How Java Virtual Threads Handles Blocking Operations Efficiently

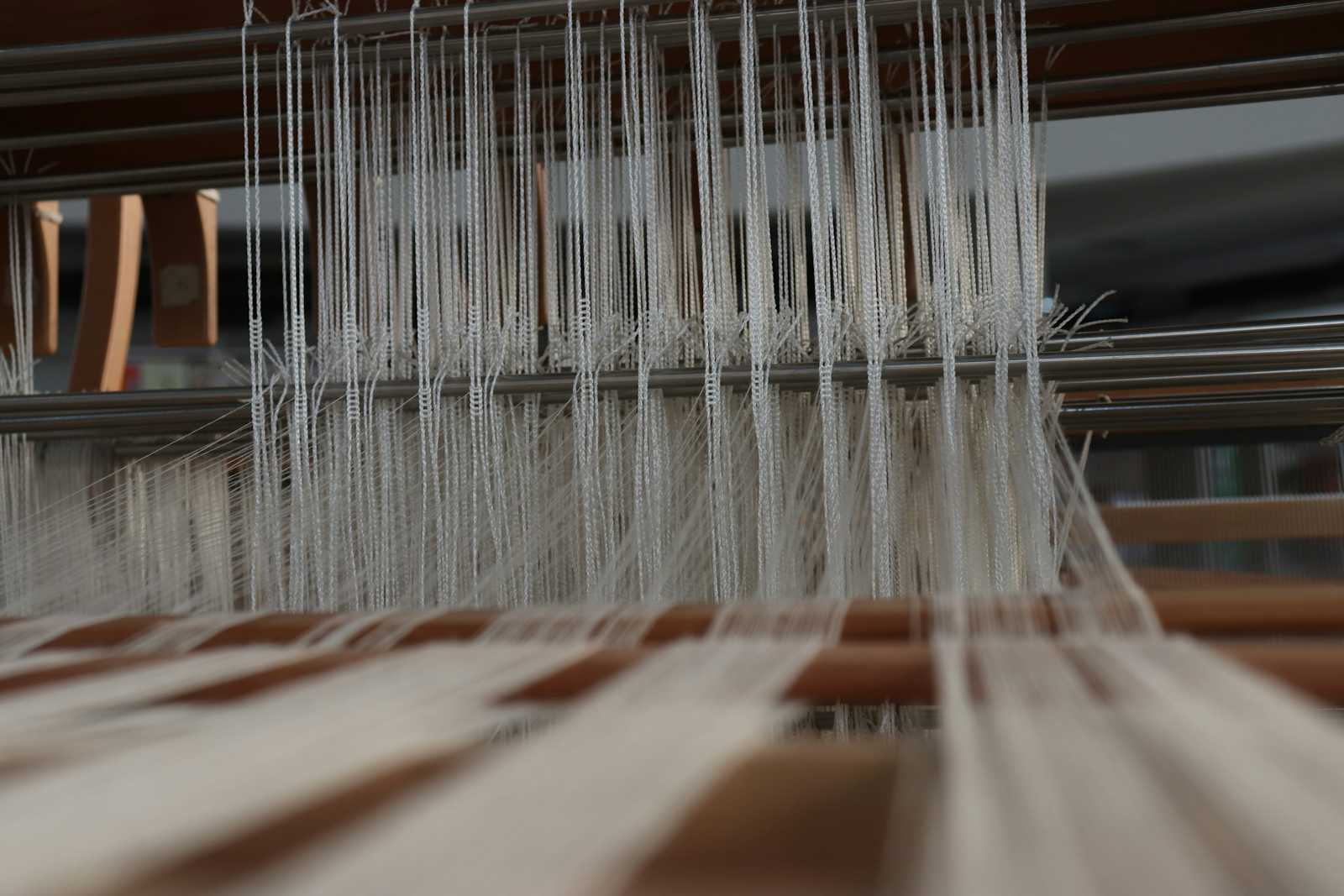
Java’s threading model has long been a cornerstone of its concurrency capabilities. However, the limitations of traditional platform threads (wrappers around OS threads) have persisted for decades—until the release of java 21. Virtual threads, represent a significant evolution in Java concurrency. Unlike traditional platform threads that are directly managed by the operating system (OS), virtual threads are lightweight threads handled by the Java runtime. This innovative approach dramatically improves scalability and reduces resource usage, allowing Java applications to handle numerous concurrent operations efficiently.
The Problem with Platform Threads
Traditional Java threads (platform threads) are expensive. Each thread consumes ~2MB of memory upfront and takes milliseconds to create. Operating systems limit the number of threads (often to a few thousand), making it impossible to handle millions of concurrent tasks. For I/O-heavy applications (e.g., web servers, databases), this creates a bottleneck:
CPU underutilization: A thread blocked on I/O (e.g., waiting for a database response) idles the CPU.
Complex async code: To scale, developers resort to asynchronous frameworks (e.g., CompletableFuture, reactive streams), which split logic into non-blocking callbacks. While efficient, this leads to complex, hard-to-maintain code.
How Java Handles Blocking Operations with Virtual Threads
When a virtual thread encounter a blocking opertation :
Unmount: The JVM unmounts the virtual thread from its carrier platform thread.
Yield Control: The virtual thread’s stack is moved to heap memory, freeing the platform thread to execute other tasks.
Resume: Once the I/O completes, the virtual thread is rescheduled onto any available platform thread.
But how is the yielding done ?
How can the JVM know that the thread is waiting for a blocking operation ?
One word : Continuation.
With the project Loom, all the java blocking operations are using the Continuation objects. Once a blocking operation is run, the Continuation.yield()
is called to unmount the virtual thread, storing its execution stack in heap memory. Once the blocking operation completes, Continuation.run()
is called, triggering the retrieving of the saved stack from the heap and remounting the virtual thread onto a platform thread, possibly a different one than before. This ensures the virtual thread resumes exactly from where it was suspended
The following diagram shows how java handles blocking operation (opening a file) with virtual threads
Virtual Threads Best Practices
When to Use Virtual Threads
Blocking Operations: Ideal for operations involving I/O (network calls, database queries, file operations).
High Concurrency: Suitable for applications requiring thousands or millions of concurrent threads due to their low resource overhead.
When Not to Use Virtual Threads
In-Memory Computations: Avoid using virtual threads for purely CPU-bound tasks as they introduce additional overhead compared to platform threads.
Native or Synchronized Blocks: Use cautiously with native calls or synchronized blocks, as virtual threads may become pinned to a platform thread, potentially reducing performance.
Conclusion
Java virtual threads provide a scalable and efficient approach to concurrency, particularly benefiting applications with numerous blocking operations. By effectively managing the mount, unmount, and remount lifecycle through Continuation objects, Java ensures optimal resource utilization and simpler, maintainable code. Virtual threads significantly enhance efficiency by preventing OS threads from idly waiting on I/O operations, thereby reducing overhead. Additionally, they greatly improve scalability by allowing thousands of virtual threads to be managed simultaneously without performance degradation. This model simplifies coding by enabling developers to write straightforward, synchronous code while the runtime handles concurrency intricacies seamlessly, leading to cleaner and more readable applications.
Subscribe to my newsletter
Read articles from MAADA Loukmane directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

MAADA Loukmane
MAADA Loukmane
Moroccan software developer, Java/Spring. Love to learn, eager to write.