powerful constructs for controlling program flow. This post focuses on two fundamental elements: if
statements for conditional execution and for
loops for iterative processing. Mastering these will significantly enhance your shell scripting abilities.
The if
Statement
The if
statement allows you to execute commands conditionally. Its basic structure involves a test condition followed by one or more commands that are executed if the condition evaluates to true. A simple example might look like this:
#!/bin/bash
number=10
if [ $number -gt 5 ]; then
echo "The number is greater than 5"
fi
This script checks if the variable number
is greater than 5. The -gt
operator performs the numerical comparison. If true, the message is printed. The fi
keyword marks the end of the if
block. More complex conditions can be created using elif
(else if) and else
clauses.
The for
Loop
for
loops are ideal when you need to execute a block of code multiple times. Bash offers several ways to use for
loops, including iterating over a sequence of numbers or a list of words. A common pattern iterates over a set of files:
#!/bin/bash
for file in *.txt; do
echo "Processing file: $file"
done
This script processes all files ending in .txt
in the current directory. Each filename is assigned to the variable file
in each iteration, and the done
keyword marks the end of the loop. Another way to use the for loop is by using an array:
#!/bin/bash
myArray=(
"apple"
"banana"
"cherry"
)
for i in "${myArray[@]}"; do
echo "item is: $i"
done
Output:
item is: apple
item is: banana
item is: cherry
Further Reading
To delve deeper, explore Bash's case
statement for multiple-condition decision-making and the while
and until
loops for conditions that are checked before and after each iteration, respectively. The Bash manual provides comprehensive documentation on these and other control flow structures.
Subscribe to my newsletter
Read articles from Hossein Margani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
